RTC library for Max31341/2 devices
Fork of max3134x by
Embed:
(wiki syntax)
Show/hide line numbers
RtcBase.h
00001 /******************************************************************************* 00002 * Copyright (C) 2018 Maxim Integrated Products, Inc., All Rights Reserved. 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a 00005 * copy of this software and associated documentation files (the "Software"), 00006 * to deal in the Software without restriction, including without limitation 00007 * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 * and/or sell copies of the Software, and to permit persons to whom the 00009 * Software is furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included 00012 * in all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00015 * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00016 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00017 * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00018 * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00019 * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00020 * OTHER DEALINGS IN THE SOFTWARE. 00021 * 00022 * Except as contained in this notice, the name of Maxim Integrated 00023 * Products, Inc. shall not be used except as stated in the Maxim Integrated 00024 * Products, Inc. Branding Policy. 00025 * 00026 * The mere transfer of this software does not imply any licenses 00027 * of trade secrets, proprietary technology, copyrights, patents, 00028 * trademarks, maskwork rights, or any other form of intellectual 00029 * property whatsoever. Maxim Integrated Products, Inc. retains all 00030 * ownership rights. 00031 ******************************************************************************* 00032 */ 00033 00034 #ifndef RTCBASE_H_ 00035 #define RTCBASE_H_ 00036 00037 #include "mbed.h" 00038 #include <ctime> 00039 00040 00041 /** 00042 * @brief RTC base driver class for Maxim Max3134x RTC series. 00043 */ 00044 class RtcBase 00045 { 00046 public: 00047 /** 00048 * @brief Mode of the comparator 00049 */ 00050 typedef enum { 00051 POW_MGMT_MODE_COMPARATOR, /**< Comparator */ 00052 POW_MGMT_MODE_POWER_MANAGEMENT, /**< Power Management / Trickle Charger Mode */ 00053 } power_mgmt_mode_t; 00054 00055 /** 00056 * @brief Analog comparator threshold voltage 00057 */ 00058 typedef enum { 00059 COMP_THRESH_1V4, /**< 1.4V */ 00060 COMP_THRESH_1V6, /**< 1.6V */ 00061 COMP_THRESH_1V8, /**< 1.8V */ 00062 COMP_THRESH_2V0, /**< 2.0V */ 00063 } comp_thresh_t; 00064 00065 /** 00066 * @brief Supply voltage select. 00067 */ 00068 typedef enum { 00069 POW_MGMT_SUPPLY_SEL_AUTO, /**< Circuit decides whether to use VCC or VBACKUP */ 00070 POW_MGMT_SUPPLY_SEL_VCC, /**< Use VCC as supply */ 00071 POW_MGMT_SUPPLY_SEL_AIN, /**< Use AIN as supply */ 00072 } power_mgmt_supply_t; 00073 00074 /** 00075 * @brief Selection of charging path's resistor value 00076 */ 00077 typedef enum { 00078 TRICKLE_CHARGER_3K5, /**< 3500 Ohm */ 00079 TRICKLE_CHARGER_3K5_2, /**< 3500 Ohm */ 00080 TRICKLE_CHARGER_7K, /**< 7000 Ohm */ 00081 TRICKLE_CHARGER_13K, /**< 13000 Ohm */ 00082 } trickle_charger_ohm_t; 00083 00084 /** 00085 * @brief Timer frequency selection 00086 */ 00087 typedef enum { 00088 TIMER_FREQ_1024HZ, /**< 1024Hz */ 00089 TIMER_FREQ_256HZ, /**< 256Hz */ 00090 TIMER_FREQ_64HZ, /**< 64Hz */ 00091 TIMER_FREQ_16HZ, /**< 16Hz */ 00092 } timer_freq_t; 00093 00094 /** 