
Factory firmware for the MultiTech Dotbox (MTDOT-BOX) and EVB (MTDOT-EVB) products.
Dependencies: NCP5623B GpsParser ISL29011 libmDot-mbed5 MTS-Serial MMA845x DOGS102 MPL3115A2
LoRaHandler.h
00001 /* Copyright (c) <2016> <MultiTech Systems>, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00005 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00006 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00007 * furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 00019 #ifndef __LORAHANDLER_H__ 00020 #define __LORAHANDLER_H__ 00021 00022 #include "mDot.h" 00023 00024 #define loraSignal (int32_t)0x02 00025 00026 class LoRaHandler { 00027 public: 00028 typedef enum { 00029 none = 0, 00030 busy, 00031 link_check_success, 00032 send_success, 00033 join_success, 00034 link_check_failure, 00035 send_failure, 00036 join_failure, 00037 send_failure_payload 00038 } LoRaStatus; 00039 00040 typedef struct { 00041 bool status; 00042 mDot::link_check up; 00043 mDot::ping_response down; 00044 } LoRaLink; 00045 00046 typedef enum { 00047 green = 0, 00048 red 00049 } LedColor; 00050 00051 LoRaHandler(osThreadId main, mDot* dot); 00052 ~LoRaHandler(); 00053 00054 bool linkCheck(); 00055 bool send(std::vector<uint8_t> data); 00056 bool join(); 00057 bool action(uint8_t cmd); 00058 LoRaStatus getStatus(); 00059 LoRaLink getLinkCheckResults(); 00060 uint32_t getJoinAttempts(); 00061 void resetJoinAttempts(); 00062 void blinker(); 00063 void resetActivityLed(); 00064 00065 osThreadId _main; 00066 Thread _thread; 00067 LoRaStatus _status; 00068 LoRaLink _link; 00069 mDot* _dot; 00070 Mutex _mutex; 00071 uint32_t _join_attempts; 00072 DigitalInOut _activity_led; 00073 Ticker _tick; 00074 }; 00075 00076 #endif 00077
Generated on Tue Jul 12 2022 17:02:18 by
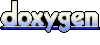