A library for talking to Multi-Tech's Cellular SocketModem Devices.
Dependents: M2X_dev axeda_wrapper_dev MTS_M2x_Example1 MTS_Cellular_Connect_Example ... more
test_TCP_Socket.h
00001 /* Universal Socket Modem Interface Library 00002 * Copyright (c) 2013 Multi-Tech Systems 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef _TEST_TCP_SOCKET_H_ 00018 #define _TEST_TCP_SOCKET_H_ 00019 00020 // 0 for shield board with wifi 00021 // 1 for shield board with cellular 00022 #define CELL_SHIELD 0 00023 00024 /* test TCP socket communication 00025 * will keep talking to server until data doesn't match expected */ 00026 00027 using namespace mts; 00028 const char PATTERN_LINE1[] = "abcdefghijklmnopqrstuvwzyzABCDEFGHIJKLMNOPQRSTUVWXYZ1234567890!@#$%^&*()[]{}|"; 00029 const char PATTERN[] = "abcdefghijklmnopqrstuvwzyzABCDEFGHIJKLMNOPQRSTUVWXYZ1234567890!@#$%^&*()[]{}|\r\n" 00030 "abcdefghijklmnopqrstuvwzyzABCDEFGHIJKLMNOPQRSTUVWXYZ1234567890!@#$%^&*()[]{}/\r\n" 00031 "abcdefghijklmnopqrstuvwzyzABCDEFGHIJKLMNOPQRSTUVWXYZ1234567890!@#$%^&*()[]{}-\r\n" 00032 "abcdefghijklmnopqrstuvwzyzABCDEFGHIJKLMNOPQRSTUVWXYZ1234567890!@#$%^&*()[]{}\\\r\n" 00033 "abcdefghijklmnopqrstuvwzyzABCDEFGHIJKLMNOPQRSTUVWXYZ1234567890!@#$%^&*()[]{}|\r\n" 00034 "abcdefghijklmnopqrstuvwzyzABCDEFGHIJKLMNOPQRSTUVWXYZ1234567890!@#$%^&*()[]{}/\r\n" 00035 "abcdefghijklmnopqrstuvwzyzABCDEFGHIJKLMNOPQRSTUVWXYZ1234567890!@#$%^&*()[]{}-\r\n" 00036 "abcdefghijklmnopqrstuvwzyzABCDEFGHIJKLMNOPQRSTUVWXYZ1234567890!@#$%^&*()[]{}\\\r\n" 00037 "abcdefghijklmnopqrstuvwzyzABCDEFGHIJKLMNOPQRSTUVWXYZ1234567890!@#$%^&*()[]{}*"; 00038 00039 const char MENU[] = "1 send ascii pattern until keypress\r\n" 00040 "2 send ascii pattern (numbered)\r\n" 00041 "3 send pattern and close socket\r\n" 00042 "4 send [ETX] and wait for keypress\r\n" 00043 "5 send [DLE] and wait for keypress\r\n" 00044 "6 send all hex values (00-FF)\r\n" 00045 "q quit\r\n" 00046 ">:\r\n"; 00047 00048 const char WELCOME[] = "Connected to: TCP test server"; 00049 00050 bool testTcpSocketIteration(); 00051 00052 void testTcpSocket() { 00053 Code code; 00054 /* this test is set up to interact with a server listening at the following address and port */ 00055 const int TEST_PORT = 7000; 00056 const std::string TEST_SERVER("204.26.122.5"); 00057 00058 printf("TCP SOCKET TESTING\r\n"); 00059 #if CELL_SHIELD 00060 Transport::setTransport(Transport::CELLULAR); 00061 MTSSerialFlowControl* serial = new MTSSerialFlowControl(PTD3, PTD2, PTA12, PTC8); 00062 serial->baud(115200); 00063 Cellular::getInstance()->init(serial); 00064 00065 printf("Setting APN\r\n"); 00066 code = Cellular::getInstance()->setApn("wap.cingular"); 00067 if(code == SUCCESS) { 00068 printf("Success!\r\n"); 00069 } else { 00070 printf("Error during APN setup [%d]\r\n", (int)code); 00071 } 00072 #else 00073 for (int i = 6; i >= 0; i = i - 2) { 00074 wait(2); 00075 printf("Waiting %d seconds...\n\r", i); 00076 } 00077 Transport::setTransport(Transport::WIFI); 00078 MTSSerial* serial = new MTSSerial(PTD3, PTD2, 256, 256); 00079 serial->baud(9600); 00080 Wifi::getInstance()->init(serial); 00081 00082 code = Wifi::getInstance()->setNetwork("your wireless network" /* SSID of wireless */, Wifi::WPA2 /* security type of wireless */, "your wireless network password" /* password for wireless */); 00083 if(code == SUCCESS) { 00084 printf("Success!\r\n"); 00085 } else { 00086 printf("Error during network setup [%d]\r\n", (int)code); 00087 } 00088 code = Wifi::getInstance()->setDeviceIP(); 00089 if(code == SUCCESS) { 00090 printf("Success!\r\n"); 00091 } else { 00092 printf("Error during IP setup [%d]\r\n", (int)code); 00093 } 00094 #endif 00095 00096 printf("Establishing Connection\r\n"); 00097 #if CELL_SHIELD 00098 if(Cellular::getInstance()->connect()) { 00099 #else 00100 if(Wifi::getInstance()->connect()) { 00101 #endif 00102 printf("Success!\r\n"); 00103 } else { 00104 printf("Error during connection\r\n"); 00105 } 00106 00107 printf("Opening a TCP Socket\r\n"); 00108 #if CELL_SHIELD 00109 if(Cellular::getInstance()->open(TEST_SERVER, TEST_PORT, IPStack::TCP)) { 00110 #else 00111 if(Wifi::getInstance()->open(TEST_SERVER, TEST_PORT, IPStack::TCP)) { 00112 #endif 00113 printf("Success!