Stable version of the xDot library for mbed 5. This version of the library is suitable for deployment scenarios.
Dependents: Dot-Examples XDOT-Devicewise Dot-Examples-delujoc Dot-Examples_receive ... more
Fork of libxDot-dev-mbed5-deprecated by
MTSLog.h
00001 #ifndef MTSLOG_H 00002 #define MTSLOG_H 00003 00004 #include <string> 00005 00006 inline const char* className(const std::string& prettyFunction) 00007 { 00008 size_t colons = prettyFunction.find_last_of("::"); 00009 if (colons == std::string::npos) 00010 return ""; 00011 size_t begin = prettyFunction.substr(0,colons).rfind(" ") + 1; 00012 size_t end = colons - begin; 00013 00014 return prettyFunction.substr(begin,end).c_str(); 00015 } 00016 00017 #define __CLASSNAME__ className(__PRETTY_FUNCTION__) 00018 00019 #ifdef MTS_TIMESTAMP_LOG 00020 #define __LOG__(logLevel, format, ...) \ 00021 mts::MTSLog::printMessage(logLevel, "%s| [%s] " format "\r\n", \ 00022 mts::MTSLog::getTime().c_str(), mts::MTSLog::getLogLevelString(logLevel), ##__VA_ARGS__) 00023 #else 00024 #define __LOG__(logLevel, format, ...) \ 00025 mts::MTSLog::printMessage(logLevel, "[%s] " format "\r\n", mts::MTSLog::getLogLevelString(logLevel), ##__VA_ARGS__) 00026 #endif 00027 00028 #ifdef MTS_DEBUG 00029 #define logFatal(format, ...) \ 00030 mts::MTSLog::printMessage(mts::MTSLog::FATAL_LEVEL, "%s:%s:%d| [%s] " format "\r\n", __CLASSNAME__, __func__, __LINE__, mts::MTSLog::FATAL_LABEL, ##__VA_ARGS__) 00031 #define logError(format, ...) \ 00032 mts::MTSLog::printMessage(mts::MTSLog::ERROR_LEVEL, "%s:%s:%d| [%s] " format "\r\n", __CLASSNAME__, __func__, __LINE__, mts::MTSLog::ERROR_LABEL, ##__VA_ARGS__) 00033 #define logWarning(format, ...) \ 00034 mts::MTSLog::printMessage(mts::MTSLog::WARNING_LEVEL, "%s:%s:%d| [%s] " format "\r\n", __CLASSNAME__, __func__, __LINE__, mts::MTSLog::WARNING_LABEL, ##__VA_ARGS__) 00035 #define logInfo(format, ...) \ 00036 mts::MTSLog::printMessage(mts::MTSLog::INFO_LEVEL, "%s:%s:%d| [%s] " format "\r\n", __CLASSNAME__, __func__, __LINE__, mts::MTSLog::INFO_LABEL, ##__VA_ARGS__) 00037 #define logDebug(format, ...) \ 00038 mts::MTSLog::printMessage(mts::MTSLog::DEBUG_LEVEL, "%s:%s:%d| [%s] " format "\r\n", __CLASSNAME__, __func__, __LINE__, mts::MTSLog::DEBUG_LABEL, ##__VA_ARGS__) 00039 #define logTrace(format, ...) \ 00040 mts::MTSLog::printMessage(mts::MTSLog::TRACE_LEVEL, "%s:%s:%d| [%s] " format "\r\n", __CLASSNAME__, __func__, __LINE__, mts::MTSLog::TRACE_LABEL, ##__VA_ARGS__) 00041 #elif defined(MTS_DEBUG_OFF) 00042 #define logFatal(...) 00043 #define logError(...) 00044 #define logWarning(...) 00045 #define logInfo(...) 00046 #define logDebug(...) 00047 #define logTrace(...) 00048 #else 00049 #define logFatal(format, ...) \ 00050 __LOG__(mts::MTSLog::FATAL_LEVEL, format, ##__VA_ARGS__) 00051 #define logError(format, ...) \ 00052 __LOG__(mts::MTSLog::ERROR_LEVEL, format, ##__VA_ARGS__) 00053 #define logWarning(format, ...) \ 00054 __LOG__(mts::MTSLog::WARNING_LEVEL, format, ##__VA_ARGS__) 00055 #define logInfo(format, ...) \ 00056 __LOG__(mts::MTSLog::INFO_LEVEL, format, ##__VA_ARGS__) 00057 #define logDebug(format, ...) \ 00058 __LOG__(mts::MTSLog::DEBUG_LEVEL, format, ##__VA_ARGS__) 00059 #define logTrace(format, ...) \ 00060 __LOG__(mts::MTSLog::TRACE_LEVEL, format, ##__VA_ARGS__) 00061 #endif 00062 00063 namespace mts { 00064 00065 class MTSLog 00066 { 00067 public: 00068 00069 /** Enum of log levels. 00070 */ 00071 enum logLevel { 00072 NONE_LEVEL = 0, 00073 FATAL_LEVEL = 1, 00074 ERROR_LEVEL = 2, 00075 WARNING_LEVEL = 3, 00076 INFO_LEVEL = 4, 00077 DEBUG_LEVEL = 5, 00078 TRACE_LEVEL = 6 00079 }; 00080 00081 /** Print log message. 00082 */ 00083 static void printMessage(int level, const char* format, ...); 00084 00085 /** Determine if the given level is currently printable. 00086 */ 00087 static bool printable(int level); 00088 00089 /** Set log level 00090 * Messages with lower priority than the current level will not be printed. 00091 * If the level is set to NONE, no messages will print. 00092 */ 00093 static void setLogLevel(int level); 00094 00095 /** Get the current log level. 00096 */ 00097 static int getLogLevel(); 00098 00099 /** Get string representation of the current log level. 00100 */ 00101 static const char* getLogLevelString(); 00102 static const char* getLogLevelString(int level); 00103 00104 /** Get a formatted time string as HH:MM:SS 00105 */ 00106 static std::string getTime(); 00107 00108 static const char* NONE_LABEL; 00109 static const char* FATAL_LABEL; 00110 static const char* ERROR_LABEL; 00111 static const char* WARNING_LABEL; 00112 static const char* INFO_LABEL; 00113 static const char* DEBUG_LABEL; 00114 static const char* TRACE_LABEL; 00115 00116 private: 00117 00118 /** Constructor 00119 */ 00120 MTSLog(); 00121 00122 static int currentLevel; 00123 00124 }; 00125 00126 } 00127 00128 #endif
Generated on Wed Jul 13 2022 04:34:59 by
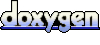