MAX7032 Transceiver Mbed Driver
Embed:
(wiki syntax)
Show/hide line numbers
Max7032.h
00001 /******************************************************************************* 00002 * Copyright(C) Maxim Integrated Products, Inc., All Rights Reserved. 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a 00005 * copy of this software and associated documentation files(the "Software"), 00006 * to deal in the Software without restriction, including without limitation 00007 * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 * and/or sell copies of the Software, and to permit persons to whom the 00009 * Software is furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included 00012 * in all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00015 * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00016 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00017 * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00018 * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00019 * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00020 * OTHER DEALINGS IN THE SOFTWARE. 00021 * 00022 * Except as contained in this notice, the name of Maxim Integrated 00023 * Products, Inc.shall not be used except as stated in the Maxim Integrated 00024 * Products, Inc.Branding Policy. 00025 * 00026 * The mere transfer of this software does not imply any licenses 00027 * of trade secrets, proprietary technology, copyrights, patents, 00028 * trademarks, maskwork rights, or any other form of intellectual 00029 * property whatsoever. Maxim Integrated Products, Inc.retains all 00030 * ownership rights. 00031 ******************************************************************************* 00032 */ 00033 00034 #ifndef MAX7032_MAX7032_H_ 00035 #define MAX7032_MAX7032_H_ 00036 00037 #include "mbed.h" 00038 #include "Max7032_regs.h" 00039 #include "rtos.h" 00040 00041 #define MAX7032_SPI MXC_SPIM2 00042 00043 #define MAX7032_SPI MXC_SPIM2 00044 #define MAX7032_SPI_MOSI P5_1 00045 #define MAX7032_SPI_MISO P5_2 00046 #define MAX7032_SPI_SCK P5_0 00047 #define MAX7032_SPI_CS P5_5 00048 00049 #define MAX7032_PIN_POWER P4_0 00050 #define MAX7032_PIN_RSSI AIN_1 00051 #define MAX7032_TRX_PIN P3_3 00052 00053 #define MAX7032_MBED_DATA_PIN P5_6 00054 #define MAX7032_GPIO_DATA_PORT PORT_5 00055 #define MAX7032_GPIO_DATA_PIN PIN_6 00056 00057 #define Q_START_CONF_LEN 17 00058 00059 class MAX7032 00060 { 00061 private: 00062 max7032_reg_map_t *reg_map; 00063 SPI *spi_handler; 00064 DigitalOut *ssel; 00065 DigitalOut *power_pin; 00066 DigitalOut *trx_pin; 00067 DigitalOut *data_send; 00068 PinName data_pin; 00069 DigitalOut *dio; 00070 00071 DigitalIn *data_read; 00072 00073 uint8_t preset_mode; 00074 float f_xtal ; 00075 float f_rf; 00076 float data_rate; 00077 float fsk_dev; 00078 const float fsk_dev_max = 0.1f; //100khz 00079 00080 protected: 00081 00082 00083 public: 00084 00085 //Constructors 00086 MAX7032(SPI *spi, DigitalOut *cs, DigitalOut *powerPin, PinName dataPin, DigitalOut *dioPin, DigitalOut *trxPin); 00087 00088 MAX7032(SPI *spi, DigitalOut *powerPin, PinName dataPin, DigitalOut *dioPin, DigitalOut *trxPin); 00089 00090 //Destructor 00091 ~MAX7032(); 00092 00093 typedef enum { 00094 POWER_ADDR = 0x00, 00095 CONTRL_ADDR = 0x01, 00096 CONF0_ADDR = 0x02, 00097 CONF1_ADDR = 0x03, 00098 OSC_ADDR = 0x05, 00099 TOFFMSB_ADDR = 0x06, 00100 TOFFLSB_ADDR = 0x07, 00101 TCPU_ADDR = 0x08, 00102 TRFMSB_ADDR = 0x09, 00103 TRFLSB_ADDR = 0x0A, 00104 TONMSB_ADDR = 0x0B, 00105 TONLSB_ADDR = 0x0C, 00106 TXLOWMSB_ADDR = 0x0D, 00107 TXLOWLSB_ADDR = 0x0E, 00108 TXHIGHMSB_ADDR = 0x0F, 00109 TXHIGHLSB_ADDR = 0x10, 00110 STATUS_ADDR = 0x1A, 00111 }register_address_t; 00112 00113 typedef enum { 00114 X0, 00115 RSSIO, 00116 PA, 00117 PkDet, 00118 BaseB, 00119 MIXER, 00120 AGC, 00121 LNA, 00122 SLEEP, 00123 CKOUT, 00124 FCAL, 00125 PCAL, 00126 X1, 00127 TRK_EN, 00128 GAIN, 00129 AGCLK, 00130 ONPS0, 00131 