
Factory firmware for the MultiTech Dotbox (MTDOT-BOX) and EVB (MTDOT-EVB) products.
Dependencies: NCP5623B GpsParser ISL29011 libmDot-mbed5 MTS-Serial MMA845x DOGS102 MPL3115A2
ModeRegion.cpp
00001 /* Copyright (c) <2018> <MultiTech Systems>, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00005 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00006 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00007 * furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 00019 #include "ChannelPlans.h" 00020 #include "ModeRegion.h" 00021 #include "MTSLog.h" 00022 00023 ModeRegion::ModeRegion( 00024 DOGS102* lcd, 00025 ButtonHandler* buttons, 00026 mDot* dot, 00027 LoRaHandler* lora, 00028 GPSPARSER* gps, 00029 SensorHandler* sensors, 00030 const char* file_cp) 00031 : Mode(lcd, buttons, dot, lora, gps, sensors) 00032 , _file_cp(file_cp) 00033 { 00034 // Add all of our supported regions 00035 _regions.push_back("US915"); 00036 _regions.push_back("AU915"); 00037 _regions.push_back("EU868"); 00038 _regions.push_back("AS923"); 00039 _regions.push_back("AS923-JAPAN"); 00040 _regions.push_back("KR920"); 00041 _regions.push_back("IN865"); 00042 00043 _menu = new LayoutScrollSelect(lcd, _regions, "Select Region"); 00044 } 00045 00046 ModeRegion::~ModeRegion() 00047 { 00048 delete _menu; 00049 } 00050 00051 bool ModeRegion::start() 00052 { 00053 std::string region(""); 00054 00055 // clear any stale signals 00056 osSignalClear(_main_id, buttonSignal | loraSignal); 00057 00058 _menu->display(); 00059 00060 while (true) { 00061 osEvent e = Thread::signal_wait(0, 250); 00062 if (e.status == osEventSignal) { 00063 if (e.value.signals & buttonSignal) { 00064 _be = _buttons->getButtonEvent(); 00065 00066 switch (_be) { 00067 case ButtonHandler::sw1_press: 00068 region = _menu->select(); 00069 setFreqBand(region); 00070 return true; 00071 case ButtonHandler::sw2_press: 00072 _menu->scroll(); 00073 break; 00074 case ButtonHandler::sw1_hold: 00075 return true; 00076 default: 00077 break; 00078 } 00079 } 00080 } 00081 } 00082 } 00083 00084 void ModeRegion::setFreqBand(std::string region) 00085 { 00086 uint8_t cp[] = { 0 }; 00087 mDot::mdot_file file = _dot->openUserFile(_file_cp, mDot::FM_RDWR); 00088 00089 // First delete the existing plan 00090 lora::ChannelPlan *plan = _dot->getChannelPlan(); 00091 if (plan != NULL) 00092 delete plan; 00093 00094 // Construct a channel plan for the selected region 00095 if (region == "US915") { 00096 plan = new lora::ChannelPlan_US915(); 00097 cp[0] = CP_US915; 00098 } else if (region == "AU915") { 00099 plan = new lora::ChannelPlan_AU915(); 00100 cp[0] = CP_AU915; 00101 } else if (region == "EU868") { 00102 plan = new lora::ChannelPlan_EU868(); 00103 cp[0] = CP_EU868; 00104 } else if (region == "AS923") { 00105 plan = new lora::ChannelPlan_AS923(); 00106 cp[0] = CP_AS923; 00107 } else if (region == "AS923-JAPAN") { 00108 plan = new lora::ChannelPlan_AS923_Japan(); 00109 cp[0] = CP_AS923_JAPAN; 00110 } else if (region == "KR920") { 00111 plan = new lora::ChannelPlan_KR920(); 00112 cp[0] = CP_KR920; 00113 } else if (region == "IN865") { 00114 plan = new lora::ChannelPlan_IN865(); 00115 cp[0] = CP_IN865; 00116 } 00117 00118 if (_dot->setChannelPlan(plan) == mDot::MDOT_OK) { 00119 // Update the channel plan config 00120 _dot->seekUserFile(file, 0, SEEK_SET); 00121 _dot->writeUserFile(file, cp, 1); 00122 _dot->closeUserFile(file); 00123 _band = _dot->getFrequencyBand(); 00124 logInfo("Setting channel plan to %s", region.c_str()); 00125 } else { 00126 logError("Failed to set channel plan to %s", region.c_str()); 00127 } 00128 }
Generated on Tue Jul 12 2022 17:02:18 by
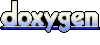