Stable version of the xDot library for mbed 5. This version of the library is suitable for deployment scenarios.
Dependents: Dot-Examples XDOT-Devicewise Dot-Examples-delujoc Dot-Examples_receive ... more
Fork of libxDot-dev-mbed5-deprecated by
ChannelPlan.h
00001 /** __ ___ ____ _ ______ __ ____ __ ____ 00002 * / |/ /_ __/ / /_(_)__/_ __/__ ____/ / / __/_ _____ / /____ __ _ ___ / _/__ ____ 00003 * / /|_/ / // / / __/ /___// / / -_) __/ _ \ _\ \/ // (_-</ __/ -_) ' \(_-< _/ // _ \/ __/ __ 00004 * /_/ /_/\_,_/_/\__/_/ /_/ \__/\__/_//_/ /___/\_, /___/\__/\__/_/_/_/___/ /___/_//_/\__/ /_/ 00005 * Copyright (C) 2015 by Multi-Tech Systems /___/ 00006 * 00007 * 00008 * @author Jason Reiss 00009 * @date 10-31-2015 00010 * @brief lora::ChannelPlan provides an interface for LoRaWAN channel schemes 00011 * 00012 * @details 00013 * 00014 */ 00015 00016 #ifndef __CHANNEL_STRATEGY_H__ 00017 #define __CHANNEL_STRATEGY_H__ 00018 00019 #include "mbed_events.h" 00020 00021 #include "Lora.h" 00022 #include "SxRadio.h" 00023 #include <vector> 00024 00025 namespace lora { 00026 00027 class ChannelPlan { 00028 public: 00029 00030 /** 00031 * Descriptions for channel plans & region information. 00032 * Bits 0-2 represent the plan type (fixed or dynamic) 00033 * 0b000 cannot be used as plans may line up with old definitions and cause much badness 00034 * 0b001 fixed channel plans 00035 * 0b010 dynamic channel plans 00036 * 0b011 - 0b111 RFU 00037 * Bits 3-7 represent the specific channel plan/region within the plan type 00038 */ 00039 enum PlanType { 00040 FIXED = 0x20, 00041 DYNAMIC = 0x40, 00042 }; 00043 00044 enum Plan { 00045 EU868_OLD = 0x00, 00046 US915_OLD = 0x01, 00047 AU915_OLD = 0x02, 00048 00049 FB_EU868 = 0x00, 00050 FB_US915 = 0x01, 00051 FB_AU915 = 0x02, 00052 00053 FB_868 = 0x00, 00054 FB_915 = 0x01, 00055 00056 US915 = FIXED | 0x00, 00057 AU915 = FIXED | 0x01, 00058 00059 EU868 = DYNAMIC | 0x00, 00060 IN865 = DYNAMIC | 0x01, 00061 AS923 = DYNAMIC | 0x02, 00062 KR920 = DYNAMIC | 0x03, 00063 AS923_JAPAN = DYNAMIC | 0x04, 00064 RU864 = DYNAMIC | 0x05, 00065 00066 NONE = 0xFF, 00067 }; 00068 00069 /** 00070 * ChannelPlan constructor 00071 * @param radio SxRadio object used to set Tx/Rx config 00072 * @param settings Settings object 00073 */ 00074 ChannelPlan(SxRadio* radio, Settings* settings); 00075 00076 /** 00077 * ChannelPlan destructor 00078 */ 00079 virtual ~ChannelPlan(); 00080 00081 /** 00082 * Checks that at least one channel exist for the data rate 00083 */ 00084 virtual uint8_t ValidateAdrDatarate(uint8_t status); 00085 00086 /** 00087 * Initialize channels, datarates and duty cycle bands according to current channel plan in settings 00088 */ 00089 virtual void Init() = 0; 00090 00091 /** 00092 * Set SxRadio object to be used to set Tx/Rx config 00093 */ 00094 virtual void SetRadio(SxRadio* radio); 00095 00096 /** 00097 * Set Settings object 00098 */ 00099 virtual void SetSettings(Settings* settings); 00100 00101 /** 00102 * Setter for the event queue 00103 */ 00104 virtual void SetEventQueue(EventQueue* queue); 00105 00106 /** 00107 * Get the next channel to use to transmit 00108 * @return LORA_OK if channel was found 00109 * @return LORA_NO_CHANS_ENABLED 00110 */ 00111 virtual uint8_t GetNextChannel() = 0; 00112 00113 /** 00114 * Set the number of channels in the plan 00115 */ 00116 virtual void SetNumberOfChannels(uint8_t channels, bool resize = true); 00117 00118 /** 00119 * Get the number of channels in the plan 00120 */ 00121 virtual uint8_t GetNumberOfChannels(); 00122 00123 /** 00124 * Check if channel is enabled 00125 * @return true if enabled 00126 */ 00127 virtual bool IsChannelEnabled(uint8_t channel); 00128 00129 /** 00130 * Set a 16 bit channel mask with offset 00131 * @param index of mask to set 0:0-15, 1:16-31 ... 