ONPS1, 00132 OFPS0, 00133 OFPS1, 00134 DRX, 00135 MGAIN, 00136 T_R, 00137 MODE, 00138 DT0, 00139 DT1, 00140 DT2, 00141 CDIV0, 00142 CDIV1, 00143 CLKOF, 00144 ACAL, 00145 X2, 00146 NUM_OF_BIT 00147 }reg_bits_t; 00148 00149 typedef enum 00150 { 00151 Manchester = 0, 00152 NRZ = 1 00153 }encoding_t; 00154 00155 encoding_t encoding; 00156 00157 /** 00158 * @brief Operation state of the RF receiver 00159 * 00160 * @details 00161 * - Default : 0x00 00162 * - Description : Indicates whether initialization is successful 00163 */ 00164 typedef enum { 00165 INITIALIZED = 0, 00166 UNINITIALIZED = 1, 00167 UNKNOWN = 2, 00168 } operation_mode_t; 00169 00170 operation_mode_t operation_mode; 00171 00172 /** 00173 * @brief Register Configuration 00174 * 00175 * @details 00176 * - Register : CONF0 (0x02) 00177 * - Bit Fields : [7] 00178 * - Default : 0x0 00179 * - Description : ASK/FSK Selection for both receive and transmit 00180 */ 00181 typedef enum { 00182 ASK_FSK_SEL_ASK, /**< 0x0: ASK modulation */ 00183 ASK_FSK_SEL_FSK /**< 0x1: FSK modulation */ 00184 } ask_fsk_sel_t; 00185 00186 00187 /* REGISTER SET & GET FUNCTION DECLARATIONS */ 00188 00189 00190 /** 00191 * @brief Sets the specified bit. 00192 * 00193 * @param[in] bit_field Bit to be set. 00194 * @param[in] val Value to be set. 00195 * 00196 * @returns 0 on success, negative error code on failure. 00197 */ 00198 int set_bit_field(reg_bits_t bit_field, uint8_t val); 00199 00200 /** 00201 * @brief Gets the specified bit. 00202 * 00203 * @param[in] bit_field Bit to get value. 00204 * @param[in] val The address to store the value of the Bit. 00205 * 00206 * @returns 0 on success, negative error code on failure. 00207 */ 00208 int get_bit_field(reg_bits_t bit_field, uint8_t *val); 00209 00210 /** 00211 * @brief Sets Low-Noise Amplifier Power-Configuration Bit. 00212 * 00213 * @param[in] lna 0x0: Disable, 0x1: Enable. 00214 * 00215 * @returns 0 on success, negative error code on failure. 00216 */ 00217 int set_lna(uint8_t lna); 00218 00219 /** 00220 * @brief Gets Low-Noise Amplifier Power-Configuration Bit. 00221 * 00222 * @returns LNA Power-Configuration Bit's State, negative error code on failure. 00223 */ 00224 int get_lna(); 00225 00226 /** 00227 * @brief Sets Automatic Gain Control Power-Configuration Bit. 00228 * 00229 * @param[in] agc 0x0: Disable, 0x1: Enable. 00230 * 00231 * @returns 0 on success, negative error code on failure. 00232 */ 00233 int set_agc(uint8_t agc); 00234 00235 /** 00236 * @brief Gets Automatic Gain Control Power-Configuration Bit. 00237 * 00238 * @returns AGC Power-Configuration Bit's State, negative error code on failure. 00239 */ 00240 int get_agc(); 00241 00242 /** 00243 * @brief Sets Mixer Power-Configuration Bit. 00244 * 00245 * @param[in] mixer 0x0: Disable, 0x1: Enable. 00246 * 00247 * @returns 0 on success, negative error code on failure. 00248 */ 00249 int set_mixer(uint8_t mixer); 00250 00251 /** 00252 * @brief Gets Mixer Power-Configuration Bit. 00253 * 00254 * @returns Mixer Power-Configuration Bit's State, negative error code on failure. 00255 */ 00256 int get_mixer(); 00257 00258 /** 00259 * @brief Sets Baseband Power-Configuration Bit. 00260 * 00261 * @param[in] baseb 0x0: Disable, 0x1: Enable. 00262 * 00263 * @returns 0 on success, negative error code on failure. 00264 */ 00265 int set_baseb(uint8_t baseb); 00266 00267 /** 00268 * @brief Gets Baseband Power-Configuration Bit. 00269 * 00270 * @returns Baseband Power-Configuration Bit's State, negative error code on failure. 00271 */ 00272 int get_baseb(); 00273 00274 /** 00275 * @brief Sets Peak-detector Power-Configuration Bit. 00276 * 00277 * @param[in] pkdet 0x0: Disable, 0x1: Enable. 00278 * 00279 * @returns 0 on success, negative error code on failure. 00280 */ 00281 int set_pkdet(uint8_t pkdet); 00282 00283 /** 00284 * @brief Gets Peak-detector Power-Configuration Bit. 00285 * 00286 * @returns Peak-detector Power-Configuration Bit's State, negative error code on failure. 