
This project serves as a template for work with the Freescale FRDM-KL46 board. Support will be added to all on-board peripherals, sensors and I/O.
Dependencies: FRDM_MMA8451Q MAG3110 TSI mbed
Fork of FRDM-KL46-Template by
main.cpp
00001 #include "mbed.h" 00002 #include "TSISensor.h" 00003 #include "MMA8451Q.h" 00004 #include "MAG3110.h" 00005 00006 00007 // This project has been created to bring together the libraries required to support 00008 // the hardware and sensors found on the Freescale FRDM-KL46Z board. The following 00009 // libraries are included and exercised in this project: 00010 // 00011 // mbed (source: official mbed library) 00012 // Serial: 00013 // Serial console routed through the mbed interface 00014 // PTA2 / UART0_TX = TX Signal - also on Arduino pin D1 00015 // PTA1 / UART0_RX = RX Signal - also on Arduino pin D0 00016 // 00017 // DigitalOut: 00018 // GPIO to drive onboard LEDs 00019 // PTD5 / GPIO = LED1 - drive low to turn on LED - also on Arduino pin D13 00020 // PTE29 / GPIO = LED2 - drive low to turn on LED 00021 // 00022 // DigitalIn: 00023 // GPIO to monitor the two onboard push buttons 00024 // PTC3 / GPIO = SW1 - low input = button pressed 00025 // PTC12 / GPIO = SW3 - low input = button pressed 00026 // 00027 // AnalogIn: 00028 // ADC channel to monitor ambient light sensor 00029 // PTE22 / ADC = Light Sensor - higher value = darker 00030 // 00031 // TSI (source: http://mbed.org/users/emilmont/code/TSI/ ) 00032 // Capacitive Touch library to support the onboard Touch-Slider 00033 // 00034 // FRDM_MMA8451Q (source: http://mbed.org/users/clemente/code/FRDM_MMA8451Q/ ) 00035 // Freescale MMA8451 Accelerometer connected on I2C0 00036 // PTE24 / I2C0_SCL = I2C bus for communication (shared with MAG3110) 00037 // PTE25 / I2C0_SDA = I2C bus for communication (shared with MAG3110) 00038 // PTC5 / INT1_ACCEL = INT1 output of MMA8451Q 00039 // PTD1 / INT2_ACCEL = INT2 output of MMA8451Q (shared with MAG3110) 00040 // 00041 // MAG3110 (source: http://mbed.org/users/mmaas/code/MAG3110/) 00042 // (based on: http://mbed.org/users/SomeRandomBloke/code/MAG3110/) 00043 // Freescale MAG3110 Magnetomoter connected on I2C0 00044 // PTE24 / I2C0_SCL = I2C bus for communication (shared with MMA8451) 00045 // PTE25 / I2C0_SDA = I2C bus for communication (shared with MMA8451) 00046 // PTD1 / INT1_MAG / INT2_ACCEL = INT1 output of MAG3110 (shared with MMA8451) 00047 // 00048 00049 00050 00051 ////////////////////////////////////////////////////////////////////// 00052 // Include support for USB Serial console 00053 Serial pc(USBTX, USBRX); 00054 00055 00056 ////////////////////////////////////////////////////////////////////// 00057 // Include support for on-board green and red LEDs 00058 #define LED_ON 0 00059 #define LED_OFF 1 00060 DigitalOut greenLED(LED_GREEN); 00061 DigitalOut redLED(LED_RED); 00062 00063 00064 ////////////////////////////////////////////////////////////////////// 00065 // Include support for onboard pushbuttons (value = 0 when pressed) 00066 DigitalIn sw1(PTC3); 00067 DigitalIn sw3(PTC12); 00068 00069 00070 ////////////////////////////////////////////////////////////////////// 00071 // Include support for onboard Capacitive Touch Slider 00072 TSISensor slider; 00073 00074 00075 ////////////////////////////////////////////////////////////////////// 00076 // Include support for analog inputs 00077 AnalogIn lightSense(PTE22); 00078 00079 00080 ///////////////////////////////////////////////////////////////////// 00081 // Include support for MMA8451Q Acceleromoter 00082 #define MMA8451_I2C_ADDRESS (0x1d<<1) 