
NuMaker Cellular(NB-IoT/4G)
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /* 00002 * Copyright (c) 2017 ARM Limited. All rights reserved. 00003 * SPDX-License-Identifier: Apache-2.0 00004 * Licensed under the Apache License, Version 2.0 (the License); you may 00005 * not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00012 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include "mbed.h" 00018 #include "common_functions.h" 00019 #include "UDPSocket.h" 00020 #include "CellularLog.h" 00021 00022 #define UDP 0 00023 #define TCP 1 00024 00025 // Number of retries / 00026 #define RETRY_COUNT 3 00027 00028 NetworkInterface *iface; 00029 00030 // Echo server hostname 00031 const char *host_name = MBED_CONF_APP_ECHO_SERVER_HOSTNAME; 00032 00033 // Echo server port (same for TCP and UDP) 00034 const int port = MBED_CONF_APP_ECHO_SERVER_PORT; 00035 00036 static rtos::Mutex trace_mutex; 00037 00038 #if MBED_CONF_MBED_TRACE_ENABLE 00039 static void trace_wait() 00040 { 00041 trace_mutex.lock(); 00042 } 00043 00044 static void trace_release() 00045 { 00046 trace_mutex.unlock(); 00047 } 00048 00049 static char time_st[50]; 00050 00051 static char* trace_time(size_t ss) 00052 { 00053 snprintf(time_st, 49, "[%08llums]", Kernel::get_ms_count()); 00054 return time_st; 00055 } 00056 00057 static void trace_open() 00058 { 00059 mbed_trace_init(); 00060 mbed_trace_prefix_function_set( &trace_time ); 00061 00062 mbed_trace_mutex_wait_function_set(trace_wait); 00063 mbed_trace_mutex_release_function_set(trace_release); 00064 00065 mbed_cellular_trace::mutex_wait_function_set(trace_wait); 00066 mbed_cellular_trace::mutex_release_function_set(trace_release); 00067 } 00068 00069 static void trace_close() 00070 { 00071 mbed_cellular_trace::mutex_wait_function_set(NULL); 00072 mbed_cellular_trace::mutex_release_function_set(NULL); 00073 00074 mbed_trace_free(); 00075 } 00076 #endif // #if MBED_CONF_MBED_TRACE_ENABLE 00077 00078 Thread dot_thread(osPriorityNormal, 512); 00079 00080 void print_function(const char *format, ...) 00081 { 00082 trace_mutex.lock(); 00083 va_list arglist; 00084 va_start( arglist, format ); 00085 vprintf(format, arglist); 00086 va_end( arglist ); 00087 trace_mutex.unlock(); 00088 } 00089 00090 void dot_event() 00091 { 00092 while (true) { 00093 ThisThread::sleep_for(4000); 00094 if (iface && iface->get_connection_status() == NSAPI_STATUS_GLOBAL_UP) { 00095 break; 00096 } else { 00097 trace_mutex.lock(); 00098 printf("."); 00099 fflush(stdout); 00100 trace_mutex.unlock(); 00101 } 00102 } 00103 } 00104 00105 /** 00106 * Connects to the Cellular Network 00107 */ 00108 nsapi_error_t do_connect() 00109 { 00110 nsapi_error_t retcode = NSAPI_ERROR_OK; 00111 uint8_t retry_counter = 0; 00112 00113 while (iface->get_connection_status() != NSAPI_STATUS_GLOBAL_UP) { 00114 retcode = iface->connect(); 00115 if (retcode == NSAPI_ERROR_AUTH_FAILURE) { 00116 print_function("\n\nAuthentication Failure. Exiting application\n"); 00117 } else if (retcode == NSAPI_ERROR_OK) { 00118 print_function("\n\nConnection Established.\n"); 00119 } else if (retry_counter > RETRY_COUNT) { 00120 print_function("\n\nFatal connection failure: %d\n", retcode); 00121 } else { 00122 print_function("\n\nCouldn't connect: %d, will retry\n", retcode); 00123 retry_counter++; 00124 continue; 00125 } 00126 break; 00127 } 00128 return retcode; 00129 } 00130 00131 /** 00132 * Opens a UDP or a TCP socket with the given echo server and performs an echo 00133 * transaction retrieving current. 