00287 */ 00288 int get_pkdet(); 00289 00290 /** 00291 * @brief Sets Transmitter Power Amplifier Power-Configuration Bit. 00292 * 00293 * @param[in] pa 0x0: Disable, 0x1: Enable. 00294 * 00295 * @returns 0 on success, negative error code on failure. 00296 */ 00297 int set_pa(uint8_t pa); 00298 00299 /** 00300 * @brief Gets Transmitter Power Amplifier Power-Configuration Bit. 00301 * 00302 * @returns Transmitter Power Amplifier Power-Configuration Bit's State, negative error code on failure. 00303 */ 00304 int get_pa(); 00305 00306 /** 00307 * @brief Sets RSSI Amplifier Power-Configuration Bit. 00308 * 00309 * @param[in] rssio 0x0: Disable, 0x1: Enable. 00310 * 00311 * @returns 0 on success, negative error code on failure. 00312 */ 00313 int set_rssio(uint8_t rssio); 00314 00315 /** 00316 * @brief Gets RSSI Amplifier Power-Configuration Bit. 00317 * 00318 * @returns RSSI Amplifier Power-Configuration Bit's State, negative error code on failure. 00319 */ 00320 int get_rssio(); 00321 00322 /** 00323 * @brief Sets AGC Locking Feature Control Bit. 00324 * 00325 * @param[in] agclk 0x0: Disable, 0x1: Enable. 00326 * 00327 * @returns 0 on success, negative error code on failure. 00328 */ 00329 int set_agclk(uint8_t agclk); 00330 00331 /** 00332 * @brief Gets AGC Locking Feature Control Bit. 00333 * 00334 * @returns AGC Locking Feature Control Bit's State, negative error code on failure. 00335 */ 00336 int get_agclk(); 00337 00338 /** 00339 * @brief Sets Gain State Control Bit. 00340 * 00341 * @param[in] gain 0x0: Force manual low-gain state if MGAIN = 1, 00342 * 0x1: Force manual high-gain state if MGAIN = 1. 00343 * 00344 * @returns 0 on success, negative error code on failure. 00345 */ 00346 int set_gain(uint8_t gain); 00347 00348 /** 00349 * @brief Gets Gain State Control Bit. 00350 * 00351 * @returns Gain State Control Bit's State, negative error code on failure. 00352 */ 00353 int get_gain(); 00354 00355 /** 00356 * @brief Sets Manual Peak-Detector Tracking Control Bit. 00357 * 00358 * @param[in] trk_en 0x0: Release peak-detector tracking, 00359 * 0x1: Force manual peak-detector tracking. 00360 * 00361 * @returns 0 on success, negative error code on failure. 00362 */ 00363 int set_trk_en(uint8_t trk_en); 00364 00365 /** 00366 * @brief Gets Manual Peak-Detector Tracking Control Bit. 00367 * 00368 * @returns Manual Peak-Detector Tracking Control Bit's State, negative error code on failure. 00369 */ 00370 int get_trk_en(); 00371 00372 /** 00373 * @brief Sets Polling Timer Calibration Control Bit. 00374 * 00375 * @description Sets PCAL Bit to 1 to Perform polling timer calibration. 00376 * Automatically reset to zero once calibration is completed 00377 * 00378 * @returns 0 on success, negative error code on failure. 00379 */ 00380 int set_pcal(); 00381 00382 /** 00383 * @brief Gets Polling Timer Calibration Control Bit. 00384 * 00385 * @returns Polling Timer Calibration Control Bit's State, negative error code on failure. 00386 */ 00387 int get_pcal(); 00388 00389 /** 00390 * @brief Sets FSK calibration Control Bit. 00391 * 00392 * @description Sets FCAL Bit to 1 to Perform FSK calibration. 00393 * Automatically reset to zero once calibration is completed 00394 * 00395 * @returns 0 on success, negative error code on failure. 00396 */ 00397 int set_fcal(); 00398 00399 /** 00400 * @brief Gets FSK calibration Control Bit. 00401 * 00402 * @returns FSK calibration Control Bit's State, negative error code on failure. 00403 */ 00404 int get_fcal(); 00405 00406 /** 00407 * @brief Sets Crystal Clock Output Enable Control Bit. 00408 * 00409 * @param[in] sleep 0x0: Disable crystal clock output, 00410 * 0x1: Enable crystal clock output. 00411 * 00412 * @returns 0 on success, negative error code on failure. 00413 */ 00414 int set_ckout(uint8_t ckout); 00415 00416 /** 00417 * @brief Gets Crystal Clock Output Enable Control Bit. 00418 * 00419 * @returns Sleep Mode Control Bit's State, negative error code on failure. 