Stable version of the xDot library for mbed 5. This version of the library is suitable for deployment scenarios.
Dependents: Dot-Examples XDOT-Devicewise Dot-Examples-delujoc Dot-Examples_receive ... more
Fork of libxDot-dev-mbed5-deprecated by
Mote.h
00001 /** __ ___ ____ _ ______ __ ____ __ ____ 00002 * / |/ /_ __/ / /_(_)__/_ __/__ ____/ / / __/_ _____ / /____ __ _ ___ / _/__ ____ 00003 * / /|_/ / // / / __/ /___// / / -_) __/ _ \ _\ \/ // (_-</ __/ -_) ' \(_-< _/ // _ \/ __/ __ 00004 * /_/ /_/\_,_/_/\__/_/ /_/ \__/\__/_//_/ /___/\_, /___/\__/\__/_/_/_/___/ /___/_//_/\__/ /_/ 00005 * Copyright (C) 2015 by Multi-Tech Systems /___/ 00006 * 00007 * 00008 * @author Jason Reiss 00009 * @date 10-31-2015 00010 * @brief lora::Mote provides a user level class that abstracts the complexity of the Mac layer 00011 * 00012 * @details 00013 * 00014 */ 00015 00016 #ifndef __LORA_MOTE_H__ 00017 #define __LORA_MOTE_H__ 00018 00019 #include "mbed.h" 00020 #include "mbed_events.h" 00021 00022 #include "MacEvents.h" 00023 00024 #include <vector> 00025 00026 class SxRadio; 00027 class SxRadio1272; 00028 00029 namespace lora { 00030 00031 class Mac; 00032 class ChannelPlan; 00033 00034 class MoteEvents: public MacEvents { 00035 00036 /** 00037 * Fired at start of TX 00038 */ 00039 virtual void TxStart(void); 00040 00041 /** 00042 * Fired at end of TX 00043 * @param dr datarate used for TX 00044 */ 00045 virtual void TxDone(uint8_t dr); 00046 00047 /** 00048 * Fired if TX timed out 00049 */ 00050 virtual void TxTimeout(void); 00051 00052 /** 00053 * Fired when JoinAccept message is received and MIC is validated 00054 * @param payload received bytes 00055 * @param size number of received bytes 00056 * @param rssi of received packet 00057 * @param snr of received packet 00058 */ 00059 virtual void JoinAccept(uint8_t *payload, uint16_t size, int16_t rssi, int16_t snr); 00060 00061 /** 00062 * Fired when JoinAccept message is received and MIC is not valid 00063 * @param payload received bytes 00064 * @param size number of received bytes 00065 * @param rssi of received packet 00066 * @param snr of received packet 00067 */ 00068 virtual void JoinFailed(uint8_t *payload, uint16_t size, int16_t rssi, int16_t snr); 00069 00070 /** 00071 * Fired when packet is received and MIC is valid 00072 * @param port of packet 00073 * @param payload received bytes 00074 * @param size number of received bytes 00075 * @param rssi of received packet 00076 * @param snr of received packet 00077 * @param ctrl Downlink control field of packet 00078 * @param slot rx window packet was received 00079 * @param retries number of attempts before ack was received 00080 * @param address of the end device 00081 * @param dupRx set if this packet has already been received 00082 */ 00083 virtual void PacketRx(uint8_t port, uint8_t *payload, uint16_t size, int16_t rssi, int16_t snr, lora::DownlinkControl ctrl, uint8_t slot, uint8_t retries = 0, uint32_t address = 0, bool dupRx=false); 00084 00085 /** 00086 * Fired when radio has received a packet, packet is not validated 00087 * @param payload received bytes 00088 * @param size number of received bytes 00089 * @param rssi of received packet 00090 * @param snr of received packet 00091 * @param ctrl Downlink control field of packet 00092 * @param slot rx window packet was received 00093 */ 00094 virtual void RxDone(uint8_t *payload, uint16_t size, int16_t rssi, int16_t snr, lora::DownlinkControl ctrl, uint8_t slot); 00095 00096 /** 00097 * Fired when a beacon is received 00098 * @param beacon_data parsed from the beacon payload 00099 * @param