Stable version of the xDot library for mbed 5. This version of the library is suitable for deployment scenarios.
Dependents: Dot-Examples XDOT-Devicewise Dot-Examples-delujoc Dot-Examples_receive ... more
Fork of libxDot-dev-mbed5-deprecated by
ChannelPlan_RU864.h
00001 /** __ ___ ____ _ ______ __ ____ __ ____ 00002 * / |/ /_ __/ / /_(_)__/_ __/__ ____/ / / __/_ _____ / /____ __ _ ___ / _/__ ____ 00003 * / /|_/ / // / / __/ /___// / / -_) __/ _ \ _\ \/ // (_-</ __/ -_) ' \(_-< _/ // _ \/ __/ __ 00004 * /_/ /_/\_,_/_/\__/_/ /_/ \__/\__/_//_/ /___/\_, /___/\__/\__/_/_/_/___/ /___/_//_/\__/ /_/ 00005 * Copyright (C) 2015 by Multi-Tech Systems /___/ 00006 * 00007 * 00008 * @author Jason Reiss 00009 * @date 10-31-2015 00010 * @brief lora::ChannelPlan provides an interface for LoRaWAN channel schemes 00011 * 00012 * @details 00013 * 00014 */ 00015 00016 #ifndef __CHANNEL_PLAN_RU864_H__ 00017 #define __CHANNEL_PLAN_RU864_H__ 00018 00019 #include "Lora.h" 00020 #include "SxRadio.h" 00021 #include <vector> 00022 #include "ChannelPlan.h" 00023 00024 namespace lora { 00025 00026 const uint8_t RU864_125K_NUM_CHANS = 16; //!< Number of 125k channels in RU864 channel plan 00027 const uint8_t RU864_DEFAULT_NUM_CHANS = 2; //!< Number of defualt channels in RU864 channel plan 00028 const uint32_t RU864_125K_FREQ_BASE = 868900000; //!< Frequency base for 125k RU864 uplink channels 00029 const uint32_t RU864_125K_FREQ_STEP = 200000; //!< Frequency step for 125k RU864 uplink channels 00030 const uint32_t RU864_RX2_FREQ = 869100000; //!< Frequency default for second rx window in RU864 00031 00032 const uint8_t RU864_TX_POWER_MAX = 16; //!< Max power for RU864 channel plan 00033 00034 // 0.1% duty cycle 864-866 00035 const uint32_t RU864_MILLI_FREQ_MIN = 864000000; 00036 const uint32_t RU864_MILLI_FREQ_MAX = 865000000; 00037 00038 00039 const uint32_t RU864_FREQ_MIN = 864000000; 00040 const uint32_t RU864_FREQ_MAX = 870000000; 00041 00042 const uint8_t RU864_MIN_DATARATE = (uint8_t) DR_0; //!< Minimum transmit datarate for RU864 00043 const uint8_t RU864_MAX_DATARATE = (uint8_t) DR_7; //!< Maximum transmit datarate for RU864 00044 00045 const uint8_t RU864_MIN_DATARATE_OFFSET = (uint8_t) 0; //!< Minimum transmit datarate for US915 00046 const uint8_t RU864_MAX_DATARATE_OFFSET = (uint8_t) 5; //!< Maximum transmit datarate for US915 00047 00048 const uint8_t RU864_BEACON_DR = DR_3; //!< Default beacon datarate 00049 const uint32_t RU864_BEACON_FREQ = 869100000U; //!< Default beacon broadcast frequency 00050 const uint32_t RU864_PING_SLOT_FREQ = 868900000U; //!< Default ping slot frequency 00051 00052 class ChannelPlan_RU864 : public lora::ChannelPlan { 00053 public: 00054 /** 00055 * ChannelPlan constructor 00056 * @param radio SxRadio object used to set Tx/Rx config 00057 * @param settings Settings object 00058 */ 00059 ChannelPlan_RU864(); 00060 ChannelPlan_RU864(Settings* settings); 00061 ChannelPlan_RU864(SxRadio* radio, Settings* settings); 00062 00063 /** 00064 * ChannelPlan destructor 00065 */ 00066 virtual ~ChannelPlan_RU864(); 00067 00068 /** 00069 * Initialize channels, datarates and duty cycle bands according to current channel plan in settings 00070 */ 00071 virtual void Init(); 00072 00073 /** 00074 * Get the next channel to use to transmit 00075 * @return LORA_OK if channel was found 00076 * @return LORA_NO_CHANS_ENABLED 00077 */ 00078 virtual uint8_t GetNextChannel(); 00079 00080 /** 00081 * Add a channel to the ChannelPlan 00082 * @param index of channel, use -1 to add to end 00083 * @param channel settings to add 00084 */ 00085 virtual uint8_t AddChannel(int8_t index, Channel channel); 00086 00087 /** 00088 * Get channel at index 00089 * @return Channel 00090 */ 00091 virtual Channel GetChannel(int8_t index); 00092 00093 /** 00094 * Get rx window settings for requested window 00095 * RX_1, RX_2, RX_BEACON, RX_SLOT 00096 * @param window 00097 * @return RxWindow 00098 */ 00099 virtual RxWindow GetRxWindow(uint8_t window, int8_t id = 0); 00100 00101 /** 00102 * Get datarate to use on the join request 00103 * @return datarate index 00104 */ 00105 virtual uint8_t GetJoinDatarate(); 00106 00107 /** 00108 * Calculate the next time a join request is possible 00109 * @param size of join frame 00110 * @returns LORA_OK 00111 */ 00112 virtual uint8_t CalculateJoinBackoff(uint8_t size); 00113 00114 /** 00115 * Get next channel and set the SxRadio tx config with current settings 00116 * @return LORA_OK 00117 */ 00118 virtual uint8_t SetTxConfig(); 00119 00120 00121 /** 00122 * Set frequency sub band if supported by plan 00123 * @param sub_band 00124 * @return LORA_OK 00125 */ 00126 virtual uint8_t SetFrequencySubBand(uint8_t sub_band); 00127 00128 /** 00129 * Callback for Join Accept packet to load optional channels 00130 * @return LORA_OK 