Zeitsteuerung
Fork of timer0 by
Embed:
(wiki syntax)
Show/hide line numbers
timer0.cpp
00001 #include "mbed.h" 00002 #include "timer0.h" 00003 00004 00005 //-------------------------------------------------------- 00006 // Construktor initialisiert den Timer 00007 timer0::timer0() 00008 { 00009 uint8_t i; 00010 00011 ms = 0; 00012 sec = 0; 00013 min = 0; 00014 hours = 0; 00015 00016 // Initialize countdown timers 00017 for (i=0; i < TIMER0_NUM_COUNTDOWNTIMERS; i++) 00018 CountDownTimers[i].status = 0xFF; 00019 00020 ticker.attach_us(this, &timer0::func, 1000); 00021 } 00022 00023 //-------------------------------------------------------- 00024 // Interruptroutine wird jede 1000µs aufgerufen, da das Senden von 00025 // einem Zeichen ca. 100µs Zeit beansprucht 00026 00027 void timer0::func(void) 00028 { 00029 uint8_t i; 00030 00031 // Zeitbasis für Systemzeit 00032 // ----- count down timers in ms ------------------------------------------------- 00033 for (i=0; i<TIMER0_NUM_COUNTDOWNTIMERS; i++) 00034 { 00035 if (CountDownTimers[i].status == 1) 00036 { // ms 00037 if (CountDownTimers[i].count_timer > 0) 00038 CountDownTimers[i].count_timer -- ; 00039 if (CountDownTimers[i].count_timer == 0) 00040 CountDownTimers[i].status = 0; 00041 } 00042 } 00043 00044 if (ms < 1000) 00045 { 00046 ms++; 00047 } 00048 else 00049 { 00050 ms = 0; 00051 00052 seconds++; 00053 set_time(seconds); 00054 00055 // ----- count down timers in seconds ------------------------------------------------- 00056 00057 for (i=0; i<TIMER0_NUM_COUNTDOWNTIMERS; i++) 00058 { 00059 if (CountDownTimers[i].status == 2) 00060 { // sekunden 00061 if (CountDownTimers[i].count_timer > 0) 00062 CountDownTimers[i].count_timer -- ; 00063 if (CountDownTimers[i].count_timer == 0) 00064 CountDownTimers[i].status = 0; 00065 } 00066 } 00067 00068 if (sec < 60) 00069 { 00070 sec++; 00071 } 00072 else 00073 { 00074 sec = 0; 00075 00076 // ----- count down timers in minuts ------------------------------------------------- 00077 00078 for (i=0; i<TIMER0_NUM_COUNTDOWNTIMERS; i++) 00079 { 00080 if (CountDownTimers[i].status == 3) 00081 { // sekunden 00082 if (CountDownTimers[i].count_timer > 0) 00083 CountDownTimers[i].count_timer -- ; 00084 if (CountDownTimers[i].count_timer == 0) 00085 CountDownTimers[i].status = 0; 00086 } 00087 } 00088 00089 if (min < 60) min++; 00090 else 00091 { 00092 min = 0; 00093 if (hours < 24) hours++; 00094 else hours = 0; 00095 } 00096 } 00097 } 00098 00099 } 00100 00101 00102 //-------------------------------------------------------- 00103 // Abfrage der Softwareuhr 00104 // 00105 // die Stunden, Minuten und Sekungen werden in einem Array zurückgegeben 00106 // die millisekunden sind separat 00107 00108 void timer0::get_time_stamp(uint8_t *tarray,uint16_t *millis) 00109 { 00110 *millis = ms; 00111 *tarray = hours; 00112 tarray++; 00113 *tarray = min; 00114 tarray++; 00115 *tarray = sec; 00116 } 00117 00118 //-------------------------------------------------------- 00119 // Abfrage nach freiem Timer 00120 // 00121 // wenn alle Timer belegt sind wird 0xFF zurückgegebne 00122 00123 uint8_t timer0::AllocateCountdownTimer (void) 00124 { 00125 uint8_t i; 00126 00127 for (i=0; i<TIMER0_NUM_COUNTDOWNTIMERS; i++) 00128 if (CountDownTimers[i].status == 0xFF) 00129 { 00130 CountDownTimers[i].status = 0x00; // Timer reserviert, nicht gestartet 00131 // printf_P(PSTR("\rallocate timer [%03d] %d\n"),i,ListPointer); 00132 00133 return i; 00134 } 00135 00136 return 0xFF; 00137 } 00138 00139 //-------------------------------------------------------- 00140 // Timer wieder freigeben 00141 void timer0::RemoveCountdownTimer(uint8_t timer) 00142 { 00143 CountDownTimers[timer].status = 0xFF; 00144 } 00145 00146 //-------------------------------------------------------- 00147 // Abfrage ob Timer 0 erreicht hat 00148 uint8_t timer0::GetTimerStatus(uint8_t timer) 00149 { 00150 return CountDownTimers[timer].status; 00151 } 00152 00153 //-------------------------------------------------------- 00154 // Abfrage der verbleibenden Zeit 00155 uint16_t timer0::GetTimerZeit(uint8_t timer) 00156 { 00157 return CountDownTimers[timer].count_timer; 00158 } 00159 00160 //-------------------------------------------------------- 00161 // Timer aktivieren 00162 void timer0::SetCountdownTimer(unsigned char timer, unsigned char status, unsigned short value) 00163 { 00164 CountDownTimers[timer].count_timer = value; 00165 CountDownTimers[timer].status = status; 00166 } 00167 00168 //-------------------------------------------------------- 00169 // Zeitstempel setzen 00170 void timer0::Set_t(uint8_t * data) 00171 { 00172 char buffer[40]; 00173 00174 t.tm_sec = data[0]; // Sekunden 0-59 00175 t.tm_min = data[1]; // Minuten 0-59 00176 t.tm_hour = data[2]; // Stunden 0-23 00177 t.tm_mday = data[3]; // Tag 1-31 00178 t.tm_mon = data[4] - 1; // Monat 0-11 0 = Januar 00179 t.tm_year = data[5]; // Jahr year since 1900 00180 00181 seconds = mktime(&t); 00182 set_time(seconds); 00183 00184 strftime(buffer, 40, "%a,%d.%m.%Y %H:%M:%S", localtime(&seconds)); 00185 // pc.printf("\ntimer0::set_t = %s", buffer); 00186 }
Generated on Sat Jul 16 2022 07:02:00 by
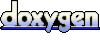