Stable version of the xDot library for mbed 5. This version of the library is suitable for deployment scenarios.
Dependents: Dot-Examples XDOT-Devicewise Dot-Examples-delujoc Dot-Examples_receive ... more
Fork of libxDot-dev-mbed5-deprecated by
Lora.h
00001 /** __ ___ ____ _ ______ __ ____ __ ____ 00002 * / |/ /_ __/ / /_(_)__/_ __/__ ____/ / / __/_ _____ / /____ __ _ ___ / _/__ ____ 00003 * / /|_/ / // / / __/ /___// / / -_) __/ _ \ _\ \/ // (_-</ __/ -_) ' \(_-< _/ // _ \/ __/ __ 00004 * /_/ /_/\_,_/_/\__/_/ /_/ \__/\__/_//_/ /___/\_, /___/\__/\__/_/_/_/___/ /___/_//_/\__/ /_/ 00005 * Copyright (C) 2015 by Multi-Tech Systems /___/ 00006 * 00007 * 00008 * @author Jason Reiss 00009 * @date 10-31-2015 00010 * @brief lora namespace defines global settings, structures and enums for the lora library 00011 * 00012 * @details 00013 * 00014 * 00015 * 00016 */ 00017 00018 #ifndef __LORA_H__ 00019 #define __LORA_H__ 00020 00021 #include "mbed.h" 00022 #include <assert.h> 00023 #include "MTSLog.h" 00024 //#include <cstring> 00025 #include <inttypes.h> 00026 00027 00028 namespace lora { 00029 00030 #ifndef MAC_VERSION 00031 const std::string MAC_VERSION = "1.0.4"; 00032 #endif 00033 00034 /** 00035 * Frequency bandwidth of a Datarate, higher bandwidth gives higher datarate 00036 */ 00037 enum Bandwidth { 00038 BW_125, 00039 BW_250, 00040 BW_500, 00041 BW_FSK = 50 00042 }; 00043 00044 /** 00045 * Spreading factor of a Datarate, lower spreading factor gives higher datarate 00046 */ 00047 enum SpreadingFactors { 00048 SF_6 = 6, 00049 SF_7, 00050 SF_8, 00051 SF_9, 00052 SF_10, 00053 SF_11, 00054 SF_12, 00055 SF_FSK, 00056 SF_INVALID 00057 }; 00058 00059 /** 00060 * Datarates for use with ChannelPlan 00061 */ 00062 enum Datarates { 00063 DR_0 = 0, 00064 DR_1, 00065 DR_2, 00066 DR_3, 00067 DR_4, 00068 DR_5, 00069 DR_6, 00070 DR_7, 00071 DR_8, 00072 DR_9, 00073 DR_10, 00074 DR_11, 00075 DR_12, 00076 DR_13, 00077 DR_14, 00078 DR_15 00079 }; 00080 00081 const uint8_t MIN_DATARATE = (uint8_t) DR_0; //!< Minimum datarate 00082 00083 00084 const uint8_t MAX_PHY_PACKET_SIZE = 255; //!< Maximum size for a packet 00085 const uint8_t MAX_APPS = 8; //!< Maximum number of apps sessions to save 00086 const uint8_t MAX_MULTICAST_SESSIONS = 8; //!< Maximum number of multicast sessions to save 00087 const uint8_t EUI_SIZE = 8; //!< Number of bytes in an EUI 00088 const uint8_t KEY_SIZE = 16; //!< Number of bytes in an AES key 00089 00090 const uint8_t DEFAULT_NUM_CHANNELS = 16; //!< Default number of channels in a plan 00091 const uint8_t DEFAULT_RX1_DR_OFFSET = 0; //!< Default datarate offset for first rx window 00092 const uint8_t DEFAULT_RX2_DATARATE = 0; //!< Default datarate for second rx window 00093 const uint8_t DEFAULT_TX_POWER = 14; //!< Default transmit power 00094 const uint8_t DEFAULT_CODE_RATE = 1; //!< Default coding rate 1:4/5, 2:4/6, 3:4/7, 4:4/8 00095 const uint8_t DEFAULT_PREAMBLE_LEN = 8; //!< Default preamble length 00096 00097 const int32_t MAX_FCNT_GAP = 16384; //!< Maximum allowed gap in sequence numbers before roll-over 00098 00099 const uint16_t PRIVATE_JOIN_DELAY = 1000; //!< Default join delay used for private network 00100 const uint16_t PUBLIC_JOIN_DELAY = 5000; //!