
AT command firmware for MultiTech Dot devices.
Fork of mDot_AT_firmware by
main.cpp
00001 #include "mbed.h" 00002 #include "mDot.h" 00003 #include "CommandTerminal.h" 00004 #include "ChannelPlans.h" 00005 00006 #if defined(TARGET_XDOT_L151CC) && defined(FOTA) 00007 #include "SPIFBlockDevice.h" 00008 #include "DataFlashBlockDevice.h" 00009 #endif 00010 00011 #if defined(TARGET_XDOT_L151CC) && defined(FOTA) 00012 00013 mbed::BlockDevice* ext_bd = NULL; 00014 00015 mbed::BlockDevice * mdot_override_external_block_device() 00016 { 00017 if (ext_bd == NULL) { 00018 ext_bd = new SPIFBlockDevice(); 00019 int ret = ext_bd->init(); 00020 if (ext_bd->init() < 0) { 00021 delete ext_bd; 00022 ext_bd = new DataFlashBlockDevice(); 00023 ret = ext_bd->init(); 00024 // Check for zero size because DataFlashBlockDevice doesn't 00025 // return an error if the chip is not present 00026 if ((ret < 0) || (ext_bd->size() == 0)) { 00027 delete ext_bd; 00028 ext_bd = NULL; 00029 } 00030 } 00031 00032 if (ext_bd != NULL) { 00033 logInfo("External flash device detected, type: %s, size: 0x%08x", 00034 ext_bd->get_type(), (uint32_t)ext_bd->size()); 00035 } 00036 } 00037 00038 return ext_bd; 00039 } 00040 #endif 00041 00042 #if defined(TARGET_MTS_MDOT_F411RE) // ----------------------------------------------------------- 00043 00044 #define AT_TX_PIN XBEE_DOUT 00045 #define AT_RX_PIN XBEE_DIN 00046 #define AT_RTS_PIN XBEE_RTS 00047 #define AT_CTS_PIN XBEE_CTS 00048 00049 #define DEBUG_TX USBTX 00050 #define DEBUG_RX USBRX 00051 00052 #elif defined(TARGET_XDOT_L151CC) // ----------------------------------------------------------- 00053 00054 #define AT_TX_PIN UART1_TX 00055 #define AT_RX_PIN UART1_RX 00056 #define AT_RTS_PIN UART1_RTS 00057 #define AT_CTS_PIN UART1_CTS 00058 00059 #define DEBUG_TX USBTX 00060 #define DEBUG_RX USBRX 00061 00062 #elif defined(TARGET_NUCLEO_L476RG) // ----------------------------------------------------------- 00063 00064 #define AT_TX_PIN D0 00065 #define AT_RX_PIN D1 00066 #define AT_RTS_PIN D7 00067 #define AT_CTS_PIN D8 00068 00069 #define DEBUG_TX USBTX 00070 #define DEBUG_RX USBRX 00071 00072 #elif defined(TARGET_MAX32630FTHR) // ----------------------------------------------------------- 00073 #define AT_TX_PIN UART2_TX 00074 #define AT_RX_PIN UART2_RX 00075 #define AT_RTS_PIN UART2_RTS 00076 #define AT_CTS_PIN UART2_CTS 00077 00078 #define DEBUG_TX USBTX 00079 #define DEBUG_RX USBRX 00080 00081 #include "MAX14690.h" 00082 00083 I2C i2cBus(I2C2_SDA, I2C2_SCL); // P5_7, P6_0 00084 00085 MAX14690 max14690(&i2cBus); 00086 00087 #elif defined(TARGET_MAX32660EVSYS) // ----------------------------------------------------------- 00088 00089 #if defined(SWAP_UARTS) 00090 #define AT_TX_PIN UART0_TX 00091 #define AT_RX_PIN UART0_RX 00092 #define AT_RTS_PIN NC 00093 #define AT_CTS_PIN NC 00094 00095 #define DEBUG_TX UART1C_TX 00096 #define DEBUG_RX UART1C_RX 00097 00098 #else 00099 #define AT_TX_PIN UART1C_TX 00100 #define AT_RX_PIN UART1C_RX 00101 #define AT_RTS_PIN NC 00102 #define AT_CTS_PIN NC 00103 00104 #define DEBUG_TX UART0_TX 00105 #define DEBUG_RX UART0_RX 00106 #endif 00107 00108 #include "flc.h" 00109 00110 #elif defined(TARGET_MAX32670EVKIT) || defined(TARGET_XDOT_MAX32670) // ----------------------------------------------------------- 00111 00112 #define AT_TX_PIN UART0_TX // UART0A_TX - P0_9 00113 #define AT_RX_PIN UART0_RX // UART0A_RX - P0_8 00114 #define AT_RTS_PIN