Stable version of the xDot library for mbed 5. This version of the library is suitable for deployment scenarios.
Dependents: Dot-Examples XDOT-Devicewise Dot-Examples-delujoc Dot-Examples_receive ... more
Fork of libxDot-dev-mbed5-deprecated by
ChannelPlan_IN865.h
00001 /** __ ___ ____ _ ______ __ ____ __ ____ 00002 * / |/ /_ __/ / /_(_)__/_ __/__ ____/ / / __/_ _____ / /____ __ _ ___ / _/__ ____ 00003 * / /|_/ / // / / __/ /___// / / -_) __/ _ \ _\ \/ // (_-</ __/ -_) ' \(_-< _/ // _ \/ __/ __ 00004 * /_/ /_/\_,_/_/\__/_/ /_/ \__/\__/_//_/ /___/\_, /___/\__/\__/_/_/_/___/ /___/_//_/\__/ /_/ 00005 * Copyright (C) 2015 by Multi-Tech Systems /___/ 00006 * 00007 * 00008 * @author Jason Reiss 00009 * @date 10-31-2015 00010 * @brief lora::ChannelPlan provides an interface for LoRaWAN channel schemes 00011 * 00012 * @details 00013 * 00014 */ 00015 00016 #ifndef __CHANNEL_PLAN_IN865_H__ 00017 #define __CHANNEL_PLAN_IN865_H__ 00018 00019 #include "Lora.h" 00020 #include "SxRadio.h" 00021 #include <vector> 00022 #include "ChannelPlan.h" 00023 00024 namespace lora { 00025 00026 const uint8_t IN865_125K_NUM_CHANS = 16; //!< Number of 125k channels in IN865 channel plan 00027 const uint8_t IN865_DEFAULT_NUM_CHANS = 3; //!< Number of default channels in IN865 channel plan 00028 const uint32_t IN865_125K_DEF_FREQ_1 = 865062500; 00029 const uint32_t IN865_125K_DEF_FREQ_2 = 865402500; 00030 const uint32_t IN865_125K_DEF_FREQ_3 = 865985000; 00031 const uint32_t IN865_RX2_FREQ = 866550000; //!< Frequency default for second rx window in IN865 00032 const uint8_t IN865_TX_POWER_MAX = 30; //!< Max power for IN865 channel plan 00033 const uint8_t IN865_BEACON_DR = DR_4; //!< Default beacon datarate 00034 const uint32_t IN865_BEACON_FREQ = 866550000U; //!< Default beacon broadcast frequency 00035 00036 class ChannelPlan_IN865 : public lora::ChannelPlan { 00037 public: 00038 /** 00039 * ChannelPlan constructor 00040 * @param radio SxRadio object used to set Tx/Rx config 00041 * @param settings Settings object 00042 */ 00043 ChannelPlan_IN865(); 00044 ChannelPlan_IN865(Settings* settings); 00045 ChannelPlan_IN865(SxRadio* radio, Settings* settings); 00046 00047 /** 00048 * ChannelPlan destructor 00049 */ 00050 virtual ~ChannelPlan_IN865(); 00051 00052 /** 00053 * Initialize channels, datarates and duty cycle bands according to current channel plan in settings 00054 */ 00055 virtual void Init(); 00056 00057 /** 00058 * Get the next channel to use to transmit 00059 * @return LORA_OK if channel was found 00060 * @return LORA_NO_CHANS_ENABLED 00061 */ 00062 virtual uint8_t GetNextChannel(); 00063 00064 /** 00065 * Add a channel to the ChannelPlan 00066 * @param index of channel, use -1 to add to end 00067 * @param channel settings to add 00068 */ 00069 virtual uint8_t AddChannel(int8_t index, Channel channel); 00070 00071 /** 00072 * Get channel at index 00073 * @return Channel 00074 */ 00075 virtual Channel GetChannel(int8_t index); 00076 00077 /** 00078 * Get rx window settings for requested window 00079 * RX_1, RX_2, RX_BEACON, RX_SLOT 00080 * @param window 00081 * @return RxWindow 00082 */ 00083 virtual RxWindow GetRxWindow(uint8_t window, int8_t id = 0); 00084 00085 /** 00086 * Get datarate to use on the join request 00087 * @return datarate index 00088 */ 00089 virtual uint8_t GetJoinDatarate(); 00090 00091 /** 00092 * Calculate the next time a join request is possible 00093 * @param size of join frame 00094 * @returns LORA_OK 00095 */ 00096 virtual uint8_t CalculateJoinBackoff(uint8_t size); 00097 00098 /** 00099 * Get next channel and set the SxRadio tx config with current settings 00100 * @return LORA_OK 00101 */ 00102 virtual uint8_t SetTxConfig(); 00103 00104 00105 /** 00106 * Set frequency sub band if supported by plan 00107 * @param sub_band 00108 * @return LORA_OK 00109 */ 00110 virtual uint8_t SetFrequencySubBand(uint8_t sub_band); 00111 00112 /** 00113 * Callback for Join Accept packet to load optional channels 00114 * @return LORA_OK 00115 */ 00116 virtual uint8_t HandleJoinAccept(const uint8_t* buffer, uint8_t size); 00117 00118 /** 00119 * Callback to for rx parameter setup ServerCommand 00120 * @param payload packet data 00121 * @param index of start of command buffer 00122 * @param size number of bytes in command buffer 00123 * @param[out] status to be returned in MoteCommand answer 00124 * @return LORA_OK 00125 */ 00126 virtual uint8_t HandleRxParamSetup(const uint8_t* payload, uint8_t index, uint8_t size, uint8_t& status); 00127 00128 /** 00129 * Callback to for new channel ServerCommand 00130 * @param payload packet data 00131 * @param index of start of command buffer 