
Example programs for MultiTech Dot devices demonstrating how to use the Dot devices and the Dot libraries for LoRa communication.
Dependencies: ISL29011
Dependents: Dot-Examples-delujoc
RadioEvent.h
00001 #ifndef __RADIO_EVENT_H__ 00002 #define __RADIO_EVENT_H__ 00003 00004 #include "dot_util.h" 00005 #include "mDotEvent.h" 00006 #include "Fota.h" 00007 #include "example_config.h" 00008 00009 class RadioEvent : public mDotEvent 00010 { 00011 00012 public: 00013 RadioEvent() {} 00014 00015 virtual ~RadioEvent() {} 00016 00017 virtual void PacketRx(uint8_t port, uint8_t *payload, uint16_t size, int16_t rssi, int16_t snr, lora::DownlinkControl ctrl, uint8_t slot, uint8_t retries, uint32_t address, uint32_t fcnt, bool dupRx) { 00018 mDotEvent::PacketRx(port, payload, size, rssi, snr, ctrl, slot, retries, address, fcnt, dupRx); 00019 00020 #if ACTIVE_EXAMPLE == FOTA_EXAMPLE 00021 if(port == 200 || port == 201 || port == 202) { 00022 Fota::getInstance()->processCmd(payload, port, size); 00023 } 00024 #endif 00025 } 00026 00027 /*! 00028 * MAC layer event callback prototype. 00029 * 00030 * \param [IN] flags Bit field indicating the MAC events occurred 00031 * \param [IN] info Details about MAC events occurred 00032 */ 00033 virtual void MacEvent(LoRaMacEventFlags* flags, LoRaMacEventInfo* info) { 00034 00035 if (mts::MTSLog::getLogLevel() == mts::MTSLog::TRACE_LEVEL) { 00036 std::string msg = "OK"; 00037 switch (info->Status) { 00038 case LORAMAC_EVENT_INFO_STATUS_ERROR: 00039 msg = "ERROR"; 00040 break; 00041 case LORAMAC_EVENT_INFO_STATUS_TX_TIMEOUT: 00042 msg = "TX_TIMEOUT"; 00043 break; 00044 case LORAMAC_EVENT_INFO_STATUS_RX_TIMEOUT: 00045 msg = "RX_TIMEOUT"; 00046 break; 00047 case LORAMAC_EVENT_INFO_STATUS_RX_ERROR: 00048 msg = "RX_ERROR"; 00049 break; 00050 case LORAMAC_EVENT_INFO_STATUS_JOIN_FAIL: 00051 msg = "JOIN_FAIL"; 00052 break; 00053 case LORAMAC_EVENT_INFO_STATUS_DOWNLINK_FAIL: 00054 msg = "DOWNLINK_FAIL"; 00055 break; 00056 case LORAMAC_EVENT_INFO_STATUS_ADDRESS_FAIL: 00057 msg = "ADDRESS_FAIL"; 00058 break; 00059 case LORAMAC_EVENT_INFO_STATUS_MIC_FAIL: 00060 msg = "MIC_FAIL"; 00061 break; 00062 default: 00063 break; 00064 } 00065 logTrace("Event: %s", msg.c_str()); 00066 00067 logTrace("Flags Tx: %d Rx: %d RxData: %d RxSlot: %d LinkCheck: %d JoinAccept: %d", 00068 flags->Bits.Tx, flags->Bits.Rx, flags->Bits.RxData, flags->Bits.RxSlot, flags->Bits.LinkCheck, flags->Bits.JoinAccept); 00069 logTrace("Info: Status: %d ACK: %d Retries: %d TxDR: %d RxPort: %d RxSize: %d RSSI: %d SNR: %d Energy: %d Margin: %d Gateways: %d", 00070 info->Status, info->TxAckReceived, info->TxNbRetries, info->TxDatarate, info->RxPort, info->RxBufferSize, 00071 info->RxRssi, info->RxSnr, info->Energy, info->DemodMargin, info->NbGateways); 00072 } 00073 00074 if (flags->Bits.Rx) { 00075 00076 logInfo("Rx %d bytes", info->RxBufferSize); 00077 00078 if (info->RxBufferSize > 0) { 00079 #if ACTIVE_EXAMPLE != FOTA_EXAMPLE 00080 // print RX data as string and hexadecimal 00081 std::string rx((const char*)info->RxBuffer, info->RxBufferSize); 00082 printf("Rx data: %s [%s]\r\n", rx.c_str(), mts::Text::bin2hexString(info->RxBuffer, info->RxBufferSize).c_str()); 00083 #endif 00084 } 00085 } 00086 } 00087 00088 00089 #if ACTIVE_EXAMPLE == FOTA_EXAMPLE 00090 virtual void ServerTime(uint32_t seconds, uint8_t sub_seconds) { 00091 mDotEvent::ServerTime(seconds, sub_seconds); 00092 00093 Fota::getInstance()->setClockOffset(seconds); 00094 } 00095 #endif 00096 }; 00097 00098 #endif 00099
Generated on Tue Jul 12 2022 13:03:22 by
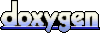