UART0_RTS 00115 #define AT_CTS_PIN UART0_CTS 00116 00117 #define DEBUG_TX UART1_TX // UART1A_TX - P0_29 00118 #define DEBUG_RX UART1_RX // UART1A_RX - P0_28 00119 00120 #include "pwrseq_regs.h" 00121 #include "lp.h" 00122 00123 #else // ----------------------------------------------------------------------------------------- 00124 #error Unsupported target 00125 #endif 00126 00127 #ifndef CHANNEL_PLAN 00128 #define CHANNEL_PLAN CP_US915 00129 #endif 00130 00131 #ifndef UNIT_TEST 00132 #ifndef UNIT_TEST_MDOT_LIB 00133 00134 00135 mbed::UnbufferedSerial debug_port(DEBUG_TX, DEBUG_RX, LOG_DEFAULT_BAUD_RATE); 00136 00137 FileHandle *mbed::mbed_override_console(int fd) 00138 { 00139 return &debug_port; 00140 } 00141 00142 00143 int main() 00144 { 00145 #if defined(TARGET_XDOT_MAX32670) || defined(TARGET_XDOTES_MAX32670) 00146 00147 // Port 1 has no pins exposed but must be configured for "low power". 00148 MXC_GPIO1->en0 = 0xFFFFFFFFUL; 00149 MXC_GPIO1->outen = 0xFFFFFFFFUL; 00150 MXC_GPIO1->padctrl0 = 0xFFFFFFFFUL; 00151 MXC_GPIO1->ps = 0x00000000UL; 00152 00153 #if defined(TARGET_XDOTES_MAX32670) 00154 printf("\r\nXDOTES\r\n"); 00155 #elif defined(TARGET_XDOT_MAX32670) 00156 printf("\r\nXDOT15\r\n"); 00157 #endif 00158 00159 // Work around to be moved to SystemInit.c 00160 // MXC_PWRSEQ->lpcn &= ~(1 << 31); // Ensure ERTCO is on 00161 00162 #endif 00163 #if defined(TARGET_MAX32630FTHR) 00164 i2cBus.frequency(400000); 00165 00166 max14690.ldo2Millivolts = 3300; 00167 max14690.ldo3Millivolts = 3300; 00168 max14690.ldo2Mode = MAX14690::LDO_ENABLED; 00169 max14690.ldo3Mode = MAX14690::LDO_ENABLED; 00170 max14690.monCfg = MAX14690::MON_HI_Z; 00171 if (max14690.init() == MAX14690_ERROR) { 00172 printf("Error initializing MAX14690.\r\n"); 00173 } else { 00174 printf("MAX14690 PMIC initialization is successful.\r\n"); 00175 } 00176 #endif 00177 #if defined(DEBUG_MAC) && defined(TARGET_MTS_MDOT_F411RE) && CHANNEL_PLAN != CP_GLOBAL 00178 lora::ChannelPlan* plan = new lora::ChannelPlan_US915(); 00179 mDot* dot = mDot::getInstance(plan); 00180 #else 00181 lora::ChannelPlan* plan; 00182 #if CHANNEL_PLAN == CP_AS923 00183 plan = new lora::ChannelPlan_AS923(); 00184 #elif CHANNEL_PLAN == CP_AS923_2 00185 plan = new lora::ChannelPlan_AS923(); 00186 #elif CHANNEL_PLAN == CP_AS923_3 00187 plan = new lora::ChannelPlan_AS923(); 00188 #elif CHANNEL_PLAN == CP_AS923_4 00189 plan = new lora::ChannelPlan_AS923(); 00190 #elif CHANNEL_PLAN == CP_US915 00191 plan = new lora::ChannelPlan_US915(); 00192 #elif CHANNEL_PLAN == CP_AU915 00193 plan = new lora::ChannelPlan_AU915(); 00194 #elif CHANNEL_PLAN == CP_EU868 00195 plan = new lora::ChannelPlan_EU868(); 00196 #elif CHANNEL_PLAN == CP_KR920 00197 plan = new lora::ChannelPlan_KR920(); 00198 #elif CHANNEL_PLAN == CP_IN865 00199 plan = new lora::ChannelPlan_IN865(); 00200 #elif CHANNEL_PLAN == CP_AS923_JAPAN 00201 plan = new lora::ChannelPlan_AS923_Japan(); 00202 #elif CHANNEL_PLAN == CP_AS923_JAPAN1 00203 plan = new lora::ChannelPlan_AS923_Japan1(); 00204 #elif CHANNEL_PLAN == CP_AS923_JAPAN2 00205 plan = new lora::ChannelPlan_AS923_Japan2(); 00206 #elif CHANNEL_PLAN == CP_RU864 00207 plan = new lora::ChannelPlan_RU864(); 00208 #elif CHANNEL_PLAN == CP_CN470 00209 plan = new lora::ChannelPlan_CN470(); 00210 #elif CHANNEL_PLAN == CP_GLOBAL 00211 plan = new lora::ChannelPlan_GLOBAL(CHANNEL_PLAN); 00212 #endif 00213 mDot* dot = mDot::getInstance(plan); 00214 #endif //MTS_MDOT_F411RE 00215 assert(dot); 00216 #if CHANNEL_PLAN == CP_GLOBAL 00217 00218 delete plan; 00219 plan = new lora::ChannelPlan_GLOBAL(dot->getDefaultFrequencyBand()); 00220 dot->setChannelPlan(plan); 00221 00222 #elif defined(TARGET_MAX32660EVSYS) || defined(TARGET_MAX32630FTHR) 00223 logDebug("Loading default channel plan %02x", dot->getDefaultFrequencyBand()); 00224 switch (dot->getDefaultFrequencyBand()) { 00225 case lora::ChannelPlan::US915: 00226 case lora::ChannelPlan::US915_OLD: 00227 delete plan; 00228 plan = new lora::ChannelPlan_US915(); 00229 break; 00230 00231 case lora::ChannelPlan::AU915: 00232 case lora::ChannelPlan::AU915_OLD: 00233 delete plan; 00234 plan = new lora::ChannelPlan_AU915(); 00235 break; 00236 00237 case lora::ChannelPlan::EU868: 00238 case lora::ChannelPlan::EU868_OLD: 00239 delete plan; 00240 plan = new lora::ChannelPlan_EU868(); 00241 break; 00242 default: 00243 logInfo("Default channel plan not valid Defaulting US915"); 00244 delete plan; 00245 plan = new lora::ChannelPlan_US915(); 00246 break; 00247 } 00248 dot->setChannelPlan(plan); 00249 #elif defined(DEBUG_MAC) && defined(TARGET_MTS_MDOT_F411RE) 00250 logDebug("Loading default channel plan %02x", dot->getDefaultFrequencyBand()); 00251 switch (dot->getDefaultFrequencyBand()) { 00252 case lora::ChannelPlan::AS923: 00253 case lora::ChannelPlan::AS923_2: 00254 case lora::ChannelPlan::AS923_3: 00255 delete plan; 00256 plan = new lora::ChannelPlan_AS923(); 00257 break; 00258 00259 case lora::ChannelPlan::US915: 00260 case lora::ChannelPlan::US915_OLD: 00261 delete plan; 00262 plan = new lora::ChannelPlan_US915(); 00263 break; 00264 00265 case lora::ChannelPlan::AU915: 00266 case lora::ChannelPlan::AU915_OLD: 00267 delete plan; 00268 plan = new lora::ChannelPlan_AU915(); 00269 break; 00270 00271 case lora::ChannelPlan::EU868: 00272 case lora::ChannelPlan::EU868_OLD: 00273 delete plan; 00274 plan = new lora::ChannelPlan_EU868(); 00275 break; 00276 00277 case lora::ChannelPlan::KR920: 00278 delete plan; 00279 plan = new lora::ChannelPlan_KR920(); 00280 break; 00281 00282 case lora::ChannelPlan::AS923_JAPAN: 00283 delete plan; 00284 plan = new lora::ChannelPlan_AS923_Japan(); 00285 break; 00286 00287 case lora::ChannelPlan::AS923_JAPAN1: 00288 delete plan; 00289 plan = new lora::ChannelPlan_AS923_Japan1(); 00290 break; 00291 00292 case lora::ChannelPlan::AS923_JAPAN2: 00293 delete plan; 00294 plan = new lora::ChannelPlan_AS923_Japan2(); 00295 break; 00296 00297 case lora::ChannelPlan::IN865: 00298 delete plan; 00299 plan = new lora::ChannelPlan_IN865(); 00300 break; 00301 00302 case lora::ChannelPlan::RU864: 00303 delete plan; 00304 plan = new lora::ChannelPlan_RU864(); 00305 break; 00306 00307 default: 00308 logInfo("Default channel plan not valid Defaulting US915"); 00309 delete plan; 00310 plan = new lora::ChannelPlan_US915(); 00311 break; 00312 } 00313 dot->setChannelPlan(plan); 00314 #endif 00315 // Seed the RNG 00316 srand(dot->getRadioRandom()); 00317 00318 mts::ATSerial serial(AT_TX_PIN, AT_RX_PIN, AT_RTS_PIN, AT_CTS_PIN, dot->getBaud()); 00319 serial.flowControl(dot->getFlowControl()); 00320 00321 debug_port.baud(dot->getDebugBaud()); 00322 00323 CommandTerminal term(serial); 00324 CommandTerminal::_dot = dot; 00325 00326 term.init(); 00327 term.start(); 00328 } 00329 00330 #endif // UNIT_TEST 00331 #endif
Generated on Wed Dec 6 2023 19:34:05 by
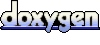