00083 MMA8451Q acc(PTE25, PTE24, MMA8451_I2C_ADDRESS); 00084 00085 00086 ///////////////////////////////////////////////////////////////////// 00087 // Include support for MAG3110 Magnetometer 00088 MAG3110 mag(PTE25, PTE24); 00089 00090 00091 ///////////////////////////////////////////////////////////////////// 00092 // Include a 1 second ticker as a heartbeat 00093 Ticker heartBeat; 00094 00095 00096 00097 ///////////////////////////////////////////////////////////////////// 00098 // Structure to hold FRDM-KL46Z sensor and input data 00099 struct KL46_SENSOR_DATA { 00100 int sw1State; 00101 int sw3State; 00102 00103 float sliderPosition; 00104 00105 float lightSensor; 00106 00107 int magXVal; 00108 int magYVal; 00109 float magHeading; 00110 00111 float accXVal; 00112 float accYVal; 00113 float accZVal; 00114 } sensorData; 00115 00116 00117 ///////////////////////////////////////////////////////////////////// 00118 // Prototype for routine to send all sensor data to serial port 00119 void serialSendSensorData(void); 00120 00121 00122 ///////////////////////////////////////////////////////////////////// 00123 // Prototype for LED flash routine 00124 void ledFlashTick(void); 00125 00126 00127 ///////////////////////////////////////////////////////////////////// 00128 // main application 00129 int main() 00130 { 00131 // Ensure LEDs are off 00132 greenLED = LED_OFF; 00133 redLED = LED_OFF; 00134 00135 // Set up heartBeat Ticker to flash an LED 00136 heartBeat.attach(&ledFlashTick, 1.0); 00137 00138 00139 // Set Serial Port data rate and say Hello 00140 pc.baud( 115200 ); 00141 pc.printf("Hello World\r\n"); 00142 00143 // Turn on pull up resistors on pushbutton inputs 00144 sw1.mode(PullUp); 00145 sw3.mode(PullUp); 00146 00147 // Calibrate Magnetometer 00148 printf("Press and release SW1, rotate the board 360 degrees.\r\n"); 00149 printf("Then press and release SW1 to complete the calibration process.\r\n"); 00150 00151 mag.calXY(PTC3, 0); 00152 00153 printf("Calibration complete.\r\n"); 00154 00155 00156 // Loop forever - read and update sensor data and print to console. 00157 while(1) 00158 { 00159 sensorData.sw1State = sw1; 00160 sensorData.sw3State = sw3; 00161 00162 sensorData.sliderPosition = slider.readPercentage() * 100; 00163 00164 sensorData.lightSensor = lightSense; 00165 00166 sensorData.accXVal = acc.getAccX(); 00167 sensorData.accYVal = acc.getAccY(); 00168 sensorData.accZVal = acc.getAccZ(); 00169 00170 sensorData.magXVal = mag.readVal(MAG_OUT_X_MSB); 00171 sensorData.magYVal = mag.readVal(MAG_OUT_Y_MSB); 00172 sensorData.magHeading = mag.getHeading(); 00173 00174 serialSendSensorData(); 00175 00176 // Blink red LED (loop running) 00177 redLED = LED_ON; 00178 wait(.03); 00179 redLED = LED_OFF; 00180 00181 wait(1); 00182 } 00183 } 00184 00185 00186 void serialSendSensorData(void) 00187 { 00188 printf("Switches:\r\n SW1 = %d\r\n SW3 = %d\r\n\r\n", sensorData.sw1State, sensorData.sw3State); 00189 printf("Slider:\r\n %2.0f %% \r\n\r\n", sensorData.sliderPosition); 00190 printf("Light Sensor:\r\n %1.3f \r\n\r\n", sensorData.lightSensor); 00191 printf("Accelerometer:\r\n X = %1.3f\r\n Y = %1.3f\r\n Z = %1.3f\r\n\r\n", sensorData.accXVal, sensorData.accYVal, sensorData.accZVal); 00192 printf("Magnetometer:\r\n X = %d\r\n Y = %d\r\n Heading = %.2f \r\n\r\n", sensorData.magXVal, sensorData.magYVal, sensorData.magHeading); 00193 00194 printf("\r\n"); 00195 00196 } 00197 00198 00199 void ledFlashTick(void) 00200 { 00201 greenLED = !greenLED; 00202 } 00203
Generated on Thu Jul 14 2022 05:03:44 by
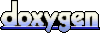