< Default join delay used for public network 00101 const uint16_t DEFAULT_JOIN_DELAY = PRIVATE_JOIN_DELAY; //!< Default join delay1 00102 const uint16_t DEFAULT_RX_DELAY = 1000; //!< Default delay for first receive window 00103 const uint16_t DEFAULT_RX_TIMEOUT = 3000; //!< Default timeout for receive windows 00104 00105 const uint8_t HI_DR_SYMBOL_TIMEOUT = 12; //!< Symbol timeout for receive at datarate with SF < 11 00106 const uint8_t LO_DR_SYMBOL_TIMEOUT = 8; //!< Symbol timeout for receive at datarate with SF > 10 00107 00108 const uint16_t RX2_DELAY_OFFSET = 1000; //!< Delay between first and second window 00109 const uint16_t RXC_OFFSET = 50; //!< Time between end of RXC after TX and RX1 00110 00111 const uint16_t BEACON_PREAMBLE_LENGTH = 10U; //!< Beacon preamble length 00112 const uint16_t DEFAULT_BEACON_PERIOD = 128U; //!< Default period of the beacon (in seconds) 00113 const uint16_t PING_SLOT_LENGTH = 30U; //!< Duration of each class B ping slot (in milliseconds) 00114 const uint32_t BEACON_RESERVED_TIME = 2120U; //!< Time reserved for beacon broadcast (in milliseconds) 00115 const uint16_t BEACON_GUARD_TIME = 3000U; //!< Guard time before beacon transmission where no ping slots can be scheduled (in milliseconds) 00116 const uint32_t MAX_BEACONLESS_OP_TIME = 7200U; //!< Maximum time to operate in class B since last beacon received (in seconds) 00117 const uint16_t MAX_CLASS_B_WINDOW_GROWTH = 3U; //!< Maximum window growth factor for beacons and ping slots in beacon-less operation 00118 const uint16_t DEFAULT_PING_NB = 1U; //!< Default number of ping slots per beacon interval 00119 const uint16_t CLS_B_PAD = 15U; //!< Pad added to the beginning of ping slot rx windows (in milliseconds) 00120 const uint16_t BEACON_PAD = 100U; //!< Pad beacon window is expanded (in milliseconds) 00121 00122 const int16_t DEFAULT_FREE_CHAN_RSSI_THRESHOLD = -90; //!< Threshold for channel activity detection (CAD) dBm 00123 00124 const uint8_t CHAN_MASK_SIZE = 16; //!< Number of bits in a channel mask 00125 const uint8_t COMMANDS_BUFFER_SIZE = 15; //!< Size of Mac Command buffer 00126 00127 const uint8_t PKT_HEADER = 0; //!< Index to packet mHdr field 00128 const uint8_t PKT_ADDRESS = 1; //!< Index to first byte of packet address field 00129 const uint8_t PKT_FRAME_CONTROL = PKT_ADDRESS + 4; //!< Index to packet fCtrl field @see UplinkControl 00130 const uint8_t PKT_FRAME_COUNTER = PKT_FRAME_CONTROL + 1; //!< Index to packet frame counter field 00131 const uint8_t PKT_OPTIONS_START = PKT_FRAME_COUNTER + 2; //!< Index to start of optional mac commands 00132 00133 const uint8_t PKT_JOIN_APP_NONCE = 1; //!< Index to application nonce in Join Accept message 00134 const uint8_t PKT_JOIN_NETWORK_ID = 4; //!< Index to network id in Join Accept message 00135 const uint8_t PKT_JOIN_NETWORK_ADDRESS = 7; //!< Index to network address in Join Accept message 00136 const uint8_t PKT_JOIN_DL_SETTINGS = 11; //!< Index to downlink settings in Join Accept message 00137 const uint8_t PKT_JOIN_RX_DELAY = 12; //!< Index to rx delay in Join Accept message 00138 00139 const uint8_t DEFAULT_ADR_ACK_LIMIT = 64; //!< Number of packets without ADR ACK Request 00140 const uint8_t DEFAULT_ADR_ACK_DELAY = 32; //!< Number of packets to expect ADR ACK Response within 00141 00142 const uint16_t ACK_TIMEOUT = 2000; //!