Stable version of the xDot library for mbed 5. This version of the library is suitable for deployment scenarios.
Dependents: Dot-Examples XDOT-Devicewise Dot-Examples-delujoc Dot-Examples_receive ... more
Fork of libxDot-dev-mbed5-deprecated by
ChannelPlan_KR920.h
00001 /** __ ___ ____ _ ______ __ ____ __ ____ 00002 * / |/ /_ __/ / /_(_)__/_ __/__ ____/ / / __/_ _____ / /____ __ _ ___ / _/__ ____ 00003 * / /|_/ / // / / __/ /___// / / -_) __/ _ \ _\ \/ // (_-</ __/ -_) ' \(_-< _/ // _ \/ __/ __ 00004 * /_/ /_/\_,_/_/\__/_/ /_/ \__/\__/_//_/ /___/\_, /___/\__/\__/_/_/_/___/ /___/_//_/\__/ /_/ 00005 * Copyright (C) 2015 by Multi-Tech Systems /___/ 00006 * 00007 * 00008 * @author Jason Reiss 00009 * @date 10-31-2015 00010 * @brief lora::ChannelPlan provides an interface for LoRaWAN channel schemes 00011 * 00012 * @details 00013 * 00014 */ 00015 00016 #ifndef __CHANNEL_PLAN_KR920_H__ 00017 #define __CHANNEL_PLAN_KR920_H__ 00018 00019 #include "Lora.h" 00020 #include "SxRadio.h" 00021 #include <vector> 00022 #include "ChannelPlan.h" 00023 00024 namespace lora { 00025 00026 const uint8_t KR920_125K_NUM_CHANS = 16; //!< Number of 125k channels in KR920 channel plan 00027 const uint8_t KR920_DEFAULT_NUM_CHANS = 3; //!< Number of default channels in KR920 channel plan 00028 const uint32_t KR920_125K_FREQ_BASE = 922100000; //!< Frequency base for 125k KR920 uplink channels 00029 const uint32_t KR920_125K_FREQ_STEP = 200000; //!< Frequency step for 125k KR920 uplink channels 00030 const uint32_t KR920_RX2_FREQ = 921900000; //!< Frequency default for second rx window in KR920 00031 const uint8_t KR920_TX_POWER_MAX = 14; //!< Max power for KR920 channel plan 00032 const uint8_t KR920_BEACON_DR = DR_3; //!< Default beacon datarate 00033 const uint32_t KR920_BEACON_FREQ = 923100000U; //!< Default beacon broadcast frequency 00034 00035 class ChannelPlan_KR920 : public lora::ChannelPlan { 00036 public: 00037 /** 00038 * ChannelPlan constructor 00039 * @param radio SxRadio object used to set Tx/Rx config 00040 * @param settings Settings object 00041 */ 00042 ChannelPlan_KR920(); 00043 ChannelPlan_KR920(Settings* settings); 00044 ChannelPlan_KR920(SxRadio* radio, Settings* settings); 00045 00046 /** 00047 * ChannelPlan destructor 00048 */ 00049 virtual ~ChannelPlan_KR920(); 00050 00051 /** 00052 * Initialize channels, datarates and duty cycle bands according to current channel plan in settings 00053 */ 00054 virtual void Init(); 00055 00056 /** 00057 * Get the next channel to use to transmit 00058 * @return LORA_OK if channel was found 00059 * @return LORA_NO_CHANS_ENABLED 00060 */ 00061 virtual uint8_t GetNextChannel(); 00062 00063 /** 00064 * Add a channel to the ChannelPlan 00065 * @param index of channel, use -1 to add to end 00066 * @param channel settings to add 00067 */ 00068 virtual uint8_t AddChannel(int8_t index, Channel channel); 00069 00070 /** 00071 * Get channel at index 00072 * @return Channel 00073 */ 00074 virtual Channel GetChannel(int8_t index); 00075 00076 /** 00077 * Get rx window settings for requested window 00078 * RX_1, RX_2, RX_BEACON, RX_SLOT 00079 * @param window 00080 * @return RxWindow 00081 */ 00082 virtual RxWindow GetRxWindow(uint8_t window, int8_t id = 0); 00083 00084 /** 00085 * Get datarate to use on the join request 00086 * @return datarate index 00087 */ 00088 virtual uint8_t GetJoinDatarate(); 00089 00090 /** 00091 * Calculate the next time a join request is possible 00092 * @param size of join frame 00093 * @returns LORA_OK 00094 */ 00095 virtual uint8_t CalculateJoinBackoff(uint8_t size); 00096 00097 /** 00098 * Get next channel and set the SxRadio tx config with current settings 00099 * @return LORA_OK 00100 */ 00101 virtual uint8_t SetTxConfig(); 00102 00103 /** 00104 * Set frequency sub band if supported by plan 00105 * @param sub_band 00106 * @return LORA_OK 00107 */ 00108 virtual uint8_t SetFrequencySubBand(uint8_t sub_band); 00109 00110 /** 00111 * Callback for Join Accept packet to load optional channels 00112 * @return LORA_OK 00113 */ 00114 virtual uint8_t HandleJoinAccept(const uint8_t* buffer, uint8_t size); 00115 00116 /** 00117 * Callback to for rx parameter setup ServerCommand 00118 * @param payload packet data 00119 * @param index of start of command buffer 00120 * @param size number of bytes in command buffer 00121 * @param[out] status to be returned in MoteCommand answer 00122 * @return LORA_OK 00123 */ 00124 virtual uint8_t HandleRxParamSetup(const uint8_t* payload, uint8_t index, uint8_t size, uint8_t& status); 00125 00126 /** 00127 * Callback to for new channel