fota lib for mdot
Embed:
(wiki syntax)
Show/hide line numbers
SxRadioEvents.h
00001 /* 00002 / _____) _ | | 00003 ( (____ _____ ____ _| |_ _____ ____| |__ 00004 \____ \| ___ | (_ _) ___ |/ ___) _ \ 00005 _____) ) ____| | | || |_| ____( (___| | | | 00006 (______/|_____)_|_|_| \__)_____)\____)_| |_| 00007 (C)2013 Semtech 00008 00009 Description: Generic radio driver definition 00010 00011 License: Revised BSD License, see LICENSE.TXT file include in the project 00012 00013 Maintainer: Miguel Luis and Gregory Cristian 00014 */ 00015 #ifndef __SXRADIOEVENTS_H__ 00016 #define __SXRADIOEVENTS_H__ 00017 00018 /*! 00019 * \brief Radio driver callback functions 00020 */ 00021 class SxRadioEvents 00022 { 00023 public: 00024 /*! 00025 * \brief Tx Start callback prototype. 00026 */ 00027 virtual void TxStart( void ) {} 00028 /*! 00029 * \brief Tx Done callback prototype. 00030 */ 00031 virtual void TxDone( void ) {} 00032 /*! 00033 * \brief Tx Timeout callback prototype. 00034 */ 00035 virtual void TxTimeout( void ) {} 00036 /*! 00037 * \brief Rx Done callback prototype. 00038 * 00039 * \param [IN] payload Received buffer pointer 00040 * \param [IN] size Received buffer size 00041 * \param [IN] rssi RSSI value computed while receiving the frame [dBm] 00042 * \param [IN] snr Raw SNR value given by the radio hardware 00043 * FSK : N/A ( set to 0 ) 00044 * LoRa: SNR value is two's complement in 1/4 dB 00045 */ 00046 virtual void RxDone( uint8_t *payload, uint16_t size, int16_t rssi, int8_t snr ) {} 00047 /*! 00048 * \brief Rx Timeout callback prototype. 00049 */ 00050 virtual void RxTimeout( void ) {} 00051 /*! 00052 * \brief Rx Error callback prototype. 00053 */ 00054 virtual void RxError( void ) {} 00055 /*! 00056 * \brief FHSS Change Channel callback prototype. 00057 * 00058 * \param [IN] currentChannel Index number of the current channel 00059 */ 00060 virtual void FhssChangeChannel( uint8_t currentChannel ) {} 00061 /*! 00062 * \brief CAD Done callback prototype. 00063 * 00064 * \param [IN] channelActivityDetected Channel Activity detected during the CAD 00065 */ 00066 virtual void CadDone( bool channelActivityDetected ) {} 00067 /*! 00068 * \brief Mac Event callback prototype. 00069 */ 00070 virtual void MacEvent( void ) {} 00071 00072 virtual void LinkIdle(void) {} 00073 }; 00074 00075 #endif // __SXRADIOEVENTS_H__ 00076
Generated on Tue Jul 12 2022 12:07:34 by
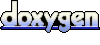