
Factory firmware for the MultiTech Dotbox (MTDOT-BOX) and EVB (MTDOT-EVB) products.
Dependencies: NCP5623B GpsParser ISL29011 libmDot-mbed5 MTS-Serial MMA845x DOGS102 MPL3115A2
CmdFrequencyBand.cpp
00001 #include "CmdFrequencyBand.h" 00002 #include "ChannelPlans.h" 00003 CmdFrequencyBand::CmdFrequencyBand(mDot* dot, mts::MTSSerial& serial) 00004 : Command(dot,"Current Frequency Band", "AT+FREQ", "Select Frequency Band 'US915', 'AU915', 'EU868', 'AS923', 'AS923-JAPAN','KR920', or 'IN865'") 00005 , _serial(serial) 00006 { 00007 _help = std::string(text()) + ": " + std::string(desc()); 00008 _usage = ""; 00009 _queryable = true; 00010 } 00011 00012 uint32_t CmdFrequencyBand::action(std::vector<std::string> args) 00013 { 00014 if (args.size() == 1) 00015 { 00016 // using getChannelPlanName here instead of mDot::FrequencyBandStr allows AT firmware to properly display custom channel plan names 00017 if(_dot->getVerbose()) 00018 _serial.writef("Frequency Band: "); 00019 00020 _serial.writef("%s\r\n", _dot->getChannelPlanName().c_str()); 00021 } 00022 00023 else if (args.size() == 2) 00024 { 00025 std::string band_str = mts::Text::toUpper(args[1]); 00026 lora::ChannelPlan* plan = _dot->getChannelPlan(); 00027 mDot::mdot_file file = _dot->openUserFile("ChannelPlan", mDot::FM_RDWR); 00028 _dot->seekUserFile(file, 0, SEEK_SET); 00029 uint8_t cp[] = {0}; 00030 00031 if (mDot::FrequencyBandStr(lora::ChannelPlan::US915) == band_str) { 00032 if(plan != NULL) delete plan; 00033 plan = new lora::ChannelPlan_US915(); 00034 cp[0] = CP_US915; 00035 } 00036 else if (mDot::FrequencyBandStr(lora::ChannelPlan::AU915) == band_str) { 00037 if(plan != NULL) delete plan; 00038 plan = new lora::ChannelPlan_AU915(); 00039 cp[0] = CP_AU915; 00040 } 00041 else if (mDot::FrequencyBandStr(lora::ChannelPlan::EU868) == band_str) { 00042 if(plan != NULL) delete plan; 00043 plan = new lora::ChannelPlan_EU868(); 00044 cp[0] = CP_EU868; 00045 } 00046 else if (mDot::FrequencyBandStr(lora::ChannelPlan::AS923) == band_str) { 00047 if(plan != NULL) delete plan; 00048 plan = new lora::ChannelPlan_AS923(); 00049 cp[0] = CP_AS923; 00050 } 00051 else if (mDot::FrequencyBandStr(lora::ChannelPlan::KR920) == band_str) { 00052 if(plan != NULL) delete plan; 00053 plan = new lora::ChannelPlan_KR920(); 00054 cp[0] = CP_KR920; 00055 } 00056 else if (mDot::FrequencyBandStr(lora::ChannelPlan::IN865) == band_str) { 00057 if(plan != NULL) delete plan; 00058 plan = new lora::ChannelPlan_IN865(); 00059 cp[0] = CP_IN865; 00060 } 00061 else if (mDot::FrequencyBandStr(lora::ChannelPlan::AS923_JAPAN) == band_str) { 00062 if(plan != NULL) delete plan; 00063 plan = new lora::ChannelPlan_AS923_Japan(); 00064 cp[0] = CP_AS923_JAPAN; 00065 } 00066 00067 if(cp[0] > 0) { 00068 _dot->writeUserFile(file, cp, 1); 00069 } 00070 _dot->closeUserFile(file); 00071 00072 _serial.writef("Setting Plan \r\n"); 00073 00074 if (_dot->setChannelPlan(plan) != mDot::MDOT_OK) { 00075 setErrorMessage(_dot->getLastError()); 00076 return 1; 00077 } 00078 } 00079 return 0; 00080 } 00081 00082 bool CmdFrequencyBand::verify(std::vector<std::string> args) 00083 { 00084 if (args.size() == 1) 00085 return true; 00086 00087 if (args.size() == 2) 00088 { 00089 std::string band = mts::Text::toUpper(args[1]); 00090 00091 if (mDot::FrequencyBandStr(lora::ChannelPlan::US915) != band && 00092 mDot::FrequencyBandStr(lora::ChannelPlan::AU915) != band && 00093 mDot::FrequencyBandStr(lora::ChannelPlan::EU868) != band && 00094 mDot::FrequencyBandStr(lora::ChannelPlan::AS923) != band && 00095 mDot::FrequencyBandStr(lora::ChannelPlan::KR920) != band && 00096 mDot::FrequencyBandStr(lora::ChannelPlan::IN865) != band && 00097 mDot::FrequencyBandStr(lora::ChannelPlan::AS923_JAPAN) != band) 00098 { 00099 setErrorMessage("Invalid parameter, expects (US915,AU915,EU868,AS923,AS923-JAPAN,KR920,IN865)"); 00100 return false; 00101 } 00102 return true; 00103 } 00104 }
Generated on Tue Jul 12 2022 17:02:17 by
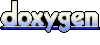