
AT command firmware for MultiTech Dot devices.
Fork of mDot_AT_firmware by
CmdLbt.cpp
00001 #include "CmdLbt.h" 00002 00003 CmdLbt::CmdLbt() : 00004 #if MTS_CMD_TERM_VERBOSE 00005 Command("Listen Before Talk", "AT+LBT", "Enable/Disable listen before talk (0,0: disable, time,threshold: enable)", "time(0-65535 us),threshold(-128-127 dBm) (0,0: disable, time,threshold: enable)") 00006 #else 00007 Command("AT+LBT") 00008 #endif 00009 { 00010 _queryable = true; 00011 } 00012 00013 uint32_t CmdLbt::action(const std::vector<std::string>& args) 00014 { 00015 if (args.size() == 1) 00016 { 00017 CommandTerminal::Serial()->writef("%" SCNu32 ",%" SCNd32 "\r\n", CommandTerminal::Dot()->getLbtTimeUs(), CommandTerminal::Dot()->getLbtThreshold()); 00018 } 00019 else 00020 { 00021 uint32_t us; 00022 int32_t rssi; 00023 00024 sscanf(args[1].c_str(), "%" SCNu32, &us); 00025 sscanf(args[2].c_str(), "%" SCNd32, &rssi); 00026 00027 CommandTerminal::Dot()->setLbtTimeUs((uint16_t)us); 00028 CommandTerminal::Dot()->setLbtThreshold((int8_t)rssi); 00029 } 00030 00031 return 0; 00032 } 00033 00034 bool CmdLbt::verify(const std::vector<std::string>& args) 00035 { 00036 if (args.size() == 1) 00037 return true; 00038 00039 if (args.size() == 3) { 00040 uint32_t us; 00041 int32_t rssi; 00042 00043 if (args[1].find("-") != std::string::npos || sscanf(args[1].c_str(), "%" SCNu32, &us) != 1 || us > 65535) { 00044 #if MTS_CMD_TERM_VERBOSE 00045 CommandTerminal::setErrorMessage("Invalid LBT time, expects 0-65535 us"); 00046 #endif 00047 return false; 00048 } 00049 00050 if (sscanf(args[2].c_str(), "%" SCNd32, &rssi) != 1 || rssi < -128 || rssi > 127) { 00051 #if MTS_CMD_TERM_VERBOSE 00052 CommandTerminal::setErrorMessage("Invalid LBT threshold, expects -128-127 dBm"); 00053 #endif 00054 return false; 00055 } 00056 00057 return true; 00058 } 00059 00060 #if MTS_CMD_TERM_VERBOSE 00061 CommandTerminal::setErrorMessage("Invalid arguments"); 00062 #endif 00063 return false; 00064 }
Generated on Wed Dec 6 2023 19:34:05 by
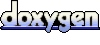