< Base millisecond timeout to resend after missed ACK 00143 const uint16_t ACK_TIMEOUT_RND = 1000; //!< Random millisecond adjustment to resend after missed ACK 00144 00145 const uint8_t FRAME_OVERHEAD = 13; //!< Bytes of network info overhead in a frame 00146 00147 const uint16_t MAX_OFF_AIR_WAIT = 5000U; //!< Max time in ms to block for a duty cycle restriction to expire before erroring out 00148 /** 00149 * Settings for type of network 00150 * 00151 * PRIVATE_MTS - Sync Word 0x12, US/AU Downlink frequencies per Frequency Sub Band 00152 * PUBLIC_LORAWAN - Sync Word 0x34 00153 * PRIVATE_LORAWAN - Sync Word 0x12 00154 * PEER_TO_PEER - Sync Word 0x56 used for Dot to Dot communication 00155 * 00156 * Join Delay window settings are independent of Network Type setting 00157 */ 00158 enum NetworkType { 00159 PRIVATE_MTS = 0, 00160 PUBLIC_LORAWAN = 1, 00161 PRIVATE_LORAWAN = 2, 00162 PEER_TO_PEER = 4 00163 }; 00164 00165 /** 00166 * Enum for on/off settings 00167 */ 00168 enum Enabled { 00169 OFF = 0, 00170 ON = 1 00171 }; 00172 00173 /** 00174 * Return status of mac functions 00175 */ 00176 enum MacStatus { 00177 LORA_OK = 0, 00178 LORA_ERROR = 1, 00179 LORA_JOIN_ERROR = 2, 00180 LORA_SEND_ERROR = 3, 00181 LORA_MIC_ERROR = 4, 00182 LORA_ADDRESS_ERROR = 5, 00183 LORA_NO_CHANS_ENABLED = 6, 00184 LORA_COMMAND_BUFFER_FULL = 7, 00185 LORA_UNKNOWN_MAC_COMMAND = 8, 00186 LORA_ADR_OFF = 9, 00187 LORA_BUSY = 10, 00188 LORA_LINK_BUSY = 11, 00189 LORA_RADIO_BUSY = 12, 00190 LORA_BUFFER_FULL = 13, 00191 LORA_JOIN_BACKOFF = 14, 00192 LORA_NO_FREE_CHAN = 15, 00193 LORA_AGGREGATED_DUTY_CYCLE = 16, 00194 LORA_MAC_COMMAND_ERROR = 17, 00195 LORA_MAX_PAYLOAD_EXCEEDED = 18, 00196 LORA_LBT_CHANNEL_BUSY = 19, 00197 LORA_BEACON_SIZE = 20, 00198 LORA_BEACON_CRC = 21 00199 }; 00200 00201 /** 00202 * State for Link 00203 */ 00204 enum LinkState { 00205 LINK_IDLE = 0, //!< Link ready to send or receive 00206 LINK_TX, //!< Link is busy sending 00207 LINK_ACK_TX, //!< Link is busy resending after missed ACK 00208 LINK_REP_TX, //!< Link is busy repeating 00209 LINK_RX, //!< Link has receive window open 00210 LINK_RX1, //!< Link has first received window open 00211 LINK_RX2, //!< Link has second received window open 00212 LINK_RXC, //!< Link has class C received window open 00213 LINK_RX_BEACON, //!< Link has a beacon receive window open 00214 LINK_RX_PING, //!< Link has a ping slot receive window open 00215 LINK_P2P //!< Link is busy sending 00216 }; 00217 00218 /** 00219 * State for MAC 00220 */ 00221 enum MacState { 00222 MAC_IDLE, 00223 MAC_RX1, 00224 MAC_RX2, 00225 MAC_RXC, 00226 MAC_TX, 00227 MAC_JOIN 00228 }; 00229 00230 /** 00231 * Operation class for device 00232 */ 00233 enum MoteClass { 00234 CLASS_A = 0x00, //!< Device can only receive in windows opened after a transmit 00235 CLASS_B = 0x01, //!< Device can receive in windows sychronized with gateway beacon 00236 CLASS_C = 0x02 //!< Device can receive any time when not transmitting 00237 }; 00238 00239 /** 00240 * Direction of a packet 00241 */ 00242 enum Direction { 00243 DIR_UP = 0, //!< Packet is sent from mote to gateway 00244 DIR_DOWN = 1, //!< Packet was received from gateway 00245 DIR_PEER = 2 //!