Patched version of nrf51822 FOTA compatible driver, with GPTIO disabled, as it clashed with the mbed definitions...
Fork of nRF51822 by
dfu.h
00001 /* Copyright (c) 2013 Nordic Semiconductor. All Rights Reserved. 00002 * 00003 * The information contained herein is property of Nordic Semiconductor ASA. 00004 * Terms and conditions of usage are described in detail in NORDIC 00005 * SEMICONDUCTOR STANDARD SOFTWARE LICENSE AGREEMENT. 00006 * 00007 * Licensees are granted free, non-transferable use of the information. NO 00008 * WARRANTY of ANY KIND is provided. This heading must NOT be removed from 00009 * the file. 00010 * 00011 */ 00012 00013 /**@file 00014 * 00015 * @defgroup nrf_dfu Device Firmware Update API. 00016 * @{ 00017 * 00018 * @brief Device Firmware Update module interface. 00019 */ 00020 00021 #ifndef DFU_H__ 00022 #define DFU_H__ 00023 00024 #include "dfu_types.h " 00025 #include <stdbool.h> 00026 #include <stdint.h> 00027 00028 00029 /**@brief DFU event callback for asynchronous calls. 00030 * 00031 * @param[in] packet Packet type for which this callback is related. START_PACKET, DATA_PACKET. 00032 * @param[in] result Operation result code. NRF_SUCCESS when a queued operation was successful. 00033 * @param[in] p_data Pointer to the data to which the operation is related. 00034 */ 00035 typedef void (*dfu_callback_t)(uint32_t packet, uint32_t result, uint8_t * p_data); 00036 00037 /**@brief Function for initializing the Device Firmware Update module. 00038 * 00039 * @return NRF_SUCCESS on success, an error_code otherwise. 00040 */ 00041 uint32_t dfu_init(void); 00042 00043 /**@brief Function for registering a callback listener for \ref dfu_data_pkt_handle callbacks. 00044 * 00045 * @param[in] callback_handler Callback handler for receiving DFU events on completed operations 00046 * of DFU packets. 00047 */ 00048 void dfu_register_callback(dfu_callback_t callback_handler); 00049 00050 /**@brief Function for setting the DFU image size. 00051 * 00052 * @details Function sets the DFU image size. This function must be called when an update is started 00053 * in order to notify the DFU of the new image size. If multiple images are to be 00054 * transferred within the same update context then this function must be called with size 00055 * information for each image being transfered. 00056 * If an image type is not being transfered, e.g. SoftDevice but no Application , then the 00057 * image size for application must be zero. 00058 * 00059 * @param[in] p_packet Pointer to the DFU packet containing information on DFU update process to 00060 * be started. 00061 * 00062 * @return NRF_SUCCESS on success, an error_code otherwise. 00063 */ 00064 uint32_t dfu_start_pkt_handle(dfu_update_packet_t * p_packet); 00065 00066 /**@brief Function for handling DFU data packets. 00067 * 00068 * @param[in] p_packet Pointer to the DFU packet. 00069 * 00070 * @return NRF_SUCCESS on success, an error_code otherwise. 00071 */ 00072 uint32_t dfu_data_pkt_handle(dfu_update_packet_t * p_packet); 00073 00074 /**@brief Function for handling DFU init packets. 00075 * 00076 * @return NRF_SUCCESS on success, an error_code otherwise. 00077 */ 00078 uint32_t dfu_init_pkt_handle(dfu_update_packet_t * p_packet); 00079 00080 /**@brief Function for validating a transferred image after the transfer has completed. 00081 * 00082 * @return NRF_SUCCESS on success, an error_code otherwise. 00083 */ 00084 uint32_t dfu_image_validate(void); 00085 00086 /**@brief Function for activating the transfered image after validation has successfully completed. 00087 * 00088 * @return NRF_SUCCESS on success, an error_code otherwise. 00089 */ 00090 uint32_t dfu_image_activate(void); 00091 00092 /**@brief Function for reseting the current update procedure and return to initial state. 00093 * 00094 * @details This function call will result in a system reset to ensure correct system behavior. 00095 * The reset will might be scheduled to execute at a later point in time to ensure pending 00096 * flash operations has completed. 00097 */ 00098 void dfu_reset(void); 00099 00100 /**@brief Function for validating that new bootloader has been correctly installed. 00101 * 00102 * @return NRF_SUCCESS if install was successful. NRF_ERROR_NULL if the images differs. 00103 */ 00104 uint32_t dfu_bl_image_validate(void); 00105 00106 /**@brief Function for validating that new SoftDevicehas been correctly installed. 00107 * 00108 * @return NRF_SUCCESS if install was successful. NRF_ERROR_NULL if the images differs. 00109 */ 00110 uint32_t dfu_sd_image_validate(void); 00111 00112 /**@brief Function for swapping existing bootloader with newly received. 00113 * 00114 * @return NRF_SUCCESS on succesfull swapping. For error code please refer to 00115 * \ref sd_mbr_command_copy_bl_t. 00116 */ 00117 uint32_t dfu_bl_image_swap(void); 00118 00119 /**@brief Function for swapping existing SoftDevice with newly received. 00120 * 00121 * @return NRF_SUCCESS on succesfull swapping. For error code please refer to 00122 * \ref sd_mbr_command_copy_sd_t. 00123 */ 00124 uint32_t dfu_sd_image_swap(void); 00125 00126 /**@brief Function for handling DFU init packet complete. 00127 * 00128 * @return NRF_SUCCESS on success, an error_code otherwise. 00129 */ 00130 uint32_t dfu_init_pkt_complete(void); 00131 00132 #endif // DFU_H__ 00133 00134 /** @} */
Generated on Tue Jul 12 2022 17:56:12 by
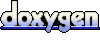