\r\n"); 00114 } else { 00115 printf("Error during TCP socket open [%s:%d]\r\n", TEST_SERVER.c_str(), TEST_PORT); 00116 } 00117 00118 //Find Welcome Message and Menu 00119 00120 int count = 0; 00121 while(testTcpSocketIteration()) { 00122 count++; 00123 printf("Successful Iterations: %d\r\n", count); 00124 } 00125 00126 printf("Closing socket\r\n"); 00127 #if CELL_SHIELD 00128 Cellular::getInstance()->close(); 00129 #else 00130 Wifi::getInstance()->close(); 00131 #endif 00132 00133 wait(10); 00134 00135 printf("Disconnecting\r\n"); 00136 #if CELL_SHIELD 00137 Cellular::getInstance()->disconnect(); 00138 #else 00139 Wifi::getInstance()->disconnect(); 00140 #endif 00141 } 00142 00143 bool testTcpSocketIteration() { 00144 Timer tmr; 00145 int bytesRead = 0; 00146 const int bufferSize = 1024; 00147 char buffer[bufferSize] = { 0 }; 00148 std::string result; 00149 00150 printf("Receiving Data\r\n"); 00151 tmr.start(); 00152 do { 00153 #if CELL_SHIELD 00154 int size = Cellular::getInstance()->read(buffer, bufferSize, 1000); 00155 #else 00156 int size = Wifi::getInstance()->read(buffer, bufferSize, 1000); 00157 #endif 00158 if(size != -1) { 00159 result.append(buffer, size); 00160 } else { 00161 printf("Error reading from socket\r\n"); 00162 return false; 00163 } 00164 printf("Total bytes read %d\r\n", result.size()); 00165 } while (tmr.read() <= 15 && bytesRead < bufferSize); 00166 00167 printf("READ: [%d] [%s]\r\n", bytesRead, result.c_str()); 00168 00169 size_t pos = result.find(PATTERN_LINE1); 00170 if(pos != std::string::npos) { 00171 //compare buffers 00172 int patternSize = sizeof(PATTERN) - 1; 00173 const char* ptr = &result.data()[pos]; 00174 bool match = true; 00175 for(int i = 0; i < patternSize; i++) { 00176 if(PATTERN[i] != ptr[i]) { 00177 printf("1ST PATTERN DOESN'T MATCH AT [%d]\r\n", i); 00178 printf("PATTERN [%02X] BUFFER [%02X]\r\n", PATTERN[i], ptr[i]); 00179 match = false; 00180 break; 00181 } 00182 } 00183 if(match) { 00184 printf("FOUND 1ST PATTERN\r\n"); 00185 } 00186 00187 pos = result.find(PATTERN_LINE1, pos + patternSize); 00188 if(pos != std::string::npos) { 00189 //compare buffers 00190 ptr = &result.data()[pos]; 00191 match = true; 00192 for(int i = 0; i < patternSize; i++) { 00193 if(PATTERN[i] != ptr[i]) { 00194 printf("2ND PATTERN DOESN'T MATCH AT [%d]\r\n", i); 00195 printf("PATTERN [%02X] BUFFER [%02X]\r\n", PATTERN[i], ptr[i]); 00196 match = false; 00197 break; 00198 } 00199 } 00200 if(match) { 00201 printf("FOUND 2ND PATTERN\r\n"); 00202 } 00203 } 00204 } 00205 00206 result.clear(); 00207 00208 printf("Writing to socket: 2\r\n"); 00209 #if CELL_SHIELD 00210 if(Cellular::getInstance()->write("2\r\n", 3, 10000) == 3) { 00211 #else 00212 if(Wifi::getInstance()->write("2\r\n", 3, 10000) == 3) { 00213 #endif 00214 printf("Successfully wrote '2'\r\n"); 00215 } else { 00216 printf("Failed to write '2'\r\n"); 00217 return false; 00218 } 00219 printf("Expecting 'how many ? >:\r\n"); 00220 #if CELL_SHIELD 00221 bytesRead = Cellular::getInstance()->read(buffer, bufferSize, 10000); 00222 #else 00223 bytesRead = Wifi::getInstance()->read(buffer, bufferSize, 10000); 00224 #endif 00225 if(bytesRead != -1) { 00226 result.append(buffer, bytesRead); 00227 printf("READ: [%d] [%s]\r\n", bytesRead, result.c_str()); 00228 if(result.find("how many") != std::string::npos) { 00229 printf("Successfully found 'how many'\r\n"); 00230 printf("Writing to socket: 2\r\n"); 00231 #if CELL_SHIELD 00232 if(Cellular::getInstance()->write("2\r\n", 3, 10000) == 3) { 00233 #else 00234 if(Wifi::getInstance()->write("2\r\n", 3, 10000) == 3) { 00235 #endif 00236 printf("Successfully wrote '2'\r\n"); 00237 } else { 00238 printf("Failed to write '2'\r\n"); 00239 return false; 00240 } 00241 } else { 00242 printf("Missing second option to menu item 2\r\n"); 00243 } 00244 } else { 00245 printf("Error reading from socket\r\n"); 00246 return false; 00247 } 00248 00249 return true; 00250 } 00251 00252 #endif
Generated on Tue Jul 12 2022 21:46:23 by
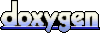