Stable version of the xDot library for mbed 5. This version of the library is suitable for deployment scenarios.
Dependents: Dot-Examples XDOT-Devicewise Dot-Examples-delujoc Dot-Examples_receive ... more
Fork of libxDot-dev-mbed5-deprecated by
SxRadio.h
00001 /* 00002 / _____) _ | | 00003 ( (____ _____ ____ _| |_ _____ ____| |__ 00004 \____ \| ___ | (_ _) ___ |/ ___) _ \ 00005 _____) ) ____| | | || |_| ____( (___| | | | 00006 (______/|_____)_|_|_| \__)_____)\____)_| |_| 00007 (C)2013 Semtech 00008 00009 Description: Generic radio driver definition 00010 00011 License: Revised BSD License, see LICENSE.TXT file include in the project 00012 00013 Maintainer: Miguel Luis and Gregory Cristian 00014 */ 00015 #ifndef __SXRADIO_H__ 00016 #define __SXRADIO_H__ 00017 00018 #include <stdint.h> 00019 #include "SxRadioEvents.h" 00020 00021 /*! 00022 * \brief Radio driver definition 00023 */ 00024 class SxRadio 00025 { 00026 public: 00027 /*! 00028 * Radio driver supported modems 00029 */ 00030 typedef enum 00031 { 00032 MODEM_FSK = 0, 00033 MODEM_LORA, 00034 }RadioModems_t ; 00035 00036 /*! 00037 * Radio driver internal state machine states definition 00038 */ 00039 typedef enum 00040 { 00041 RF_IDLE = 0, 00042 RF_RX_RUNNING, 00043 RF_TX_RUNNING, 00044 RF_CAD, 00045 RF_LBT 00046 }RadioState_t ; 00047 00048 SxRadio(uint32_t WakeupTime) : WakeupTime(WakeupTime), freq_offset(0), State(RF_IDLE), Modem(MODEM_LORA) { } 00049 virtual ~SxRadio() {}; 00050 00051 /*! 00052 * \brief Initializes the radio 00053 * 00054 * \param [IN] events Structure containing the driver callback functions 00055 */ 00056 virtual void Init( SxRadioEvents *events ) = 0; 00057 /*! 00058 * \brief Prepares the radio for destruction 00059 */ 00060 virtual void Terminate( void ) = 0; 00061 /*! 00062 * Return current radio status 00063 * 00064 * \param status Radio status.[RF_IDLE, RF_RX_RUNNING, RF_TX_RUNNING] 00065 */ 00066 virtual RadioState_t Status ( void ) { return State; } 00067 /*! 00068 * \brief Configures the radio with the given modem 00069 * 00070 * \param [IN] modem Modem to be used [0: FSK, 1: LoRa] 00071 */ 00072 virtual void SetModem( RadioModems_t modem ) = 0; 00073 virtual RadioModems_t GetModem( void ) { return Modem; } 00074 /*! 00075 * \brief Sets the channel frequency 00076 * 00077 * \param [IN] freq Channel RF frequency 00078 */ 00079 virtual void SetChannel( uint32_t freq ) = 0; 00080 /*! 00081 * \brief Sets the channels configuration 00082 * 00083 * \param [IN] modem Radio modem to be used [0: FSK, 1: LoRa] 00084 * \param [IN] freq Channel RF frequency 00085 * \param [IN] rssiThresh RSSI threshold 00086 * \param [IN] rssiVal pointer to variable to hold RSSI value if desired - ignored if NULL 00087 * 00088 * \retval isFree [true: Channel is free, false: Channel is not free] 00089 */ 00090 virtual bool IsChannelFree( RadioModems_t modem, uint32_t freq, int16_t rssiThresh, uint32_t timeout = 5000, int16_t *rssiVal = NULL ) = 0; 00091 /*! 00092 * \brief Generates a 32 bits random value based on the RSSI readings 00093 * 00094 * \remark This function sets the radio in LoRa modem mode and disables 00095 * all interrupts. 00096 * After calling this function either Radio.SetRxConfig or 00097 * Radio.SetTxConfig functions must be called. 00098 * 00099 * \retval randomValue 32 bits random value 00100 */ 00101 virtual uint32_t Random( void ) = 0; 00102 /*! 