00132 * @param mask 16 bit mask of enabled channels 00133 * @return true 00134 */ 00135 virtual bool SetChannelMask(uint8_t index, uint16_t mask); 00136 00137 /** 00138 * Get the channel mask of currently enabled channels 00139 * @return vector containing channel bit masks 00140 */ 00141 virtual std::vector<uint16_t> GetChannelMask(); 00142 00143 /** 00144 * Add a channel to the ChannelPlan 00145 * @param index of channel, use -1 to add to end 00146 * @param channel settings to add 00147 */ 00148 virtual uint8_t AddChannel(int8_t index, Channel channel) = 0; 00149 00150 /** 00151 * Get channel at index 00152 * @return Channel 00153 */ 00154 virtual Channel GetChannel(int8_t index) = 0; 00155 00156 /** 00157 * Add a downlink channel to the ChannelPlan 00158 * Set to 0 to use the default uplink channel frequency 00159 * @param index of channel, use -1 to add to end 00160 * @param channel settings to add 00161 */ 00162 virtual uint8_t AddDownlinkChannel(int8_t index, Channel channel); 00163 00164 /** 00165 * Get channel at index 00166 * @return Channel 00167 */ 00168 virtual Channel GetDownlinkChannel(uint8_t index); 00169 00170 /** 00171 * Set number of datarates in ChannelPlan 00172 * @param datarates 00173 */ 00174 virtual void SetNumberOfDatarates(uint8_t datarates); 00175 00176 /** 00177 * Add a datarate to the ChannelPlan 00178 * @param index of datarate, use -1 to add to end 00179 * @param datarate settings to add 00180 */ 00181 virtual uint8_t AddDatarate(int8_t index, Datarate datarate); 00182 00183 /** 00184 * Get datarate at index 00185 * @return Datarate 00186 */ 00187 virtual Datarate GetDatarate(int8_t index); 00188 00189 /** 00190 * Get max payload size for current datarate 00191 * @return size in bytes 00192 */ 00193 virtual uint8_t GetMaxPayloadSize(); 00194 00195 /** 00196 * Get max payload size for a given datarate 00197 * @return size in bytes 00198 */ 00199 virtual uint8_t GetMaxPayloadSize(uint8_t dr); 00200 00201 /** 00202 * Get rx window settings for requested window 00203 * RX_1, RX_2, RX_BEACON, RX_SLOT 00204 * @param window 00205 * @return RxWindow 00206 */ 00207 virtual RxWindow GetRxWindow(uint8_t window, int8_t id = 0) = 0; 00208 00209 /** 00210 * Get current channel to use for transmitting 00211 * @param channel index of channel 00212 * @return LORA_OK 00213 */ 00214 virtual uint8_t SetTxChannel(uint8_t channel); 00215 00216 /** 00217 * Get datarate to use on the join request 00218 * @return datarate index 00219 */ 00220 virtual uint8_t GetJoinDatarate() = 0; 00221 00222 /** 00223 * Calculate the next time a join request is possible 00224 * @param size of join frame 00225 * @returns LORA_OK 00226 */ 00227 virtual uint8_t CalculateJoinBackoff(uint8_t size) = 0; 00228 00229 /** 00230 * Get the current datarate 00231 * @return Datarate 00232 */ 00233 virtual Datarate GetTxDatarate(); 00234 00235 /** 00236 * Set the current datarate 00237 * @param index of datarate 00238 * @return LORA_OK 00239 */ 00240 virtual uint8_t SetTxDatarate(uint8_t index); 00241 00242 /** 00243 * Set the datarate offset used for first receive window 00244 * @param offset 00245 * @return LORA_OK 00246 */ 00247 virtual uint8_t SetRx1Offset(uint8_t offset); 00248 00249 /** 00250 * Set the frequency for second receive window 00251 * @param freq 00252 * @return LORA_OK 00253 */ 00254 virtual uint8_t SetRx2Frequency(uint32_t freq); 00255 00256 /** 00257 * Set the datarate index used for second receive window 00258 * @param index 