00420 */ 00421 int get_ckout(); 00422 00423 /** 00424 * @brief Sets Sleep Mode Control Bit. 00425 * 00426 * @param[in] sleep 0x0: Normal operation, 00427 * 0x1: Deep-sleep mode, regardless the state of ENABLE pin. 00428 * 00429 * @returns 0 on success, negative error code on failure. 00430 */ 00431 int set_sleep(uint8_t sleep); 00432 00433 /** 00434 * @brief Gets Sleep Mode Control Bit. 00435 * 00436 * @returns Sleep Mode Control Bit's State, negative error code on failure. 00437 */ 00438 int get_sleep(); 00439 00440 /** 00441 * @brief Sets FSK or ASK modulation Configuration Bit. 00442 * 00443 * @param[in] ask_fsk_sel 0x0: Enable ASK for both receive and transmit, 00444 * 0x1: Enable FSK for both receive and transmit. 00445 * 00446 * @returns 0 on success, negative error code on failure. 00447 */ 00448 int set_mode(ask_fsk_sel_t ask_fsk_sel); 00449 00450 /** 00451 * @brief Gets FSK or ASK modulation Configuration Bit. 00452 * 00453 * @param[in] ask_fsk_sel 00454 * 00455 * @returns 0 on success, negative error code on failure. 00456 */ 00457 int get_mode(ask_fsk_sel_t* ask_fsk_sel); 00458 00459 /** 00460 * @brief Gets FSK or ASK modulation Configuration Bit. 00461 * 00462 * @returns FSK or ASK modulation Configuration Bit's State, negative error code on failure. 00463 */ 00464 int get_mode(); 00465 00466 /** 00467 * @brief Sets Transmit or Receive Configuration Bit. 00468 * 00469 * @param[in] t_r 0x0: Enable receive mode of the transceiver when pin T/R = 0, 00470 * 0x1: Enable transmit mode of the transceiver, regardless the state of pin T/R. 00471 * 00472 * @returns 0 on success, negative error code on failure. 00473 */ 00474 int set_t_r(uint8_t t_r); 00475 00476 /** 00477 * @brief Gets Transmit or Receive Configuration Bit. 00478 * 00479 * @returns Transmit or Receive Configuration Bit's State, negative error code on failure. 00480 */ 00481 int get_t_r(); 00482 00483 /** 00484 * @brief Sets Manual Gain Mode Configuration Bit. 00485 * 00486 * @param[in] mgain 0x0: Disable manual-gain mode, 00487 * 0x1: Enable manual-gain mode. 00488 * 00489 * @returns 0 on success, negative error code on failure. 00490 */ 00491 int set_mgain(uint8_t mgain); 00492 00493 /** 00494 * @brief Gets Manual Gain Mode Configuration Bit. 00495 * 00496 * @returns Manual Gain Mode Configuration Bit's State, negative error code on failure. 00497 */ 00498 int get_mgain(); 00499 00500 /** 00501 * @brief Sets Discontinuous Receive Mode Configuration Bit. 00502 * 00503 * @param[in] drx 0x0: Disable DRX, 00504 * 0x1: Enable DRX. 00505 * 00506 * @returns 0 on success, negative error code on failure. 00507 */ 00508 int set_drx(uint8_t drx); 00509 00510 /** 00511 * @brief Gets Discontinuous Receive Mode Configuration Bit. 00512 * 00513 * @returns Discontinuous Receive Mode Configuration Bit's State, negative error code on failure. 00514 */ 00515 int get_drx(); 00516 00517 /** 00518 * @brief Sets Off-timer Prescaler Configuration Bits. 00519 * 00520 * @param[in] ofps Off-timer Prescaler Configuration Value. 00521 * 00522 * @returns 0 on success, negative error code on failure. 00523 */ 00524 int set_ofps(uint8_t ofps); 00525 00526 /** 00527 * @brief Gets Off-timer Prescaler Configuration Bits. 00528 * 00529 * @returns Off-timer Prescaler Configuration Bit's value, negative error code on failure. 00530 */ 00531 int get_ofps(); 00532 00533 /** 00534 * @brief Sets On-timer Prescaler Configuration Bits. 00535 * 00536 * @param[in] onps On-timer Prescaler Configuration Value. 00537 * 00538 * @returns 0 on success, negative error code on failure. 00539 */ 00540 int set_onps(uint8_t onps); 00541 00542 /** 00543 * @brief Gets On-timer Prescaler Configuration Bits. 00544 * 00545 * @returns On-timer Prescaler Configuration Bit's value, negative error code on failure. 