00131 */ 00132 virtual uint8_t HandleJoinAccept(const uint8_t* buffer, uint8_t size); 00133 00134 /** 00135 * Callback to for rx parameter setup ServerCommand 00136 * @param payload packet data 00137 * @param index of start of command buffer 00138 * @param size number of bytes in command buffer 00139 * @param[out] status to be returned in MoteCommand answer 00140 * @return LORA_OK 00141 */ 00142 virtual uint8_t HandleRxParamSetup(const uint8_t* payload, uint8_t index, uint8_t size, uint8_t& status); 00143 00144 /** 00145 * Callback to for new channel ServerCommand 00146 * @param payload packet data 00147 * @param index of start of command buffer 00148 * @param size number of bytes in command buffer 00149 * @param[out] status to be returned in MoteCommand answer 00150 * @return LORA_OK 00151 */ 00152 virtual uint8_t HandleNewChannel(const uint8_t* payload, uint8_t index, uint8_t size, uint8_t& status); 00153 00154 /** 00155 * Callback to for ping slot channel request ServerCommand 00156 * @param payload packet data 00157 * @param index of start of command buffer 00158 * @param size number of bytes in command buffer 00159 * @param[out] status to be returned in MoteCommand answer 00160 * @return LORA_OK 00161 */ 00162 virtual uint8_t HandlePingSlotChannelReq(const uint8_t* payload, uint8_t index, uint8_t size, uint8_t& status); 00163 00164 /** 00165 * Callback to for beacon frequency request ServerCommand 00166 * @param payload packet data 00167 * @param index of start of command buffer 00168 * @param size number of bytes in command buffer 00169 * @param[out] status to be returned in MoteCommand answer 00170 * @return LORA_OK 00171 */ 00172 virtual uint8_t HandleBeaconFrequencyReq(const uint8_t* payload, uint8_t index, uint8_t size, uint8_t& status); 00173 00174 /** 00175 * Callback to for adaptive datarate ServerCommand 00176 * @param payload packet data 00177 * @param index of start of command buffer 00178 * @param size number of bytes in command buffer 00179 * @param[out] status to be returned in MoteCommand answer 00180 * @return LORA_OK 00181 */ 00182 virtual uint8_t HandleAdrCommand(const uint8_t* payload, uint8_t index, uint8_t size, uint8_t& status); 00183 00184 /** 00185 * Validate the configuration after multiple ADR commands have been applied 00186 * @return status to be returned in MoteCommand answer 00187 */ 00188 virtual uint8_t ValidateAdrConfiguration(); 00189 00190 /** 00191 * Update duty cycle with at given frequency and time on air 00192 * @param freq frequency 00193 * @param time_on_air_ms tx time on air 00194 */ 00195 virtual void UpdateDutyCycle(uint32_t freq, uint32_t time_on_air_ms); 00196 00197 /** 00198 * Get the time the radio must be off air to comply with regulations 00199 * Time to wait may be dependent on duty-cycle restrictions per channel 00200 * Or duty-cycle of join requests if OTAA is being attempted 00201 * @return ms of time to wait for next tx opportunity 00202 */ 00203 virtual uint32_t GetTimeOffAir(); 00204 00205 /** 00206 * Get the channels in use by current channel plan 00207 * @return channel frequencies 00208 */ 00209 virtual std::vector<uint32_t> GetChannels(); 00210 00211 /** 00212 * Get the channel datarate ranges in use by current channel plan 00213 * @return channel datarate ranges 00214 */ 00215 virtual std::vector<uint8_t> GetChannelRanges(); 00216 00217 /** 00218 * Print log message for given rx window 00219 * @param wnd 1 or 2 00220 */ 00221 virtual void LogRxWindow(uint8_t wnd); 00222 00223 /** 00224 * Enable the default channels of the channel plan 00225 */ 00226 virtual void EnableDefaultChannels(); 00227 00228 /** 00229 * Check if this packet is a beacon and if so extract parameters needed 00230 * @param payload of potential beacon 00231 * @param size of the packet 00232 * @param [out] data extracted from the beacon if this packet was indeed a beacon 00233 * @return true if this packet is beacon, false if not 00234 */ 00235 virtual uint8_t DecodeBeacon(const uint8_t* payload, 00236 size_t size, 00237 BeaconData_t& data); 00238 00239 protected: 00240 00241 static const uint8_t RU864_TX_POWERS[8]; //!< List of available tx powers 00242 static const uint8_t RU864_RADIO_POWERS[21]; //!< List of calibrated tx powers 00243 static const uint8_t RU864_MAX_PAYLOAD_SIZE[]; //!< List of max payload sizes for each datarate 00244 static const uint8_t RU864_MAX_PAYLOAD_SIZE_REPEATER[]; //!< List of repeater compatible max payload sizes for each datarate 00245 00246 typedef struct __attribute__((packed)) { 00247 uint8_t RFU[2]; 00248 uint8_t Time[4]; 00249 uint8_t CRC1[2]; 00250 uint8_t GwSpecific[7]; 00251 uint8_t CRC2[2]; 00252 } BCNPayload; 00253 }; 00254 } 00255 00256 #endif //__CHANNEL_PLAN_RU864_H__
Generated on Wed Jul 13 2022 04:34:59 by
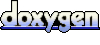