Driver library for the Freescale FXOS8700Q sensor
Dependencies: MotionSensor
Dependents: el14dg_Project frdm_serial_peopleAndComputing simple-client-app-shield pelion-example-frdm ... more
Fork of FXOS8700Q by
FXOS8700Q.cpp
00001 /* FXOS8700Q sensor driver 00002 * Copyright (c) 2014-2015 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include "FXOS8700Q.h" 00018 00019 const uint16_t uint14_max = 0x3FFF; 00020 void static inline normalize_14bits(int16_t &x) 00021 { 00022 x = ((x) > (uint14_max/2)) ? (x - uint14_max) : (x); 00023 } 00024 00025 static int16_t dummy_int16_t = 0; 00026 static float dummy_float = 0.0f; 00027 00028 FXOS8700Q::FXOS8700Q(I2C &i2c, uint8_t addr) 00029 { 00030 _i2c = &i2c; 00031 _addr = addr; 00032 // activate the peripheral 00033 uint8_t data[2] = {FXOS8700Q_CTRL_REG1, 0x00}; 00034 _i2c->frequency(400000); 00035 writeRegs(data, 2); 00036 data[0] = FXOS8700Q_M_CTRL_REG1; 00037 data[1] = 0x1F; 00038 writeRegs(data, 2); 00039 data[0] = FXOS8700Q_M_CTRL_REG2; 00040 data[1] = 0x20; 00041 writeRegs(data, 2); 00042 data[0] = FXOS8700Q_XYZ_DATA_CFG; 00043 data[1] = 0x00; 00044 writeRegs(data, 2); 00045 data[0] = FXOS8700Q_CTRL_REG1; 00046 data[1] = 0x1C; 00047 writeRegs(data, 2); 00048 } 00049 00050 FXOS8700Q::~FXOS8700Q() 00051 { 00052 _i2c = 0; 00053 _addr = 0; 00054 } 00055 00056 void FXOS8700Q::readRegs(uint8_t addr, uint8_t *data, uint32_t len) const 00057 { 00058 uint8_t t[1] = {addr}; 00059 _i2c->write(_addr, (char *)t, sizeof(t), true); 00060 _i2c->read(_addr, (char *)data, len); 00061 } 00062 00063 uint8_t FXOS8700Q::whoAmI() const 00064 { 00065 uint8_t who_am_i = 0; 00066 readRegs(FXOS8700Q_WHOAMI, &who_am_i, sizeof(who_am_i)); 00067 return who_am_i; 00068 } 00069 00070 void FXOS8700Q::writeRegs(uint8_t * data, uint32_t len) const 00071 { 00072 _i2c->write(_addr, (char *)data, len); 00073 } 00074 00075 00076 int16_t FXOS8700Q::getSensorAxis(uint8_t addr) const 00077 { 00078 uint8_t res[2]; 00079 readRegs(addr, res, sizeof(res)); 00080 return static_cast<int16_t>((res[0] << 8) | res[1]); 00081 } 00082 00083 void FXOS8700Q::enable(void) const 00084 { 00085 uint8_t data[2]; 00086 readRegs(FXOS8700Q_CTRL_REG1, &data[1], 1); 00087 data[1] |= 0x01; 00088 data[0] = FXOS8700Q_CTRL_REG1; 00089 writeRegs(data, sizeof(data)); 00090 } 00091 00092 void FXOS8700Q::disable(void) const 00093 { 00094 uint8_t data[2]; 00095 readRegs(FXOS8700Q_CTRL_REG1, &data[1], 1); 00096 data[1] &= 0xFE; 00097 data[0] = FXOS8700Q_CTRL_REG1; 00098 writeRegs(data, sizeof(data)); 00099 } 00100 00101 uint32_t FXOS8700Q::dataReady(void) const 00102 { 00103 uint8_t stat = 0; 00104 readRegs(FXOS8700Q_STATUS, &stat, 1); 00105 return (uint32_t)stat; 00106 } 00107 00108 uint32_t FXOS8700Q::sampleRate(uint32_t frequency) const 00109 { 00110 return(50); // for now sample rate is fixed at 50Hz 00111 } 00112 00113 int16_t FXOS8700QAccelerometer::getX(int16_t &x = dummy_int16_t) const 00114 { 00115 x = getSensorAxis(FXOS8700Q_OUT_X_MSB) >> 2; 00116 normalize_14bits(x); 00117 return x; 00118 } 00119 00120 int16_t FXOS8700QAccelerometer::getY(int16_t &y = dummy_int16_t) const 00121 { 00122 y = getSensorAxis(FXOS8700Q_OUT_Y_MSB) >> 2; 00123 normalize_14bits(y); 00124 return y; 00125 } 00126 00127 int16_t FXOS8700QAccelerometer::getZ(int16_t &z = dummy_int16_t) const 00128 { 00129 z = getSensorAxis(FXOS8700Q_OUT_Z_MSB) >> 2; 00130 normalize_14bits(z); 00131 return z; 00132 } 00133 00134 float FXOS8700QAccelerometer::getX(float &x = dummy_float) const 00135 { 00136 int16_t val = getSensorAxis(FXOS8700Q_OUT_X_MSB) >> 2; 00137 normalize_14bits(val); 00138 x = val / 4096.0f; 00139 return x; 00140 } 00141 00142 float FXOS8700QAccelerometer::getY(float &y = dummy_float) const 00143 { 00144 int16_t val = getSensorAxis(FXOS8700Q_OUT_Y_MSB) >> 2; 00145 normalize_14bits(val); 00146 y = val / 4096.0f; 00147 return y; 00148 } 00149 00150 float FXOS8700QAccelerometer::getZ(float &z = dummy_float) const 00151 { 00152 int16_t val = getSensorAxis(FXOS8700Q_OUT_Z_MSB) >> 2; 00153 normalize_14bits(val); 00154 z = val / 4096.0f; 00155 return z; 00156 } 00157 00158 void FXOS8700QAccelerometer::getAxis(motion_data_counts_t &xyz) const 00159 { 00160 uint8_t res[6]; 00161 readRegs(FXOS8700Q_OUT_X_MSB, res, sizeof(res)); 00162 xyz.x = static_cast<int16_t>((res[0] << 8) | res[1]) >> 2; 00163 xyz.y = static_cast<int16_t>((res[2] << 8) | res[3]) >> 2; 00164 xyz.z = static_cast<int16_t>((res[4] << 8) | res[5]) >> 2; 00165 normalize_14bits(xyz.x); 00166 normalize_14bits(xyz.y); 00167 normalize_14bits(xyz.z); 00168 } 00169 00170 void FXOS8700QAccelerometer::getAxis(motion_data_units_t &xyz) const 00171 { 00172 motion_data_counts_t _xyz; 00173 FXOS8700QAccelerometer::getAxis(_xyz); 00174 xyz.x = _xyz.x / 4096.0f; 00175 xyz.y = _xyz.y / 4096.0f; 00176 xyz.z = _xyz.z / 4096.0f; 00177 } 00178 00179 int16_t FXOS8700QMagnetometer::getX(int16_t &x = dummy_int16_t) const 00180 { 00181 x = getSensorAxis(FXOS8700Q_M_OUT_X_MSB); 00182 return x; 00183 } 00184 00185 int16_t FXOS8700QMagnetometer::getY(int16_t &y = dummy_int16_t) const 00186 { 00187 y = getSensorAxis(FXOS8700Q_M_OUT_Y_MSB); 00188 return y; 00189 } 00190 00191 int16_t FXOS8700QMagnetometer::getZ(int16_t &z = dummy_int16_t) const 00192 { 00193 z = getSensorAxis(FXOS8700Q_M_OUT_Z_MSB); 00194 return z; 00195 } 00196 00197 float FXOS8700QMagnetometer::getX(float &x = dummy_float) const 00198 { 00199 x = static_cast<float>(getSensorAxis(FXOS8700Q_M_OUT_X_MSB)) * 0.1f; 00200 return x; 00201 } 00202 00203 float FXOS8700QMagnetometer::getY(float &y = dummy_float) const 00204 { 00205 y = static_cast<float>(getSensorAxis(FXOS8700Q_M_OUT_Y_MSB)) * 0.1f; 00206 return y; 00207 } 00208 00209 float FXOS8700QMagnetometer::getZ(float &z = dummy_float) const 00210 { 00211 z = static_cast<float>(getSensorAxis(FXOS8700Q_M_OUT_Z_MSB)) * 0.1f; 00212 return z; 00213 } 00214 00215 void FXOS8700QMagnetometer::getAxis(motion_data_counts_t &xyz) const 00216 { 00217 uint8_t res[6]; 00218 readRegs(FXOS8700Q_M_OUT_X_MSB, res, sizeof(res)); 00219 xyz.x = (res[0] << 8) | res[1]; 00220 xyz.y = (res[2] << 8) | res[3]; 00221 xyz.z = (res[4] << 8) | res[5]; 00222 } 00223 00224 void FXOS8700QMagnetometer::getAxis(motion_data_units_t &xyz) const 00225 { 00226 motion_data_counts_t _xyz; 00227 FXOS8700QMagnetometer::getAxis(_xyz); 00228 xyz.x = static_cast<float>(_xyz.x * 0.1f); 00229 xyz.y = static_cast<float>(_xyz.y * 0.1f); 00230 xyz.z = static_cast<float>(_xyz.z * 0.1f); 00231 }
Generated on Tue Jul 12 2022 21:07:33 by
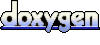