SIMO PMIC with 300mA Switching Charger
Embed:
(wiki syntax)
Show/hide line numbers
MAX77659.h
00001 /******************************************************************************* 00002 * Copyright (C) 2022 Analog Devices, Inc., All rights Reserved. 00003 * 00004 * This software is protected by copyright laws of the United States and 00005 * of foreign countries. This material may also be protected by patent laws 00006 * and technology transfer regulations of the United States and of foreign 00007 * countries. This software is furnished under a license agreement and/or a 00008 * nondisclosure agreement and may only be used or reproduced in accordance 00009 * with the terms of those agreements. Dissemination of this information to 00010 * any party or parties not specified in the license agreement and/or 00011 * nondisclosure agreement is expressly prohibited. 00012 * 00013 * The above copyright notice and this permission notice shall be included 00014 * in all copies or substantial portions of the Software. 00015 * 00016 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00017 * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00018 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00019 * IN NO EVENT SHALL ANALOG DEVICES BE LIABLE FOR ANY CLAIM, DAMAGES 00020 * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00021 * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00022 * OTHER DEALINGS IN THE SOFTWARE. 00023 * 00024 * Except as contained in this notice, the name of Maxim Integrated 00025 * Products, Inc. shall not be used except as stated in the Maxim Integrated 00026 * Products, Inc. Branding Policy. 00027 * 00028 * The mere transfer of this software does not imply any licenses 00029 * of trade secrets, proprietary technology, copyrights, patents, 00030 * trademarks, maskwork rights, or any other form of intellectual 00031 * property whatsoever. Analog Devices, Inc. retains all 00032 * ownership rights. 00033 ******************************************************************************* 00034 */ 00035 00036 #ifndef _MAX77659_H_ 00037 #define _MAX77659_H_ 00038 00039 #include "mbed.h" 00040 #include "MAX77659_regs.h" 00041 00042 #define MAX77659_NO_ERROR 0 00043 #define MAX77659_VALUE_NULL -1 00044 #define MAX77659_WRITE_DATA_FAILED -2 00045 #define MAX77659_READ_DATA_FAILED -3 00046 #define MAX77659_INVALID_DATA -4 00047 00048 #define MAX77659_I2C_ADDRESS 0x80 00049 00050 /** 00051 * @brief MAX77659 SIMO PMIC with 300mA Switching Charger. 00052 * 00053 * @details The MAX77659 provides highly-efficient integrated battery 00054 * charging and power supply solutions for low-power applications where size and efficiency are critical. 00055 * https://www.maximintegrated.com/en/products/power/power-management-ics/MAX77659.html 00056 * 00057 * @code 00058 * @endcode 00059 */ 00060 00061 class MAX77659 00062 { 00063 private: 00064 I2C *i2c_handler; 00065 InterruptIn *irq_pin; // interrupt pin 00066 00067 /** 00068 * @brief Register Addresses 00069 * @details Enumerated MAX77659 register addresses 00070 */ 00071 typedef enum { 00072 /*Global*/ 00073 INT_GLBL0 = 0x00, // Interrupt Status 0 00074 INT_GLBL1 = 0x04, // Interrupt Status 1 00075 ERCFLAG = 0x05, // Flags 00076 STAT_GLBL = 0x06, // Global Status 00077 INTM_GLBL1 = 0x08, // Interrupt Mask 1 00078 INTM_GLBL0 = 0x09, // Interrupt Mask 0 00079 CNFG_GLBL = 0x10, // Configuration Global 00080 CNFG_GPIO0 = 0x11, // GPIO0 Configuration 00081 CNFG_GPIO1 = 0x12, // GPIO1 Configuration 00082 CID = 0x14, // Chip Identification Code 00083 CNFG_WDT = 0x17, // Configuration WatchDog Timer 00084 /*Charger*/ 00085 INT_CHG = 0x01, // Charger Interrupt Status 00086 STAT_CHG_A = 0x02, // Charger Status A 00087 STAT_CHG_B = 0x03, // Charger Status B 00088 INT_M_CHG = 0x07, // Charger Interrupt Mask 00089 CNFG_CHG_A = 0x20, // Charger Configuration A 00090 CNFG_CHG_B = 0x21, // Charger Configuration B 00091 CNFG_CHG_C = 0x22, // Charger Configuration C 00092 CNFG_CHG_D = 0x23, // Charger Configuration D 00093 CNFG_CHG_E = 0x24, // Charger Configuration E 00094 CNFG_CHG_F = 0x25, // Charger Configuration F 00095 CNFG_CHG_G = 0x26, // Charger Configuration G 00096 CNFG_CHG_H = 0x27, // Charger Configuration H 00097 CNFG_CHG_I = 0x28, // Charger Configuration I 00098 /*SBB*/ 00099 CNFG_SBB0_A = 0x29, // SIMO Buck-Boost 0 Configuration A 00100 CNFG_SBB0_B = 0x2A, // SIMO Buck-Boost 0 Configuration B 00101 CNFG_SBB1_A = 0x2B, // SIMO Buck-Boost 1 Configuration A 00102 CNFG_SBB1_B = 0x2C, // SIMO Buck-Boost 1 Configuration B 00103 CNFG_SBB2_A = 0x2D, // SIMO Buck-Boost 2 Configuration A 00104 CNFG_SBB2_B = 0x2E, // SIMO Buck-Boost 2 Configuration B 00105 CNFG_SBB_TOP = 0x2F, // SIMO Buck-Boost Configuration 00106 CNFG_SBB_TOP_B = 0x30, // SIMO Buck-Boost Configuration 00107 /*LDO*/ 00108 CNFG_LDO0_A = 0x38, // LDO Configuration A 00109 CNFG_LDO0_B = 0x39 // LDO Configuration B 00110 } reg_t; 00111 00112 /** 00113 * @brief Interrupt handler function 00114 */ 00115 void interrupt_handler(); 00116 00117 void (MAX77659::*funcptr)(void); 00118 00119 /** 00120 * @brief Post interrupt jobs after interrupt is detected. 00121 */ 00122 void post_interrupt_work(); 00123 00124 Thread *post_intr_work_thread; 00125 00126 struct handler { 00127 void (*func)(void *); 00128 void *cb; 00129 }; 00130 00131 handler *interrupt_handler_list; 00132 00133 public: 00134 /** 00135 * @brief Register Configuration 00136 * All Interrupt Flags combined from INT_GLBL0, INT_GLBL1 and INT_CHG 00137 * 00138 * @details 00139 * - Register : ERCFLAG (0x05) 00140 * - Bit Fields : [7:0] 00141 * - Default : 0x0 00142 * - Description : Enumerated interrupt flags 00143 */ 00144 typedef enum { 00145 INT_GLBL0_GPI0_F, 00146 INT_GLBL0_GPI0_R, 00147 INT_GLBL0_NEN_F, 00148 INT_GLBL0_NEN_R, 00149 INT_GLBL0_TJAL1_R, 00150 INT_GLBL0_TJAL2_R, 00151 INT_GLBL0_DOD_R, 00152 INT_GLBL1_GPI1_F, 00153 INT_GLBL1_GPI1_R, 00154 INT_GLBL1_SBB_TO, 00155 INT_GLBL1_LDO_F, 00156 INT_CHG_THM_I, 00157 INT_CHG_CGH_I, 00158 INT_CHG_CHGIN_I, 00159 INT_CHG_TJ_REG_I, 00160 INT_CHG_SYS_CTRL_I, 00161 INT_CHG_END 00162 } reg_bit_int_glbl_t; 00163 00164 /** 00165 * MAX77659 constructor. 00166 */ 00167 MAX77659(I2C *i2c, PinName IRQPin = NC); 00168 00169 /** 00170 * MAX77659 destructor. 