
Factory firmware for the MultiTech Dotbox (MTDOT-BOX) and EVB (MTDOT-EVB) products.
Dependencies: NCP5623B GpsParser ISL29011 libmDot-mbed5 MTS-Serial MMA845x DOGS102 MPL3115A2
Mode.h
00001 /* Copyright (c) <2016> <MultiTech Systems>, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00005 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00006 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00007 * furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 00019 #ifndef __MODE_H__ 00020 #define __MODE_H__ 00021 00022 #include "DOGS102.h" 00023 #include "ButtonHandler.h" 00024 #include "mDot.h" 00025 #include "LoRaHandler.h" 00026 #include "GPSPARSER.h" 00027 #include "SensorHandler.h" 00028 #include "ISL29011.h" 00029 #include "MMA845x.h" 00030 #include "MPL3115A2.h" 00031 #include "FileName.h" 00032 #include "ChannelPlans.h" 00033 00034 class Mode { 00035 public: 00036 typedef enum { 00037 single = 0, 00038 sweep 00039 } DataType; 00040 00041 typedef struct { 00042 DataType type; 00043 int32_t index; 00044 bool status; 00045 bool gps_lock; 00046 int32_t gps_sats; 00047 GPSPARSER::longitude gps_longitude; 00048 GPSPARSER::latitude gps_latitude; 00049 int16_t gps_altitude; 00050 struct tm gps_time; 00051 LoRaHandler::LoRaLink link; 00052 uint8_t data_rate; 00053 uint32_t power; 00054 } DataItem; 00055 00056 typedef struct { 00057 MMA845x_DATA accel_data; 00058 MPL3115A2_DATA baro_data; 00059 uint16_t lux_data_raw; 00060 uint32_t pressure_raw; 00061 float light; 00062 float pressure; 00063 float altitude; 00064 float temperature; 00065 } SensorItem; 00066 00067 Mode(DOGS102* lcd, ButtonHandler* buttons, mDot* dot, LoRaHandler* lora, GPSPARSER* gps, SensorHandler* sensors); 00068 ~Mode(); 00069 00070 virtual bool start() = 0; 00071 00072 protected: 00073 bool deleteDataFile(); 00074 bool appendDataFile(const DataItem& data); 00075 void updateData(DataItem& data, DataType type, bool status); 00076 void updateSensorData(SensorItem& data); 00077 uint32_t getIndex(DataType type); 00078 00079 std::vector<uint8_t> formatSurveyData(DataItem& data); 00080 std::vector<uint8_t> formatSensorData(SensorItem& data); 00081 00082 DOGS102* _lcd; 00083 ButtonHandler* _buttons; 00084 mDot* _dot; 00085 LoRaHandler* _lora; 00086 GPSPARSER* _gps; 00087 SensorHandler* _sensors; 00088 osThreadId _main_id; 00089 uint32_t _index; 00090 uint8_t _band; 00091 uint8_t _sub_band; 00092 uint8_t _data_rate; 00093 uint32_t _power; 00094 uint32_t _next_tx; 00095 ButtonHandler::ButtonEvent _be; 00096 LoRaHandler::LoRaStatus _ls; 00097 LoRaHandler::LoRaLink _link_check_result; 00098 uint8_t _state; 00099 bool _send_data; 00100 bool _gps_available; 00101 uint8_t _initial_data_rate; 00102 uint8_t _initial_power; 00103 }; 00104 00105 #endif
Generated on Tue Jul 12 2022 17:02:18 by
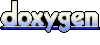