Patched version of nrf51822 FOTA compatible driver, with GPTIO disabled, as it clashed with the mbed definitions...
Fork of nRF51822 by
bootloader.h
00001 /* Copyright (c) 2013 Nordic Semiconductor. All Rights Reserved. 00002 * 00003 * The information contained herein is property of Nordic Semiconductor ASA. 00004 * Terms and conditions of usage are described in detail in NORDIC 00005 * SEMICONDUCTOR STANDARD SOFTWARE LICENSE AGREEMENT. 00006 * 00007 * Licensees are granted free, non-transferable use of the information. NO 00008 * WARRANTY of ANY KIND is provided. This heading must NOT be removed from 00009 * the file. 00010 * 00011 */ 00012 00013 /**@file 00014 * 00015 * @defgroup nrf_bootloader Bootloader API. 00016 * @{ 00017 * 00018 * @brief Bootloader module interface. 00019 */ 00020 00021 #ifndef BOOTLOADER_H__ 00022 #define BOOTLOADER_H__ 00023 00024 #include <stdbool.h> 00025 #include <stdint.h> 00026 #include "bootloader_types.h " 00027 #include "dfu_types.h " 00028 00029 /**@brief Function for initializing the Bootloader. 00030 * 00031 * @retval NRF_SUCCESS If bootloader was succesfully initialized. 00032 */ 00033 uint32_t bootloader_init(void); 00034 00035 /**@brief Function for validating application region in flash. 00036 * 00037 * @param[in] app_addr Address to the region in flash where the application is stored. 00038 * 00039 * @retval true If Application region is valid. 00040 * @retval false If Application region is not valid. 00041 */ 00042 bool bootloader_app_is_valid(uint32_t app_addr); 00043 00044 /**@brief Function for starting the Device Firmware Update. 00045 * 00046 * @retval NRF_SUCCESS If new application image was successfully transferred. 00047 */ 00048 uint32_t bootloader_dfu_start(void); 00049 00050 /**@brief Function for exiting bootloader and booting into application. 00051 * 00052 * @details This function will disable SoftDevice and all interrupts before jumping to application. 00053 * The SoftDevice vector table base for interrupt forwarding will be set the application 00054 * address. 00055 * 00056 * @param[in] app_addr Address to the region where the application is stored. 00057 */ 00058 void bootloader_app_start(uint32_t app_addr); 00059 00060 /**@brief Function for retrieving the bootloader settings. 00061 * 00062 * @param[out] p_settings A copy of the current bootloader settings is returned in the structure 00063 * provided. 00064 */ 00065 void bootloader_settings_get(bootloader_settings_t * const p_settings); 00066 00067 /**@brief Function for processing DFU status update. 00068 * 00069 * @param[in] update_status DFU update status. 00070 */ 00071 void bootloader_dfu_update_process(dfu_update_status_t update_status); 00072 00073 /**@brief Function getting state of SoftDevice update in progress. 00074 * After a successfull SoftDevice transfer the system restarts in orderto disable SoftDevice 00075 * and complete the update. 00076 * 00077 * @retval true A SoftDevice update is in progress. This indicates that second stage 00078 * of a SoftDevice update procedure can be initiated. 00079 * @retval false No SoftDevice update is in progress. 00080 */ 00081 bool bootloader_dfu_sd_in_progress(void); 00082 00083 /**@brief Function for continuing the Device Firmware Update of a SoftDevice. 00084 * 00085 * @retval NRF_SUCCESS If the final stage of SoftDevice update was successful. 00086 */ 00087 uint32_t bootloader_dfu_sd_update_continue(void); 00088 00089 /**@brief Function for finalizing the Device Firmware Update of a SoftDevice. 00090 * 00091 * @retval NRF_SUCCESS If the final stage of SoftDevice update was successful. 00092 */ 00093 uint32_t bootloader_dfu_sd_update_finalize(void); 00094 00095 #endif // BOOTLOADER_H__ 00096 00097 /**@} */
Generated on Tue Jul 12 2022 17:56:12 by
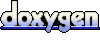