00171 */ 00172 ~MAX77659(); 00173 00174 /** 00175 * @brief Function pointer type to interrupt handler function 00176 */ 00177 typedef void (*interrupt_handler_function)(void *); 00178 00179 /** 00180 * @brief Read from a register. 00181 * 00182 * @param[in] reg Address of a register to be read. 00183 * @param[out] value Pointer to save result value. 00184 * 00185 * @returns 0 on success, negative error code on failure. 00186 */ 00187 int read_register(uint8_t reg, uint8_t *value); 00188 00189 /** 00190 * @brief Write to a register. 00191 * 00192 * @param[in] reg Address of a register to be written. 00193 * @param[out] value Pointer of value to be written to register. 00194 * 00195 * @returns 0 on success, negative error code on failure. 00196 */ 00197 int write_register(uint8_t reg, const uint8_t *value); 00198 00199 /** 00200 * @brief Register Configuration 00201 * 00202 * @details 00203 * - Register : ERCFLAG (0x05) 00204 * - Bit Fields : [7:0] 00205 * - Default : 0x0 00206 * - Description : Event Recorder Flags. 00207 */ 00208 typedef enum { 00209 ERCFLAG_TOVLD, 00210 ERCFLAG_SYSOVLO, 00211 ERCFLAG_AVLUVLO, 00212 ERCFLAG_MRST, 00213 ERCFLAG_SFT_OFF_F, 00214 ERCFLAG_SFT_CRST_F, 00215 ERCFLAG_WDT_OFF, 00216 ERCFLAG_WDT_RST 00217 }reg_bit_ercflag_t; 00218 00219 /** 00220 * @brief Get bit field of ERCFLAG (0x05) register. 00221 * 00222 * @param[in] bit_field ERCFLAG register bit field to be written. 00223 * @param[out] flag Pointer to save result of ercglag bit states. 00224 * For individual bit 00225 * 0x0: ERCFLAG has not occurred, 00226 * 0x1: ERCFLAG has occurred. 00227 * 00228 * @return 0 on success, error code on failure. 00229 */ 00230 int get_ercflag(reg_bit_ercflag_t bit_field, uint8_t *flag); 00231 00232 /** 00233 * @brief Register Configuration 00234 * 00235 * @details 00236 * - Register : STAT_GLBL (0x06) 00237 * - Bit Fields : [7:0] 00238 * - Default : 0x0 00239 * - Description : Event Recorder Flags. 00240 */ 00241 typedef enum { 00242 STAT_GLBL_STAT_IRQ, 00243 STAT_GLBL_STAT_EN, 00244 STAT_GLBL_TJAL1_S, 00245 STAT_GLBL_TJAL2_S, 00246 STAT_GLBL_RSVD, 00247 STAT_GLBL_DOD_S, 00248 STAT_GLBL_BOK, 00249 STAT_GLBL_DIDM 00250 }reg_bit_stat_glbl_t; 00251 00252 /** 00253 * @brief Get bit field of STAT_GLBL (0x06) register. 00254 * 00255 * @param[in] bit_field STAT_GLBL register bit field to be written. 00256 * @param[out] status Pointer to save result of Status Global bit state. 00257 * 00258 * @return 0 on success, error code on failure. 00259 */ 00260 int get_stat_glbl(reg_bit_stat_glbl_t bit_field, uint8_t *status); 00261 00262 /** 00263 * @brief Register Configuration 00264 * 00265 * @details 00266 * - Register : INT_M_CHG (0x07), INTM_GLBL1 (0x08) and INTM_GLBL0 (0x09) 00267 * - Bit Fields : [7:0] 00268 * - Default : 0x0 00269 * - Description : All interrupt mask bits. 00270 */ 00271 typedef enum { 00272 INT_M_CHG_THM_M, 00273 INT_M_CHG_CHG_M, 00274 INT_M_CHG_CHGIN_M, 00275 INT_M_CHG_TJ_REG_M, 00276 INT_M_CHG_SYS_CTRL_M, 00277 INTM_GLBL1_GPI1_FM = 8, 00278 INTM_GLBL1_GPI1_RM, 00279 INTM_GLBL1_SBB_TO_M, 00280 INTM_GLBL1_LDO_M, 00281 INTM_GLBL0_GPI0_FM = 16, 00282 INTM_GLBL0_GPI0_RM, 00283 INTM_GLBL0_nEN_FM, 00284 INTM_GLBL0_nEN_RM, 00285 INTM_GLBL0_TJAL1_RM, 00286 INTM_GLBL0_TJAL2_RM, 00287 INTM_GLBL0_DOD_RM, 00288 INTM_NUM_OF_BIT 00289 }reg_bit_int_mask_t; 00290 00291 /** 00292 * @brief Set bit field of INT_M_CHG (0x07), INTM_GLBL1 (0x08) or INTM_GLBL0 (0x09) register. 00293 * 00294 * @param[in] bit_field Register bit field to be set. 00295 * @param[out] maskBit 0x0: Interrupt is unmasked, 00296 * 0x1: Interrupt is masked. 00297 * 00298 * @return 0 on success, error code on failure. 00299 */ 00300 int set_interrupt_mask(reg_bit_int_mask_t bit_field, uint8_t maskBit); 00301 00302 /** 00303 * @brief Get bit field of INT_M_CHG (0x07), INTM_GLBL0 (0x08) or INTM_GLBL1 (0x09) register. 00304 * 00305 * @param[in] bit_field Register bit field to be written. 00306 * @param[out] maskBit 0x0: Interrupt is unmasked, 00307 * 0x1: Interrupt is masked. 00308 * 00309 * @return 0 on success, error code on failure. 00310 */ 00311 int get_interrupt_mask(reg_bit_int_mask_t bit_field, uint8_t *maskBit); 00312 00313 /** 00314 * @brief Register Configuration 00315 * 00316 * @details 00317 * - Register : CNFG_GLBL (0x10) 00318 * - Bit Fields : [7:0] 00319 * - Default : 0x0 00320 * - Description : Event Recorder Flags. 00321 */ 00322 typedef enum { 00323 CNFG_GLBL_SFT_CTRL, 00324 CNFG_GLBL_DBEN_nEN, 00325 CNFG_GLBL_nEN_MODE, 00326 CNFG_GLBL_SBIA_EN, 00327 CNFG_GLBL_SBIA_LPM, 00328 CNFG_GLBL_T_MRST, 00329 CNFG_GLBL_PU_DIS 00330 }reg_bit_cnfg_glbl_t; 00331 00332 /** 00333 * @brief Set CNFG_GLBL (0x10) register. 00334 * 00335 * @param[in] bit_field Register bit field to be written. 00336 * @param[in] config Register bit field to be written. 00337 * 00338 * @return 0 on success, error code on failure. 00339 */ 00340 int set_cnfg_glbl(reg_bit_cnfg_glbl_t bit_field, uint8_t config); 00341 00342 /** 00343 * @brief Get CNFG_GLBL (0x10) register. 00344 * 00345 * @param[in] bit_field Register bit field to be written. 00346 * @param[out] config Pointer of value to be read. 00347 * 00348 * @return 0 on success, error code on failure. 00349 */ 00350 int get_cnfg_glbl(reg_bit_cnfg_glbl_t bit_field, uint8_t *config); 00351 00352 /** 00353 * @brief Register Configuration 00354 * 00355 * @details 00356 * - Register : CNFG_GPIO0 (0x11) or CNFG_GPIO1 (0x12) 00357 * - Bit Fields : [7:0] 00358 * - Default : 0x0 00359 * - Description : Event Recorder Flags. 00360 */ 00361 typedef enum { 00362 CNFG_GPIO_DIR, 00363 CNFG_GPIO_DI, 00364 CNFG_GPIO_DRV, 00365 CNFG_GPIO_DO, 00366 CNFG_GPIO_DBEN_GPI, 00367 CNFG_GPIO_ALT_GPIO, 00368 CNFG_GPIO_RSVD 00369 }reg_bit_cnfg_gpio_t; 00370 00371 /** 00372 * @brief Set either CNFG_GPIO0 (0x11) or CNFG_GPIO1 (0x12). 00373 * 00374 * @param[in] bit_field Register bit field to be written. 00375 * @param[in] channel Channel number: 0 or 1 00376 * @param[in] config Register bit field to be written. 00377 * 00378 * @return 0 on success, error code on failure. 00379 */ 00380 int set_cnfg_gpio(reg_bit_cnfg_gpio_t bit_field, uint8_t channel, uint8_t config); 00381 00382 /** 00383 * @brief Get either CNFG_GPIO0 (0x11) or CNFG_GPIO1 (0x12). 00384 * 00385 * @param[in] bit_field Register bit field to be written. 