00095 * @brief CLKIN frequency selection 00096 */ 00097 typedef enum { 00098 CLKIN_FREQ_1HZ, /**< 1Hz*/ 00099 CLKIN_FREQ_50HZ, /**< 50Hz */ 00100 CLKIN_FREQ_60HZ, /**< 60Hz */ 00101 CLKIN_FREQ_32HZ768, /**< 32.768Hz */ 00102 } clkin_freq_t; 00103 00104 /** 00105 * @brief Square wave output frequency selection on CLKOUT pin 00106 */ 00107 typedef enum { 00108 SQUARE_WAVE_OUT_FREQ_1HZ, /**< 1Hz */ 00109 SQUARE_WAVE_OUT_FREQ_4096HZ, /**< 4.098kHz */ 00110 SQUARE_WAVE_OUT_FREQ_8192HZ, /**< 8.192kHz */ 00111 SQUARE_WAVE_OUT_FREQ_32768HZ, /**< 32.768kHz */ 00112 } square_wave_out_freq_t; 00113 00114 /** 00115 * @brief Selection of interrupt ids 00116 */ 00117 typedef enum { 00118 INTR_ID_ALARM1, /**< Alarm1 flag */ 00119 INTR_ID_ALARM2, /**< Alarm2 flag */ 00120 INTR_ID_TIMER, /**< Timer interrupt flag */ 00121 INTR_ID_RESERVED, 00122 INTR_ID_EXTERNAL, /**< External interrupt flag for DIN1 */ 00123 INTR_ID_ANALOG, /**< Analog Interrupt flag / Power fail flag */ 00124 INTR_ID_OSF, /**< Oscillator stop flag */ 00125 INTR_ID_LOS, /**< Loss of signal */ 00126 INTR_ID_END, 00127 } intr_id_t; 00128 00129 00130 /** 00131 * @brief Alarm number selection 00132 */ 00133 typedef enum { 00134 ALARM1, /**< Alarm number 1 */ 00135 ALARM2, /**< Alarm number 2 */ 00136 } alarm_no_t; 00137 00138 /** 00139 * @brief Alarm periodicity selection 00140 */ 00141 typedef enum { 00142 ALARM_PERIOD_EVERYSECOND, /**< Once per second */ 00143 ALARM_PERIOD_EVERYMINUTE, /**< Second match / Once per minute */ 00144 ALARM_PERIOD_HOURLY, /**< Second and Minute match */ 00145 ALARM_PERIOD_DAILY, /**< Hour, Minute and Second match*/ 00146 ALARM_PERIOD_WEEKLY, /**< Day and Time match */ 00147 ALARM_PERIOD_MONTHLY, /**< Date and Time match */ 00148 ALARM_PERIOD_YEARLY, /**< Month, Date and Time match (Max31342 only) */ 00149 ALARM_PERIOD_ONETIME, /**< Year, Month, Date and Time match (Max31342 only) */ 00150 } alarm_period_t; 00151 00152 /** 00153 * @brief Selection of INTA/CLKIN pin function 00154 */ 00155 typedef enum { 00156 CONFIGURE_PIN_AS_INTA, /**< Configure pin as interrupt out */ 00157 CONFIGURE_PIN_AS_CLKIN, /**< Configure pin as external clock in */ 00158 } config_inta_clkin_pin_t; 00159 00160 /** 00161 * @brief Selection of INTB/CLKOUT pin function 00162 */ 00163 typedef enum { 00164 CONFIGURE_PIN_AS_CLKOUT, /**< Output is square wave */ 00165 CONFIGURE_PIN_AS_INTB, /**< Output is interrupt */ 00166 } config_intb_clkout_pin_t; 00167 00168 /** 00169 * @brief Selection of sync delay 00170 */ 00171 typedef enum { 00172 SYNC_DLY_LESS_THAN_1SEC = 0, /**< Sync delay less than 1 second, recommended for external 1Hz clock */ 00173 SYNC_DLY_LESS_THAN_100MS, /**< Sync delay less than 100 msec, recommended for external 50Hz/60Hz/32KHz clock */ 00174 SYNC_DLY_LESS_THAN_20MS, /**< Sync delay less than 20 msec, recommended for internal clock */ 00175 } sync_delay_t; 00176 00177 typedef enum { 00178 TTS_INTERNAL_1SEC = 0, 00179 TTS_INTERNAL_2SEC, 00180 TTS_INTERNAL_4SEC, 00181 TTS_INTERNAL_16SEC, 00182 TTS_INTERNAL_32SEC, 00183 TTS_INTERNAL_64SEC, 00184 TTS_INTERNAL_128SEC, 00185 } ttsint_t; 00186 00187 /** 00188 * @brief Function pointer type to interrupt handler function 00189 */ 00190 typedef void (*interrupt_handler_function)(void *); 00191 00192 /** 00193 * @brief Read from a register. 00194 * 00195 * @param[in] reg Address of a register to be read. 00196 * @param[out] value Pointer to save result value. 