< Packet was received from peer 00246 }; 00247 00248 00249 /** 00250 * Receive window used by Link 00251 */ 00252 enum ReceiveWindows { 00253 RX_1 = 1, //!< First receive window 00254 RX_2, //!< Second receive window 00255 RX_SLOT, //!< Ping slot receive window 00256 RX_BEACON, //!< Beacon receive window 00257 RXC, //!< Class C continuous window 00258 RX_TEST 00259 }; 00260 00261 /** 00262 * Beacon info descriptors for the GwSpecific Info field 00263 */ 00264 enum BeaconInfoDesc { 00265 GPS_FIRST_ANTENNA = 0, //!< GPS coordinates of the gateway's first antenna 00266 GPS_SECOND_ANTENNA, //!< GPS coordinates of the gateway's second antenna 00267 GPS_THIRD_ANTENNA, //!< GPS coordinates of the gateway's third antenna 00268 }; 00269 00270 /** 00271 * Datarate range for a Channel 00272 */ 00273 typedef union { 00274 int8_t Value; 00275 struct { 00276 int8_t Min :4; 00277 int8_t Max :4; 00278 } Fields; 00279 } DatarateRange; 00280 00281 /** 00282 * Datarate used for transmitting and receiving 00283 */ 00284 typedef struct Datarate { 00285 uint8_t Index; 00286 uint8_t Bandwidth; 00287 uint8_t Coderate; 00288 uint8_t PreambleLength; 00289 uint8_t SpreadingFactor; 00290 uint8_t Crc; 00291 uint8_t TxIQ; 00292 uint8_t RxIQ; 00293 uint16_t SymbolTimeout(uint16_t pad_ms = 0); 00294 float Timeout(); 00295 Datarate(); 00296 } Datarate; 00297 00298 /** 00299 * Channel used for transmitting 00300 */ 00301 typedef struct { 00302 uint8_t Index; 00303 uint32_t Frequency; 00304 DatarateRange DrRange; 00305 } Channel; 00306 00307 /** 00308 * Receive window 00309 */ 00310 typedef struct { 00311 uint8_t Index; 00312 uint32_t Frequency; 00313 uint8_t DatarateIndex; 00314 } RxWindow; 00315 00316 /** 00317 * Duty band for limiting time-on-air for regional regulations 00318 */ 00319 typedef struct { 00320 uint8_t Index; 00321 uint32_t FrequencyMin; 00322 uint32_t FrequencyMax; 00323 uint8_t PowerMax; 00324 uint16_t DutyCycle; //!< Multiplier of time on air, 0:100%, 1:50%, 2:33%, 10:10%, 100:1%, 1000,0.1% 00325 uint32_t TimeOffEnd; //!< Timestamp when this band will be available 00326 } DutyBand; 00327 00328 /** 00329 * Beacon data content (w/o CRCs and RFUs) 00330 */ 00331 typedef struct { 00332 uint32_t Time; 00333 uint8_t InfoDesc; 00334 uint32_t Latitude; 00335 uint32_t Longitude; 00336 } BeaconData_t; 00337 00338 /** 00339 * Device configuration 00340 */ 00341 typedef struct { 00342 uint8_t FrequencyBand; //!< Used to choose ChannelPlan 00343 uint8_t EUI[8]; //!< Unique identifier assigned to device 00344 } DeviceConfig; 00345 00346 /** 00347 * Network configuration 00348 */ 00349 typedef struct { 00350 uint8_t Mode; //!< PUBLIC, PRIVATE or PEER_TO_PEER network mode 00351 uint8_t Class; //!< Operating class of device 00352 uint8_t EUI[8]; //!< Network ID or AppEUI 00353 uint16_t DevNonce; //!< Incrementing DevNonce Counter 00354 uint32_t AppNonce; //!< Incrementing AppNonce Counter 00355 uint8_t Key[16]; //!< Network Key or AppKey 00356 uint8_t GenAppKey[16]; //!< Generic App Key, will be AppKey for LW 1.1.x 00357 uint8_t McKEKey[16]; //!< Multicast Key Encryption Key 00358 uint8_t JoinDelay; //!< Number of seconds to wait before 1st RX Window 00359 uint8_t RxDelay; //!< Number of seconds to wait before 1st RX Window 00360 uint8_t FrequencySubBand; //!