
most functionality to splashdwon, find neutral and start mission. short timeouts still in code for testing, will adjust to go directly to sit_idle after splashdown
Dependencies: mbed MODSERIAL FATFileSystem
ServoDriver.hpp
00001 #ifndef SERVODRIVER_HPP 00002 #define SERVODRIVER_HPP 00003 00004 #include "mbed.h" 00005 00006 class ServoDriver { 00007 public: 00008 ServoDriver(PinName pwm); 00009 00010 void endPoints(float lower_endpoint, float upper_endpoint); 00011 void neutralPosition(float input_neutral_position); 00012 void setPosition_deg(float input_position); 00013 00014 void setPWM(float input_pwm); // 8/2/2018 00015 00016 float slope(); 00017 00018 void setMinPWM(float pwm_input); 00019 void setMaxPWM(float pwm_input); 00020 void setCenterPWM(float pwm_input); 00021 void setMinDeg(float deg); 00022 void setMaxDeg(float deg); 00023 00024 float getSetPosition_deg(); 00025 float getSetPosition_pwm(); 00026 00027 void init(); 00028 00029 void pwm_pulse_off(); 00030 void pwm_pulse_on(); 00031 void pause(); 00032 void unpause(); 00033 00034 void runServo(); 00035 00036 float getMinPWM(); 00037 float getMaxPWM(); 00038 float getCenterPWM(); 00039 float getMinDeg(); 00040 float getMaxDeg(); 00041 00042 private: 00043 float _min_pwm; 00044 float _max_pwm; 00045 float _center_pwm; 00046 float _min_deg; 00047 float _max_deg; 00048 00049 volatile bool _paused; 00050 00051 float _degrees_set_position; 00052 00053 volatile unsigned int _valid_servo_position_pwm; //signal in microseconds, for example 1580 microseconds (close to center of servo) 00054 volatile unsigned int _period_cnt; 00055 00056 Ticker pwm_pulse_on_ticker; 00057 Ticker pwm_pulse_off_ticker; 00058 Timeout pwm_pulse_off_timeout; 00059 00060 DigitalOut _pwm_out; //cannot be volatile 00061 }; 00062 00063 template <typename T> 00064 T servoClamp(T value, T min, T max) 00065 { 00066 if(value < min) { 00067 return min; 00068 } else if(value > max) { 00069 return max; 00070 } else { 00071 return value; 00072 } 00073 }; 00074 00075 #endif
Generated on Thu Jul 14 2022 10:54:35 by
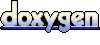