Stable version of the xDot library for mbed 5. This version of the library is suitable for deployment scenarios.
Dependents: Dot-Examples XDOT-Devicewise Dot-Examples-delujoc Dot-Examples_receive ... more
Fork of libxDot-dev-mbed5-deprecated by
ChannelPlan_AU915.h
00001 /** __ ___ ____ _ ______ __ ____ __ ____ 00002 * / |/ /_ __/ / /_(_)__/_ __/__ ____/ / / __/_ _____ / /____ __ _ ___ / _/__ ____ 00003 * / /|_/ / // / / __/ /___// / / -_) __/ _ \ _\ \/ // (_-</ __/ -_) ' \(_-< _/ // _ \/ __/ __ 00004 * /_/ /_/\_,_/_/\__/_/ /_/ \__/\__/_//_/ /___/\_, /___/\__/\__/_/_/_/___/ /___/_//_/\__/ /_/ 00005 * Copyright (C) 2015 by Multi-Tech Systems /___/ 00006 * 00007 * 00008 * @author Jason Reiss 00009 * @date 10-31-2015 00010 * @brief lora::ChannelPlan provides an interface for LoRaWAN channel schemes 00011 * 00012 * @details 00013 * 00014 */ 00015 00016 #ifndef __CHANNEL_PLAN_AU915_H__ 00017 #define __CHANNEL_PLAN_AU915_H__ 00018 00019 #include "Lora.h" 00020 #include "SxRadio.h" 00021 #include "ChannelPlan.h" 00022 #include <vector> 00023 00024 namespace lora { 00025 00026 const uint8_t AU915_125K_NUM_CHANS = 64; //!< Number of 125k channels in AU915 channel plan 00027 const uint8_t AU915_500K_NUM_CHANS = 8; //!< Number of 500k channels in AU915 channel plan 00028 00029 const uint32_t AU915_125K_FREQ_BASE = 915200000; //!< Frequency base for 125k AU915 uplink channels 00030 const uint32_t AU915_125K_FREQ_STEP = 200000; //!< Frequency step for 125k AU915 uplink channels 00031 00032 const uint32_t AU915_500K_FREQ_BASE = 915900000; //!< Frequency base for 500k AU915 uplink channels 00033 const uint32_t AU915_500K_FREQ_STEP = 1600000; //!< Frequency step for 500k AU915 uplink channels 00034 00035 const uint32_t AU915_500K_DBASE = 923300000; //!< Frequency base for 500k AU915 downlink channels 00036 const uint32_t AU915_500K_DSTEP = 600000; //!< Frequency step for 500k AU915 downlink channels 00037 00038 const uint32_t AU915_FREQ_MIN = 915000000; 00039 const uint32_t AU915_FREQ_MAX = 928000000; 00040 00041 const uint8_t AU915_MIN_DATARATE = (uint8_t) DR_0; //!< Minimum transmit datarate for AU915 00042 const uint8_t AU915_MAX_DATARATE = (uint8_t) DR_6; //!< Maximum transmit datarate for AU915 00043 00044 const uint8_t AU915_MIN_DATARATE_OFFSET = (uint8_t) 0; //!< Minimum transmit datarate for AU915 00045 const uint8_t AU915_MAX_DATARATE_OFFSET = (uint8_t) 5; //!< Maximum transmit datarate for AU915 00046 00047 const uint8_t AU915_BEACON_DR = DR_8; //!< Default beacon datarate 00048 const uint32_t AU915_BEACON_FREQ_BASE = 923300000U; //!< Base beacon broadcast frequency 00049 const uint32_t AU915_BEACON_FREQ_STEP = 600000U; //!< Step size for beacon frequencies 00050 const uint8_t AU915_BEACON_CHANNELS = 8U; //!< Number of beacon channels 00051 00052 class ChannelPlan_AU915 : public lora::ChannelPlan { 00053 public: 00054 00055 /** 00056 * ChannelPlan constructor 00057 * @param radio SxRadio object used to set Tx/Rx config 00058 * @param settings Settings object 00059 */ 00060 ChannelPlan_AU915(); 00061 ChannelPlan_AU915(Settings* settings); 00062 ChannelPlan_AU915(SxRadio* radio, Settings* settings); 00063 00064 /** 00065 * ChannelPlan destructor 00066 */ 00067 virtual ~ChannelPlan_AU915(); 00068 00069 /** 00070 * Initialize channels, datarates and duty cycle bands according to current channel plan in settings 00071 */ 00072 virtual void Init(); 00073 00074 /** 00075 * Get the next channel to use to transmit 00076 * @return LORA_OK if channel was found 00077 * @return LORA_NO_CHANS_ENABLED 00078 */ 00079 virtual uint8_t GetNextChannel(); 00080 00081 /** 00082 * Set the number of channels in the plan 00083 */ 00084 virtual void SetNumberOfChannels(uint8_t channels, bool resize = true); 00085 00086 /** 00087 * Check if channel is enabled 00088 * @return true if enabled 00089 */ 00090 virtual bool IsChannelEnabled(uint8_t channel); 00091 00092 00093 /** 00094 * Add a channel to the ChannelPlan 00095 * @param index of channel, use -1 to add to end 00096 * @param channel settings to add 00097 */ 00098 