< FrequencySubBand used for US915 hybrid operation 0:72 channels, 1:1-8 channels ... 00361 uint8_t AckEnabled; //!< Enable confirmed messages to be sent with Retries 00362 uint8_t Retries; //!< Number of times to resend a packet without receiving an ACK, redundancy 00363 uint8_t ADREnabled; //!< Enable adaptive datarate 00364 uint8_t AdrAckLimit; //!< Number of uplinks without a downlink to allow before setting ADRACKReq 00365 uint8_t AdrAckDelay; //!< Number of downlinks to expect ADR ACK Response within 00366 uint8_t CADEnabled; //!< Enable listen before talk/channel activity detection 00367 uint8_t RepeaterMode; //!< Limit payloads to repeater compatible sizes 00368 uint8_t TxPower; //!< Default radio output power in dBm 00369 uint8_t TxPowerMax; //!< Max transmit power 00370 uint8_t TxDatarate; //!< Datarate for P2P transmit 00371 uint32_t TxFrequency; //!< Frequency for P2P transmit 00372 int8_t AntennaGain; //!< Antenna Gain 00373 uint8_t DisableEncryption; //!< Disable Encryption 00374 uint8_t DisableCRC; //!< Disable CRC on uplink packets 00375 uint16_t P2PACKTimeout; 00376 uint16_t P2PACKBackoff; 00377 uint8_t JoinRx1DatarateOffset; //!< Offset for datarate for first window 00378 uint32_t JoinRx2Frequency; //!< Frequency used in second window 00379 uint8_t JoinRx2DatarateIndex; //!< Datarate for second window 00380 uint8_t PingPeriodicity; //!< Number of ping slots to open in a beacon interval (2^(7-PingPeriodicity)) 00381 } NetworkConfig; 00382 00383 /** 00384 * Network session info 00385 * Some settings are acquired in join message and others may be changed through Mac Commands from server 00386 */ 00387 typedef struct { 00388 uint8_t Joined; //!< State of session 00389 uint8_t Class; //!< Operating class of device 00390 uint8_t Rx1DatarateOffset; //!< Offset for datarate for first window 00391 uint32_t Rx2Frequency; //!< Frequency used in second window 00392 uint8_t Rx2DatarateIndex; //!< Datarate for second window 00393 uint32_t BeaconFrequency; //!< Frequency used for the beacon window 00394 bool BeaconFreqHop; //!< Beacon frequency hopping enable 00395 uint32_t PingSlotFrequency; //!< Frequency used for ping slot windows 00396 uint8_t PingSlotDatarateIndex; //!< Datarate for the ping slots 00397 bool PingSlotFreqHop; //!< Ping slot frequency hopping enable 00398 uint8_t TxPower; //!< Current total radiated output power in dBm 00399 uint8_t TxDatarate; //!< Current datarate can be changed when ADR is enabled 00400 uint32_t Address; //!< Network address 00401 uint32_t NetworkID; //!< Network ID 24-bits 00402 uint8_t NetworkSessionKey[16]; //!< Network session key 00403 uint8_t ApplicationSessionKey[16]; //!< Data session key 00404 uint16_t ChannelMask[4]; //!< Current channel mask 00405 uint16_t ChannelMask500k; //!< Current channel mask for 500k channels 00406 uint32_t DownlinkCounter; //!< Downlink counter of last packet received from server 00407 uint32_t UplinkCounter; //!< Uplink counter of last packet received from server 00408 uint8_t Redundancy; //!< Number of time to repeat an uplink 00409 uint8_t MaxDutyCycle; //!< Current Max Duty Cycle value 00410 uint32_t JoinTimeOnAir; //!< Balance of time on air used during join attempts 00411 uint32_t JoinTimeOffEnd; //!