
Example programs for MultiTech Dot devices demonstrating how to use the Dot devices and the Dot libraries for LoRa communication.
Dependencies: ISL29011
Dependents: Dot-Examples-delujoc
manual_example.cpp
00001 #include "dot_util.h" 00002 #include "RadioEvent.h" 00003 00004 #if ACTIVE_EXAMPLE == MANUAL_EXAMPLE 00005 00006 ///////////////////////////////////////////////////////////////////////////// 00007 // -------------------- DOT LIBRARY REQUIRED ------------------------------// 00008 // * Because these example programs can be used for both mDot and xDot // 00009 // devices, the LoRa stack is not included. The libmDot library should // 00010 // be imported if building for mDot devices. The libxDot library // 00011 // should be imported if building for xDot devices. // 00012 // * https://developer.mbed.org/teams/MultiTech/code/libmDot-dev-mbed5/ // 00013 // * https://developer.mbed.org/teams/MultiTech/code/libmDot-mbed5/ // 00014 // * https://developer.mbed.org/teams/MultiTech/code/libxDot-dev-mbed5/ // 00015 // * https://developer.mbed.org/teams/MultiTech/code/libxDot-mbed5/ // 00016 ///////////////////////////////////////////////////////////////////////////// 00017 00018 ///////////////////////////////////////////////////////////// 00019 // * these options must match the settings on your gateway // 00020 // * edit their values to match your configuration // 00021 // * frequency sub band is only relevant for the 915 bands // 00022 ///////////////////////////////////////////////////////////// 00023 static uint8_t network_address[] = { 0x01, 0x02, 0x03, 0x04 }; 00024 static uint8_t network_session_key[] = { 0x01, 0x02, 0x03, 0x04, 0x01, 0x02, 0x03, 0x04, 0x01, 0x02, 0x03, 0x04, 0x01, 0x02, 0x03, 0x04 }; 00025 static uint8_t data_session_key[] = { 0x01, 0x02, 0x03, 0x04, 0x01, 0x02, 0x03, 0x04, 0x01, 0x02, 0x03, 0x04, 0x01, 0x02, 0x03, 0x04 }; 00026 static uint8_t frequency_sub_band = 6; 00027 static lora::NetworkType network_type = lora::PUBLIC_LORAWAN; 00028 static uint8_t join_delay = 5; 00029 static uint8_t ack = 1; 00030 static bool adr = true; 00031 00032 // deepsleep consumes slightly less current than sleep 00033 // in sleep mode, IO state is maintained, RAM is retained, and application will resume after waking up 00034 // in deepsleep mode, IOs float, RAM is lost, and application will start from beginning after waking up 00035 // if deep_sleep == true, device will enter deepsleep mode 00036 static bool deep_sleep = true; 00037 00038 mDot* dot = NULL; 00039 lora::ChannelPlan* plan = NULL; 00040 00041 mbed::UnbufferedSerial pc(USBTX, USBRX); 00042 00043 #if defined(TARGET_XDOT_L151CC) 00044 I2C i2c(I2C_SDA, I2C_SCL); 00045 ISL29011 lux(i2c); 00046 #else 00047 AnalogIn lux(XBEE_AD0); 00048 #endif 00049 00050 int main() { 00051 // Custom event handler for automatically displaying RX data 00052 RadioEvent events; 00053 00054 pc.baud(115200); 00055 00056 #if defined(TARGET_XDOT_L151CC) 00057 i2c.frequency(400000); 00058 #endif 00059 00060 mts::MTSLog::setLogLevel(mts::MTSLog::TRACE_LEVEL); 00061 00062 // Create channel plan 00063 plan = create_channel_plan(); 00064 assert(plan); 00065 00066 dot = mDot::getInstance(plan); 00067 assert(dot); 00068 00069 // attach the custom events handler 00070 dot->setEvents(&events); 00071 00072 if (!dot->getStandbyFlag()) { 00073 logInfo("mbed-os library version: %d.%d.