00259 * @return LORA_OK 00260 */ 00261 virtual uint8_t SetRx2DatarateIndex(uint8_t index); 00262 00263 /** 00264 * Get next channel and set the SxRadio tx config with current settings 00265 * @return LORA_OK 00266 */ 00267 virtual uint8_t SetTxConfig() = 0; 00268 00269 /** 00270 * Set the SxRadio rx config provided window 00271 * @param window to be opened 00272 * @param continuous keep window open 00273 * @param wnd_growth factor to increase the rx window by 00274 * @param pad_ms time in milliseconds to add to computed window size 00275 * @return LORA_OK 00276 */ 00277 virtual uint8_t SetRxConfig(uint8_t window, 00278 bool continuous, 00279 uint16_t wnd_growth = 1, 00280 uint16_t pad_ms = 0, 00281 int8_t id = 0); 00282 00283 /** 00284 * Set frequency sub band if supported by plan 00285 * @param sub_band 00286 * @return LORA_OK 00287 */ 00288 virtual uint8_t SetFrequencySubBand(uint8_t group) = 0; 00289 00290 /** 00291 * Get frequency sub band if supported by plan 00292 * @return sub band 0-8 or 0 if not supported 00293 */ 00294 virtual uint8_t GetFrequencySubBand(); 00295 00296 /** 00297 * Callback for radio to request channel change when frequency hopping 00298 * @param currentChannel 00299 */ 00300 virtual void FhssChangeChannel(uint8_t currentChannel); 00301 00302 /** 00303 * Callback for ACK timeout event 00304 * @return LORA_OK 00305 */ 00306 virtual uint8_t HandleAckTimeout(); 00307 00308 /** 00309 * Callback for Join Accept packet to load optional channels 00310 * @return LORA_OK 00311 */ 00312 virtual uint8_t HandleJoinAccept(const uint8_t* buffer, uint8_t size) = 0; 00313 00314 /** 00315 * Callback to for rx parameter setup ServerCommand 00316 * @param payload packet data 00317 * @param index of start of command buffer 00318 * @param size number of bytes in command buffer 00319 * @param[out] status to be returned in MoteCommand answer 00320 * @return LORA_OK 00321 */ 00322 virtual uint8_t HandleRxParamSetup(const uint8_t* payload, uint8_t index, uint8_t size, uint8_t& status) = 0; 00323 00324 /** 00325 * Callback to for new channel ServerCommand 00326 * @param payload packet data 00327 * @param index of start of command buffer 00328 * @param size number of bytes in command buffer 00329 * @param[out] status to be returned in MoteCommand answer 00330 * @return LORA_OK 00331 */ 00332 virtual uint8_t HandleNewChannel(const uint8_t* payload, uint8_t index, uint8_t size, uint8_t& status) = 0; 00333 00334 /** 00335 * Callback to for downlink channel request ServerCommand 00336 * @param payload packet data 00337 * @param index of start of command buffer 00338 * @param size number of bytes in command buffer 00339 * @param[out] status to be returned in MoteCommand answer 00340 * @return LORA_OK 00341 */ 00342 virtual uint8_t HandleDownlinkChannelReq(const uint8_t* payload, uint8_t index, uint8_t size, uint8_t& status); 00343 00344 /** 00345 * Callback to for ping slot channel request ServerCommand 00346 * @param payload packet data 00347 * @param index of start of command buffer 00348 * @param size number of bytes in command buffer 00349 * @param[out] status to be returned in MoteCommand answer 00350 * @return LORA_OK 00351 */ 00352 virtual uint8_t HandlePingSlotChannelReq(const uint8_t* payload, uint8_t index, uint8_t size, uint8_t& status) = 0; 00353 00354 /** 00355 * Callback to for beacon frequency request ServerCommand 00356 * @param payload packet data 00357 * @param index of start of command buffer 00358 * @param size number of bytes in command buffer 00359 * @param[out] status to be returned in MoteCommand answer 00360 * @return LORA_OK 00361 */ 00362 virtual uint8_t