< RTC time of next join attempt 00412 uint32_t JoinFirstAttempt; //!< RTC time of first failed join attempt 00413 uint32_t AggregatedTimeOffEnd; //!< Time off air expiration for aggregate duty cycle 00414 uint16_t AggregateDutyCycle; //!< Used for enforcing time-on-air 00415 uint8_t AdrCounter; //!< Current number of packets received without downlink from server 00416 uint8_t RxDelay; //!< Number of seconds to wait before 1st RX Window 00417 uint8_t CommandBuffer[COMMANDS_BUFFER_SIZE]; //!< Buffer to hold Mac Commands and parameters to be sent in next packet 00418 uint8_t CommandBufferIndex; //!< Index to place next Mac Command, also current size of Command Buffer 00419 bool SrvRequestedAck; //!< Indicator of ACK requested by server in last packet received 00420 bool DataPending; //!< Indicator of data pending at server 00421 uint8_t RxTimingSetupReqReceived; //!< Indicator that RxTimingSetupAns should be included in uplink 00422 uint8_t RxParamSetupReqAnswer; //!< Indicator that RxParamSetupAns should be included in uplink 00423 uint8_t DlChannelReqAnswer; //!< Indicator that DlChannelAns should be included in uplink 00424 uint8_t DownlinkDwelltime; //!< On air dwell time for downlink packets 0:NONE,1:400ms 00425 uint8_t UplinkDwelltime; //!< On air dwell time for uplink packets 0:NONE,1:400ms 00426 uint8_t Max_EIRP; //!< Maximum allowed EIRP for uplink 00427 } NetworkSession; 00428 00429 /** 00430 * Multicast session info 00431 */ 00432 typedef struct MulticastSession { 00433 uint32_t Address; //!< Network address 00434 uint8_t NetworkSessionKey[16]; //!< Network session key 00435 uint8_t DataSessionKey[16]; //!< Data session key 00436 uint32_t DownlinkCounter; //!< Downlink counter of last packet received from server 00437 int8_t Periodicity; //!< Number of downlink windows to open per beacon period 00438 uint32_t Frequency; //!< Frequency used for downlink windows 00439 uint8_t DatarateIndex; //!< Datarate used for downlink windows 00440 bool DataPending; //!< Indicates network has data pending for this address 00441 uint16_t PingPeriod; 00442 int32_t NextPingSlot; 00443 MulticastSession() : 00444 Periodicity(-1) 00445 { 00446 00447 } 00448 } MulticastSession; 00449 00450 /** 00451 * Statistics of current network session 00452 */ 00453 typedef struct Statistics { 00454 uint32_t Up; //!< Number of uplink packets sent 00455 uint32_t Down; //!< Number of downlink packets received 00456 uint32_t Joins; //!< Number of join requests sent 00457 uint32_t JoinFails; //!< Number of join requests without response or invalid response 00458 uint32_t MissedAcks; //!< Number of missed acknowledgement attempts of confirmed packets 00459 uint32_t CRCErrors; //!< Number of CRC errors in received packets 00460 int32_t AvgCount; //!< Number of packets used to compute rolling average of RSSI and SNR 00461 int16_t Rssi; //!< RSSI of last packet received 00462 int16_t RssiMin; //!< Minimum RSSI of last AvgCount packets 00463 int16_t RssiMax; //!< Maximum RSSI of last AvgCount packets 00464 int16_t RssiAvg; //!< Rolling average RSSI of last AvgCount packets 00465 int16_t Snr; //!< SNR of last packet received 00466 int16_t SnrMin; //!< Minimum SNR of last AvgCount packets 00467 int16_t SnrMax; //!< Maximum SNR of last AvgCount packets 00468 int16_t SnrAvg; //!