
most functionality to splashdwon, find neutral and start mission. short timeouts still in code for testing, will adjust to go directly to sit_idle after splashdown
Dependencies: mbed MODSERIAL FATFileSystem
StaticDefs.cpp
00001 #include "StaticDefs.hpp" 00002 00003 //Declare static global variables using 'construct on use' idiom to ensure they 00004 // are always constructed correctly and avoid "static initialization order fiasco". 00005 00006 Timer & systemTime() { 00007 static Timer s; 00008 return s; 00009 } 00010 00011 Ticker & pulse() { 00012 static Ticker pulse; 00013 return pulse; 00014 } 00015 00016 //MODSERIAL & pc() { 00017 // //static MODSERIAL pc(USBTX, USBRX); 00018 // return pc; 00019 //} 00020 00021 MODSERIAL & xbee() { 00022 static MODSERIAL xb(p9, p10); //XBee tx, rx pins 00023 //static MODSERIAL xb(USBTX, USBRX); //if you want to send all the xbee() messages throught the USB connection 00024 return xb; 00025 } 00026 00027 LocalFileSystem & local() { 00028 static LocalFileSystem local("local"); 00029 return local; 00030 } 00031 00032 //SDFileSystem & sd_card() { 00033 // static SDFileSystem sd_card(p11, p12, p13, p14, "sd"); //SDFileSystem sd_card(MOSI, MISO, SCK, CS, "sd"); 00034 // return sd_card; 00035 //} 00036 00037 SpiADC & adc() { 00038 static SpiADC adc(p5,p6,p7,p8,LED2); 00039 return adc; 00040 } 00041 00042 LinearActuator & bce() { // pwm,dir,res,swt 00043 static LinearActuator bce(0.01,p22,p15,p16,p17,0); //interval , pwm, dir, reset, limit switch, adc channel (confirmed) 00044 return bce; 00045 } 00046 00047 LinearActuator & batt() { // pwm,dir,res,swt 00048 static LinearActuator batt(0.01,p21,p20,p19,p18,1); //interval , pwm, dir, reset, limit switch, adc channel (confirmed) 00049 return batt; 00050 } 00051 00052 ServoDriver & rudder() { 00053 static ServoDriver rudder(p26); //current rudder pin on the latest drawing 06/11/2018 00054 return rudder; 00055 } 00056 00057 //*************Need to adjust class************** 00058 omegaPX209 & depth() { 00059 static omegaPX209 depth(p19); // pin 00060 return depth; 00061 } 00062 00063 IMU & imu() { 00064 static IMU imu(p28, p27); // tx, rx pin 00065 return imu; 00066 } 00067 Sensors & sensors() { 00068 static Sensors sensors; 00069 return sensors; 00070 } 00071 00072 OuterLoop & depthLoop() { 00073 static OuterLoop depthLoop(0.1, 0); // interval, sensor type 00074 return depthLoop; 00075 } 00076 00077 OuterLoop & pitchLoop() { 00078 static OuterLoop pitchLoop(0.1, 1); // interval, sensor type 00079 return pitchLoop; 00080 } 00081 00082 OuterLoop & headingLoop() { 00083 static OuterLoop headingLoop(0.1, 2); // interval, sensor type 00084 return headingLoop; 00085 } 00086 OuterLoop & altimLoop() { 00087 static OuterLoop altimLoop(0.1, 3); // interval, sensor type 00088 return altimLoop; 00089 } 00090 00091 00092 StateMachine & stateMachine() { 00093 static StateMachine stateMachine; 00094 return stateMachine; 00095 } 00096 00097 ConfigFileIO & configFileIO() { 00098 static ConfigFileIO configFileIO; 00099 return configFileIO; 00100 } 00101 00102 SequenceController & sequenceController() { 00103 static SequenceController sequenceController; 00104 return sequenceController; 00105 } 00106 LegController & legController() { 00107 static LegController legController; 00108 return legController; 00109 } 00110 00111 00112 MbedLogger & mbedLogger() { 00113 static MbedLogger mbedLogger("/local/"); //local file system 00114 return mbedLogger; 00115 } 00116 00117 //MbedLogger & sdLogger() { // leaving this in with no SD card might be a problem. 00118 // static MbedLogger sdLogger("/sd/"); 00119 // return sdLogger; 00120 //} 00121 00122 DigitalOut & led1() { 00123 static DigitalOut led1(LED1); 00124 return led1; 00125 } 00126 00127 DigitalOut & led2() { 00128 static DigitalOut led2(LED2); 00129 return led2; 00130 } 00131 00132 DigitalOut & led3() { 00133 static DigitalOut led3(LED3); 00134 return led3; 00135 } 00136 00137 DigitalOut & led4() { 00138 static DigitalOut led4(LED4); 00139 return led4; 00140 } 00141 DigitalIn & motorDisconnect() { 00142 static DigitalIn motorDisconnect(p24, PullDown); 00143 return motorDisconnect; 00144 } 00145 Gui & gui() { 00146 static Gui pythonGUI; 00147 return pythonGUI; 00148 }
Generated on Thu Jul 14 2022 10:54:35 by
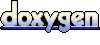