00546 */ 00547 int get_onps(); 00548 00549 /** 00550 * @brief Sets Automatic FSK Calibration Configuration Bit. 00551 * 00552 * @param[in] acal 0x0: Disable automatic FSK calibration, 00553 * 0x1: Enable automatic FSK calibration when coming out of the sleep state in DRX mode. 00554 * 00555 * @returns 0 on success, negative error code on failure. 00556 */ 00557 int set_acal(uint8_t acal); 00558 00559 /** 00560 * @brief Gets Automatic FSK Calibration Configuration Bit. 00561 * 00562 * @returns Automatic FSK Calibration Configuration Bit's State, negative error code on failure. 00563 */ 00564 int get_acal(); 00565 00566 /** 00567 * @brief Sets Continuous Clock Output (even during tOFF or when ENABLE pin is low) Configuration Bit. 00568 * 00569 * @param[in] clkof 0x0: Continuous clock output; if CKOUT = 1, clock output is active during tON (DRX mode) or 00570 * when ENABLE pin is high (continuous receive mode), 00571 * 0x1: Enable continuous clock output when CKOUT = 1. 00572 * 00573 * @returns 0 on success, negative error code on failure. 00574 */ 00575 int set_clkof(uint8_t clkof); 00576 00577 /** 00578 * @brief Gets Continuous Clock Output Configuration Bit. 00579 * 00580 * @returns Continuous Clock Output Configuration Bit's State, negative error code on failure. 00581 */ 00582 int get_clkof(); 00583 00584 /** 00585 * @brief Sets Crystal Divider Configuration Bits. 00586 * 00587 * @param[in] cdiv Crystal Divider Configuration Value. 00588 * 00589 * @returns 0 on success, negative error code on failure. 00590 */ 00591 int set_cdiv(uint8_t cdiv); 00592 00593 /** 00594 * @brief Gets Crystal Divider Configuration Bits Value. 00595 * 00596 * @returns Crystal Divider Configuration Bit's value, negative error code on failure. 00597 */ 00598 int get_cdiv(); 00599 00600 /** 00601 * @brief Sets AGC Dwell Timer Configuration Bits. 00602 * 00603 * @param[in] dt AGC Dwell Timer Configuration Value. 00604 * 00605 * @returns 0 on success, negative error code on failure. 00606 */ 00607 int set_dt(uint8_t dt); 00608 00609 /** 00610 * @brief Gets AGC Dwell Timer Configuration Bits Value. 00611 * 00612 * @returns AGC Dwell Timer Configuration Bit's value, negative error code on failure. 00613 */ 00614 int get_dt(); 00615 00616 /** 00617 * @brief Sets the OSC Register. 00618 * 00619 * @param[in] osc OSC Register Value. 00620 * 00621 * @description This register must be set to the integer result of fXTAL/100kHz. 00622 * 00623 * @returns 0 on success, negative error code on failure. 00624 */ 00625 int set_osc(uint8_t osc); 00626 00627 /** 00628 * @brief Gets the OSC Register Value. 00629 * 00630 * @returns OSC Register Value, negative error code on failure. 00631 */ 00632 int get_osc(); 00633 00634 /** 00635 * @brief Sets the tOFF Registers. 00636 * 00637 * @param[in] toff OSC Registers Value. 00638 * 00639 * @returns 0 on success, negative error code on failure. 00640 */ 00641 int set_toff(uint16_t toff); 00642 00643 /** 00644 * @brief Gets the tOFF Registers Value. 00645 * 00646 * @returns tOFF Registers Value, negative error code on failure. 00647 */ 00648 uint16_t get_toff(); 00649 00650 /** 00651 * @brief Sets the tCPU Register. 00652 * 00653 * @param[in] tcpu tCPU Register Value. 00654 * 00655 * @returns 0 on success, negative error code on failure. 00656 */ 00657 int set_tcpu(uint8_t tcpu); 00658 00659 /** 00660 * @brief Gets the tCPU Register Value. 00661 * 00662 * @returns tCPU Register Value, negative error code on failure. 00663 */ 00664 uint8_t get_tcpu(); 00665 00666 /** 00667 * @brief Sets the tRF Registers. 00668 * 00669 * @param[in] trf tRF Registers Value. 00670 * 00671 * @returns 0 on success, negative error code on failure. 00672 */ 00673 int set_trf(uint16_t trf); 00674 00675 /** 00676 * @brief Gets the tRF Registers Value. 00677 * 00678 * @returns tRF Registers Value, negative error code on failure. 00679 */ 00680 uint16_t get_trf(); 00681 00682 /** 00683 * @brief Sets the tON Registers. 