
Factory firmware for the MultiTech Dotbox (MTDOT-BOX) and EVB (MTDOT-EVB) products.
Dependencies: NCP5623B GpsParser ISL29011 libmDot-mbed5 MTS-Serial MMA845x DOGS102 MPL3115A2
LayoutDemoSampling.cpp
00001 /* Copyright (c) <2016> <MultiTech Systems>, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00005 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00006 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00007 * furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 00019 #include "LayoutDemoSampling.h" 00020 00021 LayoutDemoSampling::LayoutDemoSampling(DOGS102* lcd) 00022 : Layout(lcd), 00023 _lAccx(0, 0, "AccX"), 00024 _lAccy(6, 0, "AccY"), 00025 _lAccz(12, 0, "AccZ"), 00026 _lPres(0, 2, "Press="), 00027 _lAlt(0, 3, "Alt="), 00028 _lTemp(0, 4, "Temp="), 00029 _lLight(0, 5, "Light="), 00030 _fAccx(0, 1, 5), 00031 _fAccy(6, 1, 5), 00032 _fAccz(12, 1, 5), 00033 _fPres(6, 2, 11), 00034 _fAlt(4, 3, 13), 00035 _fTemp(5, 4, 12), 00036 _fLight(6, 5, 11), 00037 _fInfo(0, 6, 17), 00038 _fSw1(9, 7, 8), 00039 _fSw2(0, 7, 8) 00040 {} 00041 00042 LayoutDemoSampling::~LayoutDemoSampling() {} 00043 00044 void LayoutDemoSampling::display() { 00045 clear(); 00046 startUpdate(); 00047 00048 writeLabel(_lAccx); 00049 writeLabel(_lAccy); 00050 writeLabel(_lAccz); 00051 writeLabel(_lPres); 00052 writeLabel(_lAlt); 00053 writeLabel(_lTemp); 00054 writeLabel(_lLight); 00055 00056 endUpdate(); 00057 } 00058 00059 void LayoutDemoSampling::updateInfo(std::string info) { 00060 writeField(_fInfo, info, true); 00061 } 00062 00063 void LayoutDemoSampling::updateSw1(std::string sw1) { 00064 writeField(_fSw1, sw1, true); 00065 } 00066 00067 void LayoutDemoSampling::updateSw2(std::string sw2) { 00068 writeField(_fSw2, sw2, true); 00069 } 00070 00071 void LayoutDemoSampling::updateCountdown(uint32_t seconds) { 00072 char buf[32]; 00073 size_t size; 00074 std::string s; 00075 00076 // make sure the string version is used 00077 writeField(_fInfo, string("No Free Channel"), true); 00078 size = snprintf(buf, sizeof(buf), "%lu s", seconds); 00079 for (int i = 0; i < _fSw1._maxSize - size; i++) 00080 s.append(" "); 00081 s.append(buf, size); 00082 writeField(_fSw1, s, true); 00083 } 00084 00085 void LayoutDemoSampling::updateInterval(uint32_t seconds) { 00086 char buf[32]; 00087 size_t size; 00088 00089 if (seconds < 60) 00090 size = snprintf(buf, sizeof(buf), "Interval %lu s", seconds); 00091 else if (seconds < 60 * 60) 00092 size = snprintf(buf, sizeof(buf), "Interval %lu min", seconds / 60); 00093 else 00094 size = snprintf(buf, sizeof(buf), "Interval %lu hr", seconds / (60 * 60)); 00095 00096 writeField(_fInfo, buf, size, true); 00097 } 00098 00099 void LayoutDemoSampling::updateAccelerationX(int16_t x) { 00100 char buf[16]; 00101 size_t size; 00102 float fx = (float)x; 00103 fx /= 1024; 00104 // We can only display 5 characters. 00105 // For numbers < -1, we display -#.#g. For example -1.3g 00106 if(fx < -1){ 00107 size = snprintf(buf, sizeof(buf), "%4.1fg", fx); 00108 } 00109 // For numbers > -1 and < 0, we display -.##g. For example -.13g 00110 else if(fx < 0){ 00111 size = snprintf(buf, sizeof(buf), "%4.2fg", fx); 00112 for(uint8_t i = 1; i < 5; i++ ){ 00113 buf[i] = buf[i+1]; 00114 } 00115 } 00116 // For numbers > 0, we display #.##g. For example 0.13g. 00117 else{ 00118 size = snprintf(buf, sizeof(buf), "%4.2fg", fx); 00119 } 00120 writeField(_fAccx, buf, size, true); 00121 } 00122 00123 void LayoutDemoSampling::updateAccelerationY(int16_t y) { 00124 char buf[16]; 00125 size_t size; 00126 float fy = (float)y; 00127 fy /= 1024; 00128 if(fy < -1){ 00129 size = snprintf(buf, sizeof(buf), "%4.1fg", fy); 00130 } 00131 else if(fy < 0){ 00132 size = snprintf(buf, sizeof(buf), "%4.2fg", fy); 00133 for(uint8_t i = 1; i < 5; i++ ){ 00134 buf[i] = buf[i+1]; 00135 } 00136 } 00137 else{ 00138 size = snprintf(buf, sizeof(buf), "%4.2fg", fy); 00139 } 00140 writeField(_fAccy, buf, size, true); 00141 } 00142 00143 void LayoutDemoSampling::updateAccelerationZ(int16_t z) { 00144 char buf[16]; 00145 size_t size; 00146 float fz = (float)z; 00147 fz /= 1024; 00148 if(fz < -1){ 00149 size = snprintf(buf, sizeof(buf), "%1.1fg", fz); 00150 } 00151 else if(fz < 0){ 00152 size = snprintf(buf, sizeof(buf), "%4.2fg", fz); 00153 for(uint8_t i = 1; i < 5; i++ ){ 00154 buf[i] = buf[i+1]; 00155 } 00156 } 00157 else{ 00158 size = snprintf(buf, sizeof(buf), "%1.2fg", fz); 00159 } 00160 writeField(_fAccz, buf, size, true); 00161 } 00162 00163 void LayoutDemoSampling::updatePressure(float pressure) { 00164 char buf[16]; 00165 size_t size; 00166 size = snprintf(buf, sizeof(buf), "%3.2f kPa", pressure/1000); 00167 writeField(_fPres, buf, size, true); 00168 } 00169 00170 void LayoutDemoSampling::updateAltitude(float altitude) { 00171 char buf[16]; 00172 size_t size; 00173 size = snprintf(buf, sizeof(buf), "%5.2f m", altitude); 00174 writeField(_fAlt, buf, size, true); 00175 } 00176 00177 void LayoutDemoSampling::updateTemperature(float temperature) { 00178 char buf[16]; 00179 size_t size; 00180 size = snprintf(buf, sizeof(buf), "%3.2f C", temperature); 00181 writeField(_fTemp, buf, size, true); 00182 } 00183 00184 void LayoutDemoSampling::updateLight(float light) { 00185 char buf[16]; 00186 size_t size; 00187 size = snprintf(buf, sizeof(buf), "%4.2f lx", light); 00188 writeField(_fLight, buf, size, true); 00189 }
Generated on Tue Jul 12 2022 17:02:18 by
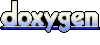