Minor temporary patch to allow DFU packet callback
Fork of BLE_API by
GapAdvertisingData.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef __GAP_ADVERTISING_DATA_H__ 00018 #define __GAP_ADVERTISING_DATA_H__ 00019 00020 #include <string.h> 00021 00022 #include "blecommon.h" 00023 00024 #define GAP_ADVERTISING_DATA_MAX_PAYLOAD (31) 00025 00026 /**************************************************************************/ 00027 /*! 00028 \brief 00029 This class provides several helper functions to generate properly 00030 formatted GAP Advertising and Scan Response data payloads 00031 00032 \note 00033 See Bluetooth Specification 4.0 (Vol. 3), Part C, Section 11 and 18 00034 for further information on Advertising and Scan Response data. 00035 00036 \par Advertising and Scan Response Payloads 00037 Advertising data and Scan Response data are organized around a set of 00038 data types called 'AD types' in Bluetooth 4.0 (see the Bluetooth Core 00039 Specification v4.0, Vol. 3, Part C, Sections 11 and 18). 00040 00041 \par 00042 Each AD type has it's own standardized 'assigned number', as defined 00043 by the Bluetooth SIG: 00044 https://www.bluetooth.org/en-us/specification/assigned-numbers/generic-access-profile 00045 00046 \par 00047 For convenience sake, all appropriate AD types have been encapsulated 00048 into GapAdvertisingData::DataType. 00049 00050 \par 00051 Before the AD Types and their payload (if any) can be inserted into 00052 the Advertising or Scan Response frames, they need to be formatted as 00053 follows: 00054 00055 \li \c Record length (1 byte) 00056 \li \c AD Type (1 byte) 00057 \li \c AD payload (optional, only present if record length > 1) 00058 00059 \par 00060 This class takes care of properly formatting the payload, performs 00061 some basic checks on the payload length, and tries to avoid common 00062 errors like adding an exclusive AD field twice in the Advertising 00063 or Scan Response payload. 00064 00065 \par EXAMPLE 00066 00067 \code 00068 00069 // ToDo 00070 00071 \endcode 00072 */ 00073 /**************************************************************************/ 00074 class GapAdvertisingData 00075 { 00076 public: 00077 /**********************************************************************/ 00078 /*! 00079 \brief 00080 A list of Advertising Data types commonly used by peripherals. 00081 These AD types are used to describe the capabilities of the 00082 peripheral, and get inserted inside the advertising or scan 00083 response payloads. 00084 00085 \par Source 00086 \li \c Bluetooth Core Specification 4.0 (Vol. 3), Part C, Section 11, 18 00087 \li \c https://www.bluetooth.org/en-us/specification/assigned-numbers/generic-access-profile 00088 */ 00089 /**********************************************************************/ 00090 enum DataType { 00091 FLAGS = 0x01, /**< \ref *Flags */ 00092 INCOMPLETE_LIST_16BIT_SERVICE_IDS = 0x02, /**< Incomplete list of 16-bit Service IDs */ 00093 COMPLETE_LIST_16BIT_SERVICE_IDS = 0x03, /**< Complete list of 16-bit Service IDs */ 00094 INCOMPLETE_LIST_32BIT_SERVICE_IDS = 0x04, /**< Incomplete list of 32-bit Service IDs (not relevant for Bluetooth 