< Rolling average SNR of last AvgCount packets 00469 } Statistics; 00470 00471 /** 00472 * Testing settings 00473 */ 00474 typedef struct { 00475 uint8_t TestMode; 00476 uint8_t SkipMICCheck; 00477 uint8_t DisableDutyCycle; 00478 uint8_t DisableRx1; 00479 uint8_t DisableRx2; 00480 uint8_t FixedUplinkCounter; 00481 uint8_t DisableRandomJoinDatarate; 00482 uint8_t DisableAppNonceValidation; 00483 } Testing; 00484 00485 /** 00486 * Combination of device, network, testing settings and statistics 00487 */ 00488 typedef struct { 00489 DeviceConfig Device; 00490 NetworkConfig Network; 00491 NetworkSession Session; 00492 MulticastSession Multicast[MAX_MULTICAST_SESSIONS]; 00493 Statistics Stats; 00494 Testing Test; 00495 } Settings; 00496 00497 /** 00498 * Downlink settings sent in Join Accept message 00499 */ 00500 typedef union { 00501 uint8_t Value; 00502 struct { 00503 uint8_t Rx2Datarate :4; 00504 uint8_t Rx1Offset :3; 00505 uint8_t RFU :1; 00506 }; 00507 } DownlinkSettings; 00508 00509 /** 00510 * Frame structure for Join Request 00511 */ 00512 typedef struct { 00513 uint8_t Type; 00514 uint8_t AppEUI[8]; 00515 uint8_t DevEUI[8]; 00516 uint8_t Nonce[2]; 00517 uint8_t MIC[4]; 00518 } JoinRequestFrame; 00519 00520 /** 00521 * Mac header of uplink and downlink packets 00522 */ 00523 typedef union { 00524 uint8_t Value; 00525 struct { 00526 uint8_t Major :2; 00527 uint8_t RFU :3; 00528 uint8_t MType :3; 00529 } Bits; 00530 } MacHeader; 00531 00532 /** 00533 * Frame control field of uplink packets 00534 */ 00535 typedef union { 00536 uint8_t Value; 00537 struct { 00538 uint8_t OptionsLength :4; 00539 uint8_t ClassB :1; 00540 uint8_t Ack :1; 00541 uint8_t AdrAckReq :1; 00542 uint8_t Adr :1; 00543 } Bits; 00544 } UplinkControl; 00545 00546 /** 00547 * Frame control field of downlink packets 00548 */ 00549 typedef union { 00550 uint8_t Value; 00551 struct { 00552 uint8_t OptionsLength :4; 00553 uint8_t FPending :1; 00554 uint8_t Ack :1; 00555 uint8_t RFU :1; 00556 uint8_t Adr :1; 00557 } Bits; 00558 } DownlinkControl; 00559 00560 typedef struct PingSlot { 00561 uint32_t MSec; 00562 int8_t Id; 00563 PingSlot() : MSec(0), Id(-1) {} 00564 PingSlot(uint32_t msec, int8_t id) : MSec(msec), Id(id) {} 00565 } PingSlot; 00566 00567 /** 00568 * Frame type of packet 00569 */ 00570 typedef enum { 00571 FRAME_TYPE_JOIN_REQ = 0x00, 00572 FRAME_TYPE_JOIN_ACCEPT = 0x01, 00573 FRAME_TYPE_DATA_UNCONFIRMED_UP = 0x02, 00574 FRAME_TYPE_DATA_UNCONFIRMED_DOWN = 0x03, 00575 FRAME_TYPE_DATA_CONFIRMED_UP = 0x04, 00576 FRAME_TYPE_DATA_CONFIRMED_DOWN = 0x05, 00577 FRAME_TYPE_RFU = 0x06, 00578 FRAME_TYPE_PROPRIETARY = 0x07, 00579 } FrameType; 00580 00581 /** 00582 * LoRaWAN mote MAC commands 00583 */ 00584 typedef enum { 00585 /* Class A */ 00586 MOTE_MAC_LINK_CHECK_REQ = 0x02, 00587 MOTE_MAC_LINK_ADR_ANS = 0x03, 00588 MOTE_MAC_DUTY_CYCLE_ANS = 0x04, 00589 MOTE_MAC_RX_PARAM_SETUP_ANS = 0x05, 00590 MOTE_MAC_DEV_STATUS_ANS = 0x06, 00591 MOTE_MAC_NEW_CHANNEL_ANS = 0x07, 00592 MOTE_MAC_RX_TIMING_SETUP_ANS = 0x08, 00593 MOTE_MAC_TX_PARAM_SETUP_ANS = 0x09, 00594 MOTE_MAC_DL_CHANNEL_ANS = 0x0A, 00595 MOTE_MAC_REKEY_IND = 0x0B, 00596 MOTE_MAC_ADR_PARAM_SETUP_ANS = 0x0C, 00597 MOTE_MAC_DEVICE_TIME_REQ = 0x0D, 00598 