
AT command firmware for MultiTech Dot devices.
Fork of mDot_AT_firmware by
CmdFota.cpp
00001 #include "CmdFota.h" 00002 CmdFota::CmdFota() : 00003 #if MTS_CMD_TERM_VERBOSE 00004 Command("Fota and Multicast", "AT+FOTA", "Enable/disable Fota (0: off, 1: on, 2: reset, 3: time", "(0,1,2,3)") 00005 #else 00006 Command("AT+FOTA") 00007 #endif 00008 { 00009 _queryable = true; 00010 } 00011 00012 uint32_t CmdFota::action(const std::vector<std::string>& args) { 00013 if (args.size() == 1) { 00014 CommandTerminal::Serial()->writef("%d\r\n", (CommandTerminal::Dot()->getFota() || Fota::getInstance()->enable())); 00015 } 00016 else if (args.size() == 2) { 00017 if (args[1] == "0") { 00018 CommandTerminal::Dot()->setFota(false); 00019 Fota::getInstance()->enable(false); 00020 } else if(args[1] == "1") { 00021 CommandTerminal::Dot()->setFota(true); 00022 Fota::getInstance()->enable(true); 00023 } else if(args[1] == "2") { 00024 Fota::getInstance()->reset(); 00025 } else if(args[1] == "3") { 00026 CommandTerminal::Serial()->writef("%d\r\n",Fota::getInstance()->timeToStart()); 00027 } 00028 } 00029 return 0; 00030 } 00031 00032 bool CmdFota::verify(const std::vector<std::string>& args) { 00033 if (args.size() == 1) 00034 return true; 00035 00036 if (args.size() == 2) { 00037 if (args[1] != "3" && args[1] != "2" && args[1] != "1" && args[1] != "0") { 00038 #if MTS_CMD_TERM_VERBOSE 00039 CommandTerminal::setErrorMessage("Invalid parameter, expects (0: off, 1: on, 2: reset, 3: time)"); 00040 #endif 00041 return false; 00042 } 00043 00044 return true; 00045 } 00046 00047 #if MTS_CMD_TERM_VERBOSE 00048 CommandTerminal::setErrorMessage("Invalid arguments"); 00049 #endif 00050 return false; 00051 } 00052
Generated on Wed Dec 6 2023 19:34:05 by
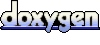