00386 * @param[in] channel Channel number: 0 or 1 00387 * @param[out] config Pointer of value to be read. 00388 * 00389 * @return 0 on success, error code on failure. 00390 */ 00391 int get_cnfg_gpio(reg_bit_cnfg_gpio_t bit_field, uint8_t channel, uint8_t *config); 00392 00393 /** 00394 * @brief Get bit field of CID (0x14) register. 00395 * 00396 * @return CID on success, error code on failure. 00397 */ 00398 int get_cid(void); 00399 00400 /** 00401 * @brief Register Configuration 00402 * 00403 * @details 00404 * - Register : CNFG_WDT (0x17) 00405 * - Bit Fields : [7:0] 00406 * - Default : 0x0 00407 * - Description : Watchdog Timer Configuration. 00408 */ 00409 typedef enum { 00410 CNFG_WDT_WDT_LOCK, 00411 CNFG_WDT_WDT_EN, 00412 CNFG_WDT_WDT_CLR, 00413 CNFG_WDT_WDT_MODE, 00414 CNFG_WDT_WDT_PER, 00415 CNFG_WDT_RSVD 00416 }reg_bit_cnfg_wdt_t; 00417 00418 /** 00419 * @brief Set CNFG_WDT (0x17) register. 00420 * 00421 * @param[in] bit_field Register bit field to be written. 00422 * @param[in] config Field value to be written. 00423 * 00424 * @return 0 on success, error code on failure. 00425 */ 00426 int set_cnfg_wdt(reg_bit_cnfg_wdt_t bit_field, uint8_t config); 00427 00428 /** 00429 * @brief Get CNFG_WDT (0x17) register. 00430 * 00431 * @param[in] bit_field Register bit field to be written. 00432 * @param[out] config Pointer of value to be read. 00433 * 00434 * @return 0 on success, error code on failure. 00435 */ 00436 int get_cnfg_wdt(reg_bit_cnfg_wdt_t bit_field, uint8_t *config); 00437 00438 /** 00439 * @brief Register Configuration 00440 * 00441 * @details 00442 * - Register : STAT_CHG_A (0x02) 00443 * - Bit Fields : [7:0] 00444 * - Default : 0x0 00445 * - Description : Watchdog Timer Configuration. 00446 */ 00447 typedef enum { 00448 STAT_CHG_A_THM_DTLS, 00449 STAT_CHG_A_TJ_REG_STAT, 00450 STAT_CHG_A_VSYS_MIN_STAT, 00451 STAT_CHG_A_RSVD 00452 }reg_bit_stat_chg_a_t; 00453 00454 /** 00455 * @brief Get STAT_CHG_A (0x02) register. 00456 * 00457 * @param[in] bit_field Register bit field to be written. 00458 * @param[out] status Pointer of value to be read. 00459 * For individual bit, 00460 * 0x0 = It is not engaged, 00461 * 0x1 = It is engaged. 00462 * 00463 * @return 0 on success, error code on failure. 00464 */ 00465 int get_stat_chg_a(reg_bit_stat_chg_a_t bit_field, uint8_t *status); 00466 00467 /** 00468 * @brief Register Configuration 00469 * 00470 * @details 00471 * - Register : STAT_CHG_A (0x02) 00472 * - Bit Fields : [2:0] 00473 * - Default : 0x0 00474 * - Description : Battery Temperature Details. 00475 */ 00476 typedef enum { 00477 THM_DTLS_THERMISTOR_DISABLED, 00478 THM_DTLS_BATTERY_COLD, 00479 THM_DTLS_BATTERY_COOL, 00480 THM_DTLS_BATTERY_WARM, 00481 THM_DTLS_BATTERY_HOT, 00482 THM_DTLS_BATTERY_NORMAL, 00483 THM_DTLS_RESERVED_0x06, 00484 THM_DTLS_RESERVED_0x07 00485 }decode_thm_dtls_t; 00486 00487 /** 00488 * @brief Get Battery Temperature Details. 00489 * Valid only when CHGIN_DTLS[1:0] = 0b11. 00490 * 00491 * @param[out] thm_dtls Battery temperature details field to be read. 00492 * 00493 * @return 0 on success, error code on failure. 00494 */ 00495 int get_thm_dtls(decode_thm_dtls_t *thm_dtls); 00496 00497 /** 00498 * @brief Register Configuration 00499 * 00500 * @details 00501 * - Register : STAT_CHG_B (0x03) 00502 * - Bit Fields : [7:0] 00503 * - Default : 0x0 00504 * - Description : Watchdog Timer Configuration. 00505 */ 00506 typedef enum { 00507 STAT_CHG_B_TIME_SUS, 00508 STAT_CHG_B_CHG, 00509 STAT_CHG_B_CHGIN_DTLS, 00510 STAT_CHG_B_CHG_DTLS 00511 }reg_bit_stat_chg_b_t; 00512 00513 /** 00514 * @brief Get STAT_CHG_B (0x03) register. 00515 * 00516 * @param[in] bit_field Register bit field to be written. 00517 * @param[out] status Pointer of value to be read. 00518 * 00519 * @return 0 on success, error code on failure. 00520 */ 00521 int get_stat_chg_b(reg_bit_stat_chg_b_t bit_field, uint8_t *status); 00522 00523 /** 00524 * @brief Register Configuration 00525 * 00526 * @details 00527 * - Register : STAT_CHG_B (0x03) 00528 * - Bit Fields : [7:4] 00529 * - Default : 0x0 00530 * - Description : CHG_DTLS[3:0] Charger Details. 00531 */ 00532 typedef enum { 00533 CHG_DTLS_OFF, 00534 CHG_DTLS_PREQUALIFICATION_MODE, 00535 CHG_DTLS_FAST_CHARGE_CC, 00536 CHG_DTLS_JEITA_FAST_CHARGE_CC, 00537 CHG_DTLS_FAST_CHARGE_CV, 00538 CHG_DTLS_JEITA_FAST_CHARGE_CV, 00539 CHG_DTLS_TOP_OFF_MODE, 00540 CHG_DTLS_JEITA_MODIFIED_TOP_OFF_MODE, 00541 CHG_DTLS_DONE, 00542 CHG_DTLS_JEITA_MODIFIED_DONE, 00543 CHG_DTLS_PREQUALIFICATION_TIMER_FAULT, 00544 CHG_DTLS_FAST_CHARGE_TIMER_FAULT, 00545 CHG_DTLS_BATTERY_TEMPERATURE_FAULT, 00546 CHG_DTLS_RESERVED_0x0D, 00547 CHG_DTLS_RESERVED_0x0E, 00548 CHG_DTLS_RESERVED_0x0F 00549 }decode_chg_dtls_t; 00550 00551 /** 00552 * @brief Get Charger Details. 00553 * 00554 * @param[out] chg_dtls Charger details field to be read. 00555 * 00556 * @return 0 on success, error code on failure. 00557 */ 00558 int get_chg_dtls(decode_chg_dtls_t *chg_dtls); 00559 00560 /** 00561 * @brief Register Configuration 00562 * 00563 * @details 00564 * - Register : CNFG_CHG_A (0x20) 00565 * - Bit Fields : [7:6] 00566 * - Default : 0x0 00567 * - Description : VHOT JEITA Temperature Threshold. 00568 */ 00569 typedef enum { 00570 THM_HOT_VOLT_0_411V, 00571 THM_HOT_VOLT_0_367V, 00572 THM_HOT_VOLT_0_327V, 00573 THM_HOT_VOLT_0_291V 00574 }decode_thm_hot_t; 00575 00576 /** 00577 * @brief Set the VHOT JEITA Temperature Threshold. 00578 * 00579 * @param[in] thm_hot The VHOT JEITA temperature threshold field to be written. 00580 * 00581 * @return 0 on success, error code on failure. 00582 */ 00583 int set_thm_hot(decode_thm_hot_t thm_hot); 00584 00585 /** 00586 * @brief Get the VHOT JEITA Temperature Threshold. 00587 * 00588 * @param[out] thm_hot The VHOT JEITA temperature threshold field to be read. 00589 * 00590 * @return 0 on success, error code on failure. 00591 */ 00592 int get_thm_hot(decode_thm_hot_t *thm_hot); 00593 00594 /** 00595 * @brief Register Configuration 00596 * 00597 * @details 00598 * - Register : CNFG_CHG_A (0x20) 00599 * - Bit Fields : [5:4] 00600 * - Default : 0x0 00601 * - Description : VWARM JEITA Temperature Threshold. 00602 */ 00603 typedef enum { 00604 THM_WARM_VOLT_0_511V, 00605 THM_WARM_VOLT_0_459V, 00606 THM_WARM_VOLT_0_411V, 00607 THM_WARM_VOLT_0_367V 00608 }decode_thm_warm_t; 00609 00610 /** 00611 * @brief Set the VWARM JEITA Temperature Threshold. 