
Factory firmware for the MultiTech Dotbox (MTDOT-BOX) and EVB (MTDOT-EVB) products.
Dependencies: NCP5623B GpsParser ISL29011 libmDot-mbed5 MTS-Serial MMA845x DOGS102 MPL3115A2
ModeGps.h
00001 /* Copyright (c) <2016> <MultiTech Systems>, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00005 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00006 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00007 * furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 00019 #ifndef __MODEGPS_H__ 00020 #define __MODEGPS_H__ 00021 00022 #include "Mode.h" 00023 #include "ModeJoin.h" 00024 #include "LayoutHelp.h" 00025 #include "LayoutSurveyGps.h" 00026 00027 class ModeGps : public Mode{ 00028 public: 00029 ModeGps(DOGS102* lcd, ButtonHandler* buttons, mDot* dot, LoRaHandler* lora, GPSPARSER* gps, SensorHandler* sensors, ModeJoin* join); 00030 ~ModeGps(); 00031 bool start(); 00032 00033 private: 00034 enum {DATA_RATE, 00035 FSB, 00036 PADDING, 00037 POWER, 00038 INTERVAL 00039 }; 00040 enum {BAND_CHANGE, 00041 SENDING, 00042 PARAMETERS 00043 }; 00044 00045 LayoutHelp _help; 00046 LayoutSurveyGps _sem; 00047 LayoutJoin _sem_join; 00048 00049 ModeJoin* _join; 00050 00051 Timer _send_timer, _button_timer; 00052 00053 float _temp_C; 00054 std::vector<uint8_t> _send_data; 00055 uint8_t _parameter, _padding, _interval, _max_padding; 00056 bool _drAll, _link_check, _GPS; 00057 string _Sw1, _Sw2; 00058 00059 struct tm _time; 00060 mDot::snr_stats _snr; 00061 mDot::rssi_stats _rssi; 00062 GPSPARSER::latitude _latitude; 00063 GPSPARSER::longitude _longitude; 00064 00065 void init(); 00066 void send(); 00067 void setBand(); 00068 void sendData(); 00069 void formatData(); 00070 void drIncrement(); 00071 void updateScreen(); 00072 void editParameter(); 00073 void changeDataRate(); 00074 void changeParameter(); 00075 string intToString(int num); 00076 }; 00077 #endif
Generated on Tue Jul 12 2022 17:02:18 by
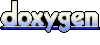