HandleBeaconFrequencyReq(const uint8_t* payload, uint8_t index, uint8_t size, uint8_t& status) = 0; 00363 00364 /** 00365 * Callback to for adaptive datarate ServerCommand 00366 * @param payload packet data 00367 * @param index of start of command buffer 00368 * @param size number of bytes in command buffer 00369 * @param[out] status to be returned in MoteCommand answer 00370 * @return LORA_OK 00371 */ 00372 virtual uint8_t HandleAdrCommand(const uint8_t* payload, uint8_t index, uint8_t size, uint8_t& status) = 0; 00373 00374 /** 00375 * Validate the configuration after multiple ADR commands have been applied 00376 * @return status to be returned in MoteCommand answer 00377 */ 00378 virtual uint8_t ValidateAdrConfiguration() = 0; 00379 00380 /** 00381 * Check that Rf Frequency is within channel plan range 00382 * @param freq frequency in Hz 00383 * @return true if valid frequency 00384 */ 00385 virtual bool CheckRfFrequency(uint32_t freq); 00386 00387 /** 00388 * Flag for ADR 00389 * @return true if ADR is enable in settings 00390 */ 00391 virtual bool IsAdrEnabled(); 00392 00393 /** 00394 * Flag if ADR ACK should be sent in next packet 00395 * @return true when flag should be set 00396 */ 00397 virtual bool AdrAckReq(); 00398 00399 /** 00400 * Increment the ADR counter to track when ADR ACK request should be sent 00401 * @return current value 00402 */ 00403 virtual uint8_t IncAdrCounter(); 00404 00405 /** 00406 * Reset the ADR counter when valid downlink is received from network server 00407 */ 00408 virtual void ResetAdrCounter(); 00409 00410 /** 00411 * Get the time the radio must be off air to comply with regulations 00412 * Time to wait may be dependent on duty-cycle restrictions per channel 00413 * Or duty-cycle of join requests if OTAA is being attempted 00414 * @return ms of time to wait for next tx opportunity 00415 */ 00416 virtual uint32_t GetTimeOffAir() = 0; 00417 00418 /** 00419 * Get the channels in use by current channel plan 00420 * @return channel frequencies 00421 */ 00422 virtual std::vector<uint32_t> GetChannels() = 0; 00423 00424 /** 00425 * Get the downlink channels in use by current channel plan 00426 * @return channel frequencies 00427 */ 00428 virtual std::vector<uint32_t> GetDownlinkChannels(); 00429 00430 /** 00431 * Get the channel datarate ranges in use by current channel plan 00432 * @return channel datarate ranges 00433 */ 00434 virtual std::vector<uint8_t> GetChannelRanges() = 0; 00435 00436 /** 00437 * Set the time off air for the given duty band 00438 * @param band index 00439 * @param time off air in ms 00440 */ 00441 virtual void SetDutyBandTimeOff(uint8_t band, uint32_t timeoff); 00442 00443 /** 00444 * Get the time off air for the given duty band 00445 * @param band index 00446 * @return time off air in ms 00447 */ 00448 virtual uint32_t GetDutyBandTimeOff(uint8_t band); 00449 00450 /** 00451 * Get the number of duty bands in the current channel plan 00452 * @return number of bands 00453 */ 00454 virtual uint8_t GetNumDutyBands(); 00455 00456 /** 00457 * Get the duty band index for the given frequency 00458 * @param freq frequency in Hz 00459 * @return index of duty band 00460 */ 00461 virtual int8_t GetDutyBand(uint32_t freq); 00462 00463 /** 00464 * Add duty band 00465 * @param index of duty band or -1 to append 00466 * @param band DutyBand definition 00467 * @return LORA_OK 00468 */ 00469 virtual uint8_t AddDutyBand(int8_t index, DutyBand band); 00470 00471 /** 00472 * Update duty cycle with current settings 00473 */ 00474 void UpdateDutyCycle(uint8_t bytes); 00475 00476 /** 00477 * Update duty cycle with at given frequency and time on air 