00197 * @param[in] len Size of result to be read. 00198 * 00199 * @returns 0 on success, negative error code on failure. 00200 */ 00201 int read_register(uint8_t reg, uint8_t *value, uint8_t len); 00202 00203 /** 00204 * @brief Write to a register. 00205 * 00206 * @param[in] reg Address of a register to be written. 00207 * @param[out] value Pointer of value to be written to register. 00208 * @param[in] len Size of result to be written. 00209 * 00210 * @returns 0 on success, negative error code on failure. 00211 */ 00212 int write_register(uint8_t reg, const uint8_t *value, uint8_t len); 00213 00214 /** 00215 * @brief Read time info from RTC. 00216 * 00217 * @param[out] rtc_time Time info from RTC. 00218 * 00219 * @returns 0 on success, negative error code on failure. 00220 */ 00221 int get_time(struct tm *rtc_ctime); 00222 00223 /** 00224 * @brief Set time info to RTC. 00225 * 00226 * @param[in] rtc_time Time info to be written to RTC. 00227 * 00228 * @returns 0 on success, negative error code on failure. 00229 */ 00230 int set_time(const struct tm *rtc_ctime); 00231 00232 /** 00233 * @brief Non-volatile memory write 00234 * 00235 * @param[out] buffer Pointer to the data to be written 00236 * @param[in] offset Offset of location in NVRAM 00237 * @param[in] length Number of bytes to write 00238 * 00239 * @return 0 on success, error code on failure 00240 */ 00241 int nvram_write(const uint8_t *buffer, int offset, int length); 00242 00243 /** 00244 * @brief Non-volatile memory read 00245 * 00246 * @param[in] buffer Buffer to read in to 00247 * @param[in] offset Offset of location in NVRAM 00248 * @param[in] length Number of bytes to read 00249 * 00250 * @return 0 on success, error code on failure 00251 */ 00252 int nvram_read(uint8_t *buffer, int offset, int length); 00253 00254 /** 00255 * @brief NVRAM size of the part 00256 * 00257 * @return 0 if part does not have a NVRAM, otherwise returns size 00258 */ 00259 int nvram_size(); 00260 00261 /** 00262 * @brief Set an alarm condition 00263 * 00264 * @param[in] alarm_no Alarm number, ALARM1 or ALARM2 00265 * @param[in] alarm_time Pointer to alarm time to be set 00266 * @param[in] period Alarm periodicity, one of ALARM_PERIOD_* 00267 * 00268 * @return 0 on success, error code on failure 00269 */ 00270 int set_alarm(alarm_no_t alarm_no, const struct tm *alarm_time, alarm_period_t period); 00271 00272 /** 00273 * @brief Get alarm data & time 00274 * 00275 * @param[in] alarm_no Alarm number, ALARM1 or ALARM2 00276 * @param[out] alarm_time Pointer to alarm time to be filled in 00277 * @param[out] period Pointer to the period of alarm, one of ALARM_PERIOD_* 00278 * @param[out] is_enabled Pointer to the state of alarm 00279 * 00280 * @return 0 on success, error code on failure 00281 */ 00282 int get_alarm(alarm_no_t alarm_no, struct tm *alarm_time, alarm_period_t *period, bool *is_enabled); 00283 00284 /** 00285 * @brief Select power management mode of operation 00286 * 00287 * @param[in] mode Mode selection, one of COMP_MODE_* 00288 * 00289 * @return 0 on success, error code on failure 00290 */ 00291 int set_power_mgmt_mode(power_mgmt_mode_t mode); 00292 00293 /** 00294 * @brief Set comparator threshold 00295 * 00296 * @param[in] th Set Analog Comparator Threshold level, one of COMP_THRESH_* 00297 * 00298 * @return 0 on success, error code on failure 00299 */ 00300 