Serial library for MTS Socket Modem Arduino Shield devices from Multi-Tech Systems
Dependents: mDot_AT_firmware mtsas mtsas MTDOT-EVB-LinkCheck-AL ... more
MTSSerialFlowControl.h
00001 #ifndef MTSSERIALFLOWCONTROL_H 00002 #define MTSSERIALFLOWCONTROL_H 00003 00004 #include "MTSSerial.h" 00005 00006 namespace mts 00007 { 00008 00009 /** This class derives from MTSBufferedIO/MTSSerial and provides a buffered wrapper to the 00010 * standard mbed Serial class along with generic RTS/CTS HW flow control. Since it 00011 * depends only on the mbed Serial, DigitalOut and DigitalIn classes for accessing 00012 * the serial data, this class is inherently portable accross different mbed platforms 00013 * and provides HW flow control even when not natively supported by the processors 00014 * serial port. If HW flow control is not needed, use MTSSerial instead. It should also 00015 * be noted that the RTS/CTS functionality in this class is implemented as a DTE device. 00016 */ 00017 class MTSSerialFlowControl : public MTSSerial 00018 { 00019 public: 00020 /** Creates a new MTSSerialFlowControl object that can be used to talk to an mbed serial 00021 * port through internal SW buffers. Note that this class also adds the ability to use 00022 * RTS/CTS HW Flow Conrtol through and standard mbed DigitalIn and DigitalOut pins. 00023 * The RTS and CTS functionality assumes this is a DTE device. 00024 * 00025 * @param TXD the transmit data pin on the desired mbed serial interface. 00026 * @param RXD the receive data pin on the desired mbed serial interface. 00027 * @param RTS the DigitalOut pin that RTS will be attached to. (DTE) 00028 * @param CTS the DigitalIn pin that CTS will be attached to. (DTE) 00029 * @param txBufferSize the size in bytes of the internal SW transmit buffer. The 00030 * default is 256 bytes. 00031 * @param rxBufferSize the size in bytes of the internal SW receive buffer. The 00032 * default is 256 bytes. 00033 */ 00034 MTSSerialFlowControl(PinName TXD, PinName RXD, PinName RTS, PinName CTS, int txBufSize = 256, int rxBufSize = 256); 00035 00036 /** Destructs an MTSSerialFlowControl object and frees all related resources. 00037 */ 00038 ~MTSSerialFlowControl(); 00039 00040 //Overriden from MTSBufferedIO to support flow control correctly 00041 virtual void rxClear(); 00042 00043 private: 00044 void notifyStartSending(); // Used to set cts start signal 00045 void notifyStopSending(); // Used to set cts stop signal 00046 00047 //This device acts as a DTE 00048 bool rxReadyFlag; //Tracks state change for rts signaling 00049 DigitalOut rts; // Used to tell DCE to send or not send data 00050 DigitalIn cts; // Used to check if DCE is ready for data 00051 int highThreshold; // High water mark for setting cts to stop 00052 int lowThreshold; // Low water mark for setting cts to start 00053 00054 virtual void handleRead(); // Method for handling data to be read 00055 virtual void handleWrite(); // Method for handling data to be written 00056 }; 00057 00058 } 00059 00060 #endif /* MTSSERIALFLOWCONTROL */
Generated on Thu Jul 14 2022 05:10:02 by
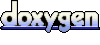