
AT command firmware for MultiTech Dot devices.
Fork of mDot_AT_firmware by
CmdMacCmd.cpp
00001 #include "CmdMacCmd.h" 00002 #include "CommandTerminal.h" 00003 00004 CmdMacCmd::CmdMacCmd() : 00005 #if MTS_CMD_TERM_VERBOSE 00006 Command("MAC Command", "AT+MAC", "Inject MAC command to MAC layer or read uplink MAC command buffer, pass '0' argument to clear buffer", "(hex:242)") 00007 #else 00008 Command("AT+MAC") 00009 #endif 00010 { 00011 } 00012 00013 uint32_t CmdMacCmd::action(const std::vector<std::string>& args) { 00014 00015 if (args.size() == 1) { 00016 std::vector<uint8_t> cmds = CommandTerminal::Dot()->getMacCommands(); 00017 00018 if (cmds.size() > 0) { 00019 for (size_t i = 0; i < cmds.size(); i++) { 00020 CommandTerminal::Serial()->writef("%02x", cmds[i]); 00021 } 00022 CommandTerminal::Serial()->writef("\r\n"); 00023 } 00024 00025 } else { 00026 if (args[1].size() == 1 && args[1] == "0") { 00027 CommandTerminal::Dot()->clearMacCommands(); 00028 } else { 00029 std::vector<uint8_t> data; 00030 int32_t code; 00031 int temp; 00032 uint32_t length = args[1].size(); 00033 00034 // Convert the ASCII hex data to binary... 00035 for (uint32_t i = 0; i < length; i += 2) { 00036 sscanf(&args[1][i], "%2x", &temp); 00037 data.push_back(temp); 00038 } 00039 00040 if ((code = CommandTerminal::Dot()->injectMacCommand(data)) != mDot::MDOT_OK) { 00041 // std::string error = mDot::getReturnCodeString(code); 00042 00043 // if (code != mDot::MDOT_NOT_JOINED) 00044 // error += +" - " + CommandTerminal::Dot()->getLastError(); 00045 00046 // CommandTerminal::setErrorMessage(CommandTerminal::Dot()->getLastError()); 00047 return 1; 00048 } 00049 } 00050 } 00051 00052 return 0; 00053 } 00054 00055 bool CmdMacCmd::verify(const std::vector<std::string>& args) { 00056 if (args.size() == 1) { 00057 return true; 00058 } else if (args.size() == 2) { 00059 00060 if (args[1].size() == 1 && args[1] == "0") { 00061 return true; 00062 } else if (args[1].size() > 484 || !isHexString(args[1], args[1].size() / 2)) { 00063 #if MTS_CMD_TERM_VERBOSE 00064 CommandTerminal::setErrorMessage("Invalid hex string, (hex:242)"); 00065 #endif 00066 return false; 00067 } 00068 00069 return true; 00070 } 00071 00072 #if MTS_CMD_TERM_VERBOSE 00073 CommandTerminal::setErrorMessage("Invalid arguments"); 00074 #endif 00075 return false; 00076 } 00077
Generated on Wed Dec 6 2023 19:34:05 by
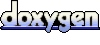