USB device stack
Dependents: blinky_max32630fthr FTHR_USB_serial FTHR_OLED HSP_RPC_GUI_3_0_1 ... more
Fork of USBDevice by
USBCDC.h
00001 /* Copyright (c) 2010-2011 mbed.org, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without 00005 * restriction, including without limitation the rights to use, copy, modify, merge, publish, 00006 * distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the 00007 * Software is furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 00019 #ifndef USBCDC_H 00020 #define USBCDC_H 00021 00022 /* These headers are included for child class. */ 00023 #include "USBEndpoints.h" 00024 #include "USBDescriptor.h" 00025 #include "USBDevice_Types.h" 00026 00027 #include "USBDevice.h" 00028 00029 class USBCDC: public USBDevice { 00030 public: 00031 00032 /* 00033 * Constructor 00034 * 00035 * @param vendor_id Your vendor_id 00036 * @param product_id Your product_id 00037 * @param product_release Your preoduct_release 00038 * @param connect_blocking define if the connection must be blocked if USB not plugged in 00039 */ 00040 USBCDC(uint16_t vendor_id, uint16_t product_id, uint16_t product_release, bool connect_blocking); 00041 00042 volatile bool terminal_connected; 00043 00044 protected: 00045 00046 /* 00047 * Get device descriptor. Warning: this method has to store the length of the report descriptor in reportLength. 00048 * 00049 * @returns pointer to the device descriptor 00050 */ 00051 virtual uint8_t * deviceDesc(); 00052 00053 /* 00054 * Get string product descriptor 00055 * 00056 * @returns pointer to the string product descriptor 00057 */ 00058 virtual uint8_t * stringIproductDesc(); 00059 00060 /* 00061 * Get string interface descriptor 00062 * 00063 * @returns pointer to the string interface descriptor 00064 */ 00065 virtual uint8_t * stringIinterfaceDesc(); 00066 00067 /* 00068 * Get configuration descriptor 00069 * 00070 * @returns pointer to the configuration descriptor 00071 */ 00072 virtual uint8_t * configurationDesc(); 00073 00074 /* 00075 * Send a buffer 00076 * 00077 * @param endpoint endpoint which will be sent the buffer 00078 * @param buffer buffer to be sent 00079 * @param size length of the buffer 00080 * @returns true if successful 00081 */ 00082 bool send(uint8_t * buffer, uint32_t size); 00083 00084 /* 00085 * Read a buffer from a certain endpoint. Warning: blocking 00086 * 00087 * @param endpoint endpoint to read 00088 * @param buffer buffer where will be stored bytes 00089 * @param size the number of bytes read will be stored in *size 00090 * @param maxSize the maximum length that can be read 00091 * @returns true if successful 00092 */ 00093 bool readEP(uint8_t * buffer, uint32_t * size); 00094 00095 /* 00096 * Read a buffer from a certain endpoint. Warning: non blocking 00097 * 00098 * @param endpoint endpoint to read 00099 * @param buffer buffer where will be stored bytes 00100 * @param size the number of bytes read will be stored in *size 00101 * @param maxSize the maximum length that can be read 00102 * @returns true if successful 00103 */ 00104 bool readEP_NB(uint8_t * buffer, uint32_t * size); 00105 00106 /* 00107 * Called by USBCallback_requestCompleted when CDC line coding is changed 00108 * Warning: Called in ISR 00109 * 00110 * @param baud The baud rate 00111 * @param bits The number of bits in a word (5-8) 00112 * @param parity The parity 00113 * @param stop The number of stop bits (1 or 2) 00114 */ 00115 virtual void lineCodingChanged(int baud, int bits, int parity, int stop) {}; 00116 00117 protected: 00118 virtual bool USBCallback_request(); 00119 virtual void USBCallback_requestCompleted(uint8_t *buf, uint32_t length); 00120 virtual bool USBCallback_setConfiguration(uint8_t configuration); 00121 00122 }; 00123 00124 #endif
Generated on Wed Jul 13 2022 12:44:03 by
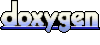