
NuMaker-PFM-NUC472 : I2C1 LCD draw bitmap
Embed:
(wiki syntax)
Show/hide line numbers
ssd1306.cpp
00001 // SSD1306Z LCD Driver: 0.96" lcd LY096BG30 00002 #include "mbed.h" 00003 #include "ssd1306.h" 00004 #include "Font8x16.h" 00005 #include "Font5x7.h" 00006 00007 I2C ssd1306_i2c(PD_12, PD_10); // I2C1_SDA, I2C1_SCL 00008 00009 char DisplayBuffer[128*8]; 00010 00011 void lcdWriteCommand(uint8_t lcd_Command) 00012 { 00013 char data[2]; 00014 data[0]=0x00; 00015 data[1]=lcd_Command; 00016 ssd1306_i2c.write(SSD1306_slave_addr, data, 2, 0); 00017 } 00018 00019 void lcdWriteData(uint8_t lcd_Data) 00020 { 00021 char data[2]; 00022 data[0]=0x40; 00023 data[1]=lcd_Data; 00024 ssd1306_i2c.write(SSD1306_slave_addr, data, 2, 0); 00025 } 00026 00027 void lcdSetAddr(uint8_t column, uint8_t page) 00028 { 00029 lcdWriteCommand(0xb0+page); // set page address 00030 lcdWriteCommand(0x10 | ((column & 0xf0) >> 4)); // set column address MSB 00031 lcdWriteCommand(0x00 | (column & 0x0f) ); // set column address LSB 00032 } 00033 00034 void SSD1306::initialize(void) 00035 { 00036 lcdWriteCommand(0xae); //display off 00037 lcdWriteCommand(0x20); //Set Memory Addressing Mode 00038 lcdWriteCommand(0x10); //00,Horizontal Addressing Mode;01,Vertical Addressing Mode;10,Page Addressing Mode (RESET);11,Invalid 00039 lcdWriteCommand(0xb0); //Set Page Start Address for Page Addressing Mode,0-7 00040 lcdWriteCommand(0xc8); //Set COM Output Scan Direction 00041 lcdWriteCommand(0x00);//---set low column address 00042 lcdWriteCommand(0x10);//---set high column address 00043 lcdWriteCommand(0x40);//--set start line address 00044 lcdWriteCommand(0x81);//--set contrast control register 00045 lcdWriteCommand(0x7f); 00046 lcdWriteCommand(0xa1);//--set segment re-map 0 to 127 00047 lcdWriteCommand(0xa6);//--set normal display 00048 lcdWriteCommand(0xa8);//--set multiplex ratio(1 to 64) 00049 lcdWriteCommand(0x3F);// 00050 lcdWriteCommand(0xa4);//0xa4,Output follows RAM content;0xa5,Output ignores RAM content 00051 lcdWriteCommand(0xd3);//-set display offset 00052 lcdWriteCommand(0x00);//-not offset 00053 lcdWriteCommand(0xd5);//--set display clock divide ratio/oscillator frequency 00054 lcdWriteCommand(0xf0);//--set divide ratio 00055 lcdWriteCommand(0xd9);//--set pre-charge period 00056 lcdWriteCommand(0x22); // 00057 lcdWriteCommand(0xda);//--set com pins hardware configuration 00058 lcdWriteCommand(0x12); 00059 lcdWriteCommand(0xdb);//--set vcomh 00060 lcdWriteCommand(0x20);//0x20,0.77xVcc 00061 lcdWriteCommand(0x8d);//--set DC-DC enable 00062 lcdWriteCommand(0x14);// 00063 lcdWriteCommand(0xaf);//--turn on lcd panel 00064 } 00065 00066 void SSD1306::clearscreen(void) 00067 { 00068 int16_t x, Y; 00069 for (Y=0;Y<LCD_Ymax/8;Y++) 00070 { 00071 lcdSetAddr(0, Y); 00072 for (x=0;x<LCD_Xmax;x++) 00073 lcdWriteData(0x00); 00074 } 00075 } 00076 00077 // print char function using Font5x7 00078 void SSD1306::printC_5x7 (int x, int