MOTE_MAC_REJOIN_PARAM_SETUP_ANS = 0x0F, 00599 00600 /* Class B */ 00601 MOTE_MAC_PING_SLOT_INFO_REQ = 0x10, 00602 MOTE_MAC_PING_SLOT_CHANNEL_ANS = 0x11, 00603 MOTE_MAC_BEACON_TIMING_REQ = 0x12, 00604 MOTE_MAC_BEACON_FREQ_ANS = 0x13, 00605 00606 /* Multitech */ 00607 MOTE_MAC_PING_REQ = 0x80, 00608 MOTE_MAC_CHANGE_CLASS = 0x81, 00609 MOTE_MAC_MULTIPART_START_REQ = 0x82, 00610 MOTE_MAC_MULTIPART_START_ANS = 0x83, 00611 MOTE_MAC_MULTIPART_CHUNK = 0x84, 00612 MOTE_MAC_MULTIPART_END_REQ = 0x85, 00613 MOTE_MAC_MULTIPART_END_ANS = 0x86 00614 } MoteCommand; 00615 00616 /*! 00617 * LoRaWAN server MAC commands 00618 */ 00619 typedef enum { 00620 /* Class A */ 00621 SRV_MAC_LINK_CHECK_ANS = 0x02, 00622 SRV_MAC_LINK_ADR_REQ = 0x03, 00623 SRV_MAC_DUTY_CYCLE_REQ = 0x04, 00624 SRV_MAC_RX_PARAM_SETUP_REQ = 0x05, 00625 SRV_MAC_DEV_STATUS_REQ = 0x06, 00626 SRV_MAC_NEW_CHANNEL_REQ = 0x07, 00627 SRV_MAC_RX_TIMING_SETUP_REQ = 0x08, 00628 SRV_MAC_TX_PARAM_SETUP_REQ = 0x09, 00629 SRV_MAC_DL_CHANNEL_REQ = 0x0A, 00630 SRV_MAC_REKEY_CONF = 0x0B, 00631 SRV_MAC_ADR_PARAM_SETUP_REQ = 0x0C, 00632 SRV_MAC_DEVICE_TIME_ANS = 0x0D, 00633 SRV_MAC_FORCE_REJOIN_REQ = 0x0E, 00634 SRV_MAC_REJOIN_PARAM_SETUP_REQ = 0x0F, 00635 00636 /* Class B */ 00637 SRV_MAC_PING_SLOT_INFO_ANS = 0x10, 00638 SRV_MAC_PING_SLOT_CHANNEL_REQ = 0x11, 00639 SRV_MAC_BEACON_TIMING_ANS = 0x12, 00640 SRV_MAC_BEACON_FREQ_REQ = 0x13, 00641 00642 /* Multitech */ 00643 SRV_MAC_PING_ANS = 0x80, 00644 SRV_MAC_CHANGE_CLASS = 0x81, 00645 SRV_MAC_MULTIPART_START_REQ = 0x82, 00646 SRV_MAC_MULTIPART_START_ANS = 0x83, 00647 SRV_MAC_MULTIPART_CHUNK = 0x84, 00648 SRV_MAC_MULTIPART_END_REQ = 0x85, 00649 SRV_MAC_MULTIPART_END_ANS = 0x86 00650 } ServerCommand; 00651 00652 /** 00653 * Radio configuration options 00654 */ 00655 typedef enum RadioCfg { 00656 NO_RADIO_CFG, 00657 TX_RADIO_CFG, 00658 RX_RADIO_CFG 00659 } RadioCfg_t; 00660 00661 /** 00662 * Random seed for software RNG 00663 */ 00664 void srand(uint32_t seed); 00665 00666 /** 00667 * Software RNG for consistent results across differing hardware 00668 */ 00669 int rand(void); 00670 00671 /** 00672 * Generate random number bounded by min and max 00673 */ 00674 int32_t rand_r(int32_t min, int32_t max); 00675 00676 uint8_t CountBits(uint16_t mask); 00677 00678 /** 00679 * Copy 3-bytes network order from array into LSB of integer value 00680 */ 00681 void CopyNetIDtoInt(const uint8_t* arr, uint32_t& val); 00682 00683 /** 00684 * Copy LSB 3-bytes from integer value into array network order 00685 */ 00686 void CopyNetIDtoArray(uint32_t val, uint8_t* arr); 00687 00688 /** 00689 * Copy 4-bytes network order from array in to integer value 00690 */ 00691 void CopyAddrtoInt(const uint8_t* arr, uint32_t& val); 00692 00693 /** 00694 * Copy 4-bytes from integer in to array network order 00695 */ 00696 void CopyAddrtoArray(uint32_t val, uint8_t* arr); 00697 00698 /** 00699 * Copy 3-bytes network order from array into integer value and multiply by 100 00700 */ 00701 void CopyFreqtoInt(const uint8_t* arr, uint32_t& freq); 00702 00703 /** 00704 * Reverse memory copy 00705 */ 00706 void memcpy_r(uint8_t *dst, const uint8_t *src, size_t n); 00707 00708 } 00709 00710 #endif 00711
Generated on Wed Jul 13 2022 04:34:59 by
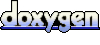