00612 * 00613 * @param[in] thm_warm The VWARM JEITA temperature threshold field to be written. 00614 * 00615 * @return 0 on success, error code on failure. 00616 */ 00617 int set_thm_warm(decode_thm_warm_t thm_warm); 00618 00619 /** 00620 * @brief Get the VWARM JEITA Temperature Threshold. 00621 * 00622 * @param[out] thm_warm The VWARM JEITA temperature threshold field to be read. 00623 * 00624 * @return 0 on success, error code on failure. 00625 */ 00626 int get_thm_warm(decode_thm_warm_t *thm_warm); 00627 00628 /** 00629 * @brief Register Configuration 00630 * 00631 * @details 00632 * - Register : CNFG_CHG_A (0x20) 00633 * - Bit Fields : [3:2] 00634 * - Default : 0x0 00635 * - Description : VCOOL JEITA Temperature Threshold. 00636 */ 00637 typedef enum { 00638 THM_COOL_VOLT_0_923V, 00639 THM_COOL_VOLT_0_867V, 00640 THM_COOL_VOLT_0_807V, 00641 THM_COOL_VOLT_0_747V 00642 }decode_thm_cool_t; 00643 00644 /** 00645 * @brief Set the VCOOL JEITA Temperature Threshold. 00646 * 00647 * @param[in] thm_cool The VCOOL JEITA temperature threshold field to be written. 00648 * 00649 * @return 0 on success, error code on failure. 00650 */ 00651 int set_thm_cool(decode_thm_cool_t thm_cool); 00652 00653 /** 00654 * @brief Get the VCOOL JEITA Temperature Threshold. 00655 * 00656 * @param[out] thm_cool The VCOOL JEITA temperature threshold field to be read. 00657 * 00658 * @return 0 on success, error code on failure. 00659 */ 00660 int get_thm_cool(decode_thm_cool_t *thm_cool); 00661 00662 /** 00663 * @brief Register Configuration 00664 * 00665 * @details 00666 * - Register : CNFG_CHG_A (0x20) 00667 * - Bit Fields : [1:0] 00668 * - Default : 0x0 00669 * - Description : VCOLD JEITA Temperature Threshold. 00670 */ 00671 typedef enum { 00672 THM_COLD_VOLT_1_024V, 00673 THM_COLD_VOLT_0_976V, 00674 THM_COLD_VOLT_0_923V, 00675 THM_COLD_VOLT_0_867V 00676 }decode_thm_cold_t; 00677 00678 /** 00679 * @brief Set the VCOLD JEITA Temperature Threshold. 00680 * 00681 * @param[in] thm_cold The VCOLD JEITA temperature threshold field to be written. 00682 * 00683 * @return 0 on success, error code on failure. 00684 */ 00685 int set_thm_cold(decode_thm_cold_t thm_cold); 00686 00687 /** 00688 * @brief Get the VCOLD JEITA Temperature Threshold. 00689 * 00690 * @param[out] thm_cold The VCOLD JEITA temperature threshold field to be read. 00691 * 00692 * @return 0 on success, error code on failure. 00693 */ 00694 int get_thm_cold(decode_thm_cold_t *thm_cold); 00695 00696 /** 00697 * @brief Register Configuration 00698 * 00699 * @details 00700 * - Register : CNFG_CHG_B (0x21) 00701 * - Bit Fields : [7:0] 00702 * - Default : 0x0 00703 * - Description : Watchdog Timer Configuration. 00704 */ 00705 typedef enum { 00706 CNFG_CHG_B_CHG_EN, 00707 CNFG_CHG_B_I_PQ, 00708 CNFG_CHG_B_RSVD 00709 }reg_bit_cnfg_chg_b_t; 00710 00711 /** 00712 * @brief Set CNFG_CHG_B (0x21) register. 00713 * 00714 * @param[in] bit_field Register bit field to be written. 00715 * @param[in] config Register bit field to be written. 00716 * 00717 * @return 0 on success, error code on failure. 00718 */ 00719 int set_cnfg_chg_b(reg_bit_cnfg_chg_b_t bit_field, uint8_t config); 00720 00721 /** 00722 * @brief Get CNFG_CHG_B (0x21) register. 00723 * 00724 * @param[in] bit_field Register bit field to be written. 00725 * @param[out] config Pointer of value to be read. 00726 * 00727 * @return 0 on success, error code on failure. 00728 */ 00729 int get_cnfg_chg_b(reg_bit_cnfg_chg_b_t bit_field, uint8_t *config); 00730 00731 /** 00732 * @brief Set Battery Prequalification Voltage Threshold (VPQ). 00733 * Bit 7:5 of CNFG_CHG_C (0x22) register. 00734 * 00735 * @param[in] voltV 00736 * 2.3V, 2.4V, 2.5V, 2.6V, 00737 * 2.7V, 2.8V, 2.9V, 3.0V. 00738 * 00739 * @return 0 on success, error code on failure. 00740 */ 00741 int set_chg_pq(float voltV); 00742 00743 /** 00744 * @brief Get Battery Prequalification Voltage Threshold (VPQ). 00745 * Bit 7:5 of CNFG_CHG_C (0x22) register. 00746 * 00747 * @param[out] voltV Pointer of value to be read. 00748 * 2.3V, 2.4V, 2.5V, 2.6V, 00749 * 2.7V, 2.8V, 2.9V, 3.0V. 00750 * 00751 * @return 0 on success, error code on failure. 00752 */ 00753 int get_chg_pq(float *voltV); 00754 00755 /** 00756 * @brief Set Charger Termination Current (ITERM). 00757 * I_TERM[1:0] sets the charger termination current 00758 * as a percentage of the fast charge current IFAST-CHG. 00759 * Bit 4:3 of CNFG_CHG_C (0x22) register. 00760 * 00761 * @param[in] percent 00762 * 5%, 7.5%, 10%, 15%. 00763 * 00764 * @return 0 on success, error code on failure. 00765 */ 00766 int set_i_term(float percent); 00767 00768 /** 00769 * @brief Get Charger Termination Current (ITERM). 00770 * I_TERM[1:0] sets the charger termination current 00771 * as a percentage of the fast charge current IFAST-CHG. 00772 * Bit 4:3 of CNFG_CHG_C (0x22) register. 00773 * 00774 * @param[out] percent Pointer of value to be read. 00775 * 5%, 7.5%, 10%, 15%. 00776 * 00777 * @return 0 on success, error code on failure. 00778 */ 00779 int get_i_term(float *percent); 00780 00781 /** 00782 * @brief Set Top-off Timer Value. 00783 * Bit 2:0 of CNFG_CHG_C (0x22) register. 00784 * 00785 * @param[in] minute 00786 * 0 minutes, 5 minutes, 10 minutes 00787 * 15 minutes, 20 minutes, 25 minutes, 00788 * 30 minutes, 35 minutes. 00789 * 00790 * @return 0 on success, error code on failure. 00791 */ 00792 int set_t_topoff(uint8_t minute); 00793 00794 /** 00795 * @brief Get Top-off Timer Value. 00796 * Bit 2:0 of CNFG_CHG_C (0x22) register. 00797 * 00798 * @param[out] minute Pointer of value to be read. 00799 0 minutes, 5 minutes, 10 minutes 00800 * 15 minutes, 20 minutes, 25 minutes, 00801 * 30 minutes, 35 minutes. 00802 * 00803 * @return 0 on success, error code on failure. 00804 */ 00805 int get_t_topoff(uint8_t *minute); 00806 00807 /** 00808 * @brief Set the Die Junction Temperature Regulation Point, TJ-REG. 00809 * Bit 7:5 of CNFG_CHG_D (0x23) register. 00810 * 00811 * @param[in] tempDegC 60ºC, 70ºC, 80ºC, 00812 * 90ºC, 100ºC. 00813 * 00814 * @return 0 on success, error code on failure. 00815 */ 00816 int set_tj_reg(uint8_t tempDegC); 00817 00818 /** 00819 * @brief Get the Die Junction Temperature Regulation Point, TJ-REG. 00820 * Bit 7:5 of CNFG_CHG_D (0x23) register. 