00478 * @param freq frequency 00479 * @param time_on_air_ms tx time on air 00480 */ 00481 virtual void UpdateDutyCycle(uint32_t freq, uint32_t time_on_air_ms); 00482 00483 /** 00484 * Get time on air with current settings 00485 * @param bytes number of bytes to be sent 00486 * @param cfg for setting up the radio before getting time on air 00487 */ 00488 virtual uint32_t GetTimeOnAir(uint8_t bytes, RadioCfg_t cfg = TX_RADIO_CFG); 00489 00490 /** 00491 * Reset the duty timers with the current time off air 00492 */ 00493 virtual void ResetDutyCycleTimer(); 00494 00495 /** 00496 * Print log message for given rx window 00497 * @param wnd 1 or 2 00498 */ 00499 virtual void LogRxWindow(uint8_t wnd) = 0; 00500 00501 /** 00502 * Indicator of P2P mode 00503 * @return true if enabled 00504 */ 00505 virtual bool P2PEnabled(); 00506 00507 /** 00508 * Ack timeout for P2P mode 00509 * @return timeout in ms 00510 */ 00511 virtual uint16_t P2PTimeout(); 00512 00513 /** 00514 * Ack backoff for P2P mode 00515 * @return backoff in ms 00516 */ 00517 virtual uint16_t P2PBackoff(); 00518 00519 /** 00520 * Enable the default channels of the channel plan 00521 */ 00522 virtual void EnableDefaultChannels() = 0; 00523 00524 /** 00525 * Callback for radio thread to signal 00526 */ 00527 virtual void MacEvent(); 00528 00529 /** 00530 * Called when MAC layer doesn't know about a command. 00531 * Use to add custom or new mac command handling 00532 * @return LORA_OK 00533 */ 00534 virtual uint8_t HandleMacCommand(uint8_t* payload, uint8_t& index); 00535 00536 virtual void DecrementDatarate(); 00537 virtual void IncrementDatarate(); 00538 00539 virtual std::string GetPlanName(); 00540 virtual uint8_t GetPlan(); 00541 virtual bool IsPlanFixed(); 00542 virtual bool IsPlanDynamic(); 00543 static bool IsPlanFixed(uint8_t plan); 00544 static bool IsPlanDynamic(uint8_t plan); 00545 virtual uint32_t GetMinFrequency(); 00546 virtual uint32_t GetMaxFrequency(); 00547 00548 virtual uint8_t GetMinDatarate(); 00549 virtual uint8_t GetMaxDatarate(); 00550 virtual uint8_t GetMinDatarateOffset(); 00551 virtual uint8_t GetMaxDatarateOffset(); 00552 00553 virtual uint8_t GetMinRx2Datarate(); 00554 virtual uint8_t GetMaxRx2Datarate(); 00555 virtual uint8_t GetMaxTxPower(); 00556 virtual uint8_t GetMinTxPower(); 00557 00558 virtual uint16_t GetLBT_TimeUs(); 00559 virtual void SetLBT_TimeUs(uint16_t us); 00560 00561 virtual int8_t GetLBT_Threshold(); 00562 virtual void SetLBT_Threshold(int8_t rssi); 00563 00564 /** 00565 * Set LBT time and threshold to defaults 00566 */ 00567 virtual void DefaultLBT(); 00568 00569 virtual bool ListenBeforeTalk(); 00570 00571 /** 00572 * use to clear downlink channels on join 00573 */ 00574 virtual void ClearChannels(); 00575 00576 /** 00577 * Check if this packet is a beacon and if so extract parameters needed 00578 * @param payload of potential beacon 00579 * @param size of the packet 00580 * @param [out] data extracted from the beacon if this packet was indeed a beacon 00581 * @return true if this packet is beacon, false if not 00582 */ 00583 virtual uint8_t DecodeBeacon(const uint8_t* payload, 00584 size_t size, 00585 BeaconData_t& data) = 0; 00586 00587 /** 00588 * Update class B beacon and ping slot settings if frequency hopping enabled 00589 * @param time received in the last beacon 00590 * @param period of the beacon 00591 * @param devAddr of this end device 00592 */ 00593 virtual void FrequencyHop(uint32_t time, uint32_t period, uint32_t devAddr) { } 00594 00595 00596 /* 00597 * Get default number of channels for a plan 00598 */ 00599 virtual uint8_t GetNumDefaultChans(); 00600 00601 /* 00602 * Search enabled channels for lowest available datarate 00603 */ 00604 virtual uint8_t GetMinEnabledDatarate(); 00605 00606 SxRadio* GetRadio(); //!