int comparator_threshold_level(comp_thresh_t th); 00301 00302 /** 00303 * @brief Select device power source 00304 * 00305 * @param[in] supply Supply selection, one of POW_MGMT_SUPPLY_SEL_* 00306 * 00307 * @return 0 on success, error code on failure 00308 */ 00309 int supply_select(power_mgmt_supply_t supply); 00310 00311 /** 00312 * @brief Configure trickle charger charging path, also enable it 00313 * 00314 * @param[in] res Value of resister 00315 * @param[in] diode Enable diode 00316 * 00317 * @return 0 on success, error code on failure 00318 */ 00319 int trickle_charger_enable(trickle_charger_ohm_t res, bool diode); 00320 00321 /** 00322 * @brief Disable trickle charger 00323 * 00324 * @return 0 on success, error code on failure 00325 */ 00326 int trickle_charger_disable(); 00327 00328 /** 00329 * @brief Select square wave output frequency selection 00330 * 00331 * @param[in] freq Clock frequency, one of CLKOUT_FREQ_* 00332 * 00333 * @return 0 on success, error code on failure 00334 */ 00335 int set_output_square_wave_frequency(square_wave_out_freq_t freq); 00336 00337 /** 00338 * @brief Select external clock input frequency 00339 * 00340 * @param[in] freq Clock frequency, one of CLKIN_FREQ_* 00341 * 00342 * @return 0 on success, error code on failure 00343 */ 00344 int set_clkin_frequency(clkin_freq_t freq); 00345 00346 /** 00347 * @brief Select direction of INTB/CLKOUT pin 00348 * 00349 * @param[in] sel Pin function, one of CONFIGURE_PIN_B3_AS_INTB or CONFIGURE_PIN_B3_AS_CLKOUT 00350 * 00351 * @return 0 on success, error code on failure 00352 */ 00353 int configure_intb_clkout_pin(config_intb_clkout_pin_t sel); 00354 00355 /** 00356 * @brief Select direction of INTA/CLKIN pin 00357 * 00358 * @param[in] sel Pin function, one of CONFIGURE_PIN_B3_AS_INTA or CONFIGURE_PIN_B3_AS_CLKIN 00359 * 00360 * @return 0 on success, error code on failure 00361 */ 00362 int configure_inta_clkin_pin(config_inta_clkin_pin_t sel); 00363 00364 /** 00365 * @brief Initialize timer 00366 * 00367 * @param[in] value Timer initial value 00368 * @param[in] repeat Timer repeat mode enable/disable 00369 * @param[in] freq Timer frequency, one of TIMER_FREQ_* 00370 * @param[in] mode Timer mode, 0 or 1 00371 * 00372 * @return 0 on success, error code on failure 00373 * 00374 * @note \p mode controls the countdown timer interrupt function 00375 * along with \p repeat. 00376 * Pulse interrupt when \p mode = 0, irrespective of \p repeat (true or false) 00377 * Pulse interrupt when \p mode = 1 and \p repeat = true 00378 * Level interrupt when \p mode = 1 and \p repeat = false 00379 */ 00380 int timer_init(uint8_t value, bool repeat, timer_freq_t freq); 00381 00382 /** 00383 * @brief Read timer value 00384 * 00385 * @return 0 on success, error code on failure 00386 */ 00387 uint8_t timer_get(); 00388 00389 /** 00390 * @brief Enable timer 00391 * 00392 * @return 0 on success, error code on failure 00393 */ 00394 int timer_start(); 00395 00396 /** 00397 * @brief Pause timer, timer value is preserved 00398 * 00399 * @return 0 on success, error code on failure 00400 */ 00401 int timer_pause(); 00402 00403 /** 00404 * @brief Start timer from the paused value 00405 * 00406 * @return 0 on success, error code on failure 00407 */ 00408 int timer_continue(); 00409 00410 /** 00411 * @brief Disable timer 00412 * 00413 * @return 0 on success, error code on failure 00414 */ 00415 