00821 * 00822 * @param[out] tempDegC Pointer of value to be read. 00823 * 60ºC, 70ºC, 80ºC, 90ºC, 100ºC. 00824 * 00825 * @return 0 on success, error code on failure. 00826 */ 00827 int get_tj_reg(uint8_t *tempDegC); 00828 00829 /** 00830 * @brief Register Configuration 00831 * 00832 * @details 00833 * - Register : CNFG_CHG_D (0x23) 00834 * - Bit Fields : [4] 00835 * - Default : 0x0 00836 * - Description : SYS Headroom Voltage Regulation. 00837 */ 00838 typedef enum { 00839 VSYS_HDRM_VOLT_0_15, 00840 VSYS_HDRM_VOLT_0_20 00841 }decode_vsys_hdrm_t; 00842 00843 /** 00844 * @brief Set SYS Headroom Voltage Regulation. 00845 * 00846 * @param[in] vsys_hdrm SYS Headroom Voltage field to be written. 00847 * 00848 * @return 0 on success, error code on failure. 00849 */ 00850 int set_vsys_hdrm(decode_vsys_hdrm_t vsys_hdrm); 00851 00852 /** 00853 * @brief Get SYS Headroom Voltage Regulation. 00854 * 00855 * @param[out] vsys_hdrm SYS Headroom Voltage field to be read. 00856 * 00857 * @return 0 on success, error code on failure. 00858 */ 00859 int get_vsys_hdrm(decode_vsys_hdrm_t *vsys_hdrm); 00860 00861 /** 00862 * @brief Register Configuration 00863 * 00864 * @details 00865 * - Register : CNFG_CHG_D (0x23) 00866 * - Bit Fields : [1:0] 00867 * - Default : 0x0 00868 * - Description : Minimum SYS Voltage. 00869 */ 00870 typedef enum { 00871 VSYS_MIN_VOLT_3_2, 00872 VSYS_MIN_VOLT_3_3, 00873 VSYS_MIN_VOLT_3_4, 00874 VSYS_MIN_VOLT_3_5 00875 }decode_vsys_min_t; 00876 00877 /** 00878 * @brief Set Minimum SYS Voltage. 00879 * Bit 1:0 of CNFG_CHG_D (0x23) register. 00880 * 00881 * @param[in] vsys_min Decoded values for 3.2V, 3.3V, 3.4V, 3.5V. 00882 * 00883 * @return 0 on success, error code on failure. 00884 */ 00885 int set_vsys_min(decode_vsys_min_t vsys_min); 00886 00887 /** 00888 * @brief Get Minimum SYS Voltage. 00889 * Bit 1:0 of CNFG_CHG_D (0x23) register. 00890 * 00891 * @param[out] vsys_min Pointer of value to be read. 00892 * 00893 * @return 0 on success, error code on failure. 00894 */ 00895 int get_vsys_min(decode_vsys_min_t *vsys_min); 00896 00897 /** 00898 * @brief Set the Fast-Charge Constant Current Value, IFAST-CHG. 00899 * Bit 7:2 of CNFG_CHG_E (0x24) register. 00900 * 00901 * @param[in] currentmA 7.5mA, 15.0mA, 22.5mA, ... 00902 * 292.5mA, 300.0mA. 00903 * 00904 * @return 0 on success, error code on failure. 00905 */ 00906 int set_chg_cc(float currentmA); 00907 00908 /** 00909 * @brief Get the Fast-Charge Constant Current Value, IFAST-CHG. 00910 * Bit 7:2 of CNFG_CHG_E (0x24) register. 00911 * 00912 * @param[out] currentmA Pointer of value to be read. 00913 * 7.5mA, 15.0mA, 22.5mA, ... 00914 * 292.5mA, 300.0mA. 00915 * 00916 * @return 0 on success, error code on failure. 00917 */ 00918 int get_chg_cc(float *currentmA); 00919 00920 /** 00921 * @brief Register Configuration 00922 * 00923 * @details 00924 * - Register : CNFG_CHG_E (0x24) 00925 * - Bit Fields : [1:0] 00926 * - Default : 0x0 00927 * - Description : Fast-charge Safety timer, tFC. 00928 */ 00929 typedef enum { 00930 T_FAST_CHG_TIMER_DISABLED, 00931 T_FAST_CHG_HOUR_3H, 00932 T_FAST_CHG_HOUR_5H, 00933 T_FAST_CHG_HOUR_7H 00934 }decode_t_fast_chg_t; 00935 00936 /** 00937 * @brief Set the Fast-charge Safety timer, tFC. 00938 * Bit 1:0 of CNFG_CHG_E (0x24) register. 00939 * 00940 * @param[in] t_fast_chg Fast-charge safety timer field to be written. 00941 * 00942 * @return 0 on success, error code on failure. 00943 */ 00944 int set_t_fast_chg(decode_t_fast_chg_t t_fast_chg); 00945 00946 /** 00947 * @brief Get the Fast-charge Safety timer, tFC. 00948 * Bit 1:0 of CNFG_CHG_E (0x24) register. 00949 * 00950 * @param[out] t_fast_chg Fast-charge safety timer field to be read. 00951 * 00952 * @return 0 on success, error code on failure. 00953 */ 00954 int get_t_fast_chg(decode_t_fast_chg_t *t_fast_chg); 00955 00956 /** 00957 * @brief Set IFAST-CHG-JEITA 00958 * when the battery is either cool or warm as defined by the 00959 * VCOOL and VWARM temperature thresholds. 00960 * Bit 7:2 of CNFG_CHG_F (0x25) register. 00961 * 00962 * @param[in] currentmA 7.5mA, 15.0mA, 22.5mA, ... 00963 * 292.5mA, 300.0mA. 00964 * 00965 * @return 0 on success, error code on failure. 00966 */ 00967 int set_chg_cc_jeita(float currentmA); 00968 00969 /** 00970 * @brief Get IFAST-CHG-JEITA 00971 * when the battery is either cool or warm as defined by the 00972 * VCOOL and VWARM temperature thresholds. 00973 * Bit 7:2 of CNFG_CHG_F (0x25) register. 00974 * 00975 * @param[out] currentmA Pointer of value to be read. 00976 * 7.5mA, 15.0mA, 22.5mA, ... 00977 * 292.5mA, 300.0mA. 00978 * 00979 * @return 0 on success, error code on failure. 00980 */ 00981 int get_chg_cc_jeita(float *currentmA); 00982 00983 /** 00984 * @brief Register Configuration 00985 * 00986 * @details 00987 * - Register : CNFG_CHG_F (0x25) 00988 * - Bit Fields : [1] 00989 * - Default : 0x0 00990 * - Description : Thermistor Enable Bit 00991 */ 00992 typedef enum { 00993 THM_EN_DISABLED, 00994 THM_EN_ENABLED 00995 }decode_thm_en_t; 00996 00997 /** 00998 * @brief Set Thermistor Enable Bit. 00999 * Bit 1 of CNFG_CHG_F (0x25) register. 01000 * 01001 * @param[in] thm_en Thermistor Enable Bit to be written. 01002 * 01003 * @return 0 on success, error code on failure. 01004 */ 01005 int set_thm_en(decode_thm_en_t thm_en); 01006 01007 /** 01008 * @brief Get Thermistor Enable Bit. 01009 * Bit 1:0 of CNFG_CHG_F (0x25) register. 01010 * 01011 * @param[out] thm_en Thermistor Enable Bit field to be read. 01012 * 01013 * @return 0 on success, error code on failure. 01014 */ 01015 int get_thm_en(decode_thm_en_t *thm_en); 01016 01017 /** 01018 * @brief Set Fast-Charge Battery Regulation Voltage, VFAST-CHG. 01019 * Bit 7:2 of CNFG_CHG_G (0x26) register. 01020 * 01021 * @param[in] voltV 3.600V, 3.625V, 3.650V, ... 01022 * 4.575V, 4.600V. 01023 * 01024 * @return 0 on success, error code on failure. 01025 */ 01026 int set_chg_cv(float voltV); 01027 01028 /** 01029 * @brief Get Fast-Charge Battery Regulation Voltage, VFAST-CHG. 01030 * Bit 7:2 of CNFG_CHG_G (0x26) register. 01031 * 01032 * @param[out] voltV Pointer of value to be read. 01033 * 3.600V, 3.625V, 3.650V, ... 01034 * 4.575V, 4.600V. 01035 * 01036 * @return 0 on success, error code on failure. 