
AT command firmware for MultiTech Dot devices.
Fork of mDot_AT_firmware by
CmdSweep.cpp
00001 #include "CmdSweep.h" 00002 #include "CommandTerminal.h" 00003 00004 CmdSweep::CmdSweep() : 00005 #if MTS_CMD_TERM_VERBOSE 00006 Command("Sweep", "AT+SW", "Send un-modulated data continuously over range", "[START],[STOP],[STEP],[DURATION],[POWER]") 00007 #else 00008 Command("AT+SW") 00009 #endif 00010 { 00011 } 00012 00013 uint32_t CmdSweep::action(const std::vector<std::string>& args) { 00014 00015 int timeout = 0; 00016 int fstart = 0; 00017 int fstop = 0; 00018 int fstep = 0; 00019 int power = 20; 00020 int loops = 1; 00021 00022 if (args.size() > 1) { 00023 sscanf(args[1].c_str(), "%d", &fstart); 00024 } 00025 if (args.size() > 2) { 00026 sscanf(args[2].c_str(), "%d", &fstop); 00027 } 00028 if (args.size() > 3) { 00029 sscanf(args[3].c_str(), "%d", &fstep); 00030 } 00031 if (args.size() > 4) { 00032 sscanf(args[4].c_str(), "%d", &timeout); 00033 } 00034 if (args.size() > 5) { 00035 sscanf(args[5].c_str(), "%d", &power); 00036 } 00037 if (args.size() > 6) { 00038 sscanf(args[6].c_str(), "%d", &loops); 00039 } 00040 00041 int frequency = fstart; 00042 00043 00044 while (loops > 0) { 00045 while (frequency < fstop) { 00046 CommandTerminal::Serial()->writef("CW on %lu at %d dBm\r\n", frequency, power); 00047 CommandTerminal::Dot()->sendContinuous(true, timeout, frequency, power); 00048 frequency += fstep; 00049 if (CommandTerminal::Serial()->escaped()) { 00050 loops = 0; 00051 break; 00052 } 00053 } 00054 frequency = fstart; 00055 loops--; 00056 } 00057 00058 CommandTerminal::Serial()->clearEscaped(); 00059 00060 return 0; 00061 } 00062 00063 bool CmdSweep::verify(const std::vector<std::string>& args) 00064 { 00065 int arg; 00066 00067 if (args.size() == 1) 00068 return true; 00069 00070 // Verify all optional arguments are valid numbers 00071 // No range checking because this command is for test use 00072 for (size_t i = 1; i < args.size(); ++i) { 00073 if (sscanf(args[i].c_str(), "%d", &arg) != 1) { 00074 #if MTS_CMD_TERM_VERBOSE 00075 CommandTerminal::setErrorMessage("Invalid argument"); 00076 #endif 00077 return false; 00078 } 00079 } 00080 00081 return true; 00082 }
Generated on Wed Dec 6 2023 19:34:05 by
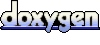