
AT command firmware for MultiTech Dot devices.
Fork of mDot_AT_firmware by
CmdChannelMask.cpp
00001 #include "CmdChannelMask.h" 00002 #include "ChannelPlan.h" 00003 00004 CmdChannelMask::CmdChannelMask() : 00005 #if MTS_CMD_TERM_VERBOSE 00006 Command("Channel Mask", "AT+CHM", "Get/set channel mask (OFFSET:0-4,MASK:0000-FFFF)", "(OFFSET:0-4,MASK:0000-FFFF)") 00007 #else 00008 Command("AT+CHM") 00009 #endif 00010 { 00011 _queryable = true; 00012 } 00013 00014 uint32_t CmdChannelMask::action(const std::vector<std::string>& args) { 00015 00016 if (args.size() == 1) { 00017 if (CommandTerminal::Dot()->getVerbose()) 00018 CommandTerminal::Serial()->writef("Channel Mask: "); 00019 00020 std::vector<uint16_t> mask = CommandTerminal::Dot()->getChannelMask(); 00021 00022 for (int i = int(mask.size()) - 1; i >= 0; i--) { 00023 CommandTerminal::Serial()->writef("%04X", mask[i]); 00024 } 00025 00026 CommandTerminal::Serial()->writef("\r\n"); 00027 00028 } else if (args.size() == 3) { 00029 int temp = 0; 00030 int offset = 0; 00031 uint16_t mask = 0; 00032 00033 sscanf(args[1].c_str(), "%d", &offset); 00034 00035 // Convert the ASCII hex data to binary... 00036 sscanf(&args[2][0], "%02x", &temp); 00037 mask = uint8_t(temp) << 8; 00038 sscanf(&args[2][2], "%02x", &temp); 00039 mask |= uint8_t(temp); 00040 00041 if (CommandTerminal::Dot()->setChannelMask(offset, mask) != mDot::MDOT_OK) { 00042 return 1; 00043 } 00044 } 00045 00046 return 0; 00047 } 00048 00049 bool CmdChannelMask::verify(const std::vector<std::string>& args) { 00050 if (args.size() == 1) 00051 return true; 00052 00053 if (args.size() == 3) { 00054 00055 int offset; 00056 if (sscanf(args[1].c_str(), "%d", &offset) != 1) { 00057 #if MTS_CMD_TERM_VERBOSE 00058 CommandTerminal::setErrorMessage("Invalid argument"); 00059 #endif 00060 return false; 00061 } 00062 00063 if (lora::ChannelPlan::IsPlanDynamic(CommandTerminal::Dot()->getFrequencyBand())) { 00064 if (offset > 0) { 00065 #if MTS_CMD_TERM_VERBOSE 00066 CommandTerminal::setErrorMessage("Invalid offset, expects (0)"); 00067 #endif 00068 return false; 00069 } 00070 } else { 00071 00072 if (offset < 0 || offset > 4) { 00073 00074 #if MTS_CMD_TERM_VERBOSE 00075 CommandTerminal::setErrorMessage("Invalid offset, expects (0-4)"); 00076 #endif 00077 return false; 00078 } 00079 } 00080 00081 if (!isHexString(args[2], 2)) { 00082 #if MTS_CMD_TERM_VERBOSE 00083 CommandTerminal::setErrorMessage("Invalid mask, expect (0000-FFFF)"); 00084 #endif 00085 return false; 00086 } 00087 00088 return true; 00089 } 00090 00091 #if MTS_CMD_TERM_VERBOSE 00092 CommandTerminal::setErrorMessage("Invalid arguments"); 00093 #endif 00094 return false; 00095 }
Generated on Wed Dec 6 2023 19:34:05 by
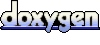