virtual uint8_t AddChannel(int8_t index, Channel channel); 00099 00100 /** 00101 * Get channel at index 00102 * @return Channel 00103 */ 00104 virtual Channel GetChannel(int8_t index); 00105 00106 /** 00107 * Get rx window settings for requested window 00108 * RX_1, RX_2, RX_BEACON, RX_SLOT 00109 * @param window 00110 * @return RxWindow 00111 */ 00112 virtual RxWindow GetRxWindow(uint8_t window, int8_t id = -1); 00113 00114 /** 00115 * Get datarate to use on the join request 00116 * @return datarate index 00117 */ 00118 virtual uint8_t GetJoinDatarate(); 00119 00120 /** 00121 * Calculate the next time a join request is possible 00122 * @param size of join frame 00123 * @returns LORA_OK 00124 */ 00125 virtual uint8_t CalculateJoinBackoff(uint8_t size); 00126 00127 /** 00128 * Set the datarate offset used for first receive window 00129 * @param offset 00130 * @return LORA_OK 00131 */ 00132 virtual uint8_t SetRx1Offset(uint8_t offset); 00133 00134 /** 00135 * Set the frequency for second receive window 00136 * @param freq 00137 * @return LORA_OK 00138 */ 00139 virtual uint8_t SetRx2Frequency(uint32_t freq); 00140 00141 /** 00142 * Set the datarate index used for second receive window 00143 * @param index 00144 * @return LORA_OK 00145 */ 00146 virtual uint8_t SetRx2DatarateIndex(uint8_t index); 00147 00148 /** 00149 * Get next channel and set the SxRadio tx config with current settings 00150 * @return LORA_OK 00151 */ 00152 virtual uint8_t SetTxConfig(); 00153 00154 /** 00155 * Set frequency sub band if supported by plan 00156 * @param sub_band 00157 * @return LORA_OK 00158 */ 00159 virtual uint8_t SetFrequencySubBand(uint8_t sub_band); 00160 00161 /** 00162 * Callback for Join Accept packet to load optional channels 00163 * @return LORA_OK 00164 */ 00165 virtual uint8_t HandleJoinAccept(const uint8_t* buffer, uint8_t size); 00166 00167 /** 00168 * Callback to for rx parameter setup ServerCommand 00169 * @param payload packet data 00170 * @param index of start of command buffer 00171 * @param size number of bytes in command buffer 00172 * @param[out] status to be returned in MoteCommand answer 00173 * @return LORA_OK 00174 */ 00175 virtual uint8_t HandleRxParamSetup(const uint8_t* payload, uint8_t index, uint8_t size, uint8_t& status); 00176 00177 /** 00178 * Callback to for new channel ServerCommand 00179 * @param payload packet data 00180 * @param index of start of command buffer 00181 * @param size number of bytes in command buffer 00182 * @param[out] status to be returned in MoteCommand answer 00183 * @return LORA_OK 00184 */ 00185 virtual uint8_t HandleNewChannel(const uint8_t* payload, uint8_t index, uint8_t size, uint8_t& status); 00186 00187 /** 00188 * Callback to for downlink channel request ServerCommand 00189 * @param payload packet data 00190 * @param index of start of command buffer 00191 * @param size number of bytes in command buffer 00192 * @param[out] status to be returned in MoteCommand answer 00193 * @return LORA_OK 00194 */ 00195 virtual uint8_t HandleDownlinkChannelReq(const uint8_t* payload, uint8_t index, uint8_t size, uint8_t& status); 00196 00197 /** 00198 * Callback to for ping slot channel request ServerCommand 00199 * @param payload packet data 00200 * @param index of start of command buffer 00201 * @param size number of bytes in command buffer 00202 * @param[out] status to be returned in MoteCommand answer 00203 * @return LORA_OK 00204 */ 00205 virtual uint8_t HandlePingSlotChannelReq(const uint8_t* payload, uint8_t index, uint8_t size, uint8_t& status); 00206 00207 /** 00208 * Callback to for beacon frequency request ServerCommand 00209 * @param payload packet data 00210 * @param index of start of command buffer 00211 * @param size number of bytes in command buffer 00212 * @param[out] status to be returned in MoteCommand answer 00213 * @return LORA_OK 00214 */ 00215 virtual uint8_t HandleBeaconFrequencyReq(const uint8_t* payload, uint8_t index, uint8_t size, uint8_t& status); 00216 00217 /** 00218 * Callback to for adaptive datarate ServerCommand 00219 * @param