%d", MBED_MAJOR_VERSION, MBED_MINOR_VERSION, MBED_PATCH_VERSION); 00074 00075 // start from a well-known state 00076 logInfo("defaulting Dot configuration"); 00077 dot->resetConfig(); 00078 dot->resetNetworkSession(); 00079 00080 // make sure library logging is turned on 00081 dot->setLogLevel(mts::MTSLog::INFO_LEVEL); 00082 00083 // update configuration if necessary 00084 if (dot->getJoinMode() != mDot::MANUAL) { 00085 logInfo("changing network join mode to MANUAL"); 00086 if (dot->setJoinMode(mDot::MANUAL) != mDot::MDOT_OK) { 00087 logError("failed to set network join mode to MANUAL"); 00088 } 00089 } 00090 // in MANUAL join mode there is no join request/response transaction 00091 // as long as the Dot is configured correctly and provisioned correctly on the gateway, it should be able to communicate 00092 // network address - 4 bytes (00000001 - FFFFFFFE) 00093 // network session key - 16 bytes 00094 // data session key - 16 bytes 00095 // to provision your Dot with a Conduit gateway, follow the following steps 00096 // * ssh into the Conduit 00097 // * provision the Dot using the lora-query application: http://www.multitech.net/developer/software/lora/lora-network-server/ 00098 // lora-query -a 01020304 A 0102030401020304 <your Dot's device ID> 01020304010203040102030401020304 01020304010203040102030401020304 00099 // * if you change the network address, network session key, or data session key, make sure you update them on the gateway 00100 // to provision your Dot with a 3rd party gateway, see the gateway or network provider documentation 00101 update_manual_config(network_address, network_session_key, data_session_key, frequency_sub_band, network_type, ack); 00102 00103 // enable or disable Adaptive Data Rate 00104 dot->setAdr(adr); 00105 00106 // Configure the join delay 00107 dot->setJoinDelay(join_delay); 00108 00109 // save changes to configuration 00110 logInfo("saving configuration"); 00111 if (!dot->saveConfig()) { 00112 logError("failed to save configuration"); 00113 } 00114 00115 // display configuration 00116 display_config(); 00117 } else { 00118 // restore the saved session if the dot woke from deepsleep mode 00119 // useful to use with deepsleep because session info is otherwise lost when the dot enters deepsleep 00120 logInfo("restoring network session from NVM"); 00121 dot->restoreNetworkSession(); 00122 } 00123 00124 while (true) { 00125 uint16_t light; 00126 std::vector<uint8_t> tx_data; 00127 00128 #if defined(TARGET_XDOT_L151CC) 00129 // configure the ISL29011 sensor on the xDot-DK for continuous ambient light sampling, 16 bit conversion, and maximum range 00130 lux.setMode(ISL29011::ALS_CONT); 00131 lux.setResolution(ISL29011::ADC_16BIT); 00132 lux.setRange(ISL29011::RNG_64000); 00133 00134 // get the latest light sample and send it to the gateway 00135 light = lux.getData(); 00136 tx_data.push_back((light >> 8) & 0xFF); 00137 tx_data.push_back(light & 0xFF); 00138 logInfo("light: %lu [0x%04X]", light, light); 00139 send_data(tx_data); 00140 00141 // put the LSL29011 ambient light sensor into a low power state 00142 lux.setMode(ISL29011::PWR_DOWN); 00143 #else 00144 // get some dummy data and send it to the gateway 00145 light = lux.read_u16(); 00146 tx_data.push_back((light >> 8) & 0xFF); 00147 tx_data.push_back(light & 0xFF); 00148 logInfo("light: %lu [0x%04X]", light, light); 00149 send_data(tx_data); 00150 #endif 00151 00152 // if going into deepsleep mode, save the session so we don't need to join again after waking up 00153 // not necessary if going into sleep mode since RAM is retained 00154 if (deep_sleep) { 00155 logInfo("saving network session to NVM"); 00156 dot->saveNetworkSession(); 00157 } 00158 00159 // ONLY ONE of the three functions below should be uncommented depending on the desired wakeup method 00160 //sleep_wake_rtc_only(deep_sleep); 00161 //sleep_wake_interrupt_only(deep_sleep); 00162 sleep_wake_rtc_or_interrupt(deep_sleep); 00163 } 00164 00165 return 0; 00166 } 00167 00168 #endif
Generated on Tue Jul 12 2022 13:03:22 by
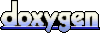