4.0) */ 00095 COMPLETE_LIST_32BIT_SERVICE_IDS = 0x05, /**< Complete list of 32-bit Service IDs (not relevant for Bluetooth 4.0) */ 00096 INCOMPLETE_LIST_128BIT_SERVICE_IDS = 0x06, /**< Incomplete list of 128-bit Service IDs */ 00097 COMPLETE_LIST_128BIT_SERVICE_IDS = 0x07, /**< Complete list of 128-bit Service IDs */ 00098 SHORTENED_LOCAL_NAME = 0x08, /**< Shortened Local Name */ 00099 COMPLETE_LOCAL_NAME = 0x09, /**< Complete Local Name */ 00100 TX_POWER_LEVEL = 0x0A, /**< TX Power Level (in dBm) */ 00101 DEVICE_ID = 0x10, /**< Device ID */ 00102 SLAVE_CONNECTION_INTERVAL_RANGE = 0x12, /**< Slave Connection Interval Range */ 00103 SERVICE_DATA = 0x16, /**< Service Data */ 00104 APPEARANCE = 0x19, /**< \ref Appearance */ 00105 ADVERTISING_INTERVAL = 0x1A, /**< Advertising Interval */ 00106 MANUFACTURER_SPECIFIC_DATA = 0xFF /**< Manufacturer Specific Data */ 00107 }; 00108 00109 /**********************************************************************/ 00110 /*! 00111 \brief 00112 A list of values for the FLAGS AD Type 00113 00114 \note 00115 You can use more than one value in the FLAGS AD Type (ex. 00116 LE_GENERAL_DISCOVERABLE and BREDR_NOT_SUPPORTED). 00117 00118 \par Source 00119 \li \c Bluetooth Core Specification 4.0 (Vol. 3), Part C, Section 18.1 00120 */ 00121 /**********************************************************************/ 00122 enum Flags { 00123 LE_LIMITED_DISCOVERABLE = 0x01, /**< *Peripheral device is discoverable for a limited period of time */ 00124 LE_GENERAL_DISCOVERABLE = 0x02, /**< Peripheral device is discoverable at any moment */ 00125 BREDR_NOT_SUPPORTED = 0x04, /**< Peripheral device is LE only */ 00126 SIMULTANEOUS_LE_BREDR_C = 0x08, /**< Not relevant - central mode only */ 00127 SIMULTANEOUS_LE_BREDR_H = 0x10 /**< Not relevant - central mode only */ 00128 }; 00129 00130 /**********************************************************************/ 00131 /*! 00132 \brief 00133 A list of values for the APPEARANCE AD Type, which describes the 00134 physical shape or appearance of the device 00135 00136 \par Source 00137 \li \c Bluetooth Core Specification Supplement, Part A, Section 1.12 00138 \li \c Bluetooth Core Specification 4.0 (Vol. 3), Part C, Section 12.2 00139 \li \c https://developer.bluetooth.org/gatt/characteristics/Pages/CharacteristicViewer.aspx?u=org.bluetooth.characteristic.gap.appearance.xml 00140 */ 00141 /**********************************************************************/ 00142 enum Appearance { 00143 UNKNOWN = 0, /**< Unknown of unspecified appearance type */ 00144 GENERIC_PHONE = 64, /**< Generic Phone */ 00145 GENERIC_COMPUTER = 128, /**< Generic Computer */ 00146 GENERIC_WATCH = 192, /**< Generic Watch */ 00147 WATCH_SPORTS_WATCH = 193, /**< Sports Watch */ 00148 GENERIC_CLOCK = 256, /**< Generic Clock */ 00149 GENERIC_DISPLAY = 320, /**< Generic Display */ 00150 GENERIC_REMOTE_CONTROL = 384, /**< Generic Remote Control */ 00151 GENERIC_EYE_GLASSES = 448, /**< Generic Eye Glasses */ 00152 GENERIC_TAG = 512, /**< Generic Tag */ 00153 GENERIC_KEYRING = 576, /**< Generic Keyring */ 00154 GENERIC_MEDIA_PLAYER = 640, /**< Generic Media Player */ 00155 GENERIC_BARCODE_SCANNER = 704, /**< Generic Barcode Scanner */ 00156 GENERIC_THERMOMETER = 768, /**< Generic Thermometer */ 00157 THERMOMETER_EAR = 769, /**< Ear Thermometer */ 00158 GENERIC_HEART_RATE_SENSOR = 832, /**< Generic Heart Rate Sensor */ 00159 HEART_RATE_SENSOR_HEART_RATE_BELT = 833, /**< Belt Heart Rate Sensor */ 00160 GENERIC_BLOOD_PRESSURE = 896, /**< Generic Blood Pressure */ 00161 BLOOD_PRESSURE_ARM = 897, /**< Arm Blood Pressure */ 00162 BLOOD_PRESSURE_WRIST = 898, /**< Wrist Blood Pressure */ 00163 HUMAN_INTERFACE_DEVICE_HID = 960, /**< Human Interface Device (HID) */ 00164 KEYBOARD = 961, /**< Keyboard */ 00165 MOUSE = 962, /**< Mouse */ 00166 JOYSTICK = 963, /**< Joystick */ 00167 GAMEPAD = 964, /**< Gamepad */ 00168 DIGITIZER_TABLET = 965, /**< Digitizer Tablet */ 00169 CARD_READER = 966, /**< Card Read */ 00170 DIGITAL_PEN = 967, /**< Digital Pen */ 00171 BARCODE_SCANNER = 968, /**< Barcode Scanner */ 00172 GENERIC_GLUCOSE_METER = 1024, /**< Generic Glucose Meter */ 00173 GENERIC_RUNNING_WALKING_SENSOR = 1088, /**< Generic Running/Walking Sensor */ 00174 RUNNING_WALKING_SENSOR_IN_SHOE = 1089, /**< In Shoe Running/Walking Sensor */ 00175 RUNNING_WALKING_SENSOR_ON_SHOE = 1090, /**< On Shoe Running/Walking Sensor */ 00176 RUNNING_WALKING_SENSOR_ON_HIP = 1091, /**< On Hip Running/Walking Sensor */ 00177 GENERIC_CYCLING = 1152, /**< Generic Cycling */ 00178 CYCLING_CYCLING_COMPUTER = 1153, /**< Cycling Computer */ 00179 CYCLING_SPEED_SENSOR = 1154, /**< Cycling Speed Senspr */ 00180 CYCLING_CADENCE_SENSOR = 1155, /**< Cycling Cadence Sensor */ 00181 CYCLING_POWER_SENSOR = 1156, /**< Cycling Power Sensor */ 00182 CYCLING_SPEED_AND_CADENCE_SENSOR = 1157, /**< Cycling Speed and Cadence Sensor */ 00183 PULSE_OXIMETER_GENERIC = 3136, /**< Generic Pulse Oximeter */ 00184 PULSE_OXIMETER_FINGERTIP = 3137, /**< Fingertip Pulse Oximeter */ 00185 PULSE_OXIMETER_WRIST_WORN = 3138, /**< Wrist Worn Pulse Oximeter */ 00186 OUTDOOR_GENERIC = 5184, /**< Generic Outdoor */ 00187 OUTDOOR_LOCATION_DISPLAY_DEVICE = 5185, /**< Outdoor Location Display Device */ 00188 OUTDOOR_LOCATION_AND_NAVIGATION_DISPLAY_DEVICE = 5186, /**< Outdoor Location and Navigation Display Device */ 00189 OUTDOOR_LOCATION_POD = 5187, /**< Outdoor Location Pod */ 00190 OUTDOOR_LOCATION_AND_NAVIGATION_POD = 5188 /**< Outdoor Location and Navigation Pod */ 00191 }; 00192 00193 GapAdvertisingData(void) : _payload(), _payloadLen(0), _appearance(GENERIC_TAG) { 00194 /* empty */ 00195 } 00196 00197 /** 00198 * Adds advertising data based on the specified AD type (see DataType) 00199 * 00200 * @param advDataType The Advertising 'DataType' to add 00201 * @param payload Pointer to the payload contents 00202 * @param len Size of the payload in bytes 00203 * 00204 * @return BLE_ERROR_BUFFER_OVERFLOW if the specified data would cause the 00205 * advertising buffer to overflow, else BLE_ERROR_NONE. 00206 */ 00207 ble_error_t addData(DataType advDataType, const uint8_t *payload, uint8_t len) 00208 { 00209 /* ToDo: Check if an AD type already exists and if the existing */ 00210 /* value is exclusive or not (flags, etc.) */ 00211 00212 /* Make sure we don't exceed the 31 