rssi of received beacon 00100 * @param snr of received beacon 00101 */ 00102 virtual void BeaconRx(const BeaconData_t& beacon_data, int16_t rssi, int16_t snr); 00103 00104 /** 00105 * Fired upon losing beacon synchronization (120 minutes elapsed from last beacon reception) 00106 */ 00107 virtual void BeaconLost(); 00108 00109 /** 00110 * Fired if rx window times out 00111 * @param slot rx window that timed out 00112 */ 00113 virtual void RxTimeout(uint8_t slot); 00114 00115 /** 00116 * Fired if rx CRC error 00117 * @param slot rx window that errored 00118 */ 00119 virtual void RxError(uint8_t slot); 00120 00121 /** 00122 * Fired if pong packet is received 00123 * @param m_rssi of received packet at mote 00124 * @param m_snr of received packet at mote 00125 * @param s_rssi of received packet at server 00126 * @param s_snr of received packet at server 00127 */ 00128 virtual void Pong(int16_t m_rssi, int16_t m_snr, int16_t s_rssi, int16_t s_snr); 00129 00130 /** 00131 * Fired if network link check answer is received 00132 * @param m_rssi of received packet at mote 00133 * @param m_snr of received packet at mote 00134 * @param s_snr margin of received packet at server 00135 * @param s_gateways number of gateways reporting the packet 00136 */ 00137 virtual void NetworkLinkCheck(int16_t m_rssi, int16_t m_snr, int16_t s_snr, uint8_t s_gateways); 00138 00139 /** 00140 * Fired upon receiving a server time answer 00141 * @param seconds from the GPS epoch 00142 * @param sub_seconds from the GPS epoch 00143 */ 00144 virtual void ServerTime(uint32_t seconds, uint8_t sub_seconds); 00145 00146 /** 00147 * Callback to for device to measure the battery level and report to server 00148 * @return battery level 0-255, 0 - external power, 1-254 level min-max, 255 device unable to measure battery 00149 */ 00150 virtual uint8_t MeasureBattery(); 00151 00152 /** 00153 * Fired when ack attempts are exhausted and RxTimeout or RxError occur 00154 * @param retries number of attempts to resend the packet 00155 */ 00156 virtual void MissedAck(uint8_t retries); 00157 }; 00158 00159 class Mote { 00160 public: 00161 Mote(Settings* settings, ChannelPlan* plan); 00162 virtual ~Mote(); 00163 00164 /** 00165 * MTS LoRa version 00166 * @return string containing version information 00167 */ 00168 const char* getId(); 00169 00170 /** 00171 * MAC version 00172 * 00173 * @return string containing version information of supported LoRaWAN MAC Version 00174 */ 00175 const char* getMACVersion(); 00176 00177 /** 00178 * Indicator for network session join status 00179 * @return true if joined to network 00180 */ 00181 bool Joined(); 00182 00183 /** 00184 * Send join request 00185 * @return LORA_OK if request was sent 00186 */ 00187 uint8_t Join(); 00188 00189 /** 00190 * Send a packet 00191 * @param port to send packet 00192 * @param payload of packet 00193 * @param size in bytes 00194 * @return LORA_OK if successful 00195 * @return LORA_MAX_PAYLOAD_EXCEEDED if payload size exceeds datarate maximum 00196 * @return LORA_NO_CHANS_ENABLED if there is not an available channel that supports the current datarate 00197 * @return LORA_LINK_BUSY if link was busy 00198 * @return LORA_RADIO_BUSY if radio was busy 00199 * @return LORA_BUFFER_FULL if mac commands filled the packet, client should resend the packet 00200 */ 00201 uint8_t Send(uint8_t port, const uint8_t* payload, uint8_t size); 00202 00203 /** 00204 * Configure the channel plan 00205 * @param plan pointer to ChannelPlan object 00206 * @return LORA_OK 00207 */ 00208 uint8_t SetChannelPlan(ChannelPlan* plan); 00209 00210 00211 Settings* GetSettings(); 00212 00213 /** 00214 * Get the channel mask of currently enabled channels 00215 * @return vector containing channel bit masks 00216 */ 00217 std::vector<uint16_t> GetChannelMask(); 00218 00219 /** 00220 * Set a 16 bit channel mask with index 00221 * @param index of mask to set 0:0-15, 1:16-31 ... 