payload packet data 00220 * @param index of start of command buffer 00221 * @param size number of bytes in command buffer 00222 * @param[out] status to be returned in MoteCommand answer 00223 * @return LORA_OK 00224 */ 00225 virtual uint8_t HandleAdrCommand(const uint8_t* payload, uint8_t index, uint8_t size, uint8_t& status); 00226 00227 /** 00228 * Validate the configuration after multiple ADR commands have been applied 00229 * @return status to be returned in MoteCommand answer 00230 */ 00231 virtual uint8_t ValidateAdrConfiguration(); 00232 00233 /** 00234 * Get the time the radio must be off air to comply with regulations 00235 * Time to wait may be dependent on duty-cycle restrictions per channel 00236 * Or duty-cycle of join requests if OTAA is being attempted 00237 * @return ms of time to wait for next tx opportunity 00238 */ 00239 virtual uint32_t GetTimeOffAir(); 00240 00241 /** 00242 * Get the channels in use by current channel plan 00243 * @return channel frequencies 00244 */ 00245 virtual std::vector<uint32_t> GetChannels(); 00246 00247 /** 00248 * Get the channel datarate ranges in use by current channel plan 00249 * @return channel datarate ranges 00250 */ 00251 virtual std::vector<uint8_t> GetChannelRanges(); 00252 00253 00254 /** 00255 * Print log message for given rx window 00256 * @param wnd 1 or 2 00257 */ 00258 virtual void LogRxWindow(uint8_t wnd); 00259 00260 /** 00261 * Enable the default channels of the channel plan 00262 */ 00263 virtual void EnableDefaultChannels(); 00264 00265 /** 00266 * Called when MAC layer doesn't know about a command. 00267 * Use to add custom or new mac command handling 00268 * @return LORA_OK 00269 */ 00270 virtual uint8_t HandleMacCommand(uint8_t* payload, uint8_t& index); 00271 00272 /** 00273 * Get max payload size for current datarate 00274 * @return size in bytes 00275 */ 00276 virtual uint8_t GetMaxPayloadSize(); 00277 00278 /** 00279 * Get max payload size for given datarate 00280 * @return size in bytes 00281 */ 00282 virtual uint8_t GetMaxPayloadSize(uint8_t dr) { return ChannelPlan::GetMaxPayloadSize(dr); } 00283 00284 virtual uint8_t GetMinDatarate(); 00285 00286 virtual uint8_t GetMaxDatarate(); 00287 00288 /** 00289 * Check if this packet is a beacon and if so extract parameters needed 00290 * @param payload of potential beacon 00291 * @param size of the packet 00292 * @param [out] data extracted from the beacon if this packet was indeed a beacon 00293 * @return true if this packet is beacon, false if not 00294 */ 00295 virtual uint8_t DecodeBeacon(const uint8_t* payload, 00296 size_t size, 00297 BeaconData_t& data); 00298 00299 /** 00300 * Update class B beacon and ping slot settings if frequency hopping enabled 00301 * @param time received in the last beacon 00302 * @param period of the beacon 00303 * @param devAddr of this end device 00304 */ 00305 virtual void FrequencyHop(uint32_t time, uint32_t period, uint32_t devAddr); 00306 00307 protected: 00308 00309 static const uint8_t AU915_TX_POWERS[11]; //!< List of available tx powers 00310 static const uint8_t AU915_RADIO_POWERS[21]; //!< List of calibrated tx powers 00311 static const uint8_t AU915_MAX_PAYLOAD_SIZE[]; //!< List of max payload sizes for each datarate 00312 static const uint8_t AU915_MAX_PAYLOAD_SIZE_400[]; //!< List of max payload sizes for each datarate 00313 static const uint8_t AU915_MAX_PAYLOAD_SIZE_REPEATER[]; //!< List of repeater compatible max payload sizes for each datarate 00314 static const uint8_t AU915_MAX_PAYLOAD_SIZE_REPEATER_400[]; //!< List of repeater compatible max payload sizes for each datarate 00315 static const uint8_t MAX_ERP_VALUES[]; //!< Lookup table for Max EIRP (dBm) codes 00316 00317 typedef struct __attribute__((packed)) { 00318 uint8_t RFU1[3]; 00319 uint8_t Time[4]; 00320 uint8_t CRC1[2]; 00321 uint8_t GwSpecific[7]; 00322 uint8_t RFU2[1]; 00323 uint8_t CRC2[2]; 00324 } BCNPayload; 00325 }; 00326 } 00327 00328 #endif // __CHANNEL_PLAN_AU915_H__
Generated on Wed Jul 13 2022 04:34:59 by
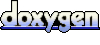