00684 * 00685 * @param[in] ton tON Registers Value. 00686 * 00687 * @returns 0 on success, negative error code on failure. 00688 */ 00689 int set_ton(uint16_t ton); 00690 00691 /** 00692 * @brief Gets the tON Registers Value. 00693 * 00694 * @returns tON Registers Value, negative error code on failure. 00695 */ 00696 uint16_t get_ton(); 00697 00698 /** 00699 * @brief Sets the TxLOW Registers. 00700 * 00701 * @param[in] txlow_val TxLOW Registers Value. 00702 * 00703 * @returns 0 on success, negative error code on failure. 00704 */ 00705 int set_txlow(int txlow_val); 00706 00707 /** 00708 * @brief Gets the TxLOW Registers Value. 00709 * 00710 * @returns the TxLOW Registers Value, negative error code on failure. 00711 */ 00712 int get_txlow(); 00713 00714 /** 00715 * @brief Sets the TxHIGH Registers. 00716 * 00717 * @param[in] txhigh_val TxHIGH Registers Value. 00718 * 00719 * @returns 0 on success, negative error code on failure. 00720 */ 00721 int set_txhigh(int txhigh_val); 00722 00723 /** 00724 * @brief Gets the TxHIGH Registers Value. 00725 * 00726 * @returns the TxHIGH Registers Value, negative error code on failure. 00727 */ 00728 int get_txhigh(); 00729 00730 /** 00731 * @brief Gets Lock Detect Status Bit. 00732 * 00733 * @returns Lock Detect Status Bit's State, negative error code on failure. 00734 */ 00735 int get_lckd(); 00736 00737 /** 00738 * @brief Gets AGC Gain State Status Bit. 00739 * 00740 * @returns AGC Gain State Status Bit's State, negative error code on failure. 00741 */ 00742 int get_gains(); 00743 00744 /** 00745 * @brief Gets Clock/Crystal Alive Status Bit. 00746 * 00747 * @returns Clock/Crystal Alive Status Bit's State, negative error code on failure. 00748 */ 00749 int get_clkon(); 00750 00751 /** 00752 * @brief Gets Polling Timer Calibration Done Status Bit. 00753 * 00754 * @returns Polling Timer Calibration Done Status Bit's State, negative error code on failure. 00755 */ 00756 int get_pcald(); 00757 00758 /** 00759 * @brief Gets FSK Calibration Done Status Bit. 00760 * 00761 * @returns FSK Calibration Done Status Bit's State, negative error code on failure. 00762 */ 00763 int get_fcald(); 00764 00765 /* END OF REGISTER SET & GET FUNCTION DECLARATIONS */ 00766 00767 /* DEVICE CONFIGURATION FUNCTION DECLARATIONS */ 00768 typedef enum { 00769 DISABLE, /**< 0x0: Disable */ 00770 F_XTAL, /**< 0x1: fXTAL */ 00771 F_XTAL_X0_5, /**< 0x2: fXTAL/2 */ 00772 F_XTAL_X0_25, /**< 0x3: fXTAL/4 */ 00773 F_XTAL_X0_125 /**< 0x4: fXTAL/8 */ 00774 } cdiv_t; 00775 00776 /** 00777 * @brief Configures Crystal Clock Output. 00778 * 00779 * @param[in] cdiv Clock Output Configuration. 00780 * 00781 * @returns 0 on success, negative error code on failure. 00782 */ 00783 int adjust_clockout(cdiv_t cdiv); 00784 00785 /** 00786 * @brief Gets Clock Output Configuration. 00787 * 00788 * @param[in] cdiv Clock Output Configuration. 00789 * 00790 * @returns 0 on success, negative error code on failure. 00791 */ 00792 int get_clockout_conf(cdiv_t *cdiv); 00793 00794 /** 00795 * @brief Configures Internal Clock Frequency Divisor. 00796 * 00797 * @param[in] osc_freq Oscillator Frequency. 00798 * 00799 * @returns 0 on success, negative error code on failure. 00800 */ 00801 int adjust_osc_freq(float osc_freq); 00802 00803 /** 00804 * @brief Gets Oscillator Frequency. 00805 * 00806 * @returns Oscillator Frequency. 00807 */ 00808 float get_osc_freq(); 00809 00810 typedef enum { 00811 US_120, /**< 0x0: 120 us */ 00812 US_480, /**< 0x1: 480 us */ 00813 US_1920, /**< 0x2: 1920 us */ 00814 US_7680 /**< 0x3: 7680 us */ 00815 } timer_base_t; 00816 00817 /** 00818 * @brief Configures the off timer. 00819 * 00820 * @param[in] time_base Timer Base Configuration. 00821 * @param[in] timer_val tOFF Registers Value. 00822 * 00823 * @returns 0 on success, negative error code on failure. 00824 */ 00825 int adjust_off_timer(timer_base_t time_base, int timer_val); 00826 00827 /** 00828 * @brief Configures the off timer. 