ServerCommand 00128 * @param payload packet data 00129 * @param index of start of command buffer 00130 * @param size number of bytes in command buffer 00131 * @param[out] status to be returned in MoteCommand answer 00132 * @return LORA_OK 00133 */ 00134 virtual uint8_t HandleNewChannel(const uint8_t* payload, uint8_t index, uint8_t size, uint8_t& status); 00135 00136 /** 00137 * Callback to for ping slot channel request ServerCommand 00138 * @param payload packet data 00139 * @param index of start of command buffer 00140 * @param size number of bytes in command buffer 00141 * @param[out] status to be returned in MoteCommand answer 00142 * @return LORA_OK 00143 */ 00144 virtual uint8_t HandlePingSlotChannelReq(const uint8_t* payload, uint8_t index, uint8_t size, uint8_t& status); 00145 00146 /** 00147 * Callback to for beacon frequency request ServerCommand 00148 * @param payload packet data 00149 * @param index of start of command buffer 00150 * @param size number of bytes in command buffer 00151 * @param[out] status to be returned in MoteCommand answer 00152 * @return LORA_OK 00153 */ 00154 virtual uint8_t HandleBeaconFrequencyReq(const uint8_t* payload, uint8_t index, uint8_t size, uint8_t& status); 00155 00156 /** 00157 * Callback to for adaptive datarate ServerCommand 00158 * @param payload packet data 00159 * @param index of start of command buffer 00160 * @param size number of bytes in command buffer 00161 * @param[out] status to be returned in MoteCommand answer 00162 * @return LORA_OK 00163 */ 00164 virtual uint8_t HandleAdrCommand(const uint8_t* payload, uint8_t index, uint8_t size, uint8_t& status); 00165 00166 /** 00167 * Validate the configuration after multiple ADR commands have been applied 00168 * @return status to be returned in MoteCommand answer 00169 */ 00170 virtual uint8_t ValidateAdrConfiguration(); 00171 00172 /** 00173 * Update duty cycle with at given frequency and time on air 00174 * @param freq frequency 00175 * @param time_on_air_ms tx time on air 00176 */ 00177 virtual void UpdateDutyCycle(uint32_t freq, uint32_t time_on_air_ms); 00178 00179 /** 00180 * Get the time the radio must be off air to comply with regulations 00181 * Time to wait may be dependent on duty-cycle restrictions per channel 00182 * Or duty-cycle of join requests if OTAA is being attempted 00183 * @return ms of time to wait for next tx opportunity 00184 */ 00185 virtual uint32_t GetTimeOffAir(); 00186 00187 /** 00188 * Get the channels in use by current channel plan 00189 * @return channel frequencies 00190 */ 00191 virtual std::vector<uint32_t> GetChannels(); 00192 00193 /** 00194 * Get the channel datarate ranges in use by current channel plan 00195 * @return channel datarate ranges 00196 */ 00197 virtual std::vector<uint8_t> GetChannelRanges(); 00198 00199 /** 00200 * Print log message for given rx window 00201 * @param wnd 1 or 2 00202 */ 00203 virtual void LogRxWindow(uint8_t wnd); 00204 00205 /** 00206 * Enable the default channels of the channel plan 00207 */ 00208 virtual void EnableDefaultChannels(); 00209 00210 /** 00211 * Called when MAC layer doesn't know about a command. 00212 * Use to add custom or new mac command handling 00213 * @return LORA_OK 00214 */ 00215 virtual uint8_t HandleMacCommand(uint8_t* payload, uint8_t& index); 00216 00217 /** 00218 * Set LBT time and threshold to defaults 00219 */ 00220 virtual void DefaultLBT(); 00221 00222 /** 00223 * Check if this packet is a beacon and if so extract parameters needed 00224 * @param payload of potential beacon 00225 * @param size of the packet 00226 * @param [out] data extracted from the beacon if this packet was indeed a beacon 00227 * @return true if this packet is beacon, false if not 00228 */ 00229 virtual uint8_t DecodeBeacon(const uint8_t* payload, 00230 size_t size, 00231 BeaconData_t& data); 00232 00233 protected: 00234 00235 static const uint8_t KR920_TX_POWERS[8]; //!< List of available tx powers 00236 static const uint8_t KR920_RADIO_POWERS[21]; //!< List of calibrated tx powers 00237 static const uint8_t KR920_MAX_PAYLOAD_SIZE[]; //!< List of max payload sizes for each datarate 00238 static const uint8_t KR920_MAX_PAYLOAD_SIZE_REPEATER[]; //!< List of repeater compatible max payload sizes for each datarate 00239 00240 typedef struct __attribute__((packed)) { 00241 uint8_t RFU[2]; 00242 uint8_t Time[4]; 00243 uint8_t CRC1[2]; 00244 uint8_t GwSpecific[7]; 00245 uint8_t CRC2[2]; 00246 } BCNPayload; 00247 }; 00248 } 00249 00250 #endif //__CHANNEL_PLAN_KR920_H__
Generated on Wed Jul 13 2022 04:34:59 by
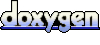