00132 * @param size number of bytes in command buffer 00133 * @param[out] status to be returned in MoteCommand answer 00134 * @return LORA_OK 00135 */ 00136 virtual uint8_t HandleNewChannel(const uint8_t* payload, uint8_t index, uint8_t size, uint8_t& status); 00137 00138 /** 00139 * Callback to for ping slot channel request ServerCommand 00140 * @param payload packet data 00141 * @param index of start of command buffer 00142 * @param size number of bytes in command buffer 00143 * @param[out] status to be returned in MoteCommand answer 00144 * @return LORA_OK 00145 */ 00146 virtual uint8_t HandlePingSlotChannelReq(const uint8_t* payload, uint8_t index, uint8_t size, uint8_t& status); 00147 00148 /** 00149 * Callback to for beacon frequency request ServerCommand 00150 * @param payload packet data 00151 * @param index of start of command buffer 00152 * @param size number of bytes in command buffer 00153 * @param[out] status to be returned in MoteCommand answer 00154 * @return LORA_OK 00155 */ 00156 virtual uint8_t HandleBeaconFrequencyReq(const uint8_t* payload, uint8_t index, uint8_t size, uint8_t& status); 00157 00158 /** 00159 * Callback to for adaptive datarate ServerCommand 00160 * @param payload packet data 00161 * @param index of start of command buffer 00162 * @param size number of bytes in command buffer 00163 * @param[out] status to be returned in MoteCommand answer 00164 * @return LORA_OK 00165 */ 00166 virtual uint8_t HandleAdrCommand(const uint8_t* payload, uint8_t index, uint8_t size, uint8_t& status); 00167 00168 /** 00169 * Validate the configuration after multiple ADR commands have been applied 00170 * @return status to be returned in MoteCommand answer 00171 */ 00172 virtual uint8_t ValidateAdrConfiguration(); 00173 00174 /** 00175 * Update duty cycle with at given frequency and time on air 00176 * @param freq frequency 00177 * @param time_on_air_ms tx time on air 00178 */ 00179 virtual void UpdateDutyCycle(uint32_t freq, uint32_t time_on_air_ms); 00180 00181 /** 00182 * Get the time the radio must be off air to comply with regulations 00183 * Time to wait may be dependent on duty-cycle restrictions per channel 00184 * Or duty-cycle of join requests if OTAA is being attempted 00185 * @return ms of time to wait for next tx opportunity 00186 */ 00187 virtual uint32_t GetTimeOffAir(); 00188 00189 /** 00190 * Get the channels in use by current channel plan 00191 * @return channel frequencies 00192 */ 00193 virtual std::vector<uint32_t> GetChannels(); 00194 00195 /** 00196 * Get the channel datarate ranges in use by current channel plan 00197 * @return channel datarate ranges 00198 */ 00199 virtual std::vector<uint8_t> GetChannelRanges(); 00200 00201 /** 00202 * Print log message for given rx window 00203 * @param wnd 1 or 2 00204 */ 00205 virtual void LogRxWindow(uint8_t wnd); 00206 00207 /** 00208 * Enable the default channels of the channel plan 00209 */ 00210 virtual void EnableDefaultChannels(); 00211 00212 /** 00213 * Called when MAC layer doesn't know about a command. 00214 * Use to add custom or new mac command handling 00215 * @return LORA_OK 00216 */ 00217 virtual uint8_t HandleMacCommand(uint8_t* payload, uint8_t& index); 00218 00219 /** 00220 *Decrements the datarate based on TxDwellTime 00221 */ 00222 virtual void DecrementDatarate(); 00223 00224 /** 00225 *Decrements the datarate based on TxDwellTime 00226 */ 00227 virtual void IncrementDatarate(); 00228 00229 /** 00230 * Check if this packet is a beacon and if so extract parameters needed 00231 * @param payload of potential beacon 00232 * @param size of the packet 00233 * @param [out] data extracted from the beacon if this packet was indeed a beacon 00234 * @return true if this packet is beacon, false if not 00235 */ 00236 virtual uint8_t DecodeBeacon(const uint8_t* payload, 00237 size_t size, 00238 BeaconData_t& data); 00239 00240 protected: 00241 00242 static const uint8_t IN865_TX_POWERS[11]; //!< List of available tx powers 00243 static const uint8_t IN865_RADIO_POWERS[21]; //!< List of calibrated tx powers 00244 static const uint8_t IN865_MAX_PAYLOAD_SIZE[]; //!< List of max payload sizes for each datarate 00245 static const uint8_t IN865_MAX_PAYLOAD_SIZE_REPEATER[]; //!< List of repeater compatible max payload sizes for each datarate 00246 00247 typedef struct __attribute__((packed)) { 00248 uint8_t RFU1[1]; 00249 uint8_t Time[4]; 00250 uint8_t CRC1[2]; 00251 uint8_t GwSpecific[7]; 00252 uint8_t RFU2[3]; 00253 uint8_t CRC2[2]; 00254 } BCNPayload; 00255 }; 00256 } 00257 00258 #endif //__CHANNEL_PLAN_IN865_H__
Generated on Wed Jul 13 2022 04:34:59 by
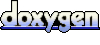