00222 * @param mask 16 bit mask of enabled channels 00223 * @return true 00224 */ 00225 virtual uint8_t SetChannelMask(uint8_t index, uint16_t mask); 00226 00227 /** 00228 * Set the current frequency sub band for hybrid operation 1-8 else 0 for 64 channel operation 00229 * @param sub_band 0-8 00230 */ 00231 uint8_t SetFrequencySubBand(uint8_t sub_band); 00232 00233 /** 00234 * Get the current frequency sub band 00235 * @return sub band 0-8 00236 */ 00237 uint8_t GetFrequencySubBand(); 00238 00239 /** 00240 * Add a channel to the channel plan 00241 * EU868, AS923 and KR920 allows additional channels to be added 00242 * Channels 0-2 are fixed default channels 00243 * 00244 * @param index of the channel 00245 * @param frequency of the channel or 0 to remove channel 00246 * @param range of datarates allowed by the channel 00247 * @return LORA_OK if channel was added 00248 */ 00249 uint8_t AddChannel(uint8_t index, uint32_t frequency, lora::DatarateRange range); 00250 00251 /** 00252 * Add a downlink channel to the channel plan 00253 * EU868, AS923 and KR920 allows downlink channels to be added 00254 * 00255 * @param index of the channel 00256 * @param frequency of the channel or 0 to remove channel 00257 * @return LORA_OK if channel was added 00258 */ 00259 uint8_t AddDownlinkChannel(uint8_t index, uint32_t frequency); 00260 00261 /** 00262 * Set network mode 00263 * Choose Public LoRaWAN mode or Private Multitech mode 00264 * 00265 * Public mode uses 0x34 sync word with 5/6 second join windows 00266 * Private mode uses 0x12 sync word with 1/2 second join windows 00267 * US915/AU915 Rx1 and Rx2 are fixed per frequency sub band setting 00268 * 00269 * @param mode public or private 00270 * @return LORA_OK 00271 */ 00272 uint8_t SetNetworkMode(uint8_t mode); 00273 00274 /** 00275 * Get a pointer to the mac layer 00276 * @return Mac mac 00277 */ 00278 Mac* GetMac(); 00279 00280 /** 00281 * Get a pointer to the radio 00282 * Can be used to read radio registers or get a random value based on RSSI 00283 * 00284 * @return SxRadio pointer 00285 */ 00286 SxRadio* GetRadio(); 00287 00288 /** 00289 * Get the current statistics for the device 00290 * @return Statistics 00291 */ 00292 Statistics& GetStats(); 00293 00294 /** 00295 * Reset the current statistics for the device 00296 */ 00297 void ResetStats(); 00298 00299 /** 00300 * Get time on air with current settings for provided payload bytes 00301 * 13 overhead bytes will be added to payload 00302 * @param bytes of payload data 00303 * @return time-on-air in ms 00304 */ 00305 uint32_t GetTimeOnAir(uint8_t bytes); 00306 00307 /** 00308 * Get time off air required to adhere to duty-cycle limitations 00309 * @return time-off-air in ms 00310 */ 00311 uint32_t GetTimeOffAir(); 00312 00313 /** 00314 * Call before setting device in sleep mode to place radio in sleep 00315 */ 00316 void Sleep(); 00317 00318 protected: 00319 SxRadio1272* _radio; 00320 Settings* _settings; 00321 Mac* _mac; 00322 00323 private: 00324 ChannelPlan* _plan; 00325 MoteEvents _events; 00326 00327 // Event queue objects for timing events 00328 EventQueue _evtQueue; 00329 Thread _dispatch_thread; 00330 }; 00331 00332 } 00333 #endif
Generated on Wed Jul 13 2022 04:34:59 by
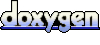