00103 * \brief Sets the reception parameters 00104 * 00105 * \param [IN] modem Radio modem to be used [0: FSK, 1: LoRa] 00106 * \param [IN] bandwidth Sets the bandwidth 00107 * FSK : >= 2600 and <= 250000 Hz 00108 * LoRa: [0: 125 kHz, 1: 250 kHz, 00109 * 2: 500 kHz, 3: Reserved] 00110 * \param [IN] datarate Sets the Datarate 00111 * FSK : 600..300000 bits/s 00112 * LoRa: [6: 64, 7: 128, 8: 256, 9: 512, 00113 * 10: 1024, 11: 2048, 12: 4096 chips] 00114 * \param [IN] coderate Sets the coding rate (LoRa only) 00115 * FSK : N/A ( set to 0 ) 00116 * LoRa: [1: 4/5, 2: 4/6, 3: 4/7, 4: 4/8] 00117 * \param [IN] bandwidthAfc Sets the AFC Bandwidth (FSK only) 00118 * FSK : >= 2600 and <= 250000 Hz 00119 * LoRa: N/A ( set to 0 ) 00120 * \param [IN] preambleLen Sets the Preamble length 00121 * FSK : Number of bytes 00122 * LoRa: Length in symbols (the hardware adds 4 more symbols) 00123 * \param [IN] symbTimeout Sets the RxSingle timeout value (LoRa only) 00124 * FSK : N/A ( set to 0 ) 00125 * LoRa: timeout in symbols 00126 * \param [IN] fixLen Fixed length packets [0: variable, 1: fixed] 00127 * \param [IN] payloadLen Sets payload length when fixed length is used 00128 * \param [IN] crcOn Enables/Disables the CRC [0: OFF, 1: ON] 00129 * \param [IN] FreqHopOn Enables disables the intra-packet frequency hopping 00130 * FSK : N/A ( set to 0 ) 00131 * LoRa: [0: OFF, 1: ON] 00132 * \param [IN] HopPeriod Number of symbols bewteen each hop 00133 * FSK : N/A ( set to 0 ) 00134 * LoRa: Number of symbols 00135 * \param [IN] iqInverted Inverts IQ signals (LoRa only) 00136 * FSK : N/A ( set to 0 ) 00137 * LoRa: [0: not inverted, 1: inverted] 00138 * \param [IN] rxContinuous Sets the reception in continuous mode 00139 * [false: single mode, true: continuous mode] 00140 * \param [IN] fskPad Duration in ms to increase FSK rx window 00141 * FSK: time in ms to increase FSK rx window duration 00142 * LoRa: N/A 00143 */ 00144 virtual void SetRxConfig( RadioModems_t modem, uint32_t bandwidth, 00145 uint32_t datarate, uint8_t coderate, 00146 uint32_t bandwidthAfc, uint16_t preambleLen, 00147 uint16_t symbTimeout, bool fixLen, 00148 uint8_t payloadLen, 00149 bool crcOn, bool FreqHopOn, uint8_t HopPeriod, 00150 bool iqInverted, bool rxContinuous , uint32_t fskPad = 0) = 0; 00151 /*! 00152 * \brief Sets the transmission parameters 00153 * 00154 * \param [IN] modem Radio modem to be used [0: FSK, 1: LoRa] 00155 * \param [IN] power Sets the output power [dBm] 00156 * \param [IN] fdev Sets the frequency deviation (FSK only) 00157 * FSK : [Hz] 00158 * LoRa: 0 00159 * \param [IN] bandwidth Sets the bandwidth (LoRa only) 00160 * FSK : 0 00161 * LoRa: [0: 125 kHz, 1: 250 kHz, 00162 * 2: 500 kHz, 3: Reserved] 00163 * \param [IN] datarate Sets the Datarate 00164 * FSK : 600..300000 bits/s 00165 * LoRa: [6: 64, 7: 128, 8: 256, 9: 512, 00166 * 10: 1024, 11: 2048, 12: 4096 chips] 00167 * \param [IN] coderate Sets the coding rate (LoRa only) 00168 * FSK : N/A ( set to 0 ) 00169 * LoRa: [1: 4/5, 2: 4/6, 3: 4/7, 4: 4/8] 00170 * \param [IN] preambleLen Sets the preamble length 00171 * FSK : Number of bytes 00172 * LoRa: Length in symbols (the hardware adds 4 more symbols) 00173 * \param [IN] fixLen Fixed length packets [0: variable, 1: fixed] 00174 * \param [IN] crcOn Enables disables the CRC [0: OFF, 1: ON] 00175 * \param [IN] FreqHopOn Enables disables the intra-packet frequency hopping 00176 * FSK : N/A ( set to 0 ) 00177 * LoRa: [0: OFF, 1: ON] 00178 * \param [IN] HopPeriod Number of symbols bewteen each hop 00179 * FSK : N/A ( set to 0 ) 00180 * LoRa: Number of symbols 00181 * \param [IN] iqInverted Inverts IQ signals (LoRa only) 00182 * FSK : N/A ( set to 0 ) 00183 * LoRa: [0: not inverted, 1: inverted] 00184 * \param [IN] timeout Transmission timeout [us] 00185 */ 00186 virtual void SetTxConfig( RadioModems_t modem, int8_t power, uint32_t fdev, 00187 uint32_t bandwidth, uint32_t datarate, 00188 uint8_t coderate, uint16_t preambleLen, 00189 bool fixLen, bool crcOn, bool FreqHopOn, 00190 uint8_t HopPeriod, bool iqInverted, uint32_t timeout ) = 0; 00191 00192 virtual void SetTxPower(int8_t power) = 0; 00193 00194 virtual void SetTxContinuousWave( uint32_t freq, int8_t power, uint16_t time ) = 0; 00195 00196 /*! 