< Get pointer to the SxRadio object or assert if it is null 00607 Settings* GetSettings(); //!< Get pointer to the settings object or assert if it is null 00608 00609 protected: 00610 00611 /** 00612 * 16 bit ITU-T CRC implementation 00613 */ 00614 uint16_t CRC16(const uint8_t* data, size_t size); 00615 00616 uint8_t _txChannel; //!< Current channel for transmit 00617 uint8_t _txFrequencySubBand; //!< Current frequency sub band for hybrid operation 00618 00619 std::vector<Datarate> _datarates; //!< List of datarates 00620 00621 std::vector<Channel> _channels; //!< List of channels for transmit 00622 std::vector<Channel> _dlChannels; //!< List of channels for receive if changed from default 00623 00624 std::vector<DutyBand> _dutyBands; //!< List of duty bands to limit radio time on air 00625 00626 uint8_t _maxTxPower; //!< Max Tx power for channel Plan 00627 uint8_t _minTxPower; 00628 00629 uint32_t _minFrequency; //!< Minimum Frequency 00630 uint32_t _maxFrequency; //!< Maximum Frequency 00631 00632 uint8_t _minDatarate; //!< Minimum datarate to accept in ADR request 00633 uint8_t _maxDatarate; //!< Maximum datarate to accept in ADR request 00634 00635 uint8_t _minRx2Datarate; //!< Minimum datarate to accept in for Rx2 00636 uint8_t _maxRx2Datarate; //!< Maximum datarate to accept in for Rx2 00637 uint8_t _minDatarateOffset; //!< Minimum datarate offset to accept 00638 uint8_t _maxDatarateOffset; //!< Maximum datarate offset to accept 00639 00640 uint32_t _freqUBase125k; //!< Start of 125K uplink channels 00641 uint32_t _freqUStep125k; //!< Step between 125K uplink channels 00642 uint32_t _freqUBase500k; //!< Start of 500K uplink channels 00643 uint32_t _freqUStep500k; //!< Step between 500K uplink channels 00644 uint32_t _freqDBase500k; //!< Start of 500K downlink channels 00645 uint32_t _freqDStep500k; //!< Step between 500K downlink channels 00646 00647 uint8_t _numChans; //!< Number of total channels in plan 00648 uint8_t _numChans125k; //!< Number of 125K channels in plan 00649 uint8_t _numChans500k; //!< Number of 500K channels in plan 00650 uint8_t _numDefaultChans; //!< Number of default channels in plan 00651 00652 uint16_t _LBT_TimeUs; //!< Sample time in us for LBT 00653 int8_t _LBT_Threshold; //!< Threshold in dBm for LBT 00654 00655 std::vector<uint16_t> _channelMask; //!< Bit mask for currently enabled channels 00656 00657 Timer _dutyCycleTimer; //!< Timer for tracking time-off-air 00658 int _txDutyEvtId; //!< Event ID for expiration of time-off-air 00659 00660 bool _txDutyCyclePending; //!< Flag for pending duty cycle event 00661 00662 void OnTxDutyCycleEvent(); //!< Callback for duty cycle event 00663 void OnTxDutyCycleEventBottom(); //!< Callback for duty cycle event 00664 00665 static const uint8_t* TX_POWERS; //!< List of available tx powers 00666 static const uint8_t* RADIO_POWERS; //!< List of available tx powers 00667 static const uint8_t* MAX_PAYLOAD_SIZE; //!< List of max payload sizes for each datarate 00668 static const uint8_t* MAX_PAYLOAD_SIZE_REPEATER; //!< List of repeater compatible max payload sizes for each datarate 00669 00670 uint8_t _beaconSize; 00671 00672 uint8_t _plan; 00673 std::string _planName; 00674 00675 private: 00676 00677 SxRadio* _radio; //!< Injected SxRadio dependency 00678 Settings* _settings; //!< Current settings 00679 EventQueue* _evtQueue; //!< mbed Event Queue 00680 }; 00681 } 00682 00683 #endif
Generated on Wed Jul 13 2022 04:34:59 by
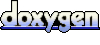