Patched version of nrf51822 FOTA compatible driver, with GPTIO disabled, as it clashed with the mbed definitions...
Fork of nRF51822 by
nrf_ecb.c
00001 /* Copyright (c) 2012 Nordic Semiconductor. All Rights Reserved. 00002 * 00003 * The information contained herein is property of Nordic Semiconductor ASA. 00004 * Terms and conditions of usage are described in detail in NORDIC 00005 * SEMICONDUCTOR STANDARD SOFTWARE LICENSE AGREEMENT. 00006 * 00007 * Licensees are granted free, non-transferable use of the information. NO 00008 * WARRANTY of ANY KIND is provided. This heading must NOT be removed from 00009 * the file. 00010 * 00011 * $LastChangedRevision: 25419 $ 00012 */ 00013 00014 /** 00015 * @file 00016 * @brief Implementation of AES ECB driver 00017 */ 00018 00019 00020 //lint -e438 00021 00022 #include <stdlib.h> 00023 #include <stdbool.h> 00024 #include <string.h> 00025 #include "nrf.h" 00026 #include "nrf_ecb.h" 00027 00028 static uint8_t ecb_data[48]; ///< ECB data structure for RNG peripheral to access. 00029 static uint8_t* ecb_key; ///< Key: Starts at ecb_data 00030 static uint8_t* ecb_cleartext; ///< Cleartext: Starts at ecb_data + 16 bytes. 00031 static uint8_t* ecb_ciphertext; ///< Ciphertext: Starts at ecb_data + 32 bytes. 00032 00033 bool nrf_ecb_init(void) 00034 { 00035 ecb_key = ecb_data; 00036 ecb_cleartext = ecb_data + 16; 00037 ecb_ciphertext = ecb_data + 32; 00038 00039 NRF_ECB->ECBDATAPTR = (uint32_t)ecb_data; 00040 return true; 00041 } 00042 00043 00044 bool nrf_ecb_crypt(uint8_t * dest_buf, const uint8_t * src_buf) 00045 { 00046 uint32_t counter = 0x1000000; 00047 if(src_buf != ecb_cleartext) 00048 { 00049 memcpy(ecb_cleartext,src_buf,16); 00050 } 00051 NRF_ECB->EVENTS_ENDECB = 0; 00052 NRF_ECB->TASKS_STARTECB = 1; 00053 while(NRF_ECB->EVENTS_ENDECB == 0) 00054 { 00055 counter--; 00056 if(counter == 0) 00057 { 00058 return false; 00059 } 00060 } 00061 NRF_ECB->EVENTS_ENDECB = 0; 00062 if(dest_buf != ecb_ciphertext) 00063 { 00064 memcpy(dest_buf,ecb_ciphertext,16); 00065 } 00066 return true; 00067 } 00068 00069 void nrf_ecb_set_key(const uint8_t * key) 00070 { 00071 memcpy(ecb_key,key,16); 00072 } 00073 00074
Generated on Tue Jul 12 2022 17:56:13 by
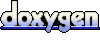