00197 * \brief Checks if the given RF frequency is supported by the hardware 00198 * 00199 * \param [IN] frequency RF frequency to be checked 00200 * \retval isSupported [true: supported, false: unsupported] 00201 */ 00202 virtual bool CheckRfFrequency( uint32_t frequency ) { return true; } 00203 /*! 00204 * \brief Computes the packet time on air for the given payload 00205 * 00206 * \Remark Can only be called once SetRxConfig or SetTxConfig have been called 00207 * 00208 * \param [IN] modem Radio modem to be used [0: FSK, 1: LoRa] 00209 * \param [IN] pktLen Packet payload length 00210 * 00211 * \retval airTime Computed airTime for the given packet payload length 00212 */ 00213 virtual double TimeOnAir( RadioModems_t modem, uint8_t pktLen ) = 0; 00214 /*! 00215 * \brief Sends the buffer of size. Prepares the packet to be sent and sets 00216 * the radio in transmission 00217 * 00218 * \param [IN]: buffer Buffer pointer 00219 * \param [IN]: size Buffer size 00220 */ 00221 virtual void Send( const uint8_t *buffer, uint8_t size ) = 0; 00222 /*! 00223 * \brief Sets the radio in sleep mode 00224 */ 00225 virtual void Sleep( void ) = 0; 00226 /*! 00227 * \brief Sets the radio in standby mode 00228 */ 00229 virtual void Standby( void ) = 0; 00230 /*! 00231 * \brief Sets the radio in reception mode for the given time 00232 * \param [IN] timeout Reception timeout [us] 00233 * [0: continuous, others timeout] 00234 */ 00235 virtual void Rx( uint32_t timeout ) = 0; 00236 /*! 00237 * \brief Start a Channel Activity Detection 00238 */ 00239 virtual void StartCad( void ) = 0; 00240 /*! 00241 * \brief Reads the current RSSI value 00242 * 00243 * \retval rssiValue Current RSSI value in [dBm] 00244 */ 00245 virtual int16_t Rssi( RadioModems_t modem ) = 0; 00246 /*! 00247 * \brief Writes the radio register at the specified address 00248 * 00249 * \param [IN]: addr Register address 00250 * \param [IN]: data New register value 00251 */ 00252 virtual void Write( uint8_t addr, uint8_t data ) = 0; 00253 /*! 00254 * \brief Reads the radio register at the specified address 00255 * 00256 * \param [IN]: addr Register address 00257 * \retval data Register value 00258 */ 00259 virtual uint8_t Read ( uint8_t addr ) = 0; 00260 /*! 00261 * \brief Writes multiple radio registers starting at address 00262 * 00263 * \param [IN] addr First Radio register address 00264 * \param [IN] buffer Buffer containing the new register's values 00265 * \param [IN] size Number of registers to be written 00266 */ 00267 virtual void WriteBuffer( uint8_t addr, const uint8_t *buffer, uint8_t size ) = 0; 00268 /*! 00269 * \brief Reads multiple radio registers starting at address 00270 * 00271 * \param [IN] addr First Radio register address 00272 * \param [OUT] buffer Buffer where to copy the registers data 00273 * \param [IN] size Number of registers to be read 00274 */ 00275 virtual void ReadBuffer( uint8_t addr, uint8_t *buffer, uint8_t size ) = 0; 00276 00277 virtual void SignalMacEvent(void) {}; 00278 virtual void SignalLinkEvent(void) {}; 00279 00280 virtual void ResetRadio(void) {}; 00281 00282 virtual uint32_t GetTimeOnAir(void) = 0; 00283 00284 void GrabMutex(void) { mutex .lock(); } 00285 void ReleaseMutex(void) { mutex .unlock(); } 00286 00287 int32_t GetFrequencyOffset() { return freq_offset; } 00288 void SetFrequencyOffset(int32_t offset) { freq_offset = offset; } 00289 00290 const uint32_t WakeupTime; 00291 00292 00293 00294 00295 protected: 00296 int32_t freq_offset; 00297 00298 RadioState_t State; 00299 00300 RadioModems_t Modem; 00301 00302 /*! 00303 * Access protection 00304 */ 00305 Mutex mutex ; 00306 00307 }; 00308 00309 #endif // __SXRADIO_H__ 00310
Generated on Wed Jul 13 2022 04:34:59 by
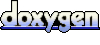