
AT command firmware for MultiTech Dot devices.
Fork of mDot_AT_firmware by
CmdDeviceClass.cpp
00001 #include "CmdDeviceClass.h" 00002 #include "CommandTerminal.h" 00003 00004 CmdDeviceClass::CmdDeviceClass() : 00005 #if MTS_CMD_TERM_VERBOSE 00006 Command("Device Class", "AT+DC", "Device class (A,B,C)", "(A,B,C)") 00007 #else 00008 Command("AT+DC") 00009 #endif 00010 { 00011 00012 _queryable = true; 00013 } 00014 00015 CmdDeviceClass::~CmdDeviceClass() { 00016 00017 } 00018 00019 uint32_t CmdDeviceClass::action(const std::vector<std::string>& args) { 00020 00021 if (args.size() == 1) { 00022 CommandTerminal::Serial()->writef("%s\r\n", CommandTerminal::Dot()->getClass().c_str()); 00023 } else { 00024 if (CommandTerminal::Dot()->setClass(args[1]) != mDot::MDOT_OK) { 00025 return 1; 00026 } 00027 } 00028 00029 return 0; 00030 } 00031 00032 bool CmdDeviceClass::verify(const std::vector<std::string>& args) 00033 { 00034 if (args.size() == 1) 00035 return true; 00036 00037 if ((args.size() == 2) && (args[1].size() == 1)) { 00038 char dev_class = args[1][0]; 00039 if (dev_class == 'A' || dev_class == 'B' || dev_class == 'C' || 00040 dev_class == 'a' || dev_class == 'b' || dev_class == 'c') { 00041 return true; 00042 } 00043 } 00044 00045 #if MTS_CMD_TERM_VERBOSE 00046 CommandTerminal::setErrorMessage("Invalid arguments"); 00047 #endif 00048 return false; 00049 }
Generated on Wed Dec 6 2023 19:34:05 by
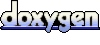