
Factory firmware for the MultiTech Dotbox (MTDOT-BOX) and EVB (MTDOT-EVB) products.
Dependencies: NCP5623B GpsParser ISL29011 libmDot-mbed5 MTS-Serial MMA845x DOGS102 MPL3115A2
CmdGetSurveyDataFile.cpp
00001 /* Copyright (c) <2016> <MultiTech Systems>, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00005 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00006 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00007 * furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 00019 #include "CmdGetSurveyDataFile.h" 00020 #include "FileName.h" 00021 #include "MTSLog.h" 00022 00023 CmdGetSurveyDataFile::CmdGetSurveyDataFile(mDot* dot, mts::MTSSerial& serial) : 00024 Command(dot, "Get Survey Data File", "AT+GSDF", "Outputs the survey data file contents to the command port"), _serial(serial) 00025 { 00026 _help = std::string(text()) + ": " + std::string(desc()); 00027 } 00028 00029 uint32_t CmdGetSurveyDataFile::action(std::vector<std::string> args) 00030 { 00031 mDot::mdot_file file; 00032 int buf_size = 512; 00033 char buf[buf_size]; 00034 int read = 0; 00035 int read_size = 0; 00036 00037 file = _dot->openUserFile(file_name, mDot::FM_RDONLY); 00038 if (file.fd < 0) { 00039 setErrorMessage("Failed to open file"); 00040 return 1; 00041 } 00042 00043 // print header line with column labels 00044 _serial.writef("ID,Status,Lock,Lat,Long,Alt,Time,Gateways,Margin,RSSIdown,SNRdown,DataRate,Power\r\n"); 00045 00046 while (read < file.size) { 00047 read_size = (file.size - read) > buf_size ? buf_size : file.size - read; 00048 int size = _dot->readUserFile(file, (void*)buf, read_size); 00049 if (size < 0) { 00050 setErrorMessage("Failed to read file"); 00051 _dot->closeUserFile(file); 00052 return 1; 00053 } 00054 00055 for (int i = 0; i < size; i++) { 00056 if (buf[i] == '\n') 00057 _serial.writef("\r\n"); 00058 else 00059 _serial.writef("%c", buf[i]); 00060 } 00061 00062 read += size; 00063 } 00064 00065 _dot->closeUserFile(file); 00066 00067 return 0; 00068 }
Generated on Tue Jul 12 2022 17:02:17 by
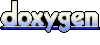