
AT command firmware for MultiTech Dot devices.
Fork of mDot_AT_firmware by
CmdDutyCycle.cpp
00001 #include "CmdDutyCycle.h" 00002 00003 00004 static inline void printDutyBand(uint8_t i) { 00005 const lora::DutyBand* band = CommandTerminal::Dot()->getDutyBand(i); 00006 if (band) { 00007 #if MTS_CMD_TERM_VERBOSE 00008 CommandTerminal::Serial()->writef("%-5" PRIu16 "\t%9" PRIu32 "\t%9" PRIu32 "\t%" PRIu16 "\r\n", 00009 (uint16_t)i, band->FrequencyMin, band->FrequencyMax, band->DutyCycle); 00010 #else 00011 CommandTerminal::Serial()->writef("%" PRIu16 ",%" PRIu32 ",%" PRIu32 ",%" PRIu16 "\r\n", 00012 (uint16_t)i, band->FrequencyMin, band->FrequencyMax, band->DutyCycle); 00013 #endif 00014 } 00015 } 00016 00017 CmdDutyCycle::CmdDutyCycle() : 00018 #if MTS_CMD_TERM_VERBOSE 00019 Command("Duty Cycle", "AT+DUTY", "Set duty cycle maximum or per band.", "<0-15> or <BAND_INDEX>,<OFF_RATIO>") 00020 #else 00021 Command("AT+DUTY") 00022 #endif 00023 { 00024 _queryable = true; 00025 } 00026 00027 uint32_t CmdDutyCycle::action(const std::vector<std::string>& args) { 00028 if (args.size() == 1) { 00029 #if MTS_CMD_TERM_VERBOSE 00030 CommandTerminal::Serial()->writef( "Max %" PRIu16 "\r\n", (uint16_t)CommandTerminal::Dot()->getDutyCycle()); 00031 CommandTerminal::Serial()->writef( "Index\tFreq Low \tFreq High\tOff Ratio\r\n"); 00032 #else 00033 CommandTerminal::Serial()->writef( "%" PRIu16 "\r\n", (uint16_t)CommandTerminal::Dot()->getDutyCycle()); 00034 #endif 00035 00036 for (uint8_t i = 0; i < CommandTerminal::Dot()->getDutyBands(); ++i) { 00037 printDutyBand(i); 00038 } 00039 00040 } else if (args.size() == 2) { 00041 int dc; 00042 sscanf(args[1].c_str(), "%d", &dc); 00043 if (CommandTerminal::Dot()->setDutyCycle(dc) != mDot::MDOT_OK) { 00044 return 1; 00045 } 00046 } else if (args.size() == 3) { 00047 int band_index; 00048 int dc; 00049 00050 sscanf(args[1].c_str(), "%d", &band_index); 00051 sscanf(args[2].c_str(), "%d", &dc); 00052 int32_t ret = CommandTerminal::Dot()->setDutyBandDutyCycle((uint8_t)band_index, dc); 00053 if (ret == mDot::MDOT_OK) { 00054 printDutyBand(band_index); 00055 } else { 00056 return 1; 00057 } 00058 } 00059 return 0; 00060 } 00061 00062 bool CmdDutyCycle::verify(const std::vector<std::string>& args) { 00063 00064 if (args.size() == 1) { 00065 return true; 00066 } else if (args.size() == 2) { 00067 int dc; 00068 00069 sscanf(args[1].c_str(), "%d", &dc); 00070 00071 if ((dc < 0) || (dc > 15)) { 00072 #if MTS_CMD_TERM_VERBOSE 00073 CommandTerminal::setErrorMessage("Invalid parameter, expects (0-15)"); 00074 #endif 00075 return false; 00076 } 00077 return true; 00078 } else if (args.size() == 3) { 00079 int band; 00080 int dc; 00081 00082 sscanf(args[1].c_str(), "%d", &band); 00083 00084 if ((band < 0) || (band >= CommandTerminal::Dot()->getDutyBands())) { 00085 #if MTS_CMD_TERM_VERBOSE 00086 CommandTerminal::setErrorMessage("Invalid parameter, expects valid duty band index"); 00087 #endif 00088 return false; 00089 } 00090 00091 sscanf(args[2].c_str(), "%d", &dc); 00092 00093 if ((dc < 0) || (dc > 65535)) { 00094 #if MTS_CMD_TERM_VERBOSE 00095 CommandTerminal::setErrorMessage("Invalid parameter, expects (0-65535)"); 00096 #endif 00097 return false; 00098 } 00099 return true; 00100 } 00101 00102 #if MTS_CMD_TERM_VERBOSE 00103 CommandTerminal::setErrorMessage("Invalid arguments"); 00104 #endif 00105 return false; 00106 }
Generated on Wed Dec 6 2023 19:34:05 by
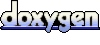