00134 */ 00135 nsapi_error_t test_send_recv() 00136 { 00137 nsapi_size_or_error_t retcode; 00138 #if MBED_CONF_APP_SOCK_TYPE == TCP 00139 TCPSocket sock; 00140 #else 00141 UDPSocket sock; 00142 #endif 00143 00144 retcode = sock.open(iface); 00145 if (retcode != NSAPI_ERROR_OK) { 00146 #if MBED_CONF_APP_SOCK_TYPE == TCP 00147 print_function("TCPSocket.open() fails, code: %d\n", retcode); 00148 #else 00149 print_function("UDPSocket.open() fails, code: %d\n", retcode); 00150 #endif 00151 return -1; 00152 } 00153 00154 SocketAddress sock_addr; 00155 retcode = iface->gethostbyname(host_name, &sock_addr); 00156 if (retcode != NSAPI_ERROR_OK) { 00157 print_function("Couldn't resolve remote host: %s, code: %d\n", host_name, retcode); 00158 return -1; 00159 } 00160 00161 sock_addr.set_port(port); 00162 00163 sock.set_timeout(15000); 00164 int n = 0; 00165 const char *echo_string = "TEST"; 00166 char recv_buf[4]; 00167 #if MBED_CONF_APP_SOCK_TYPE == TCP 00168 retcode = sock.connect(sock_addr); 00169 if (retcode < 0) { 00170 print_function("TCPSocket.connect() fails, code: %d\n", retcode); 00171 return -1; 00172 } else { 00173 print_function("TCP: connected with %s server\n", host_name); 00174 } 00175 retcode = sock.send((void*) echo_string, sizeof(echo_string)); 00176 if (retcode < 0) { 00177 print_function("TCPSocket.send() fails, code: %d\n", retcode); 00178 return -1; 00179 } else { 00180 print_function("TCP: Sent %d Bytes to %s\n", retcode, host_name); 00181 } 00182 00183 n = sock.recv((void*) recv_buf, sizeof(recv_buf)); 00184 #else 00185 00186 retcode = sock.sendto(sock_addr, (void*) echo_string, sizeof(echo_string)); 00187 if (retcode < 0) { 00188 print_function("UDPSocket.sendto() fails, code: %d\n", retcode); 00189 return -1; 00190 } else { 00191 print_function("UDP: Sent %d Bytes to %s\n", retcode, host_name); 00192 } 00193 00194 n = sock.recvfrom(&sock_addr, (void*) recv_buf, sizeof(recv_buf)); 00195 #endif 00196 00197 sock.close(); 00198 00199 if (n > 0) { 00200 print_function("Received from echo server %d Bytes\n", n); 00201 return 0; 00202 } 00203 00204 return -1; 00205 } 00206 00207 int main() 00208 { 00209 #ifdef MBED_MAJOR_VERSION 00210 printf("Mbed OS version %d.%d.%d\r\n\n", MBED_MAJOR_VERSION, MBED_MINOR_VERSION, MBED_PATCH_VERSION); 00211 #endif 00212 print_function("\n\nmbed-os-example-cellular\n"); 00213 print_function("\n\nBuilt: %s, %s\n", __DATE__, __TIME__); 00214 #ifdef MBED_CONF_NSAPI_DEFAULT_CELLULAR_PLMN 00215 print_function("\n\n[MAIN], plmn: %d\n", MBED_CONF_NSAPI_DEFAULT_CELLULAR_PLMN); 00216 #endif 00217 00218 print_function("Establishing connection\n"); 00219 #if MBED_CONF_MBED_TRACE_ENABLE 00220 trace_open(); 00221 #else 00222 dot_thread.start(dot_event); 00223 #endif // #if MBED_CONF_MBED_TRACE_ENABLE 00224 00225 // sim pin, apn, credentials and possible plmn are taken atuomtically from json when using get_default_instance() 00226 iface = NetworkInterface::get_default_instance(); 00227 MBED_ASSERT(iface); 00228 00229 nsapi_error_t retcode = NSAPI_ERROR_NO_CONNECTION; 00230 00231 /* Attempt to connect to a cellular network */ 00232 if (do_connect() == NSAPI_ERROR_OK) { 00233 SocketAddress a; 00234 iface->get_ip_address(&a); 00235 printf("IP Address is %s\r\n", a.get_ip_address()); 00236 // printf("IP Address is %s\r\n", iface->get_ip_address()); 00237 retcode = test_send_recv(); 00238 } 00239 00240 if (iface->disconnect() != NSAPI_ERROR_OK) { 00241 print_function("\n\n disconnect failed.\n\n"); 00242 } 00243 00244 if (retcode == NSAPI_ERROR_OK) { 00245 print_function("\n\nSuccess. Exiting \n\n"); 00246 } else { 00247 print_function("\n\nFailure. Exiting \n\n"); 00248 } 00249 00250 #if MBED_CONF_MBED_TRACE_ENABLE 00251 trace_close(); 00252 #else 00253 dot_thread.terminate(); 00254 #endif // #if MBED_CONF_MBED_TRACE_ENABLE 00255 00256 return 0; 00257 } 00258 // EOF
Generated on Wed Mar 1 2023 09:07:03 by
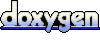