int timer_stop(); 00416 00417 /** 00418 * @brief Put device into data retention mode 00419 * 00420 * @return 0 on success, error code on failure 00421 */ 00422 int data_retention_mode_enter(); 00423 00424 /** 00425 * @brief Remove device from data retention mode 00426 * 00427 * @return 0 on success, error code on failure 00428 */ 00429 int data_retention_mode_exit(); 00430 00431 /** 00432 * @brief Enable I2C bus timeout mechanism 00433 * 00434 * @return 0 on success, error code on failure 00435 */ 00436 int i2c_timeout_enable(); 00437 00438 /** 00439 * @brief Disable I2C bus timeout mechanism 00440 * 00441 * @return 0 on success, error code on failure 00442 */ 00443 int i2c_timeout_disable(); 00444 00445 /** 00446 * @brief Enable interrupt 00447 * 00448 * @param[in] id Interrupt id, one of INTR_ID_* 00449 * 00450 * @return 0 on success, error code on failure 00451 */ 00452 int irq_enable(intr_id_t id); 00453 00454 /** 00455 * @brief Disable interrupt 00456 * 00457 * @param[in] id Interrupt id, one of INTR_ID_* 00458 * 00459 * @return 0 on success, error code on failure 00460 */ 00461 int irq_disable(intr_id_t id); 00462 00463 /** 00464 * @brief Disable all interrupts 00465 * 00466 * @return 0 on success, error code on failure 00467 */ 00468 int irq_disable_all(); 00469 00470 /** 00471 * @brief Set interrupt handler for a specific interrupt id 00472 * 00473 * @param[in] id Interrupt id, one of INTR_ID_* 00474 * @param[in] func Interrupt handler function 00475 * @param[in] cb Interrupt handler data 00476 */ 00477 void set_intr_handler(intr_id_t id, interrupt_handler_function func, void *cb); 00478 00479 /** 00480 * @brief Put device into reset state 00481 * 00482 * @return 0 on success, error code on failure 00483 */ 00484 int sw_reset_assert(); 00485 00486 /** 00487 * @brief Release device from state state 00488 * 00489 * @return 0 on success, error code on failure 00490 */ 00491 int sw_reset_release(); 00492 00493 /** 00494 * @brief Enable the RTC oscillator 00495 * 00496 * @return 0 on success, error code on failure 00497 */ 00498 int rtc_start(); 00499 00500 /** 00501 * @brief Disable the RTC oscillator 00502 * 00503 * @return 0 on success, error code on failure 00504 */ 00505 int rtc_stop(); 00506 00507 /** 00508 * @brief Base class destructor. 00509 */ 00510 ~RtcBase(); 00511 00512 protected: 00513 typedef struct { 00514 uint8_t config_reg1; 00515 uint8_t config_reg2; 00516 uint8_t int_ploarity_config; 00517 uint8_t timer_config; 00518 uint8_t int_en_reg; 00519 uint8_t int_status_reg; 00520 uint8_t seconds; 00521 uint8_t minutes; 00522 uint8_t hours; 00523 uint8_t day; 00524 uint8_t date; 00525 uint8_t month; 00526 uint8_t year; 00527 uint8_t alm1_sec; 00528 uint8_t alm1_min; 00529 uint8_t alm1_hrs; 00530 uint8_t alm1day_date; 00531 uint8_t alm1_mon; 00532 uint8_t alm1_year; 00533 uint8_t alm2_min; 00534 uint8_t alm2_hrs; 00535 uint8_t alm2day_date; 00536 uint8_t timer_count; 00537 uint8_t timer_init; 00538 uint8_t ram_start; 00539 uint8_t ram_end; 00540 uint8_t pwr_mgmt_reg; 00541 uint8_t trickle_reg; 00542 uint8_t clock_sync_delay; 00543 uint8_t temp_msb; 00544 uint8_t temp_lsb; 00545 uint8_t ts_config; 00546 } regmap_t; 00547 00548 typedef struct { 00549 union { 00550 unsigned char raw; 00551 struct { 00552 unsigned char seconds : 4; /**< RTC seconds value. */ 00553 unsigned char sec_10 : 3; /**< RTC seconds in multiples of 10 */ 00554 unsigned char : 1; 00555 } bits; 00556 struct { 00557 unsigned char value : 7; 00558 unsigned char : 1; 00559 } bcd; 00560 } seconds; 00561 00562 union { 00563 unsigned char raw; 00564 struct { 00565 unsigned char minutes : 4; /**< RTC minutes value */ 00566 unsigned char min_10 : 3; /**< RTC minutes in multiples of 10 */ 00567 unsigned char : 1; 00568 } bits; 00569 struct { 00570 unsigned char value : 7; 00571 unsigned char : 1; 00572 } bcd; 00573 } minutes; 00574 00575 union { 00576 unsigned char raw; 00577 struct { 00578 unsigned char hour : 4; /**< RTC hours value */ 00579 unsigned char hr_10 : 2; /**< RTC hours in multiples of 10 */ 00580 unsigned char : 2; 00581 } bits; 00582 struct { 00583 unsigned char value : 6; 00584 unsigned char : 2; 00585 } bcd; 00586 } hours; 00587 00588 union { 00589 unsigned char raw; 00590 struct { 00591 unsigned char day : 3; /**< RTC days */ 00592 unsigned char : 5; 00593 } bits; 00594 struct { 00595 unsigned char value : 3; 00596 unsigned char : 5; 00597 } bcd; 00598 } day; 00599 00600 union { 00601 unsigned char raw; 00602 struct { 00603 unsigned char date : 4; /**< RTC date */ 00604 unsigned char date_10 : 2; /**< RTC date in multiples of 10 */ 00605 unsigned char : 2; 00606 } bits; 00607 struct { 00608 unsigned char value : 6; 00609 unsigned char : 2; 00610 } bcd; 00611 } date; 00612 00613 union { 00614 unsigned char raw; 00615 struct { 00616 unsigned char month : 4; /**< RTC months */ 00617 unsigned char month_10 : 1; /**< RTC month in multiples of 10 */ 00618 unsigned char : 2; 00619 unsigned char century : 1; /**< Century bit */ 00620 } bits; 00621 struct { 00622 unsigned char value : 5; 00623 unsigned char : 3; 00624 } bcd; 00625 } month; 00626 00627 union { 00628 unsigned char raw; 00629 struct { 00630 unsigned char year : 4; /**< RTC years */ 00631 unsigned char year_10 : 4; /**< RTC year multiples of 10 */ 00632 } bits; 00633 struct { 00634 unsigned char value : 8; 00635 } bcd; 00636 } year; 00637 } rtc_time_regs_t; 00638 00639 typedef struct { 00640 union { 00641 unsigned char raw; 00642 struct { 00643 unsigned char seconds : 4; /**< Alarm1 seconds */ 00644 unsigned char sec_10 : 3; /**< Alarm1 seconds in multiples of 10 */ 00645 unsigned char a1m1 : 1; /**< Alarm1 mask bit for minutes */ 00646 } bits; 00647 struct { 00648 unsigned char value : 7; 00649 unsigned char : 1; 00650 } bcd; 00651 } sec; 00652 00653 union { 00654 unsigned char raw; 00655 struct { 00656 unsigned char minutes : 4; /**< Alarm1 minutes */ 00657 unsigned char min_10 : 3; /**< Alarm1 minutes in multiples of 10 */ 00658 unsigned char a1m2 : 1; /**< Alarm1 mask bit for minutes */ 00659 } bits; 00660 struct { 00661 unsigned char value : 7; 00662 unsigned char : 1; 00663 } bcd; 00664 } min; 00665 00666 union { 00667 unsigned char raw; 00668 struct { 00669 unsigned char hour : 4; /**< Alarm1 hours */ 00670 unsigned char hr_10 : 2; /**< Alarm1 hours in multiples of 10 */ 00671 unsigned char : 1; 00672 unsigned char a1m3 : 1; /**< Alarm1 mask bit for hours */ 00673 } bits; 00674 struct { 00675 unsigned char value : 6; 00676 unsigned char : 2; 00677 } bcd; 00678 } hrs; 00679 00680 union { 00681 unsigned char raw; 00682 struct { 00683 unsigned char day_date : 4; /**< Alarm1 day/date */ 00684 unsigned char date_10 : 2; /**< Alarm1 date in multiples of 10 */ 00685 unsigned char dy_dt : 1; 00686 unsigned char a1m4 : 