
Example program for the LWIPInterface
Dependencies: LWIPInterface NetworkSocketAPI mbed-rtos mbed
Fork of HelloESP8266Interface by
main.cpp
00001 /* NetworkSocketAPI Example Program 00002 * Copyright (c) 2015 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include "mbed.h" 00018 #include "LWIPInterface.h" 00019 #include "TCPSocket.h" 00020 00021 LWIPInterface eth; 00022 00023 DigitalOut led(LED_GREEN); 00024 void blink() 00025 { 00026 led = !led; 00027 } 00028 00029 int main() 00030 { 00031 Ticker blinky; 00032 blinky.attach(blink, 0.4f); 00033 00034 printf("NetworkSocketAPI Example\r\n"); 00035 00036 eth.connect(); 00037 const char *ip = eth.get_ip_address(); 00038 const char *mac = eth.get_mac_address(); 00039 printf("IP address is: %s\r\n", ip ? ip : "No IP"); 00040 printf("MAC address is: %s\r\n", mac ? mac : "No MAC"); 00041 00042 SocketAddress addr(ð, "mbed.org"); 00043 printf("mbed.org resolved to: %s\r\n", addr.get_ip_address()); 00044 00045 TCPSocket socket(ð); 00046 socket.connect("4.ifcfg.me", 23); 00047 00048 char buffer[64]; 00049 int count = socket.recv(buffer, sizeof buffer); 00050 printf("public IP address is: %.15s\r\n", &buffer[15]); 00051 00052 socket.close(); 00053 eth.disconnect(); 00054 00055 printf("Done\r\n"); 00056 }
Generated on Wed Jul 13 2022 15:59:22 by
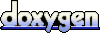