Board library for MAX32630FTHR platform
Dependencies: MAX14690
Dependents: FTHR_OLED MAX32630FTHR_ADC_Example Test_TFT_RT MAX32630FTHR_RGB ... more
Fork of max32630fthr by
max32630fthr.h
00001 /******************************************************************************* 00002 * Copyright (C) 2016 Maxim Integrated Products, Inc., All Rights Reserved. 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a 00005 * copy of this software and associated documentation files (the "Software"), 00006 * to deal in the Software without restriction, including without limitation 00007 * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 * and/or sell copies of the Software, and to permit persons to whom the 00009 * Software is furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included 00012 * in all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00015 * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00016 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00017 * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00018 * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00019 * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00020 * OTHER DEALINGS IN THE SOFTWARE. 00021 * 00022 * Except as contained in this notice, the name of Maxim Integrated 00023 * Products, Inc. shall not be used except as stated in the Maxim Integrated 00024 * Products, Inc. Branding Policy. 00025 * 00026 * The mere transfer of this software does not imply any licenses 00027 * of trade secrets, proprietary technology, copyrights, patents, 00028 * trademarks, maskwork rights, or any other form of intellectual 00029 * property whatsoever. Maxim Integrated Products, Inc. retains all 00030 * ownership rights. 00031 ******************************************************************************* 00032 */ 00033 00034 #ifndef _MAX32630FTHR_H_ 00035 #define _MAX32630FTHR_H_ 00036 00037 #include "mbed.h" 00038 #include "MAX14690.h" 00039 00040 /** 00041 * @brief MAX32630FTHR Board Support Library 00042 * 00043 * @details The MAX32630FTHR is a rapid development application board for 00044 * ultra low power wearable applications. It includes common peripherals and 00045 * expansion connectors all power optimized for getting the longest life from 00046 * the battery. This library configures the power and I/O for the board. 00047 * <br>https://www.maximintegrated.com/max32630fthr 00048 * 00049 * @code 00050 * #include "mbed.h" 00051 * #include "max32630fthr.h" 00052 * 00053 * DigitalOut led1(LED1); 00054 * MAX32630FTHR pegasus(MAX32630FTHR::VIO_3V3); 00055 * 00056 * // main() runs in its own thread in the OS 00057 * // (note the calls to Thread::wait below for delays) 00058 * int main() 00059 * { 00060 * // initialize power and I/O on MAX32630FTHR board 00061 * pegasus.init(); 00062 * 00063 * while (true) { 00064 * led1 = !led1; 00065 * Thread::wait(500); 00066 * } 00067 * } 00068 * @endcode 00069 */ 00070 class MAX32630FTHR 00071 { 00072 public: 00073 // max32630fthr configuration utilities 00074 00075 /** 00076 * @brief IO Voltage 00077 * @details Enumerated options for operating voltage 00078 */ 00079 typedef enum { 00080 VIO_1V8 = 0x00, ///< 1.8V IO voltage at headers (from BUCK2) 00081 VIO_3V3 = 0x01, ///< 3.3V IO voltage at headers (from LDO2) 00082 } vio_t; 00083 00084 /** 00085 * MAX32630FTHR constructor. 00086 * 00087 */ 00088 MAX32630FTHR(); 00089 00090 /** 00091 * MAX32630FTHR constructor. 00092 * 00093 */ 00094 MAX32630FTHR(vio_t vio); 00095 00096 /** 00097 * MAX32630FTHR destructor. 00098 */ 00099 ~MAX32630FTHR(); 00100 00101 /** 00102 * @brief Initialize MAX32630FTHR board 00103 * @details Initializes PMIC and I/O on MAX32630FTHR board. 00104 * Configures PMIC to enable LDO2 and LDO3 at 3.3V. 00105 * Disables resisitive pulldown on MON(AIN_0) 00106 * Sets default I/O voltages to 3V3 for micro SD card. 00107 * Sets I/O voltage for header pins to hdrVio specified. 00108 * @param hdrVio I/O voltage for header pins 00109 * @returns 0 if no errors, -1 if error. 00110 */ 00111 int init(vio_t hdrVio); 00112 00113 /** 00114 * @brief Sets I/O Voltage 00115 * @details Sets the voltage rail to be used for a given pin. 00116 * VIO_1V8 selects VDDIO which is supplied by Buck2, which is set at 1.8V, 00117 * VIO_3V3 selects VDDIOH which is supplied by LDO2, which is typically 3.3V/ 00118 * @param pin Pin whose voltage supply is being assigned. 00119 * @param vio Voltage rail to be used for specified pin. 00120 * @returns 0 if no errors, -1 if error. 00121 */ 00122 int vddioh(PinName pin, vio_t vio); 00123 00124 /// Local I2C bus for configuring PMIC and accessing BMI160 IMU. 00125 I2C i2c; 00126 00127 /// MAX14690 PMIC Instance 00128 MAX14690 max14690; 00129 00130 }; 00131 00132 #endif /* _MAX32630FTHR_H_ */
Generated on Thu Jul 14 2022 04:57:55 by
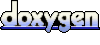