00829 * 00830 * @param[in] toff_time Off Time in microseconds. 00831 * 00832 * @returns 0 on success, negative error code on failure. 00833 */ 00834 int adjust_off_timer(int toff_time); 00835 00836 /** 00837 * @brief Gets the off time in us. 00838 * 00839 * @returns the off time in us, negative error code on failure. 00840 */ 00841 int get_off_timer(); 00842 00843 /** 00844 * @brief Configures the CPU recovery timer. 00845 * 00846 * @param[in] tcpu_time desired CPU recovery time in us. 00847 * 00848 * @returns 0 on success, negative error code on failure. 00849 */ 00850 int adjust_cpu_recovery_timer(int tcpu_time); 00851 00852 /** 00853 * @brief Gets the CPU recovery time in us. 00854 * 00855 * @returns the CPU recovery time in us, negative error code on failure. 00856 */ 00857 int get_cpu_recovery_timer(); 00858 00859 /** 00860 * @brief Configures the RF settling timer. 00861 * 00862 * @param[in] trf_time RF settling time in us. 00863 * 00864 * @returns 0 on success, negative error code on failure. 00865 */ 00866 int adjust_rf_settling_timer(int trf_time); 00867 00868 /** 00869 * @brief Gets the RF settling time in us. 00870 * 00871 * @returns the RF settling time in us, negative error code on failure. 00872 */ 00873 int get_rf_settling_timer(); 00874 00875 /** 00876 * @brief Configures the on timer. 00877 * 00878 * @param[in] time_base Timer Base Configuration. 00879 * @param[in] timer_val tON Registers Value. 00880 * 00881 * @returns 0 on success, negative error code on failure. 00882 */ 00883 int adjust_on_timer(timer_base_t time_base, int timer_val); 00884 00885 /** 00886 * @brief Configures the on timer. 00887 * 00888 * @param[in] ton_time On Time in microseconds. 00889 * 00890 * @returns 0 on success, negative error code on failure. 00891 */ 00892 int adjust_on_timer(int ton_time); 00893 00894 /** 00895 * @brief Gets the on time in us. 00896 * 00897 * @returns the on time in us, negative error code on failure. 00898 */ 00899 int get_on_timer(); 00900 00901 /** 00902 * @brief Sets the devices center frequency. 00903 * 00904 * @param[in] center_freq desired center frequency 00905 * 00906 * @returns 0 on success, negative error code on failure. 00907 */ 00908 int set_center_freq(float center_freq); 00909 00910 /** 00911 * @brief Gets the devices center frequency. 00912 * 00913 * @returns 0 on success, negative error code on failure. 00914 */ 00915 float get_center_freq(); 00916 00917 /** 00918 * @brief Sets the devices data rate. 00919 * 00920 * @param[in] data_rate_set desired data rate 00921 * 00922 * @returns 0 on success, negative error code on failure. 00923 */ 00924 int set_data_rate(float data_rate_set); 00925 00926 /** 00927 * @brief Gets the devices data rate. 00928 * 00929 * @returns 0 on success, negative error code on failure. 00930 */ 00931 float get_data_rate(); 00932 00933 /** 00934 * @brief Sets the FSK deviation. 00935 * 00936 * @param[in] fsk_dev_set desired FSK deviation 00937 * 00938 * @returns 0 on success, negative error code on failure. 00939 */ 00940 int set_fsk_dev(float fsk_dev_set); 00941 00942 /** 00943 * @brief Gets the FSK deviation. 00944 * 00945 * @returns 0 on success, negative error code on failure. 00946 */ 00947 float get_fsk_dev(); 00948 00949 /** 00950 * @brief Sets the encoding type. 00951 * 00952 * @param[in] encoding_set desired encoding type 00953 * 00954 * @returns 0 on success, negative error code on failure. 00955 */ 00956 int set_encoding(encoding_t encoding_set); 00957 00958 /** 00959 * @brief Gets the encoding type. 00960 * 00961 * @returns 0 on success, negative error code on failure. 00962 */ 00963 int get_encoding(); 00964 00965 /** 00966 * @brief Configures the AGC Dwell Timer. 00967 * 00968 * @param[in] k_val K value for dwell timer configuration. 00969 * 00970 * @returns 0 on success, negative error code on failure. 