01037 */ 01038 int get_chg_cv(float *voltV); 01039 01040 /** 01041 * @brief Register Configuration 01042 * 01043 * @details 01044 * - Register : CNFG_CHG_G (0x26) 01045 * - Bit Fields : [1] 01046 * - Default : 0x0 01047 * - Description : Setting this bit places CHGIN in USB suspend mode. 01048 */ 01049 typedef enum { 01050 USBS_CHGIN_NOT_SUSPENDED, 01051 USBS_CHGIN_SUSPENDED 01052 }decode_usbs_t; 01053 01054 /** 01055 * @brief Set USB Suspend Mode Bit. 01056 * Bit 1 of CNFG_CHG_G (0x26) register. 01057 * 01058 * @param[in] usbs CHGIN in USB suspend mode bit to be written. 01059 * 01060 * @return 0 on success, error code on failure. 01061 */ 01062 int set_usbs(decode_usbs_t usbs); 01063 01064 /** 01065 * @brief Get USB Suspend Mode Bit. 01066 * Bit 1:0 of CNFG_CHG_G (0x26) register. 01067 * 01068 * @param[out] usbs CHGIN in USB suspend mode bit field to be read. 01069 * 01070 * @return 0 on success, error code on failure. 01071 */ 01072 int get_usbs(decode_usbs_t *usbs); 01073 01074 /** 01075 * @brief Set the modified VFAST-CHG-JEITA for when the battery is either 01076 * cool or warm as defined by the VCOOL and VWARM temperature thresholds. 01077 * Bit 7:2 of CNFG_CHG_H (0x27) register. 01078 * 01079 * @param[in] voltV Pointer of value to be read. 01080 * 3.600V, 3.625V, 3.650V, ... 01081 * 4.575V, 4.600V. 01082 * 01083 * @return 0 on success, error code on failure. 01084 */ 01085 int set_chg_cv_jeita(float voltV); 01086 01087 /** 01088 * @brief Get the modified VFAST-CHG-JEITA for when the battery is either 01089 * cool or warm as defined by the VCOOL and VWARM temperature thresholds. 01090 * Bit 7:2 of CNFG_CHG_H (0x27) register. 01091 * 01092 * @param[out] voltV Pointer of value to be read. 01093 * 3.600V, 3.625V, 3.650V, ... 01094 * 4.575V, 4.600V. 01095 * 01096 * @return 0 on success, error code on failure. 01097 */ 01098 int get_chg_cv_jeita(float *voltV); 01099 01100 /** 01101 * @brief Set the Battery Discharge Current Full-Scale Current Value. 01102 * Bit 7:4 of CNFG_CHG_I (0x28) register. 01103 * 01104 * @param[in] currentmA 8.2mA, 40.5mA, 72.3mA, 103.4mA, 01105 * 134.1mA, 164.1mA, 193.7mA, 222.7mA, 01106 * 251.2mA, 279.3mA, 300.0mA 01107 * 01108 * @return 0 on success, error code on failure. 01109 */ 01110 int set_imon_dischg_scale(float currentmA); 01111 01112 /** 01113 * @brief Get the Battery Discharge Current Full-Scale Current Value. 01114 * Bit 7:4 of CNFG_CHG_I (0x28) register. 01115 * 01116 * @param[out] currentmA Pointer of value to be read. 01117 * 8.2mA, 40.5mA, 72.3mA, 103.4mA, 01118 * 134.1mA, 164.1mA, 193.7mA, 222.7mA, 01119 * 251.2mA, 279.3mA, 300.0mA 01120 * 01121 * @return 0 on success, error code on failure. 01122 */ 01123 int get_imon_dischg_scale(float *currentmA); 01124 01125 /** 01126 * @brief Register Configuration 01127 * 01128 * @details 01129 * - Register : CNFG_CHG_I (0x28) 01130 * - Bit Fields : [3:0] 01131 * - Default : 0x0 01132 * - Description : Analog channel to connect to AMUX. 01133 */ 01134 typedef enum { 01135 MUX_SEL_MULTIPLEXER_DISABLED, 01136 MUX_SEL_CHGIN_VOLTAGE_MONITOR, 01137 MUX_SEL_CHGIN_CURRENT_MONITOR, 01138 MUX_SEL_BATTERY_VOLTAGE_MONITOR, 01139 MUX_SEL_BATTERY_CHARGE_CURRENT_MONITOR, 01140 MUX_SEL_BATTERY_DISCHARGE_CURRENT_MONITOR_NORMAL, 01141 MUX_SEL_BATTERY_DISCHARGE_CURRENT_MONITOR_NULL, 01142 MUX_SEL_RESERVED_0x07, 01143 MUX_SEL_RESERVED_0x08, 01144 MUX_SEL_AGND_VOLTAGE_MONITOR, 01145 MUX_SEL_SYS_VOLTAGE_MONITOR, 01146 MUX_SEL_SYS_VOLTAGE_MONITOR_0x0B, 01147 MUX_SEL_SYS_VOLTAGE_MONITOR_0x0C, 01148 MUX_SEL_SYS_VOLTAGE_MONITOR_0x0D, 01149 MUX_SEL_SYS_VOLTAGE_MONITOR_0x0E, 01150 MUX_SEL_SYS_VOLTAGE_MONITOR_0x0F 01151 }decode_mux_sel_t; 01152 01153 /** 01154 * @brief Set the analog channel to connect to AMUX. 01155 * 01156 * @param[in] selection AMUX value field to be written. 01157 * 01158 * @return 0 on success, error code on failure. 01159 */ 01160 int set_mux_sel(decode_mux_sel_t selection); 01161 01162 /** 01163 * @brief Get the analog channel to connect to AMUX. 01164 * 01165 * @param[out] selection AMUX value field to be read. 01166 * 01167 * @return 0 on success, error code on failure. 01168 */ 01169 int get_mux_sel(decode_mux_sel_t *selection); 01170 01171 /** 01172 * @brief Set SIMO Buck-Boost Channel x Target Output Voltage. 01173 * CNFG_SBB0_A (0x29), CNFG_SBB1_A (0x2B) and CNFG_SBB2_A (0x2D) 01174 * 01175 * @param[in] channel Channel number: 0, 1 or 2. 01176 * @param[in] voltV SIMO buck-boost channel x target output voltage field to be written. 01177 * SBBx = 500mV + 25mV x TV_SBBx[7:0] 01178 * 0.500V, 0.525V, 0.550V, 0.575V, 0.600V, 0.625V, 01179 * 0.650V, 0.675V, 0.700V, ... 01180 * 5.425V, 5.450V, 5.475V, 5.500V. 01181 * 01182 * @return 0 on success, error code on failure. 01183 */ 01184 int set_tv_sbb(uint8_t channel, float voltV); 01185 01186 /** 01187 * @brief Get SIMO Buck-Boost Channel x Target Output Voltage. 01188 * CNFG_SBB0_A (0x29), CNFG_SBB1_A (0x2B) and CNFG_SBB2_A (0x2D) 01189 * 01190 * @param[in] channel Channel number: 0, 1 or 2. 01191 * @param[out] voltV SIMO buck-boost channel x target output voltage field to be read. 01192 * SBBx = 500mV + 25mV x TV_SBBx[7:0] 01193 * 0.500V, 0.525V, 0.550V, 0.575V, 0.600V, 0.625V, 01194 * 0.650V, 0.675V, 0.700V, ... 01195 * 5.425V, 5.450V, 5.475V, 5.500V. 01196 * 01197 * @return 0 on success, error code on failure. 01198 */ 01199 int get_tv_sbb(uint8_t channel, float *voltV); 01200 01201 /** 01202 * @brief Register Configuration 01203 * 01204 * @details 01205 * - Register : CNFG_SBB0_B (0x2A), CNFG_SBB1_B (0x2C) and CNFG_SBB2_B (0x2E) 01206 * - Bit Fields : [6] 01207 * - Default : 0x0 01208 * - Description : Operation mode of SBB0, 1 or 2. 01209 */ 01210 typedef enum { 01211 OP_MODE_BUCK_BOOST_MODE, 01212 OP_MODE_BUCK_MODE 01213 }decode_op_mode_t; 01214 01215 /** 01216 * @brief Set Operation mode of SBBx. 01217 * 01218 * @param[in] channel Channel number: 0, 1 or 2. 01219 * @param[in] mode Operation mode of SBBx bit to be written. 01220 * 01221 * @return 0 on success, error code on failure. 01222 */ 01223 int set_op_mode(uint8_t channel, decode_op_mode_t mode); 01224 01225 /** 01226 * @brief Get Operation mode of SBBx. 01227 * 01228 * @param[in] channel Channel number: 0, 1 or 2. 