y, unsigned char ascii_code) 00079 { 00080 int8_t i; 00081 if (x<(LCD_Xmax-5) && y<(LCD_Ymax-7)) { 00082 if (ascii_code<0x20) ascii_code=0x20; 00083 else if (ascii_code>0x7F) ascii_code=0x20; 00084 for (i=0;i<5;i++) { 00085 lcdSetAddr((x+i), (y/8)); 00086 lcdWriteData(Font5x7[(ascii_code-0x20)*5+i]); 00087 } 00088 } 00089 } 00090 00091 void SSD1306::printC(int Line, int Col, unsigned char ascii_code) 00092 { 00093 uint8_t j, i, tmp; 00094 for (j=0;j<2;j++) { 00095 lcdSetAddr(Col*8, Line*2+j); 00096 for (i=0;i<8;i++) { 00097 tmp=Font8x16[(ascii_code-0x20)*16+j*8+i]; 00098 lcdWriteData(tmp); 00099 } 00100 } 00101 } 00102 00103 void SSD1306::printLine(int line, char text[]) 00104 { 00105 uint8_t Col; 00106 for (Col=0; Col<strlen(text); Col++) 00107 printC(line, Col, text[Col]); 00108 } 00109 00110 void SSD1306::printS(int x, int y, char text[]) 00111 { 00112 int8_t i; 00113 for (i=0;i<strlen(text);i++) 00114 printC(x+i*8, y,text[i]); 00115 } 00116 00117 void SSD1306::printS_5x7(int x, int y, char text[]) 00118 { 00119 int8_t i; 00120 for (i=0;i<strlen(text);i++) { 00121 printC_5x7(x,y,text[i]); 00122 x=x+5; 00123 } 00124 } 00125 00126 void SSD1306::drawPixel(int x, int y, int fgColor, int bgColor) 00127 { 00128 if (fgColor!=0) 00129 DisplayBuffer[x+y/8*LCD_Xmax] |= (0x01<<(y%8)); 00130 else 00131 DisplayBuffer[x+y/8*LCD_Xmax] &= (0xFE<<(y%8)); 00132 00133 lcdSetAddr(x, y/8); 00134 lcdWriteData(DisplayBuffer[x+y/8*LCD_Xmax]); 00135 } 00136 00137 void SSD1306::drawBmp8x8(int x, int y, int fgColor, int bgColor, unsigned char bitmap[]) 00138 { 00139 uint8_t t,i,k, kx,ky; 00140 if (x<(LCD_Xmax-7) && y<(LCD_Ymax-7)) // boundary check 00141 for (i=0;i<8;i++){ 00142 kx=x+i; 00143 t=bitmap[i]; 00144 for (k=0;k<8;k++) { 00145 ky=y+k; 00146 if (t&(0x01<<k)) drawPixel(kx,ky,fgColor,bgColor); 00147 } 00148 } 00149 } 00150 00151 void SSD1306::drawBmp32x8(int x, int y, int fgColor, int bgColor, unsigned char bitmap[]) 00152 { 00153 uint8_t t,i,k, kx,ky; 00154 if (x<(LCD_Xmax-7) && y<(LCD_Ymax-7)) // boundary check 00155 for (i=0;i<32;i++){ 00156 kx=x+i; 00157 t=bitmap[i]; 00158 for (k=0;k<8;k++) { 00159 ky=y+k; 00160 if (t&(0x01<<k)) drawPixel(kx,ky,fgColor,bgColor); 00161 } 00162 } 00163 } 00164 00165 void SSD1306::drawBmp120x8(int x, int y, int fgColor, int bgColor, unsigned char bitmap[]) 00166 { 00167 uint8_t t,i,k, kx,ky; 00168 if (x<(LCD_Xmax-7) && y<(LCD_Ymax-7)) // boundary check 00169 for (i=0;i<120;i++){ 00170 kx=x+i; 00171 t=bitmap[i]; 00172 for (k=0;k<8;k++) { 00173 ky=y+k; 00174 if (t&(0x01<<k)) drawPixel(kx,ky,fgColor,bgColor); 00175 } 00176 } 00177 } 00178 00179 void SSD1306::drawBmp8x16(int x, int y, int fgColor, int bgColor, unsigned char bitmap[]) 00180 { 00181 uint8_t t,i,k, kx,ky; 00182 if (x<(LCD_Xmax-7) && y<(LCD_Ymax-7)) // boundary check 00183 for (i=0;i<8;i++){ 00184 kx=x+i; 00185 t=bitmap[i]; 00186 for (k=0;k<8;k++) { 00187 ky=y+k; 00188 if (t&(0x01<<k)) drawPixel(kx,ky,fgColor,bgColor); 