
Factory firmware for the MultiTech Dotbox (MTDOT-BOX) and EVB (MTDOT-EVB) products.
Dependencies: NCP5623B GpsParser ISL29011 libmDot-mbed5 MTS-Serial MMA845x DOGS102 MPL3115A2
LayoutData.cpp
00001 /* Copyright (c) <2016> <MultiTech Systems>, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00005 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00006 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00007 * furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 00019 #include "LayoutData.h" 00020 00021 LayoutData::LayoutData(DOGS102* lcd) 00022 : Layout(lcd), 00023 _lDr(8, 0, "DR"), 00024 _lPwr(13, 0, "P"), 00025 _lUp(0, 1, "UP Mgn"), 00026 _lGw(10, 1, "Gw"), 00027 _lDown(0, 2, "DWN -"), 00028 _lSurveyFailed(0, 1, "Survey Failed"), 00029 _lDbm(9, 2, "dbm"), 00030 _lAlt(0, 6, "Alt"), 00031 _fId(0, 0, 5), 00032 _fDr(10, 0, 2), 00033 _fPwr(14, 0, 2), 00034 _fUpMargin(7, 1, 2), 00035 _fGw(13, 1, 2), 00036 _fRssiDown(5, 2, 3), 00037 _fSnrDown(13, 2, 4), 00038 _fGpsLat(0, 4, 17), 00039 _fGpsLong(0, 3, 17), 00040 _fGpsTime(0, 5, 17), 00041 _fAlt(4,6,13), 00042 _fSw1(12, 7, 4), 00043 _fSw2(0, 7, 4) 00044 {} 00045 00046 LayoutData::~LayoutData() {} 00047 00048 void LayoutData::display(){ 00049 clear(); 00050 startUpdate(); 00051 writeLabel(_lDr); 00052 writeLabel(_lPwr); 00053 endUpdate(); 00054 } 00055 00056 void LayoutData::noData(){ 00057 clear(); 00058 writeField(_fGpsLong, string(" No Survey Data"), true); 00059 } 00060 00061 void LayoutData::errorData(){ 00062 clear(); 00063 writeField(_fGpsLong, string(" Error opening,"), true); 00064 writeField(_fGpsLat, string("survey data file."), true); 00065 } 00066 00067 void LayoutData::updateSw1(string str){ 00068 writeField(_fSw1, str, true); 00069 } 00070 00071 void LayoutData::updateSw2(string str){ 00072 writeField(_fSw2, str, true); 00073 } 00074 00075 bool LayoutData::updateAll(singleLine& line){ 00076 clear(); 00077 startUpdate(); 00078 //this data should always exist 00079 writeLabel(_lDr); 00080 writeLabel(_lPwr); 00081 writeField(_fId, line.id, true); 00082 writeField(_fDr, line.dataRate, true); 00083 writeField(_fPwr, line.power, true); 00084 //check if survey pass/fail 00085 if(line.status=="S") { 00086 writeLabel(_lUp); 00087 writeLabel(_lDown); 00088 writeLabel(_lGw); 00089 writeLabel(_lDbm); 00090 writeField(_fGw, line.gateways, true); 00091 writeField(_fUpMargin, line.margin, true); 00092 writeField(_fRssiDown, line.rssiD, true); 00093 writeField(_fSnrDown, line.snrD, true); 00094 } else writeLabel(_lSurveyFailed); 00095 //check if gps data exists 00096 if(line.lock!="0") { 00097 writeLabel(_lAlt); 00098 writeField(_fGpsLat, line.lat, true); 00099 writeField(_fGpsLong, line.lng, true); 00100 writeField(_fGpsTime, line.time, true); 00101 writeField(_fAlt, line.alt + " m", true); 00102 } else writeField(_fGpsLong, string("No GPS Data"), true); 00103 endUpdate(); 00104 }
Generated on Tue Jul 12 2022 17:02:18 by
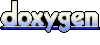