01229 * @param[out] mode Operation mode of SBBx bit to be read. 01230 * 01231 * @return 0 on success, error code on failure. 01232 */ 01233 int get_op_mode(uint8_t channel, decode_op_mode_t *mode); 01234 01235 /** 01236 * @brief Register Configuration 01237 * 01238 * @details 01239 * - Register : CNFG_SBB0_B (0x2A), CNFG_SBB1_B (0x2C) and CNFG_SBB2_B (0x2E) 01240 * - Bit Fields : [3] 01241 * - Default : 0x0 01242 * - Description : SIMO Buck-Boost Channel 0, 1 or 2 Active-Discharge Enable. 01243 */ 01244 typedef enum { 01245 ADE_SBB_DISABLED, 01246 ADE_SBB_ENABLED 01247 }decode_ade_sbb_t; 01248 01249 /** 01250 * @brief Set SIMO Buck-Boost Channel x Active-Discharge Enable. 01251 * 01252 * @param[in] channel Channel number: 0, 1 or 2. 01253 * @param[in] ade_sbb SIMO buck-boost channel 2 active-discharge enable bit to be written. 01254 * 01255 * @return 0 on success, error code on failure. 01256 */ 01257 int set_ade_sbb(uint8_t channel, decode_ade_sbb_t ade_sbb); 01258 01259 /** 01260 * @brief Get SIMO Buck-Boost Channel x Active-Discharge Enable. 01261 * 01262 * @param[in] channel Channel number: 0, 1 or 2. 01263 * @param[out] ade_sbb SIMO buck-boost channel 2 active-discharge enable bit to be read. 01264 * 01265 * @return 0 on success, error code on failure. 01266 */ 01267 int get_ade_sbb(uint8_t channel, decode_ade_sbb_t *ade_sbb); 01268 01269 /** 01270 * @brief Register Configuration 01271 * 01272 * @details 01273 * - Register : CNFG_SBB0_B (0x2A), CNFG_SBB1_B (0x2C) and CNFG_SBB2_B (0x2E) 01274 * - Bit Fields : [2:0] 01275 * - Default : 0x0 01276 * - Description : Enable Control for SIMO Buck-Boost Channel 0, 1 or 2. 01277 */ 01278 typedef enum { 01279 EN_SBB_FPS_SLOT_0, 01280 EN_SBB_FPS_SLOT_1, 01281 EN_SBB_FPS_SLOT_2, 01282 EN_SBB_FPS_SLOT_3, 01283 EN_SBB_OFF, 01284 EN_SBB_SAME_AS_0X04, 01285 EN_SBB_ON, 01286 EN_SBB_SAME_AS_0X06 01287 }decode_en_sbb_t; 01288 01289 /** 01290 * @brief Set Enable Control for SIMO Buck-Boost Channel x. 01291 * 01292 * @param[in] channel Channel number: 0, 1 or 2. 01293 * @param[in] en_sbb Enable control for SIMO buck-boost channel x field to be written. 01294 * 01295 * @return 0 on success, error code on failure. 01296 */ 01297 int set_en_sbb(uint8_t channel, decode_en_sbb_t en_sbb); 01298 01299 /** 01300 * @brief Get Enable Control for SIMO Buck-Boost Channel x. 01301 * 01302 * @param[in] channel Channel number: 0, 1 or 2. 01303 * @param[out] en_sbb Enable control for SIMO buck-boost channel x field to be read. 01304 * 01305 * @return 0 on success, error code on failure. 01306 */ 01307 int get_en_sbb(uint8_t channel, decode_en_sbb_t *en_sbb); 01308 01309 /** 01310 * @brief Register Configuration 01311 * 01312 * @details 01313 * - Register : CNFG_SBB_TOP (0x2F) 01314 * - Bit Fields : [7] 01315 * - Default : 0x0 01316 * - Description : Operation mode of the charging channel of SIMO 01317 */ 01318 typedef enum { 01319 OP_MODE_CHG_BUCK_BOOST, 01320 OP_MODE_CHG_BUCK 01321 }decode_op_mode_chg_t; 01322 01323 /** 01324 * @brief Set Operation mode of the charging channel of SIMO. 01325 * 01326 * @param[in] op_mode_chg Operation mode of the charging channel of SIMO bit to be written. 01327 * 01328 * @return 0 on success, error code on failure. 01329 */ 01330 int set_op_mode_chg(decode_op_mode_chg_t op_mode_chg); 01331 01332 /** 01333 * @brief Get Operation mode of the charging channel of SIMO. 01334 * 01335 * @param[out] op_mode_chg Operation mode of the charging channel of SIMO bit to be read. 01336 * 01337 * @return 0 on success, error code on failure. 01338 */ 01339 int get_op_mode_chg(decode_op_mode_chg_t *op_mode_chg); 01340 01341 /** 01342 * @brief Register Configuration 01343 * 01344 * @details 01345 * - Register : CNFG_SBB_TOP (0x2F) 01346 * - Bit Fields : [1:0] 01347 * - Default : 0x0 01348 * - Description : SIMO Buck-Boost (all channels) Drive Strength Trim. 01349 */ 01350 typedef enum { 01351 DRV_SBB_FASTEST_TRANSITION_TIME, 01352 DRV_SBB_A_LITTLE_SLOWER_THAN_0X00, 01353 DRV_SBB_A_LITTLE_SLOWER_THAN_0X01, 01354 DRV_SBB_A_LITTLE_SLOWER_THAN_0X02 01355 }decode_drv_sbb_t; 01356 01357 /** 01358 * @brief Set SIMO Buck-Boost (all channels) Drive Strength Trim. 01359 * 01360 * @param[in] drv_sbb SIMO buck-boost drive strength trim field to be written. 01361 * 01362 * @return 0 on success, error code on failure. 01363 */ 01364 int set_drv_sbb(decode_drv_sbb_t drv_sbb); 01365 01366 /** 01367 * @brief Get SIMO Buck-Boost (all channels) Drive Strength Trim. 01368 * 01369 * @param[out] drv_sbb SIMO buck-boost drive strength trim field to be read. 01370 * 01371 * @return 0 on success, error code on failure. 01372 */ 01373 int get_drv_sbb(decode_drv_sbb_t *drv_sbb); 01374 01375 /** 01376 * @brief Register Configuration 01377 * 01378 * @details 01379 * - Register : CNFG_SBB_TOP_B (0x30) 01380 * - Bit Fields : [7:6] 01381 * - Default : 0x0 01382 * - Description : SIMO Buck-Boost Charging Channel Peak Current Limit 01383 */ 01384 typedef enum { 01385 IP_CHG_AMP_2_000, 01386 IP_CHG_AMP_1_500, 01387 IP_CHG_AMP_1_000, 01388 IP_CHG_AMP_0_500 01389 }decode_ip_chg_t; 01390 01391 /** 01392 * @brief Set SIMO Buck-Boost Charging Channel Peak Current Limit. 01393 * 01394 * @param[in] ip_chg SIMO Buck-Boost Charging Channel Peak Current Limit field to be written. 01395 * 01396 * @return 0 on success, error code on failure. 01397 */ 01398 int set_ip_chg(decode_ip_chg_t ip_chg); 01399 01400 /** 01401 * @brief Get SIMO Buck-Boost Charging Channel Peak Current Limit. 01402 * 01403 * @param[out] ip_chg SIMO Buck-Boost Charging Channel Peak Current Limit field to be read. 01404 * 01405 * @return 0 on success, error code on failure. 01406 */ 01407 int get_ip_chg(decode_ip_chg_t *ip_chg); 01408 01409 /** 01410 * @brief Register Configuration 01411 * 01412 * @details 01413 * - Register : CNFG_SBB_TOP_B (0x30) 01414 * - Bit Fields : [5:4], [3:2] and [1:0] 01415 * - Default : 0x0 01416 * - Description : SIMO Buck-Boost Channel 0, 1 or 2 Peak Current Limit 01417 */ 01418 typedef enum { 01419 IP_SBB_AMP_1_000, 01420 IP_SBB_AMP_0_750, 01421 IP_SBB_AMP_0_500, 01422 IP_SBB_AMP_0_333 01423 }decode_ip_sbb_t; 01424 01425 /** 01426 * @brief Set SIMO Buck-Boost Channel 0, 1 or 2 Peak Current Limit. 01427 * 01428 * @param[in] channel Channel number: 0, 1 or 2. 01429 * @param[in] ip_sbb SIMO Buck-Boost Channel x Peak Current Limit field to be written. 