byte payload limit */ 00213 if (_payloadLen + len + 2 > GAP_ADVERTISING_DATA_MAX_PAYLOAD) { 00214 return BLE_ERROR_BUFFER_OVERFLOW; 00215 } 00216 00217 /* Field length */ 00218 memset(&_payload[_payloadLen], len + 1, 1); 00219 _payloadLen++; 00220 00221 /* Field ID */ 00222 memset(&_payload[_payloadLen], (uint8_t)advDataType, 1); 00223 _payloadLen++; 00224 00225 /* Payload */ 00226 memcpy(&_payload[_payloadLen], payload, len); 00227 _payloadLen += len; 00228 00229 return BLE_ERROR_NONE; 00230 } 00231 00232 /** 00233 * Helper function to add APPEARANCE data to the advertising payload 00234 * 00235 * @param appearance 00236 * The APPEARANCE value to add 00237 * 00238 * @return BLE_ERROR_BUFFER_OVERFLOW if the specified data would cause the 00239 * advertising buffer to overflow, else BLE_ERROR_NONE. 00240 */ 00241 ble_error_t addAppearance(Appearance appearance = GENERIC_TAG) { 00242 _appearance = appearance; 00243 return addData(GapAdvertisingData::APPEARANCE, (uint8_t *)&appearance, 2); 00244 } 00245 00246 /** 00247 * Helper function to add FLAGS data to the advertising payload. 00248 * @param flags 00249 * LE_LIMITED_DISCOVERABLE 00250 * The peripheral is discoverable for a limited period of time. 00251 * LE_GENERAL_DISCOVERABLE 00252 * The peripheral is permanently discoverable. 00253 * BREDR_NOT_SUPPORTED 00254 * This peripheral is a Bluetooth Low Energy only device (no EDR support). 00255 * 00256 * @return BLE_ERROR_BUFFER_OVERFLOW if the specified data would cause the 00257 * advertising buffer to overflow, else BLE_ERROR_NONE. 00258 */ 00259 ble_error_t addFlags(uint8_t flags = LE_GENERAL_DISCOVERABLE) { 00260 return addData(GapAdvertisingData::FLAGS, &flags, 1); 00261 } 00262 00263 /** 00264 * Helper function to add TX_POWER_LEVEL data to the advertising payload 00265 * 00266 * @return BLE_ERROR_BUFFER_OVERFLOW if the specified data would cause the 00267 * advertising buffer to overflow, else BLE_ERROR_NONE. 00268 */ 00269 ble_error_t addTxPower(int8_t txPower) { 00270 /* ToDo: Basic error checking to make sure txPower is in range */ 00271 return addData(GapAdvertisingData::TX_POWER_LEVEL, (uint8_t *)&txPower, 1); 00272 } 00273 00274 /** 00275 * Clears the payload and resets the payload length counter 00276 */ 00277 void clear(void) { 00278 memset(&_payload, 0, GAP_ADVERTISING_DATA_MAX_PAYLOAD); 00279 _payloadLen = 0; 00280 } 00281 00282 /** 00283 * Returns a pointer to the the current payload 00284 */ 00285 const uint8_t *getPayload(void) const { 00286 return (_payloadLen > 0) ? _payload : NULL; 00287 } 00288 00289 /** 00290 * Returns the current payload length (0..31 bytes) 00291 */ 00292 uint8_t getPayloadLen(void) const { 00293 return _payloadLen; 00294 } 00295 00296 /** 00297 * Returns the 16-bit appearance value for this device 00298 */ 00299 uint16_t getAppearance(void) const { 00300 return (uint16_t)_appearance; 00301 } 00302 00303 private: 00304 uint8_t _payload[GAP_ADVERTISING_DATA_MAX_PAYLOAD]; 00305 uint8_t _payloadLen; 00306 uint16_t _appearance; 00307 }; 00308 00309 #endif // ifndef __GAP_ADVERTISING_DATA_H__
Generated on Wed Jul 13 2022 06:54:06 by
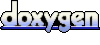