1; /**< Alarm1 mask bit for day/date */ 00687 } bits; 00688 struct { 00689 unsigned char value : 3; 00690 unsigned char : 5; 00691 } bcd_day; 00692 struct { 00693 unsigned char value : 6; 00694 unsigned char : 2; 00695 } bcd_date; 00696 } day_date; 00697 00698 union { 00699 unsigned char raw; 00700 struct { 00701 unsigned char month : 4; /**< Alarm1 months */ 00702 unsigned char month_10 : 1; /**< Alarm1 months in multiples of 10 */ 00703 unsigned char : 1; 00704 unsigned char a1m6 : 1; /**< Alarm1 mask bit for year */ 00705 unsigned char a1m5 : 1; /**< Alarm1 mask bit for month */ 00706 } bits; 00707 struct { 00708 unsigned char value : 5; 00709 unsigned char : 3; 00710 } bcd; 00711 } mon; 00712 00713 union { 00714 unsigned char raw; 00715 struct { 00716 unsigned char year : 4; /* Alarm1 years */ 00717 unsigned char year_10 : 4; /* Alarm1 multiples of 10 */ 00718 } bits; 00719 struct { 00720 unsigned char value : 8; 00721 } bcd; 00722 } year; 00723 } alarm_regs_t; 00724 00725 typedef union { 00726 unsigned char raw; 00727 struct { 00728 unsigned char swrstn : 1; /**< Software reset */ 00729 unsigned char rs : 2; /**< Square wave output frequency selection on CLKOUT pin */ 00730 unsigned char osconz : 1; /**< Oscillator is on when set to 0. Oscillator is off when set to 1. */ 00731 unsigned char clksel : 2; /**< Selects the CLKIN frequency */ 00732 unsigned char intcn : 1; /**< Interrupt control bit. Selects the direction of INTB/CLKOUT */ 00733 unsigned char eclk : 1; /**< Enable external clock input */ 00734 } bits; 00735 } config_reg1_t; 00736 00737 typedef union { 00738 unsigned char raw; 00739 struct { 00740 unsigned char : 1; 00741 unsigned char set_rtc : 1; /**< Set RTC */ 00742 unsigned char rd_rtc : 1; /**< Read RTC. */ 00743 unsigned char i2c_timeout : 1; /**< I2C timeout Enable */ 00744 unsigned char bref : 2; /**< BREF sets the analog comparator threshold voltage. */ 00745 unsigned char data_reten : 1; /**< Sets the circuit into data retention mode. */ 00746 unsigned char : 1; 00747 } bits; 00748 } config_reg2_t; 00749 00750 typedef union { 00751 unsigned char raw; 00752 struct { 00753 unsigned char tfs : 2; /**< Timer frequency selection */ 00754 unsigned char trpt : 1; /**< Timer repeat mode. It controls the timer interrupt function along with TM. */ 00755 unsigned char : 1; 00756 unsigned char te : 1; /**< Timer enable */ 00757 unsigned char tpause : 1; /**< Timer Pause.*/ 00758 unsigned char : 2; 00759 } bits; 00760 } timer_config_t; 00761 00762 typedef union { 00763 unsigned char raw; 00764 struct { 00765 unsigned char d_mode : 2; /**< Sets the mode of the comparator to one of the two following: comparator mode, and power management mode/trickle charger mode. */ 00766 unsigned char d_man_sel : 1; /**< Default low. When this bit is low, input control block decides which supply to use. When this bit is high, user can manually select whether to use V<sub>CC</sub> or VBACKUP as supply. */ 00767 unsigned char d_vback_sel : 1; /**< : Default low. When this bit is low, and D_MANUAL_SEL is high, V<sub>CC</sub> is switched to supply. When this bit is high, and D_MANUAL_SEL is high, V<sub>BACKUP</sub> is switched to supply. */ 00768 unsigned char : 4; 00769 } bits; 00770 } pwr_mgmt_reg_t; 00771 00772 typedef union { 00773 unsigned char raw; 00774 struct { 00775 unsigned char sync_delay : 2; /* Sync delay to take for the internal