Stable version of the xDot library for mbed 5. This version of the library is suitable for deployment scenarios.
Dependents: Dot-Examples XDOT-Devicewise Dot-Examples-delujoc Dot-Examples_receive ... more
Fork of libxDot-dev-mbed5-deprecated by
ChannelPlan_AS923.h
00001 /********************************************************************** 00002 * COPYRIGHT 2015 MULTI-TECH SYSTEMS, INC. 00003 * 00004 * Redistribution and use in source and binary forms, with or without modification, 00005 * are permitted provided that the following conditions are met: 00006 * 1. Redistributions of source code must retain the above copyright notice, 00007 * this list of conditions and the following disclaimer. 00008 * 2. Redistributions in binary form must reproduce the above copyright notice, 00009 * this list of conditions and the following disclaimer in the documentation 00010 * and/or other materials provided with the distribution. 00011 * 3. Neither the name of MULTI-TECH SYSTEMS, INC. nor the names of its contributors 00012 * may be used to endorse or promote products derived from this software 00013 * without specific prior written permission. 00014 * 00015 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00016 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00017 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00018 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00019 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00020 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00021 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00022 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00023 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00024 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00025 * 00026 ****************************************************************************** 00027 * 00028 * @author Jason Reiss 00029 * @date 10-31-2015 00030 * @brief lora::ChannelPlan provides an interface for LoRaWAN channel schemes 00031 * 00032 * @details 00033 * 00034 */ 00035 00036 #ifndef __CHANNEL_PLAN_AS923_H__ 00037 #define __CHANNEL_PLAN_AS923_H__ 00038 00039 #include "Lora.h" 00040 #include "SxRadio.h" 00041 #include <vector> 00042 #include "ChannelPlan.h" 00043 00044 namespace lora { 00045 00046 const uint8_t AS923_125K_NUM_CHANS = 16; //!< Number of 125k channels in AS923 channel plan 00047 const uint8_t AS923_DEFAULT_NUM_CHANS = 2; //!< Number of default channels in AS923 channel plan 00048 const uint32_t AS923_125K_FREQ_BASE = 923200000; //!< Frequency base for 125k AS923 uplink channels 00049 const uint32_t AS923_125K_FREQ_STEP = 200000; //!< Frequency step for 125k AS923 uplink channels 00050 const uint32_t AS923_RX2_FREQ = 923200000; //!< Frequency default for second rx window in AS923 00051 const uint8_t AS923_BEACON_DR = DR_3; //!< Default beacon datarate 00052 const uint32_t AS923_BEACON_FREQ = 923400000U; //!< Default beacon broadcast frequency 00053 00054 class ChannelPlan_AS923: public lora::ChannelPlan { 00055 public: 00056 /** 00057 * ChannelPlan constructor 00058 * @param radio SxRadio object used to set Tx/Rx config 00059 * @param settings Settings object 00060 */ 00061 ChannelPlan_AS923(); 00062 ChannelPlan_AS923(Settings* settings); 00063 ChannelPlan_AS923(SxRadio* radio, Settings* settings); 00064 00065 /** 00066 * ChannelPlan destructor 00067 */ 00068 virtual ~ChannelPlan_AS923(); 00069 00070 /** 00071 * Initialize channels, datarates and duty cycle bands according to current channel plan in settings 00072 */ 00073 virtual void Init(); 00074 00075 /** 00076 * Get the next channel to use to transmit 00077 * @return LORA_OK if channel was found 00078 * @return