01430 * 01431 * @return 0 on success, error code on failure. 01432 */ 01433 int set_ip_sbb(uint8_t channel, decode_ip_sbb_t ip_sbb); 01434 01435 /** 01436 * @brief Get SIMO Buck-Boost Channel 0, 1 or 2 Peak Current Limit. 01437 * 01438 * @param[in] channel Channel number: 0, 1 or 2. 01439 * @param[out] ip_sbb SIMO Buck-Boost Channel x Peak Current Limit field to be read. 01440 * 01441 * @return 0 on success, error code on failure. 01442 */ 01443 int get_ip_sbb(uint8_t channel, decode_ip_sbb_t *ip_sbb); 01444 01445 /** 01446 * @brief Register Configuration 01447 * 01448 * @details 01449 * - Register : CNFG_LDO0_A (0x38) 01450 * - Bit Fields : [7] 01451 * - Default : 0x0 01452 * - Description : LDO Output Voltage. This bit applies a 1.325V offset to the output voltage of the LDO. 01453 */ 01454 typedef enum { 01455 TV_LDO_NO_OFFSET, 01456 TV_LDO_NO_1_325V 01457 }decode_tv_ldo_offset_t; 01458 01459 /** 01460 * @brief Set LDO Output Channel 0 Target Output Voltage. Bit 7. 01461 * CNFG_LDO0_A (0x38) 01462 * 01463 * @param[in] offset LDO Output Channel 0 target output voltage offset field to be read. 01464 * 01465 * 01466 * @return 0 on success, error code on failure. 01467 */ 01468 int set_tv_ldo_offset(decode_tv_ldo_offset_t offset); 01469 01470 /** 01471 * @brief Get LDO Output Channel 0 Target Output Voltage. Bit 7. 01472 * CNFG_LDO0_A (0x38) 01473 * 01474 * @param[out] offset LDO Output Channel 0 target output voltage offset field to be read. 01475 * 01476 * @return 0 on success, error code on failure. 01477 */ 01478 int get_tv_ldo_offset(decode_tv_ldo_offset_t *offset); 01479 01480 /** 01481 * @brief Set LDO Output Channel 0 Target Output Voltage. Bit 6:0. 01482 * CNFG_LDO0_A (0x38) 01483 * 01484 * @param[in] voltV LDO Output Channel 0 target output voltage field to be read. 01485 * LDOx = 500mV + 25mV x TV_LDOx[6:0] 01486 * 0.500V, 0.525V, 0.550V, 0.575V, 0.600V, 0.625V, 01487 * 0.650V, 0.675V, 0.700V, ... 01488 * 3.650, 3.675. 01489 * 01490 * When TV_LDO[7] = 0, TV_LDO[6:0] sets the 01491 * LDO's output voltage range from 0.5V to 3.675V. 01492 * When TV_LDO[7] = 1, TV_LDO[6:0] sets the 01493 * LDO's output voltage from 1.825V to 5V. 01494 * 01495 * @return 0 on success, error code on failure. 01496 */ 01497 int set_tv_ldo_volt(float voltV); 01498 01499 /** 01500 * @brief Get LDO Output Channel 0 Target Output Voltage. Bit 6:0. 01501 * CNFG_LDO0_A (0x38) 01502 * 01503 * @param[out] voltV LDO Output Channel 0 target output voltage field to be read. 01504 * LDOx = 500mV + 25mV x TV_LDOx[6:0] 01505 * 0.500V, 0.525V, 0.550V, 0.575V, 0.600V, 0.625V, 01506 * 0.650V, 0.675V, 0.700V, ... 01507 * 3.650, 3.675. 01508 * 01509 * When TV_LDO[7] = 0, TV_LDO[6:0] sets the 01510 * LDO's output voltage range from 0.5V to 3.675V. 01511 * When TV_LDO[7] = 1, TV_LDO[6:0] sets the 01512 * LDO's output voltage from 1.825V to 5V. 01513 * 01514 * @return 0 on success, error code on failure. 01515 */ 01516 int get_tv_ldo_volt(float *voltV); 01517 01518 /** 01519 * @brief Register Configuration 01520 * 01521 * @details 01522 * - Register : CNFG_LDO0_B (0x39) 01523 * - Bit Fields : [2:0] 01524 * - Default : 0x0 01525 * - Description : Enable Control for LDO0. 01526 */ 01527 typedef enum { 01528 EN_LDO_FPS_SLOT_0, 01529 EN_LDO_FPS_SLOT_1, 01530 EN_LDO_FPS_SLOT_2, 01531 EN_LDO_FPS_SLOT_3, 01532 EN_LDO_OFF, 01533 EN_LDO_SAME_AS_0X04, 01534 EN_LDO_ON, 01535 EN_LDO_SAME_AS_0X06 01536 }decode_en_ldo_t; 01537 01538 /** 01539 * @brief Set Enable Control for LDO Channel0. 01540 * 01541 * @param[in] en_ldo Enable control for LDO channel x field to be written. 01542 * 01543 * @return 0 on success, error code on failure. 01544 */ 01545 int set_en_ldo(decode_en_ldo_t en_ldo); 01546 01547 /** 01548 * @brief Get Enable Control for LDO Channel x. 01549 * 01550 * @param[out] en_ldo Enable control for LDO channel x field to be read. 01551 * 01552 * @return 0 on success, error code on failure. 01553 */ 01554 int get_en_ldo(decode_en_ldo_t *en_ldo); 01555 01556 /** 01557 * @brief Register Configuration 01558 * 01559 * @details 01560 * - Register : CNFG_LDO0_B (0x39) 01561 * - Bit Fields : [3] 01562 * - Default : 0x0 01563 * - Description : LDO0 Active-Discharge Enable. 01564 */ 01565 typedef enum { 01566 ADE_LDO_DISABLED, 01567 ADE_LDO_ENABLED 01568 }decode_ade_ldo_t; 01569 01570 /** 01571 * @brief Set LDO0 Active-Discharge Enable. 01572 * 01573 * @param[in] ade_ldo LDO0 active-discharge enable bit to be written. 01574 * 01575 * @return 0 on success, error code on failure. 01576 */ 01577 int set_ade_ldo(decode_ade_ldo_t ade_ldo); 01578 01579 /** 01580 * @brief Get LDO0 Active-Discharge Enable. 01581 * 01582 * @param[out] ade_ldo LDO0 active-discharge enable bit to be read. 01583 * 01584 * @return 0 on success, error code on failure. 01585 */ 01586 int get_ade_ldo(decode_ade_ldo_t *ade_ldo); 01587 01588 /** 01589 * @brief Register Configuration 01590 * 01591 * @details 01592 * - Register : CNFG_LDO0_B (0x39) 01593 * - Bit Fields : [4] 01594 * - Default : 0x0 01595 * - Description : Operation mode of LDO0. 01596 */ 01597 typedef enum { 01598 LDO_MD_LDO_MODE, 01599 LDO_MD_LSW_MODE 01600 }decode_ldo_md_t; 01601 01602 /** 01603 * @brief Set Operation mode of LDOx. 01604 * 01605 * @param[in] mode Operation mode of LDOx bit to be written. 01606 * 01607 * @return 0 on success, error code on failure. 01608 */ 01609 int set_ldo_md(decode_ldo_md_t mode); 01610 01611 /** 01612 * @brief Get Operation mode of LDOx. 01613 * 01614 * @param[out] mode Operation mode of LDOx bit to be read. 01615 * 01616 * @return 0 on success, error code on failure. 01617 */ 01618 int get_ldo_md(decode_ldo_md_t *mode); 01619 01620 /** 01621 * @brief Disable all interrupts 01622 * 01623 * @return 0 on success, error code on failure 01624 */ 01625 int irq_disable_all(); 01626 01627 /** 01628 * @brief Set Interrupt Handler for a Specific Interrupt ID. 01629 * 01630 * @param[in] id Interrupt id, one of INTR_ID_*. 01631 * @param[in] func Interrupt handler function. 01632 * @param[in] cb Interrupt handler data. 01633 */ 01634 void set_interrupt_handler(reg_bit_int_glbl_t id, interrupt_handler_function func, void *cb); 01635 }; 01636 #endif /*_MAX77659_H_*/
Generated on Mon Aug 22 2022 16:08:46 by
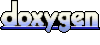