countdown chain to reset after the rising edge of Set_RTC */ 00776 unsigned char : 6; 00777 } bits; 00778 } clock_sync_reg_t; 00779 00780 typedef union { 00781 unsigned char raw; 00782 struct { 00783 unsigned char automode : 1; /**< Automatic mode of temperature measurement. This mode is valid only when ONESHOTMODE=0. */ 00784 unsigned char oneshotmode : 1; /**< One-shot user requested temp measurement in real-time. AUTOMODE must be 0 in one-shot measurement mode. */ 00785 unsigned char ttint : 3; /**< Set temp measurement interval to specified time for auto mode */ 00786 unsigned char : 3; 00787 } bits; 00788 } ts_config_t; 00789 00790 static const uint8_t REG_NOT_AVAILABLE = 0xFF; 00791 00792 /** 00793 * @brief Base class constructor. 00794 * 00795 * @param[in] regmap Pointer to device register mappings. 00796 * @param[in] i2c Pointer to I2C bus object for this device. 00797 * @param[in] inta_pin MCU's pin number that device's INTA pin connected 00798 * @param[in] intb_pin MCU's pin number that device's INTB pin connected 00799 */ 00800 RtcBase(const regmap_t *regmap, I2C *i2c, PinName inta_pin, PinName intb_pin); 00801 00802 private: 00803 /* PRIVATE TYPE DECLARATIONS */ 00804 00805 /* PRIVATE VARIABLE DECLARATIONS */ 00806 I2C *i2c_handler; 00807 InterruptIn *inta_pin; 00808 InterruptIn *intb_pin; 00809 const regmap_t *regmap; 00810 00811 /* PRIVATE CONSTANT VARIABLE DECLARATIONS */ 00812 static const uint8_t I2C_WRITE = 0; 00813 static const uint8_t I2C_READ = 1; 00814 static const uint8_t MAX3134X_I2C_ADDRESS = 0x69; 00815 static const uint8_t MAX3134X_I2C_W = ((MAX3134X_I2C_ADDRESS << 1) | I2C_WRITE); 00816 static const uint8_t MAX3134X_I2C_R = ((MAX3134X_I2C_ADDRESS << 1) | I2C_READ); 00817 00818 enum config_reg2_set_rtc { 00819 CONFIG_REG2_SET_RTC_RTCRUN = 0, /**< Setting this bit to zero doesn't allow to write into the RTC */ 00820 CONFIG_REG2_SET_RTC_RTCPRGM, /**< This bit must be set to one, before writing into the RTC. i.e to set the initial time for the RTC this bit must be high. */ 00821 }; 00822 00823 /* PRIVATE FUNCTION DECLARATIONS */ 00824 void interrupt_handler(); 00825 00826 void (RtcBase::*funcptr)(void); 00827 00828 void post_interrupt_work(); 00829 00830 Thread *post_intr_work_thread; 00831 00832 struct handler { 00833 void (*func)(void *); 00834 void *cb; 00835 }; 00836 00837 handler interrupt_handler_list[INTR_ID_END]; 00838 00839 int tm_hour_to_rtc_hr12(int hours, bool *is_am); 00840 00841 int rtc_hr12_to_tm_hour(int hours, bool is_am); 00842 00843 int rtc_regs_to_time(struct tm *time, const rtc_time_regs_t *regs); 00844 00845 int time_to_rtc_regs(rtc_time_regs_t *regs, const struct tm *time); 00846 00847 int set_alarm_regs(alarm_no_t alarm_no, const alarm_regs_t *regs); 00848 00849 int get_alarm_regs(alarm_no_t alarm_no, alarm_regs_t *regs); 00850 00851 int time_to_alarm_regs(alarm_regs_t ®s, const struct tm *alarm_time); 00852 00853 int alarm_regs_to_time(struct tm *alarm_time, const alarm_regs_t *regs); 00854 00855 int set_alarm_period(alarm_no_t alarm_no, alarm_regs_t ®s, alarm_period_t period); 00856 00857 int set_rtc_time(); 00858 00859 int data_retention_mode_config(int state); 00860 00861 int i2c_timeout_config(int enable); 00862 00863 int set_clock_sync_delay(sync_delay_t delay); 00864 }; 00865 00866 #endif /* RTCBASE_H_ */
Generated on Sun Aug 7 2022 16:13:41 by
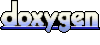