
AT command firmware for MultiTech Dot devices.
Fork of mDot_AT_firmware by
Command.h
00001 /** 00002 ****************************************************************************** 00003 * File Name : Command.h 00004 * Date : 18/04/2014 10:57:12 00005 * Description : This file provides code for command line prompt 00006 ****************************************************************************** 00007 * 00008 * COPYRIGHT(c) 2014 MultiTech Systems, Inc. 00009 * 00010 * Redistribution and use in source and binary forms, with or without modification, 00011 * are permitted provided that the following conditions are met: 00012 * 1. Redistributions of source code must retain the above copyright notice, 00013 * this list of conditions and the following disclaimer. 00014 * 2. Redistributions in binary form must reproduce the above copyright notice, 00015 * this list of conditions and the following disclaimer in the documentation 00016 * and/or other materials provided with the distribution. 00017 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00018 * may be used to endorse or promote products derived from this software 00019 * without specific prior written permission. 00020 * 00021 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00022 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00023 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00024 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00025 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00026 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00027 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00028 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00029 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00030 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00031 * 00032 ****************************************************************************** 00033 */ 00034 00035 #include "mbed.h" 00036 #include "mDot.h" 00037 #include "MTSText.h" 00038 #include <cstdlib> 00039 #include <string> 00040 #include <vector> 00041 #include "limits.h" 00042 #include "mts_at_debug.h" 00043 #include "CommandTerminal.h" 00044 00045 00046 /* Define to prevent recursive inclusion -------------------------------------*/ 00047 #ifndef __command_H 00048 #define __command_H 00049 00050 #define KEY_LENGTH 16 00051 #define EUI_LENGTH 8 00052 #define CUSTOM_FIELD_LENGTH 16 00053 #define PASSPHRASE_LENGTH 128 00054 00055 class Command : private NonCopyable<Command> 00056 { 00057 public: 00058 Command(); 00059 #if MTS_CMD_TERM_VERBOSE 00060 Command(const char* name, const char* text, const char* desc, const char* usage); 00061 #else 00062 Command(const char* text); 00063 #endif 00064 virtual ~Command() {}; 00065 00066 #if MTS_CMD_TERM_VERBOSE 00067 const char* name() const { return _name; }; 00068 const char* text() const { return _text; }; 00069 const char* desc() const { return _desc; }; 00070 const std::string help() const { 00071 return std::string(text()) + ": " + std::string(desc()); 00072 }; 00073 #else 00074 const char* text() const { return _text; }; 00075 #endif 00076 00077 virtual uint32_t action(const std::vector<std::string>& args) = 0; 00078 virtual bool verify(const std::vector<std::string>& args); 00079 00080 #if MTS_CMD_TERM_VERBOSE 00081 std::string usage() const; 00082 #endif 00083 bool queryable() const; 00084 00085 static const char newline[]; 00086 static void readByteArray(const std::string& input, std::vector<uint8_t>& out, size_t len); 00087 00088 static bool isHexString(const std::string& str, size_t bytes); 00089 static bool isBaudRate(uint32_t baud); 00090 00091 static int strToDataRate(const std::string& str); 00092 00093 static bool printRecvData(); 00094 00095 protected: 00096 bool _queryable; 00097 00098 private: 00099 #if MTS_CMD_TERM_VERBOSE 00100 const char* _name; 00101 const char* _text; 00102 const char* _desc; 00103 const char* _usage; 00104 #else 00105 const char* _text; 00106 #endif 00107 }; 00108 00109 #endif /*__ command_H */ 00110 00111 /************************ (C) COPYRIGHT MultiTech Systems, Inc *****END OF FILE****/
Generated on Wed Dec 6 2023 19:34:05 by
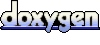