Minor temporary patch to allow DFU packet callback
Fork of BLE_API by
BLEDeviceInstanceBase.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef __BLE_DEVICE_INSTANCE_BASE__ 00018 #define __BLE_DEVICE_INSTANCE_BASE__ 00019 00020 /** 00021 * The interface for the transport object to be created by the target library's 00022 * createBLEDeviceInstance(). 00023 */ 00024 class BLEDeviceInstanceBase 00025 { 00026 public: 00027 virtual const char *getVersion(void) = 0; 00028 virtual Gap& getGap() = 0; 00029 virtual GattServer& getGattServer() = 0; 00030 virtual ble_error_t init(void) = 0; 00031 virtual ble_error_t shutdown(void) = 0; 00032 virtual ble_error_t reset(void) = 0; 00033 virtual ble_error_t initializeSecurity(bool enableBonding = true, 00034 bool requireMITM = true, 00035 Gap::SecurityIOCapabilities_t iocaps = Gap::IO_CAPS_NONE, 00036 const Gap::Passkey_t passkey = NULL) = 0; 00037 virtual ble_error_t setTxPower(int8_t txPower) = 0; 00038 virtual void getPermittedTxPowerValues(const int8_t **, size_t *) = 0; 00039 virtual void waitForEvent(void) = 0; 00040 }; 00041 00042 /** 00043 * BLEDevice uses composition to hide an interface object encapsulating the 00044 * backend transport. 00045 * 00046 * The following API is used to create the singleton interface object. An 00047 * implementation for this function must be provided by the device-specific 00048 * library, otherwise there will be a linker error. 00049 */ 00050 extern BLEDeviceInstanceBase *createBLEDeviceInstance(void); 00051 00052 #endif // ifndef __BLE_DEVICE_INSTANCE_BASE__
Generated on Wed Jul 13 2022 06:54:06 by
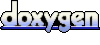