LORA_NO_CHANS_ENABLED 00079 */ 00080 virtual uint8_t GetNextChannel(); 00081 00082 /** 00083 * Add a channel to the ChannelPlan 00084 * @param index of channel, use -1 to add to end 00085 * @param channel settings to add 00086 */ 00087 virtual uint8_t AddChannel(int8_t index, Channel channel); 00088 00089 /** 00090 * Get channel at index 00091 * @return Channel 00092 */ 00093 virtual Channel GetChannel(int8_t index); 00094 00095 /** 00096 * Get rx window settings for requested window 00097 * RX_1, RX_2, RX_BEACON, RX_SLOT 00098 * @param window 00099 * @return RxWindow 00100 */ 00101 virtual RxWindow GetRxWindow(uint8_t window, int8_t id = -1); 00102 00103 /** 00104 * Get datarate to use on the join request 00105 * @return datarate index 00106 */ 00107 virtual uint8_t GetJoinDatarate(); 00108 00109 /** 00110 * Calculate the next time a join request is possible 00111 * @param size of join frame 00112 * @returns LORA_OK 00113 */ 00114 virtual uint8_t CalculateJoinBackoff(uint8_t size); 00115 00116 /** 00117 * Get next channel and set the SxRadio tx config with current settings 00118 * @return LORA_OK 00119 */ 00120 virtual uint8_t SetTxConfig(); 00121 00122 00123 /** 00124 * Set frequency sub band if supported by plan 00125 * @param sub_band 00126 * @return LORA_OK 00127 */ 00128 virtual uint8_t SetFrequencySubBand(uint8_t sub_band); 00129 00130 /** 00131 * Callback for Join Accept packet to load optional channels 00132 * @return LORA_OK 00133 */ 00134 virtual uint8_t HandleJoinAccept(const uint8_t* buffer, uint8_t size); 00135 00136 /** 00137 * Callback to for rx parameter setup ServerCommand 00138 * @param payload packet data 00139 * @param index of start of command buffer 00140 * @param size number of bytes in command buffer 00141 * @param[out] status to be returned in MoteCommand answer 00142 * @return LORA_OK 00143 */ 00144 virtual uint8_t HandleRxParamSetup(const uint8_t* payload, uint8_t index, uint8_t size, uint8_t& status); 00145 00146 /** 00147 * Callback to for new channel ServerCommand 00148 * @param payload packet data 00149 * @param index of start of command buffer 00150 * @param size number of bytes in command buffer 00151 * @param[out] status to be returned in MoteCommand answer 00152 * @return LORA_OK 00153 */ 00154 virtual uint8_t HandleNewChannel(const uint8_t* payload, uint8_t index, uint8_t size, uint8_t& status); 00155 00156 /** 00157 * Callback to for ping slot channel request ServerCommand 00158 * @param payload packet data 00159 * @param index of start of command buffer 00160 * @param size number of bytes in command buffer 00161 * @param[out] status to be returned in MoteCommand answer 00162 * @return LORA_OK 00163 */ 00164 virtual uint8_t HandlePingSlotChannelReq(const uint8_t* payload, uint8_t index, uint8_t size, uint8_t& status); 00165 00166 /** 00167 * Callback to for beacon frequency request ServerCommand 00168 * @param payload packet data 00169 * @param index of start of command buffer 00170 * @param size number of bytes in command buffer 00171 * @param[out] status to be returned in MoteCommand answer 00172 * @return LORA_OK 00173 */ 00174 virtual uint8_t HandleBeaconFrequencyReq(const uint8_t* payload, uint8_t index, uint8_t size, uint8_t& status); 00175 00176 /** 00177 * Callback to for adaptive datarate ServerCommand 00178 * @param payload packet data 00179 * @param index of start of command buffer 00180 * @param size number of bytes in command buffer 00181 * @param[out] status to be returned in MoteCommand answer 00182 * @return LORA_OK 00183 */ 00184 virtual uint8_t HandleAdrCommand(const uint8_t* payload, uint8_t index, uint8_t size, uint8_t& status); 00185 00186 /** 00187 * Validate the configuration after multiple ADR commands have been applied 00188 * @return status to be returned in MoteCommand answer 00189 */ 00190 virtual uint8_t ValidateAdrConfiguration(); 00191 00192 /** 00193 * Update duty cycle with at given frequency and time on air 00194 * @param freq frequency 00195 * @param time_on_air_ms tx time on air 00196 */ 00197 virtual void UpdateDutyCycle(uint32_t freq, uint32_t time_on_air_ms); 00198 00199 /** 00200 * Get the time the radio must be off air to comply with regulations 00201 * Time to wait may be dependent on duty-cycle restrictions per channel 00202 * Or duty-cycle of join requests if OTAA is being attempted 00203 * @return ms of time to wait for next tx opportunity 00204 */ 00205 virtual uint32_t GetTimeOffAir(); 00206 00207 /** 00208 * Get the channels in use by current channel plan 00209 * @return channel frequencies 00210 */ 00211 virtual std::vector<uint32_t> GetChannels(); 00212 00213 /** 00214 * Get the channel datarate ranges in use by current channel plan 00215 * @return channel datarate ranges 00216 */ 00217 virtual std::vector<uint8_t> GetChannelRanges(); 00218 00219 /** 00220 * Print log message for given rx window 00221 * @param wnd 1 or 2 00222 */ 00223 virtual void LogRxWindow(uint8_t wnd); 00224 00225 /** 00226 * Enable the default channels of the channel plan 00227 */ 00228 virtual void EnableDefaultChannels(); 00229 00230 /** 00231 * Called when MAC layer doesn't know about a command. 00232 * Use to add custom or new mac command handling 00233 * @return LORA_OK 00234 */ 00235 virtual uint8_t HandleMacCommand(uint8_t* payload, uint8_t& index); 00236 00237 /** 00238 * Get max payload size for current datarate 00239 * @return size in bytes 00240 */ 00241 virtual uint8_t GetMaxPayloadSize(); 00242 00243 /** 00244 * Get max payload size for given datarate 00245 * @return size in bytes 00246 */ 00247 virtual uint8_t GetMaxPayloadSize(uint8_t dr) { return ChannelPlan::GetMaxPayloadSize(dr); } 00248 00249 /** 00250 * Decrements the datarate based on TxDwellTime 00251 */ 00252 virtual void DecrementDatarate(); 00253 00254 /** 00255 * Check if this packet is a beacon and if so extract parameters needed 00256 * @param payload of potential beacon 00257 * @param size of the packet 00258 * @param [out] data extracted from the beacon if this packet was indeed a beacon 00259 * @return true if this packet is beacon, false if not 00260 */ 00261 virtual uint8_t DecodeBeacon(const uint8_t* payload, 00262 size_t size, 00263 BeaconData_t& data); 00264 00265 00266 virtual uint8_t GetMinDatarate(); 00267 00268 protected: 00269 00270 static const uint8_t AS923_TX_POWERS[8]; //!< List of available tx powers 00271 static const uint8_t AS923_RADIO_POWERS[21]; //!< List of calibrated tx powers 00272 static const uint8_t AS923_MAX_PAYLOAD_SIZE[]; //!< List of max payload sizes for each datarate 00273 static const uint8_t AS923_MAX_PAYLOAD_SIZE_400[]; //!< List of max payload sizes for each datarate 00274 static const uint8_t AS923_MAX_PAYLOAD_SIZE_REPEATER[]; //!< List of repeater compatible max payload sizes for each datarate 00275 static const uint8_t AS923_MAX_PAYLOAD_SIZE_REPEATER_400[]; //!< List of repeater compatible max payload sizes for each datarate 00276 static const uint8_t MAX_ERP_VALUES[]; //!< Lookup table for Max EIRP (dBm) codes 00277 typedef struct __attribute__((packed)) { 00278 uint8_t RFU[2]; 00279 uint8_t Time[4]; 00280 uint8_t CRC1[2]; 00281 uint8_t GwSpecific[7]; 00282 uint8_t CRC2[2]; 00283 } BCNPayload; 00284 }; 00285 } 00286 00287 #endif //__CHANNEL_PLAN_AS923_H__
Generated on Wed Jul 13 2022 04:34:59 by
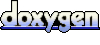