00971 */ 00972 int adjust_agc_dwell_timer(uint8_t k_val); 00973 00974 /** 00975 * @brief Configures the AGC Dwell Timer. 00976 * 00977 * @param[in] dwell_time desired dwell time. 00978 * 00979 * @returns 0 on success, negative error code on failure. 00980 */ 00981 int adjust_agc_dwell_timer(int dwell_time); 00982 00983 /** 00984 * @brief Gets the AGC Dwell Time Value. 00985 * 00986 * @returns the AGC Dwell Time Value, negative error code on failure. 00987 */ 00988 int get_agc_dwell_timer(); 00989 00990 /* END OF DEVICE CONFIGURATION FUNCTION DECLARATIONS */ 00991 00992 /* PUBLIC FUNCTION DECLARATIONS */ 00993 00994 /** 00995 * @brief Set power on/off 00996 * 00997 * @param[in] power 0 : power ON, 1: power OFF 00998 * 00999 * @returns 0 on success, negative error code on failure. 01000 */ 01001 int set_power_on_off(uint8_t power); 01002 01003 /** 01004 * @brief Read from a register. Since 3-wire spi is not supported by mbed, the read_register function must be implemented by the user. 01005 * 01006 * @param[in] reg Address of a register to be read. 01007 * @param[out] value Pointer to save result value. 01008 * 01009 * @returns 0 on success, negative error code on failure. 01010 */ 01011 int read_register(uint8_t reg, uint8_t *value); 01012 01013 /** 01014 * @brief Write to a register. 01015 * 01016 * @param[in] reg Address of a register to be written. 01017 * @param[out] value Pointer of value to be written to register. 01018 * @param[in] len Size of result to be written. 01019 * 01020 * @returns 0 on success, negative error code on failure. 01021 */ 01022 int write_register(uint8_t reg, const uint8_t *value, uint8_t len); 01023 01024 /** 01025 * @brief Initial programming steps after power on or soft reset. 01026 * 01027 * @returns 0 on success, negative error code on failure. 01028 */ 01029 int initial_programming(void); 01030 01031 /** 01032 * @brief Loads the Quick Start configuration to the device for RX mode. 01033 * 01034 * @returns 0 on success, negative error code on failure. 01035 */ 01036 int rx_quich_start(void); 01037 01038 /** 01039 * @brief Loads the Quick Start configuration to the device for TX mode. 01040 * 01041 * @returns 0 on success, negative error code on failure. 01042 */ 01043 int tx_quich_start(void); 01044 01045 typedef enum{ 01046 RECEIVE_MODE, 01047 TRANSMIT_MODE 01048 }trx_state_t; 01049 01050 /** 01051 * @brief Sets the Device to Receiver or Transmitter Mode. 01052 * 01053 * @param[in] trx_state desired mode. 01054 * 01055 * @returns 0 on success, negative error code on failure. 01056 */ 01057 int set_trx_state(trx_state_t trx_state); 01058 01059 /** 01060 * @brief Gets the Mode the Device is in. 01061 * 01062 * @returns the mode the Device is in, negative error code on failure. 01063 */ 01064 int get_trx_state(); 01065 01066 /** 01067 * @brief Transmits the RF data. 01068 * 01069 * @param[in] data Address of the data to be transmit. 01070 * @param[in] data_len Size of the data to be transmit. 01071 * 01072 * @returns 0 on success, negative error code on failure. 01073 */ 01074 int rf_transmit_data(uint8_t *data, uint8_t data_len); 01075 01076 /** 01077 * @brief Receives the RF data. 01078 * 01079 * @param[in] coded_data the address where the received data will be stored. 01080 * @param[in] coded_data_len the length of the data to be received. 01081 * 01082 * @description The coded data is sampled at twice the data rate. The coded data must be filtered. 01083 * @returns 0 on success, negative error code on failure. 01084 */ 01085 int rf_receive_data(uint8_t *coded_data, uint8_t coded_data_len); 01086 01087 /** 01088 * @brief Sets the T/R Pin if defined. 01089 * 01090 * @param[in] pin_state desired pin state. 01091 * 01092 * @returns 0 on success, negative error code on failure. 01093 */ 01094 int set_trx_pin(trx_state_t pin_state); 01095 }; 01096 01097 #endif /* MAX7032_MAX7032_H_ */
Generated on Thu Jul 14 2022 04:50:06 by
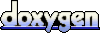