00189 } 00190 t=bitmap[i+8]; 00191 for (k=0;k<8;k++) { 00192 ky=y+k+8; 00193 if (t&(0x01<<k)) drawPixel(kx,ky,fgColor,bgColor); 00194 } 00195 } 00196 } 00197 00198 void SSD1306::drawBmp16x8(int x, int y, int fgColor, int bgColor, unsigned char bitmap[]) 00199 { 00200 uint8_t t,i,k,kx,ky; 00201 if (x<(LCD_Xmax-15) && y<(LCD_Ymax-7)) // boundary check 00202 for (i=0;i<16;i++) 00203 { 00204 kx=x+i; 00205 t=bitmap[i]; 00206 for (k=0;k<8;k++) { 00207 ky=y+k; 00208 if (t&(0x01<<k)) drawPixel(kx,ky,fgColor,bgColor); 00209 } 00210 } 00211 } 00212 00213 void SSD1306::drawBmp16x16(int x, int y, int fgColor, int bgColor, unsigned char bitmap[]) 00214 { 00215 uint8_t t,i,j,k, kx,ky; 00216 if (x<(LCD_Xmax-15) && y<(LCD_Ymax-15)) // boundary check 00217 for (j=0;j<2; j++){ 00218 for (i=0;i<16;i++) { 00219 kx=x+i; 00220 t=bitmap[i+j*16]; 00221 for (k=0;k<8;k++) { 00222 ky=y+j*8+k; 00223 if (t&(0x01<<k)) drawPixel(kx,ky,fgColor,bgColor); 00224 } 00225 } 00226 } 00227 } 00228 00229 void SSD1306::drawBmp16x24(int x, int y, int fgColor, int bgColor, unsigned char bitmap[]) 00230 { 00231 uint8_t t,i,j,k, kx,ky; 00232 if (x<(LCD_Xmax-15) && y<(LCD_Ymax-15)) // boundary check 00233 for (j=0;j<3; j++){ 00234 for (i=0;i<16;i++) { 00235 kx=x+i; 00236 t=bitmap[i+j*16]; 00237 for (k=0;k<8;k++) { 00238 ky=y+j*8+k; 00239 if (t&(0x01<<k)) drawPixel(kx,ky,fgColor,bgColor); 00240 } 00241 } 00242 } 00243 } 00244 00245 void SSD1306::drawBmp16x32(int x, int y, int fgColor, int bgColor, unsigned char bitmap[]) 00246 { 00247 uint8_t t, i,j,k, kx,ky; 00248 if (x<(LCD_Xmax-15) && y<(LCD_Ymax-31)) // boundary check 00249 for (j=0;j<4; j++) { 00250 for (i=0;i<16;i++) { 00251 kx=x+i; 00252 t=bitmap[i+j*16]; 00253 for (k=0;k<8;k++) { 00254 ky=y+j*8+k; 00255 if (t&(0x01<<k)) drawPixel(kx,ky,fgColor,bgColor); 00256 } 00257 } 00258 } 00259 } 00260 00261 void SSD1306::drawBmp16x40(int x, int y, int fgColor, int bgColor, unsigned char bitmap[]) 00262 { 00263 uint8_t t, i,j,k, kx,ky; 00264 if (x<(LCD_Xmax-15) && y<(LCD_Ymax-31)) // boundary check 00265 for (j=0;j<5; j++) { 00266 for (i=0;i<16;i++) { 00267 kx=x+i; 00268 t=bitmap[i+j*16]; 00269 for (k=0;k<8;k++) { 00270 ky=y+j*8+k; 00271 if (t&(0x01<<k)) drawPixel(kx,ky,fgColor,bgColor); 00272 } 00273 } 00274 } 00275 } 00276 00277 void SSD1306::drawBmp16x48(int x, int y, int fgColor, int bgColor, unsigned char bitmap[]) 00278 { 00279 uint8_t t,i,j,k,kx,ky; 00280 if (x<(LCD_Xmax-15) && y<(LCD_Ymax-47)) // boundary check 00281 for (j=0;j<6; j++) { 00282 k=x; 00283 for (i=0;i<16;i++) { 00284 kx=x+i; 00285 t=bitmap[i+j*16]; 00286 for (k=0;k<8;k++) { 00287 ky=y+j*8+k; 00288 if (t&(0x01<<k)) drawPixel(kx,ky,fgColor,bgColor); 00289 } 00290 } 00291 } 00292 } 00293 00294 void SSD1306::drawBmp16x64(int x, int y, int fgColor, int bgColor, unsigned char bitmap[]) 00295 { 00296 uint8_t t,i,j,k,kx,ky; 00297 if (x<(LCD_Xmax-15) && y==0) // boundary check 00298 for (j=0;j<8; j++) { 00299 k=x; 00300 for (i=0;i<16;i++) { 00301 kx=x+i; 00302 t=bitmap[i+j*16]; 00303 for (k=0;k<8;k++) { 00304 ky=y+j*8+k; 00305 if (t&(0x01<<k)) drawPixel(kx,ky,fgColor,bgColor); 00306 } 00307 } 00308 } 00309 } 00310 00311 void SSD1306::drawBmp32x16(int x, int y, int fgColor, int bgColor, unsigned char bitmap[]) 00312 { 00313 uint8_t t,i,jx,jy,k,kx,ky; 00314 if (x<(LCD_Xmax-31) && y<(LCD_Ymax-15)) // boundary check 00315 for (jy=0;jy<2;jy++) 00316 for (jx=0;jx<2;jx++) { 00317 k=x; 00318 for (i=0;i<16;i++) { 00319 kx=x+jx*16+i; 00320 t=bitmap[i+jx*16+jy*32]; 00321 for (k=0;k<8;k++) { 00322 ky=y+jy*8+k; 00323 if (t&(0x01<<k)) drawPixel(kx,ky,fgColor,bgColor); 00324 } 00325 } 00326 } 00327 } 00328 00329 void SSD1306::drawBmp32x32(int x, int y, int fgColor, int bgColor, unsigned char bitmap[]) 00330 { 00331 uint8_t t,i,jx,jy,k, kx,ky; 00332 if (x<(LCD_Xmax-31) && y<(LCD_Ymax-31)) // boundary check 00333 for (jy=0;jy<4;jy++) 00334 for (jx=0;jx<2;jx++) { 00335 k=x; 00336 for (i=0;i<16;i++) { 00337 kx=x+jx*16+i; 00338 t=bitmap[i+jx*16+jy*32]; 00339 for (k=0;k<8;k++) { 00340 ky=y+jy*8+k; 00341 if (t&(0x01<<k)) drawPixel(kx,ky,fgColor,bgColor); 00342 } 00343 } 00344 } 00345 } 00346 00347 void SSD1306::drawBmp32x48(int x, int y, int fgColor, int bgColor, unsigned char bitmap[]) 00348 { 00349 uint8_t t,i,jx,jy,k, kx,ky; 00350 if (x<(LCD_Xmax-31) && y<(LCD_Ymax-47)) // boundary check 00351 for (jy=0;jy<6;jy++) 00352 for (jx=0;jx<2;jx++) { 00353 k=x; 00354 for (i=0;i<16;i++) { 00355 kx=x+jx*16+i; 00356 t=bitmap[i+jx*16+jy*32]; 00357 for (k=0;k<8;k++) { 00358 ky=y+jy*8+k; 00359 if (t&(0x01<<k)) drawPixel(kx,ky,fgColor,bgColor); 00360 } 00361 } 00362 } 00363 } 00364 00365 void SSD1306::drawBmp32x64(int x, int y, int fgColor, int bgColor, unsigned char bitmap[]) 00366 { 00367 uint8_t t,i,jx,jy,k, kx,ky; 00368 if (x<(LCD_Xmax-31) && y==0) // boundary check 00369 for (jy=0;jy<8;jy++) 00370 for (jx=0;jx<2;jx++) { 00371 k=x; 00372 for (i=0;i<16;i++) { 00373 kx=x+jx*16+i; 00374 t=bitmap[i+jx*16+jy*32]; 00375 for (k=0;k<8;k++) { 00376 ky=y+jy*8+k; 00377 if (t&(0x01<<k)) drawPixel(kx,ky,fgColor,bgColor); 00378 } 00379 } 00380 } 00381 } 00382 00383 void SSD1306::drawBmp64x64(int x, int y, int fgColor, int bgColor, unsigned char bitmap[]) 00384 { 00385 uint8_t t, i,jx,jy,k, kx,ky; 00386 if (x<(LCD_Xmax-63) && y==0) // boundary check 00387 for (jy=0;jy<8;jy++) 00388 for (jx=0;jx<4;jx++) { 00389 k=x; 00390 for (i=0;i<16;i++) { 00391 kx=x+jx*16+i; 00392 t=bitmap[i+jx*16+jy*64]; 00393 for (k=0;k<8;k++) { 00394 ky=y+jy*8+k; 00395 if (t&(0x01<<k)) drawPixel(kx,ky,fgColor,bgColor); 00396 } 00397 } 00398 } 00399 } 00400 00401 void SSD1306::drawBMP(unsigned char *buffer) 00402 { 00403 uint8_t x,y; 00404 for (x=0; x<LCD_Xmax; x++) { 00405 for (y=0; y<(LCD_Ymax/8); y++) { 00406 lcdSetAddr(x ,y); 00407 lcdWriteData(buffer[x+y*LCD_Xmax]); 00408 } 00409 } 00410 } 00411
Generated on Mon Aug 1 2022 23:52:47 by
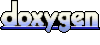